Browse Source
* Added test for AppImPanelControllerSpec, cleaned up style of all tests All tests give 0 errors when running gulp standard All credits for those test still go to @Ryuno-Ki, I did not functionaly change the testing code * add Test for DocumentModalController * added tests for the EmbedModalController and VideoModalControllermaster
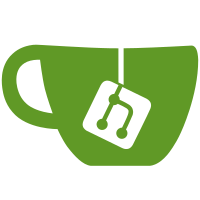
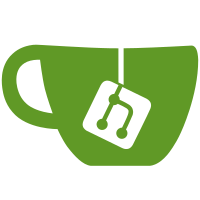
12 changed files with 594 additions and 201 deletions
@ -0,0 +1,21 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach, jasmine */ |
||||||
|
|
||||||
|
describe('AppImPanelController', function () { |
||||||
|
var $scope |
||||||
|
|
||||||
|
beforeEach(module('myApp.controllers')) |
||||||
|
|
||||||
|
beforeEach(function () { |
||||||
|
inject(function (_$controller_, _$rootScope_) { |
||||||
|
$scope = _$rootScope_.$new() |
||||||
|
$scope.$on = jasmine.createSpy('$on') |
||||||
|
_$controller_('AppImPanelController', { $scope: $scope }) |
||||||
|
}) |
||||||
|
}) |
||||||
|
|
||||||
|
// define tests
|
||||||
|
it('sets $on(user_update) to no-operation function', function (done) { |
||||||
|
expect($scope.$on).toHaveBeenCalledWith('user_update', angular.noop) |
||||||
|
done() |
||||||
|
}) |
||||||
|
}) |
@ -1,50 +1,52 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach */ |
||||||
|
|
||||||
describe('AppWelcomeController', function () { |
describe('AppWelcomeController', function () { |
||||||
var $controller, $rootScope, $scope, $location, MtApiManager, ErrorService, |
var $controller, $rootScope, $scope, $location, MtpApiManager, ErrorService, |
||||||
ChangelogNotifyService, LayoutSwitchService; |
ChangelogNotifyService, LayoutSwitchService |
||||||
|
|
||||||
beforeEach(module('myApp.controllers')); |
beforeEach(module('myApp.controllers')) |
||||||
|
|
||||||
beforeEach(function () { |
beforeEach(function () { |
||||||
ChangelogNotifyService = { |
ChangelogNotifyService = { |
||||||
checkUpdate: function () {} |
checkUpdate: function () {} |
||||||
}; |
} |
||||||
|
|
||||||
LayoutSwitchService = { |
LayoutSwitchService = { |
||||||
start: function () {} |
start: function () {} |
||||||
}; |
} |
||||||
|
|
||||||
MtpApiManager = { |
MtpApiManager = { |
||||||
getUserID: function () { |
getUserID: function () { |
||||||
return { |
return { |
||||||
then: function () {} |
then: function () {} |
||||||
}; |
} |
||||||
} |
} |
||||||
}; |
} |
||||||
|
|
||||||
module(function ($provide) { |
module(function ($provide) { |
||||||
$provide.value('MtpApiManager', MtpApiManager); |
$provide.value('MtpApiManager', MtpApiManager) |
||||||
}); |
}) |
||||||
|
|
||||||
inject(function (_$controller_, _$rootScope_, _$location_) { |
inject(function (_$controller_, _$rootScope_, _$location_) { |
||||||
$controller = _$controller_; |
$controller = _$controller_ |
||||||
$rootScope = _$rootScope_; |
$rootScope = _$rootScope_ |
||||||
$location = _$location_; |
$location = _$location_ |
||||||
|
|
||||||
$scope = $rootScope.$new(); |
$scope = $rootScope.$new() |
||||||
$controller('AppWelcomeController', { |
$controller('AppWelcomeController', { |
||||||
$scope: $scope, |
$scope: $scope, |
||||||
$location: $location, |
$location: $location, |
||||||
MtpApiManager: MtpApiManager, |
MtpApiManager: MtpApiManager, |
||||||
ErrorService: ErrorService, |
ErrorService: ErrorService, |
||||||
ChangelogNotifyService: ChangelogNotifyService, |
ChangelogNotifyService: ChangelogNotifyService, |
||||||
LayoutSwitchService: LayoutSwitchService |
LayoutSwitchService: LayoutSwitchService |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
|
||||||
// https://stackoverflow.com/a/36460924
|
// https://stackoverflow.com/a/36460924
|
||||||
it('executes a dummy spec', function (done) { |
it('executes a dummy spec', function (done) { |
||||||
expect(true).toBe(true); |
expect(true).toBe(true) |
||||||
done(); |
done() |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
@ -0,0 +1,132 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach, jasmine */ |
||||||
|
|
||||||
|
describe('DocumentModalController', function () { |
||||||
|
var $controller, $scope, $rootScope, $docManager, $errService, $input, $messManager, $pSelectService, $modalI, createController |
||||||
|
|
||||||
|
beforeEach(module('myApp.controllers')) |
||||||
|
|
||||||
|
beforeEach(function () { |
||||||
|
$docManager = {} |
||||||
|
$docManager.wrapForHistory = jasmine.createSpy('wrapForHistory') |
||||||
|
$docManager.saveDocFile = jasmine.createSpy('saveDocFile') |
||||||
|
|
||||||
|
$input = {} |
||||||
|
$errService = { |
||||||
|
confirm: function (message) { |
||||||
|
$input = message |
||||||
|
return { |
||||||
|
then: function (f) { |
||||||
|
f() |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
$pSelectService = { |
||||||
|
selectPeer: function (options) { |
||||||
|
$input = options |
||||||
|
return { |
||||||
|
then: function (f) { |
||||||
|
f('Peerselected') |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
createController = function (spyBroadcast, spyOn) { |
||||||
|
if (spyBroadcast) { |
||||||
|
$rootScope.$broadcast = jasmine.createSpy('$broadcast') |
||||||
|
} |
||||||
|
if (spyOn) { |
||||||
|
$scope.$on = jasmine.createSpy('$on') |
||||||
|
} |
||||||
|
$controller('DocumentModalController', { |
||||||
|
$scope: $scope, |
||||||
|
$rootScope: $rootScope, |
||||||
|
$modalInstance: $modalI, |
||||||
|
PeersSelectService: $pSelectService, |
||||||
|
AppMessagesManager: $messManager, |
||||||
|
AppDocsManager: $docManager, |
||||||
|
AppPeersManager: {}, |
||||||
|
ErrorService: $errService |
||||||
|
}) |
||||||
|
} |
||||||
|
|
||||||
|
$messManager = {} |
||||||
|
$messManager.deleteMessages = jasmine.createSpy('deleteMessages') |
||||||
|
|
||||||
|
$modalI = {} |
||||||
|
$modalI.dismiss = jasmine.createSpy('dismissModal') |
||||||
|
|
||||||
|
inject(function (_$controller_, _$rootScope_) { |
||||||
|
$rootScope = _$rootScope_ |
||||||
|
$scope = $rootScope.$new() |
||||||
|
$scope.docID = 'randomdoc' |
||||||
|
|
||||||
|
$controller = _$controller_ |
||||||
|
}) |
||||||
|
}) |
||||||
|
|
||||||
|
// define tests
|
||||||
|
it('sets the document in the scope', function (done) { |
||||||
|
createController(false, false) |
||||||
|
|
||||||
|
expect($docManager.wrapForHistory).toHaveBeenCalledWith($scope.docID) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('forwards a message with a document', function (done) { |
||||||
|
createController(true, false) |
||||||
|
$scope.messageID = 'id039' |
||||||
|
|
||||||
|
$scope.forward() |
||||||
|
expect($input).toEqual({canSend: true}) |
||||||
|
expect($scope.$broadcast).toHaveBeenCalledWith('history_focus', { |
||||||
|
peerString: 'Peerselected', |
||||||
|
attachment: { |
||||||
|
_: 'fwd_messages', |
||||||
|
id: [$scope.messageID] |
||||||
|
} |
||||||
|
}) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('deletes a message with a document', function (done) { |
||||||
|
createController(false, false) |
||||||
|
$scope.messageID = 'id123' |
||||||
|
|
||||||
|
$scope.delete() |
||||||
|
expect($input).toEqual({type: 'MESSAGE_DELETE'}) |
||||||
|
expect($messManager.deleteMessages).toHaveBeenCalledWith([$scope.messageID]) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('downloads the document', function (done) { |
||||||
|
createController(false, false) |
||||||
|
|
||||||
|
$scope.download() |
||||||
|
expect($docManager.saveDocFile).toHaveBeenCalledWith($scope.docID) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('delete a document linked to a message', function (done) { |
||||||
|
createController(false, true) |
||||||
|
$scope.messageID = 'id33' |
||||||
|
|
||||||
|
$rootScope.$broadcast('history_delete') |
||||||
|
expect($scope.$on).toHaveBeenCalledWith('history_delete', jasmine.any(Function)) |
||||||
|
expect($modalI.dismiss).not.toHaveBeenCalled() |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('delete a document linked to a modal instance', function (done) { |
||||||
|
createController(false, false) |
||||||
|
$scope.messageID = 'id876' |
||||||
|
|
||||||
|
var $msgs = {} |
||||||
|
$msgs[$scope.messageID] = {message: 'some non-empty message'} |
||||||
|
$rootScope.$broadcast('history_delete', {msgs: $msgs}) |
||||||
|
expect($modalI.dismiss).toHaveBeenCalled() |
||||||
|
done() |
||||||
|
}) |
||||||
|
}) |
@ -0,0 +1,91 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach, jasmine */ |
||||||
|
|
||||||
|
describe('EmbedModalController', function () { |
||||||
|
var $scope, $rootScope, $webpageManager, $errService, $input, $messManager, $pSelectService, $modalI |
||||||
|
|
||||||
|
beforeEach(module('myApp.controllers')) |
||||||
|
|
||||||
|
beforeEach(function () { |
||||||
|
$webpageManager = {} |
||||||
|
$webpageManager.wrapForFull = jasmine.createSpy('wrapForFull') |
||||||
|
|
||||||
|
$input = {} |
||||||
|
$errService = { |
||||||
|
confirm: function (message) { |
||||||
|
$input = message |
||||||
|
return { |
||||||
|
then: function (f) { |
||||||
|
f() |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
$pSelectService = { |
||||||
|
selectPeer: function (options) { |
||||||
|
$input = options |
||||||
|
return { |
||||||
|
then: function (f) { |
||||||
|
f('Peerselected') |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
$messManager = {} |
||||||
|
$messManager.deleteMessages = jasmine.createSpy('deleteMessages') |
||||||
|
|
||||||
|
$modalI = {} |
||||||
|
$modalI.dismiss = jasmine.createSpy('dismissModal') |
||||||
|
|
||||||
|
inject(function (_$controller_, _$rootScope_) { |
||||||
|
$rootScope = _$rootScope_ |
||||||
|
$rootScope.$broadcast = jasmine.createSpy('$broadcast') |
||||||
|
$scope = $rootScope.$new() |
||||||
|
$scope.webpageID = 'www.notRelevant.com' |
||||||
|
_$controller_('EmbedModalController', { |
||||||
|
$q: {}, |
||||||
|
$scope: $scope, |
||||||
|
$rootScope: $rootScope, |
||||||
|
$modalInstance: $modalI, |
||||||
|
PeersSelectService: $pSelectService, |
||||||
|
AppMessagesManager: $messManager, |
||||||
|
AppPeersManager: {}, |
||||||
|
AppPhotosManager: {}, |
||||||
|
AppWebPagesManager: $webpageManager, |
||||||
|
ErrorService: $errService |
||||||
|
}) |
||||||
|
}) |
||||||
|
}) |
||||||
|
|
||||||
|
// define tests
|
||||||
|
it('sets the embeded webpage in the scope', function (done) { |
||||||
|
expect($scope.nav).toEqual({}) |
||||||
|
expect($webpageManager.wrapForFull).toHaveBeenCalledWith($scope.webpageID) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('forwards a message with an embeded link', function (done) { |
||||||
|
$scope.messageID = 'id1234234' |
||||||
|
|
||||||
|
$scope.forward() |
||||||
|
expect($input).toEqual({canSend: true}) |
||||||
|
expect($scope.$broadcast).toHaveBeenCalledWith('history_focus', { |
||||||
|
peerString: 'Peerselected', |
||||||
|
attachment: { |
||||||
|
_: 'fwd_messages', |
||||||
|
id: [$scope.messageID] |
||||||
|
} |
||||||
|
}) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('deletes a message with an embeded link', function (done) { |
||||||
|
$scope.messageID = 'id979565673' |
||||||
|
|
||||||
|
$scope.delete() |
||||||
|
expect($input).toEqual({type: 'MESSAGE_DELETE'}) |
||||||
|
expect($messManager.deleteMessages).toHaveBeenCalledWith([$scope.messageID]) |
||||||
|
done() |
||||||
|
}) |
||||||
|
}) |
@ -1,56 +1,58 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach, jasmine, xit */ |
||||||
|
|
||||||
describe('PhonebookContactsService', function () { |
describe('PhonebookContactsService', function () { |
||||||
var PhonebookContactsService; |
var PhonebookContactsService, $modal |
||||||
|
|
||||||
beforeEach(module('ui.bootstrap')); |
beforeEach(module('ui.bootstrap')) |
||||||
beforeEach(module('myApp.services')); |
beforeEach(module('myApp.services')) |
||||||
|
|
||||||
beforeEach(inject(function (_PhonebookContactsService_) { |
beforeEach(inject(function (_PhonebookContactsService_) { |
||||||
PhonebookContactsService = _PhonebookContactsService_; |
PhonebookContactsService = _PhonebookContactsService_ |
||||||
})); |
})) |
||||||
|
|
||||||
describe('Public API:', function () { |
describe('Public API:', function () { |
||||||
it('checks availability', function () { |
it('checks availability', function () { |
||||||
expect(PhonebookContactsService.isAvailable).toBeDefined(); |
expect(PhonebookContactsService.isAvailable).toBeDefined() |
||||||
}); |
}) |
||||||
|
|
||||||
it('open the phonebook for import', function () { |
it('open the phonebook for import', function () { |
||||||
expect(PhonebookContactsService.openPhonebookImport).toBeDefined(); |
expect(PhonebookContactsService.openPhonebookImport).toBeDefined() |
||||||
}); |
}) |
||||||
|
|
||||||
it('get phonebook contacts', function () { |
it('get phonebook contacts', function () { |
||||||
expect(PhonebookContactsService.getPhonebookContacts).toBeDefined(); |
expect(PhonebookContactsService.getPhonebookContacts).toBeDefined() |
||||||
}); |
}) |
||||||
|
|
||||||
describe('usage', function () { |
describe('usage', function () { |
||||||
describe('of isAvailable()', function () { |
describe('of isAvailable()', function () { |
||||||
it('returns false in most cases', function (done) { |
it('returns false in most cases', function (done) { |
||||||
expect(PhonebookContactsService.isAvailable()).toBe(false); |
expect(PhonebookContactsService.isAvailable()).toBe(false) |
||||||
done(); |
done() |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
|
||||||
describe('of openPhonebookImport()', function () { |
describe('of openPhonebookImport()', function () { |
||||||
beforeEach(function() { |
beforeEach(function () { |
||||||
$modal = { |
$modal = { |
||||||
open: jasmine.createSpy('open') |
open: jasmine.createSpy('open') |
||||||
}; |
} |
||||||
}); |
}) |
||||||
|
|
||||||
xit('opens a modal', function () { |
xit('opens a modal', function () { |
||||||
PhonebookContactsService.openPhonebookImport(); |
PhonebookContactsService.openPhonebookImport() |
||||||
expect($modal.open).toHaveBeenCalled(); |
expect($modal.open).toHaveBeenCalled() |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
|
||||||
describe('of getPhonebookContacts()', function () { |
describe('of getPhonebookContacts()', function () { |
||||||
xit('will get rejected in most cases', function (done) { |
xit('will get rejected in most cases', function (done) { |
||||||
promise = PhonebookContactsService.getPhonebookContacts(); |
var promise = PhonebookContactsService.getPhonebookContacts() |
||||||
promise.finally(function () { |
promise.finally(function () { |
||||||
expect(promise.isFullfilled()).toBe(true); |
expect(promise.isFullfilled()).toBe(true) |
||||||
done(); |
done() |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
@ -0,0 +1,134 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach, jasmine */ |
||||||
|
|
||||||
|
describe('VideoModalController', function () { |
||||||
|
var $controller, $scope, $rootScope, $docManager, $errService, $input, $messManager, $pSelectService, $modalI, createController |
||||||
|
|
||||||
|
beforeEach(module('myApp.controllers')) |
||||||
|
|
||||||
|
beforeEach(function () { |
||||||
|
$docManager = {} |
||||||
|
$docManager.wrapVideoForFull = jasmine.createSpy('wrapVideoForFull') |
||||||
|
$docManager.saveDocFile = jasmine.createSpy('saveDocFile') |
||||||
|
|
||||||
|
$input = {} |
||||||
|
$errService = { |
||||||
|
confirm: function (message) { |
||||||
|
$input = message |
||||||
|
return { |
||||||
|
then: function (f) { |
||||||
|
f() |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
$pSelectService = { |
||||||
|
selectPeer: function (options) { |
||||||
|
$input = options |
||||||
|
return { |
||||||
|
then: function (f) { |
||||||
|
f('Peerselected') |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
createController = function (spyBroadcast, spyOn) { |
||||||
|
if (spyBroadcast) { |
||||||
|
$rootScope.$broadcast = jasmine.createSpy('$broadcast') |
||||||
|
} |
||||||
|
if (spyOn) { |
||||||
|
$scope.$on = jasmine.createSpy('$on') |
||||||
|
} |
||||||
|
$controller('VideoModalController', { |
||||||
|
$scope: $scope, |
||||||
|
$rootScope: $rootScope, |
||||||
|
$modalInstance: $modalI, |
||||||
|
PeersSelectService: $pSelectService, |
||||||
|
AppMessagesManager: $messManager, |
||||||
|
AppDocsManager: $docManager, |
||||||
|
AppPeersManager: {}, |
||||||
|
ErrorService: $errService |
||||||
|
}) |
||||||
|
} |
||||||
|
|
||||||
|
$messManager = {} |
||||||
|
$messManager.deleteMessages = jasmine.createSpy('deleteMessages') |
||||||
|
|
||||||
|
$modalI = {} |
||||||
|
$modalI.dismiss = jasmine.createSpy('dismissModal') |
||||||
|
|
||||||
|
inject(function (_$controller_, _$rootScope_) { |
||||||
|
$rootScope = _$rootScope_ |
||||||
|
$scope = $rootScope.$new() |
||||||
|
$scope.docID = 'randomvideo' |
||||||
|
|
||||||
|
$controller = _$controller_ |
||||||
|
}) |
||||||
|
}) |
||||||
|
|
||||||
|
// define tests
|
||||||
|
it('sets the video in the scope', function (done) { |
||||||
|
createController(false, false) |
||||||
|
|
||||||
|
expect($scope.progress).toEqual({enabled: false}) |
||||||
|
expect($scope.player).toEqual({}) |
||||||
|
expect($docManager.wrapVideoForFull).toHaveBeenCalledWith($scope.docID) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('forwards a message with a video', function (done) { |
||||||
|
createController(true, false) |
||||||
|
$scope.messageID = 'id68567' |
||||||
|
|
||||||
|
$scope.forward() |
||||||
|
expect($input).toEqual({canSend: true}) |
||||||
|
expect($scope.$broadcast).toHaveBeenCalledWith('history_focus', { |
||||||
|
peerString: 'Peerselected', |
||||||
|
attachment: { |
||||||
|
_: 'fwd_messages', |
||||||
|
id: [$scope.messageID] |
||||||
|
} |
||||||
|
}) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('deletes a message with a video', function (done) { |
||||||
|
createController(false, false) |
||||||
|
$scope.messageID = 'id235235' |
||||||
|
|
||||||
|
$scope.delete() |
||||||
|
expect($input).toEqual({type: 'MESSAGE_DELETE'}) |
||||||
|
expect($messManager.deleteMessages).toHaveBeenCalledWith([$scope.messageID]) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('downloads the document (video)', function (done) { |
||||||
|
createController(false, false) |
||||||
|
|
||||||
|
$scope.download() |
||||||
|
expect($docManager.saveDocFile).toHaveBeenCalledWith($scope.docID) |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('delete a video linked to a message', function (done) { |
||||||
|
createController(false, true) |
||||||
|
$scope.messageID = 'id2352' |
||||||
|
|
||||||
|
$rootScope.$broadcast('history_delete') |
||||||
|
expect($scope.$on).toHaveBeenCalledWith('history_delete', jasmine.any(Function)) |
||||||
|
expect($modalI.dismiss).not.toHaveBeenCalled() |
||||||
|
done() |
||||||
|
}) |
||||||
|
|
||||||
|
it('delete a video linked to a modal instance', function (done) { |
||||||
|
createController(false, false) |
||||||
|
$scope.messageID = 'id6234' |
||||||
|
|
||||||
|
var $msgs = {} |
||||||
|
$msgs[$scope.messageID] = {message: 'some non-empty message'} |
||||||
|
$rootScope.$broadcast('history_delete', {msgs: $msgs}) |
||||||
|
expect($modalI.dismiss).toHaveBeenCalled() |
||||||
|
done() |
||||||
|
}) |
||||||
|
}) |
@ -1,31 +1,33 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach */ |
||||||
|
|
||||||
describe('chatTitle filter', function () { |
describe('chatTitle filter', function () { |
||||||
var $filter, _, chatTitleFilter; |
var $filter, _, chatTitleFilter |
||||||
|
|
||||||
beforeEach(module('myApp.filters')); |
beforeEach(module('myApp.filters')) |
||||||
|
|
||||||
beforeEach(inject(function (_$filter_, ___) { |
beforeEach(inject(function (_$filter_, ___) { |
||||||
$filter = _$filter_; |
$filter = _$filter_ |
||||||
_ = ___; |
_ = ___ |
||||||
})); |
})) |
||||||
|
|
||||||
beforeEach(function () { |
beforeEach(function () { |
||||||
chatTitleFilter = $filter('chatTitle'); |
chatTitleFilter = $filter('chatTitle') |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays chat title deleted', function () { |
it('displays chat title deleted', function () { |
||||||
var expected = _('chat_title_deleted'); |
var expected = _('chat_title_deleted') |
||||||
var actual = chatTitleFilter(null); |
var actual = chatTitleFilter(null) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
expect(actual).toEqual(expected) |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays the chat title', function () { |
it('displays the chat title', function () { |
||||||
var chat = { |
var chat = { |
||||||
title: 'Telegraph is hot!' |
title: 'Telegraph is hot!' |
||||||
}; |
} |
||||||
var expected = chat.title; |
var expected = chat.title |
||||||
var actual = chatTitleFilter(chat); |
var actual = chatTitleFilter(chat) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
expect(actual).toEqual(expected) |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
@ -1,23 +1,25 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach */ |
||||||
|
|
||||||
describe('myHead directive', function () { |
describe('myHead directive', function () { |
||||||
var $compile, $rootScope; |
var $compile, $rootScope |
||||||
|
|
||||||
beforeEach(module('myApp.templates')); |
beforeEach(module('myApp.templates')) |
||||||
beforeEach(module('myApp.directives')); |
beforeEach(module('myApp.directives')) |
||||||
|
|
||||||
beforeEach(inject(function (_$compile_, _$rootScope_) { |
beforeEach(inject(function (_$compile_, _$rootScope_) { |
||||||
$compile = _$compile_; |
$compile = _$compile_ |
||||||
$rootScope = _$rootScope_; |
$rootScope = _$rootScope_ |
||||||
})); |
})) |
||||||
|
|
||||||
it('compiles a my-head attribute', function () { |
it('compiles a my-head attribute', function () { |
||||||
var compiledElement = $compile('<div my-head></div>')($rootScope); |
var compiledElement = $compile('<div my-head></div>')($rootScope) |
||||||
$rootScope.$digest(); // Fire watchers
|
$rootScope.$digest() // Fire watchers
|
||||||
expect(compiledElement.html()).toContain('tg_page_head'); |
expect(compiledElement.html()).toContain('tg_page_head') |
||||||
}); |
}) |
||||||
|
|
||||||
it('compiles a my-head element', function () { |
it('compiles a my-head element', function () { |
||||||
var compiledElement = $compile('<my-head></my-head>')($rootScope); |
var compiledElement = $compile('<my-head></my-head>')($rootScope) |
||||||
$rootScope.$digest(); // Fire watchers
|
$rootScope.$digest() // Fire watchers
|
||||||
expect(compiledElement.html()).toContain('tg_page_head'); |
expect(compiledElement.html()).toContain('tg_page_head') |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
@ -1,30 +1,32 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach */ |
||||||
|
|
||||||
describe('myLangFooter directive', function () { |
describe('myLangFooter directive', function () { |
||||||
var $compile, $rootScope; |
var $compile, $rootScope |
||||||
|
|
||||||
beforeEach(module('ui.bootstrap')); |
beforeEach(module('ui.bootstrap')) |
||||||
beforeEach(module('myApp.templates')); |
beforeEach(module('myApp.templates')) |
||||||
// ErrorServiceProvider in myApp.services is needed by
|
// ErrorServiceProvider in myApp.services is needed by
|
||||||
// AppLangSelectController in myApp.controllers
|
// AppLangSelectController in myApp.controllers
|
||||||
beforeEach(module('myApp.services')); |
beforeEach(module('myApp.services')) |
||||||
beforeEach(module('myApp.controllers')); |
beforeEach(module('myApp.controllers')) |
||||||
beforeEach(module('myApp.directives')); |
beforeEach(module('myApp.directives')) |
||||||
|
|
||||||
beforeEach(inject(function (_$compile_, _$rootScope_) { |
beforeEach(inject(function (_$compile_, _$rootScope_) { |
||||||
$compile = _$compile_; |
$compile = _$compile_ |
||||||
$rootScope = _$rootScope_; |
$rootScope = _$rootScope_ |
||||||
})); |
})) |
||||||
|
|
||||||
it('compiles a my-lang-footer attribute', function () { |
it('compiles a my-lang-footer attribute', function () { |
||||||
var compiledElement = $compile('<div my-lang-footer></div>')($rootScope); |
var compiledElement = $compile('<div my-lang-footer></div>')($rootScope) |
||||||
$rootScope.$digest(); // Fire watchers
|
$rootScope.$digest() // Fire watchers
|
||||||
expect(compiledElement.html()).toContain('footer_lang_link'); |
expect(compiledElement.html()).toContain('footer_lang_link') |
||||||
expect(compiledElement.html()).toContain('AppLangSelectController'); |
expect(compiledElement.html()).toContain('AppLangSelectController') |
||||||
}); |
}) |
||||||
|
|
||||||
it('compiles a my-lang-footer element', function () { |
it('compiles a my-lang-footer element', function () { |
||||||
var compiledElement = $compile('<my-lang-footer></my-lang-footer>')($rootScope); |
var compiledElement = $compile('<my-lang-footer></my-lang-footer>')($rootScope) |
||||||
$rootScope.$digest(); // Fire watchers
|
$rootScope.$digest() // Fire watchers
|
||||||
expect(compiledElement.html()).toContain('footer_lang_link'); |
expect(compiledElement.html()).toContain('footer_lang_link') |
||||||
expect(compiledElement.html()).toContain('AppLangSelectController'); |
expect(compiledElement.html()).toContain('AppLangSelectController') |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
@ -1,42 +1,43 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach */ |
||||||
|
|
||||||
describe('userFirstName filter', function () { |
describe('userFirstName filter', function () { |
||||||
var $filter, _, userFirstNameFilter; |
var $filter, _, userFirstNameFilter |
||||||
|
|
||||||
beforeEach(module('myApp.filters')); |
beforeEach(module('myApp.filters')) |
||||||
|
|
||||||
beforeEach(inject(function (_$filter_, ___) { |
beforeEach(inject(function (_$filter_, ___) { |
||||||
$filter = _$filter_; |
$filter = _$filter_ |
||||||
_ = ___; |
_ = ___ |
||||||
})); |
})) |
||||||
|
|
||||||
beforeEach(function () { |
beforeEach(function () { |
||||||
userFirstNameFilter = $filter('userFirstName'); |
userFirstNameFilter = $filter('userFirstName') |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays user first name deleted', function () { |
it('displays user first name deleted', function () { |
||||||
var expected = _('user_first_name_deleted'); |
var expected = _('user_first_name_deleted') |
||||||
var actual = userFirstNameFilter(null); |
var actual = userFirstNameFilter(null) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
expect(actual).toEqual(expected) |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays the first name', function () { |
it('displays the first name', function () { |
||||||
var user = { |
var user = { |
||||||
first_name: 'John' |
first_name: 'John' |
||||||
}; |
} |
||||||
var expected = user.first_name; |
var expected = user.first_name |
||||||
var actual = userFirstNameFilter(user); |
var actual = userFirstNameFilter(user) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
expect(actual).toEqual(expected) |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays the last name alternatively', function () { |
it('displays the last name alternatively', function () { |
||||||
var user = { |
var user = { |
||||||
last_name: 'Doe' |
last_name: 'Doe' |
||||||
}; |
} |
||||||
var expected = user.last_name; |
var expected = user.last_name |
||||||
var actual = userFirstNameFilter(user); |
var actual = userFirstNameFilter(user) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
|
||||||
}); |
|
||||||
|
|
||||||
}); |
expect(actual).toEqual(expected) |
||||||
|
}) |
||||||
|
}) |
||||||
|
@ -1,42 +1,44 @@ |
|||||||
|
/* global describe, it, inject, expect, beforeEach */ |
||||||
|
|
||||||
describe('userName filter', function () { |
describe('userName filter', function () { |
||||||
var $filter, _, userNameFilter; |
var $filter, _, userNameFilter |
||||||
|
|
||||||
beforeEach(module('myApp.filters')); |
beforeEach(module('myApp.filters')) |
||||||
|
|
||||||
beforeEach(inject(function (_$filter_, ___) { |
beforeEach(inject(function (_$filter_, ___) { |
||||||
$filter = _$filter_; |
$filter = _$filter_ |
||||||
_ = ___; |
_ = ___ |
||||||
})); |
})) |
||||||
|
|
||||||
beforeEach(function () { |
beforeEach(function () { |
||||||
userNameFilter = $filter('userName'); |
userNameFilter = $filter('userName') |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays user name deleted', function () { |
it('displays user name deleted', function () { |
||||||
var expected = _('user_name_deleted'); |
var expected = _('user_name_deleted') |
||||||
var actual = userNameFilter(null); |
var actual = userNameFilter(null) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
expect(actual).toEqual(expected) |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays the first name', function () { |
it('displays the first name', function () { |
||||||
var user = { |
var user = { |
||||||
first_name: 'John' |
first_name: 'John' |
||||||
}; |
} |
||||||
var expected = user.first_name; |
var expected = user.first_name |
||||||
var actual = userNameFilter(user); |
var actual = userNameFilter(user) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
expect(actual).toEqual(expected) |
||||||
}); |
}) |
||||||
|
|
||||||
it('displays both, the first and the last name', function () { |
it('displays both, the first and the last name', function () { |
||||||
var user = { |
var user = { |
||||||
first_name: 'John', |
first_name: 'John', |
||||||
last_name: 'Doe' |
last_name: 'Doe' |
||||||
}; |
} |
||||||
var expected = user.first_name + ' ' + user.last_name; |
var expected = user.first_name + ' ' + user.last_name |
||||||
var actual = userNameFilter(user); |
var actual = userNameFilter(user) |
||||||
|
|
||||||
expect(actual).toEqual(expected); |
expect(actual).toEqual(expected) |
||||||
}); |
}) |
||||||
}); |
}) |
||||||
|
Loading…
Reference in new issue