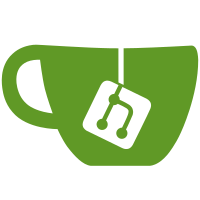
8 changed files with 88 additions and 86 deletions
@ -1,79 +0,0 @@ |
|||||||
/* |
|
||||||
* https://github.com/morethanwords/tweb
|
|
||||||
* Copyright (C) 2019-2021 Eduard Kuzmenko |
|
||||||
* https://github.com/morethanwords/tweb/blob/master/LICENSE
|
|
||||||
* |
|
||||||
* Originally from: |
|
||||||
* https://github.com/zhukov/webogram
|
|
||||||
* Copyright (C) 2014 Igor Zhukov <igor.beatle@gmail.com> |
|
||||||
* https://github.com/zhukov/webogram/blob/master/LICENSE
|
|
||||||
*/ |
|
||||||
|
|
||||||
/* import PasswordManager from './passwordManager'; |
|
||||||
import DcConfigurator from './dcConfigurator'; |
|
||||||
import RSAKeysManager from './rsaKeysManager'; |
|
||||||
import TimeManager from './timeManager'; |
|
||||||
import ServerTimeManager from './serverTimeManager'; |
|
||||||
import Authorizer from './authorizer'; |
|
||||||
import NetworkerFactory from './networkerFactory'; |
|
||||||
import ApiManager from './apiManager'; |
|
||||||
import ApiFileManager from './apiFileManager'; */ |
|
||||||
|
|
||||||
/* export class TelegramMeWebService { |
|
||||||
public disabled = Modes.test || |
|
||||||
App.domains.indexOf(location.hostname) === -1 || |
|
||||||
location.protocol !== 'http:' && location.protocol !== 'https:' || |
|
||||||
location.protocol === 'https:' && location.hostname !== 'web.telegram.org'; |
|
||||||
|
|
||||||
public setAuthorized(canRedirect: boolean) { |
|
||||||
if(this.disabled) { |
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
sessionStorage.get('tgme_sync').then((curValue) => { |
|
||||||
var ts = Date.now() / 1000; |
|
||||||
if(canRedirect && |
|
||||||
curValue && |
|
||||||
curValue.canRedirect === canRedirect && |
|
||||||
curValue.ts + 86400 > ts) { |
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
sessionStorage.set({tgme_sync: {canRedirect: canRedirect, ts: ts}}); |
|
||||||
|
|
||||||
var urls = [ |
|
||||||
'//telegram.me/_websync_?authed=' + (canRedirect ? '1' : '0'), |
|
||||||
'//t.me/_websync_?authed=' + (canRedirect ? '1' : '0') |
|
||||||
]; |
|
||||||
|
|
||||||
urls.forEach(url => { |
|
||||||
let script = document.createElement('script'); |
|
||||||
script.onload = script.onerror = function() { |
|
||||||
script.remove(); |
|
||||||
}; |
|
||||||
script.src = url; |
|
||||||
document.body.appendChild(script); |
|
||||||
}); |
|
||||||
}); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
export const telegramMeWebService = new TelegramMeWebService(); */ |
|
||||||
|
|
||||||
/* export namespace MTProto { |
|
||||||
//$($window).on('click keydown', rng_seed_time); // WARNING!
|
|
||||||
|
|
||||||
export const passwordManager = PasswordManager; |
|
||||||
export const dcConfigurator = DcConfigurator; |
|
||||||
export const rsaKeysManager = RSAKeysManager; |
|
||||||
export const timeManager = TimeManager; |
|
||||||
export const authorizer = Authorizer; |
|
||||||
export const networkerFactory = NetworkerFactory; |
|
||||||
export const apiManager = ApiManager; |
|
||||||
export const apiFileManager = ApiFileManager; |
|
||||||
export const serverTimeManager = ServerTimeManager; |
|
||||||
} |
|
||||||
|
|
||||||
//(window as any).MTProto = MTProto; */
|
|
||||||
|
|
||||||
export default null; |
|
@ -0,0 +1,67 @@ |
|||||||
|
/* |
||||||
|
* https://github.com/morethanwords/tweb
|
||||||
|
* Copyright (C) 2019-2021 Eduard Kuzmenko |
||||||
|
* https://github.com/morethanwords/tweb/blob/master/LICENSE
|
||||||
|
* |
||||||
|
* Originally from: |
||||||
|
* https://github.com/zhukov/webogram
|
||||||
|
* Copyright (C) 2014 Igor Zhukov <igor.beatle@gmail.com> |
||||||
|
* https://github.com/zhukov/webogram/blob/master/LICENSE
|
||||||
|
*/ |
||||||
|
|
||||||
|
import App from "../../config/app"; |
||||||
|
import { MOUNT_CLASS_TO } from "../../config/debug"; |
||||||
|
import Modes from "../../config/modes"; |
||||||
|
import { tsNow } from "../../helpers/date"; |
||||||
|
import sessionStorage from '../sessionStorage'; |
||||||
|
|
||||||
|
export class TelegramMeWebManager { |
||||||
|
private disabled = /* false && */(Modes.test || App.domains.indexOf(location.hostname) === -1); |
||||||
|
|
||||||
|
public async setAuthorized(canRedirect: boolean) { |
||||||
|
if(this.disabled) { |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
sessionStorage.get('tgme_sync').then((curValue) => { |
||||||
|
const ts = tsNow(true); |
||||||
|
if(canRedirect && |
||||||
|
curValue && |
||||||
|
curValue.canRedirect === canRedirect && |
||||||
|
(curValue.ts + 86400) > ts) { |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
sessionStorage.set({ |
||||||
|
tgme_sync: { |
||||||
|
canRedirect, |
||||||
|
ts |
||||||
|
} |
||||||
|
}); |
||||||
|
|
||||||
|
const urls = [ |
||||||
|
'//telegram.me/_websync_?authed=' + (canRedirect ? '1' : '0'), |
||||||
|
'//t.me/_websync_?authed=' + (canRedirect ? '1' : '0') |
||||||
|
]; |
||||||
|
|
||||||
|
const promises = urls.map(url => { |
||||||
|
const script = document.createElement('script'); |
||||||
|
const promise = new Promise<void>((resolve) => { |
||||||
|
script.onload = script.onerror = () => { |
||||||
|
script.remove(); |
||||||
|
resolve(); |
||||||
|
}; |
||||||
|
}); |
||||||
|
script.src = url; |
||||||
|
document.body.appendChild(script); |
||||||
|
return promise; |
||||||
|
}); |
||||||
|
|
||||||
|
return Promise.all(promises); |
||||||
|
}); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
const telegramMeWebManager = new TelegramMeWebManager(); |
||||||
|
MOUNT_CLASS_TO && (MOUNT_CLASS_TO.telegramMeWebManager = telegramMeWebManager); |
||||||
|
export default telegramMeWebManager; |
Loading…
Reference in new issue