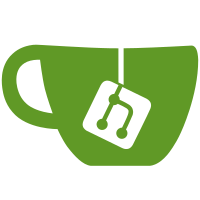
3 changed files with 43 additions and 32 deletions
@ -1,30 +0,0 @@
@@ -1,30 +0,0 @@
|
||||
<?php |
||||
|
||||
class HabrRSSDb |
||||
{ |
||||
protected $hDatabase = null; |
||||
|
||||
public function __construct($dbFile) |
||||
{ |
||||
fclose(fopen($dbFile, 'a')); // create file if not exists |
||||
$this->hDatabase = fopen($dbFile, 'r+'); |
||||
} |
||||
|
||||
public function __destruct() |
||||
{ |
||||
fclose($this->hDatabase); |
||||
} |
||||
|
||||
public function isPublished($id) |
||||
{ |
||||
fseek($this->hDatabase, $id); |
||||
$status = fgetc($this->hDatabase); |
||||
return $status === '1'; |
||||
} |
||||
|
||||
public function setPublished($id) |
||||
{ |
||||
fseek($this->hDatabase, $id); |
||||
fwrite($this->hDatabase, '1'); |
||||
} |
||||
} |
@ -0,0 +1,41 @@
@@ -0,0 +1,41 @@
|
||||
<?php |
||||
|
||||
class RSSDb |
||||
{ |
||||
protected $hDatabase = null; |
||||
protected $minId = 0; |
||||
protected $bits = array(0=>1, 2, 4, 8, 16, 32, 64, 128); |
||||
|
||||
public function __construct($dbFile, $minId = 0) |
||||
{ |
||||
fclose(fopen($dbFile, 'a')); // create file if not exists |
||||
$this->hDatabase = fopen($dbFile, 'r+'); |
||||
$this->minId = $minId; |
||||
} |
||||
|
||||
public function __destruct() |
||||
{ |
||||
fclose($this->hDatabase); |
||||
} |
||||
|
||||
public function isPublished($id) |
||||
{ |
||||
$id -= $this->minId; |
||||
if ($id < 0) return true; |
||||
$pos = intval($id/8); |
||||
fseek($this->hDatabase, $pos); |
||||
$status = ord(fgetc($this->hDatabase)); |
||||
return (bool)($status & $this->bits[$id % 8]); |
||||
} |
||||
|
||||
public function setPublished($id) |
||||
{ |
||||
$id -= $this->minId; |
||||
if ($id < 0) return; |
||||
$pos = intval($id/8); |
||||
fseek($this->hDatabase, $pos); |
||||
$status = ord(fgetc($this->hDatabase)); |
||||
fseek($this->hDatabase, $pos); |
||||
fwrite($this->hDatabase, chr($status | $this->bits[$id % 8])); |
||||
} |
||||
} |
Loading…
Reference in new issue