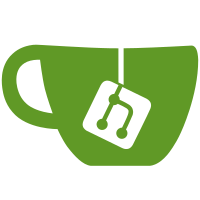
42 changed files with 549 additions and 460 deletions
@ -0,0 +1,61 @@ |
|||||||
|
import { SettingSection } from "../.."; |
||||||
|
import { attachClickEvent } from "../../../../helpers/dom"; |
||||||
|
import appStickersManager from "../../../../lib/appManagers/appStickersManager"; |
||||||
|
import Button from "../../../button"; |
||||||
|
import SidebarSlider, { SliderSuperTab } from "../../../slider"; |
||||||
|
import { wrapSticker } from "../../../wrappers"; |
||||||
|
|
||||||
|
export default class AppTwoStepVerificationSetTab extends SliderSuperTab { |
||||||
|
constructor(slider: SidebarSlider) { |
||||||
|
super(slider, true); |
||||||
|
} |
||||||
|
|
||||||
|
protected init() { |
||||||
|
this.container.classList.add('two-step-verification', 'two-step-verification-set'); |
||||||
|
this.title.innerHTML = 'Password Set!'; |
||||||
|
|
||||||
|
const section = new SettingSection({ |
||||||
|
caption: 'This password will be required when you log in on a new device in addition to the code you get via SMS.', |
||||||
|
noDelimiter: true |
||||||
|
}); |
||||||
|
|
||||||
|
const emoji = '🥳'; |
||||||
|
const doc = appStickersManager.getAnimatedEmojiSticker(emoji); |
||||||
|
const stickerContainer = document.createElement('div'); |
||||||
|
|
||||||
|
if(doc) { |
||||||
|
wrapSticker({ |
||||||
|
doc, |
||||||
|
div: stickerContainer, |
||||||
|
loop: false, |
||||||
|
play: true, |
||||||
|
width: 160, |
||||||
|
height: 160, |
||||||
|
emoji |
||||||
|
}).then(() => { |
||||||
|
// this.animation = player;
|
||||||
|
}); |
||||||
|
} else { |
||||||
|
stickerContainer.classList.add('media-sticker-wrapper'); |
||||||
|
} |
||||||
|
|
||||||
|
section.content.append(stickerContainer); |
||||||
|
|
||||||
|
const inputContent = section.generateContentElement(); |
||||||
|
|
||||||
|
const inputWrapper = document.createElement('div'); |
||||||
|
inputWrapper.classList.add('input-wrapper'); |
||||||
|
|
||||||
|
const btnReturn = Button('btn-primary btn-color-primary', {text: 'RETURN TO SETTINGS'}); |
||||||
|
|
||||||
|
attachClickEvent(btnReturn, (e) => { |
||||||
|
|
||||||
|
}); |
||||||
|
|
||||||
|
inputWrapper.append(btnReturn); |
||||||
|
|
||||||
|
inputContent.append(inputWrapper); |
||||||
|
|
||||||
|
this.scrollable.container.append(section.container); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,77 @@ |
|||||||
|
import ButtonIcon from "./buttonIcon"; |
||||||
|
import Scrollable from "./scrollable"; |
||||||
|
import SidebarSlider from "./slider"; |
||||||
|
|
||||||
|
export interface SliderTab { |
||||||
|
onOpen?: () => void, |
||||||
|
onOpenAfterTimeout?: () => void, |
||||||
|
onClose?: () => void, |
||||||
|
onCloseAfterTimeout?: () => void |
||||||
|
} |
||||||
|
|
||||||
|
export default class SliderSuperTab implements SliderTab { |
||||||
|
public container: HTMLElement; |
||||||
|
|
||||||
|
public header: HTMLElement; |
||||||
|
public closeBtn: HTMLElement; |
||||||
|
public title: HTMLElement; |
||||||
|
|
||||||
|
public content: HTMLElement; |
||||||
|
public scrollable: Scrollable; |
||||||
|
|
||||||
|
constructor(protected slider: SidebarSlider, protected destroyable = false) { |
||||||
|
this.container = document.createElement('div'); |
||||||
|
this.container.classList.add('sidebar-slider-item'); |
||||||
|
|
||||||
|
// * Header
|
||||||
|
this.header = document.createElement('div'); |
||||||
|
this.header.classList.add('sidebar-header'); |
||||||
|
|
||||||
|
this.closeBtn = ButtonIcon('arrow_back sidebar-close-button', {noRipple: true}); |
||||||
|
this.title = document.createElement('div'); |
||||||
|
this.title.classList.add('sidebar-header__title'); |
||||||
|
this.header.append(this.closeBtn, this.title); |
||||||
|
|
||||||
|
// * Content
|
||||||
|
this.content = document.createElement('div'); |
||||||
|
this.content.classList.add('sidebar-content'); |
||||||
|
|
||||||
|
this.scrollable = new Scrollable(this.content, undefined, undefined, true); |
||||||
|
|
||||||
|
this.container.append(this.header, this.content); |
||||||
|
|
||||||
|
this.slider.addTab(this); |
||||||
|
} |
||||||
|
|
||||||
|
public close() { |
||||||
|
return this.slider.closeTab(this); |
||||||
|
} |
||||||
|
|
||||||
|
public async open(...args: any[]) { |
||||||
|
if(this.init) { |
||||||
|
const result = this.init(); |
||||||
|
this.init = null; |
||||||
|
if(result instanceof Promise) { |
||||||
|
await result; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
return this.slider.selectTab(this); |
||||||
|
} |
||||||
|
|
||||||
|
protected init(): Promise<any> | any { |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
// * fix incompability
|
||||||
|
public onOpen() { |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
public onCloseAfterTimeout() { |
||||||
|
if(this.destroyable) { // ! WARNING, пока что это будет работать только с самой последней внутренней вкладкой !
|
||||||
|
this.slider.tabs.delete(this); |
||||||
|
this.container.remove(); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
Loading…
Reference in new issue