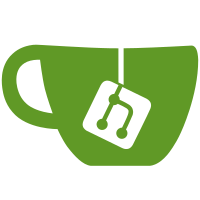
11 changed files with 298 additions and 218 deletions
@ -0,0 +1,61 @@ |
|||||||
|
import mediaSizes from "../../helpers/mediaSizes"; |
||||||
|
import appImManager from "../../lib/appManagers/appImManager"; |
||||||
|
import { cancelEvent } from "../../lib/utils"; |
||||||
|
import DivAndCaption from "../divAndCaption"; |
||||||
|
|
||||||
|
const CLASSNAME_SHOWN_MAIN = 'is-pinned-message-shown'; |
||||||
|
const CLASSNAME_BASE = 'pinned-container'; |
||||||
|
const HEIGHT = 52; |
||||||
|
|
||||||
|
export default class PinnedContainer { |
||||||
|
private close: HTMLElement; |
||||||
|
|
||||||
|
constructor(protected className: string, public divAndCaption: DivAndCaption<(title: string, subtitle: string, message?: any) => void>, onClose?: () => void | Promise<boolean>) { |
||||||
|
/* const prev = this.divAndCaption.fill; |
||||||
|
this.divAndCaption.fill = (mid, title, subtitle) => { |
||||||
|
this.divAndCaption.container.dataset.mid = '' + mid; |
||||||
|
prev(mid, title, subtitle); |
||||||
|
}; */ |
||||||
|
|
||||||
|
divAndCaption.container.classList.add(CLASSNAME_BASE, 'hide'); |
||||||
|
divAndCaption.title.classList.add(CLASSNAME_BASE + '-title'); |
||||||
|
divAndCaption.subtitle.classList.add(CLASSNAME_BASE + '-subtitle'); |
||||||
|
|
||||||
|
this.close = document.createElement('button'); |
||||||
|
this.close.classList.add(CLASSNAME_BASE + '-close', `pinned-${className}-close`, 'btn-icon', 'tgico-close'); |
||||||
|
|
||||||
|
divAndCaption.container.append(this.close); |
||||||
|
|
||||||
|
this.close.addEventListener('click', (e) => { |
||||||
|
cancelEvent(e); |
||||||
|
|
||||||
|
((onClose ? onClose() : null) || Promise.resolve(true)).then(needClose => { |
||||||
|
if(needClose) { |
||||||
|
this.toggle(true); |
||||||
|
} |
||||||
|
}); |
||||||
|
}); |
||||||
|
} |
||||||
|
|
||||||
|
public toggle(hide?: boolean) { |
||||||
|
const isHidden = this.divAndCaption.container.classList.contains('hide'); |
||||||
|
if(hide === undefined) { |
||||||
|
hide = !isHidden; |
||||||
|
} else if(hide == isHidden) { |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
const scrollTop = mediaSizes.isMobile ? appImManager.scrollable.scrollTop : undefined; |
||||||
|
this.divAndCaption.container.classList.toggle('hide', hide); |
||||||
|
appImManager.topbar.classList.toggle(`is-pinned-${this.className}-shown`, !hide); |
||||||
|
|
||||||
|
if(scrollTop !== undefined && !appImManager.topbar.classList.contains(CLASSNAME_SHOWN_MAIN)) { |
||||||
|
appImManager.scrollable.scrollTop = scrollTop + ((hide ? -1 : 1) * HEIGHT); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public fill(title: string, subtitle: string, message: any) { |
||||||
|
this.divAndCaption.container.dataset.mid = '' + message.mid; |
||||||
|
this.divAndCaption.fill(title, subtitle, message); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,59 @@ |
|||||||
|
import appPhotosManager from "../../lib/appManagers/appPhotosManager"; |
||||||
|
import { RichTextProcessor } from "../../lib/richtextprocessor"; |
||||||
|
import DivAndCaption from "../divAndCaption"; |
||||||
|
import { renderImageFromUrl } from "../misc"; |
||||||
|
|
||||||
|
export default class ReplyContainer extends DivAndCaption<(title: string, subtitle: string, message?: any) => void> { |
||||||
|
private mediaEl: HTMLElement; |
||||||
|
|
||||||
|
constructor(protected className: string) { |
||||||
|
super(className, (title: string, subtitle: string = '', message?: any) => { |
||||||
|
if(title.length > 150) { |
||||||
|
title = title.substr(0, 140) + '...'; |
||||||
|
} |
||||||
|
|
||||||
|
if(subtitle.length > 150) { |
||||||
|
subtitle = subtitle.substr(0, 140) + '...'; |
||||||
|
} |
||||||
|
|
||||||
|
title = title ? RichTextProcessor.wrapEmojiText(title) : ''; |
||||||
|
|
||||||
|
if(this.mediaEl) { |
||||||
|
this.mediaEl.remove(); |
||||||
|
this.container.classList.remove('is-media'); |
||||||
|
} |
||||||
|
|
||||||
|
const media = message && message.media; |
||||||
|
if(media) { |
||||||
|
subtitle = message.rReply; |
||||||
|
|
||||||
|
//console.log('wrap reply', media);
|
||||||
|
|
||||||
|
if(media.photo || (media.document && ['video'].indexOf(media.document.type) !== -1)) { |
||||||
|
let replyMedia = document.createElement('div'); |
||||||
|
replyMedia.classList.add(this.className + '-media'); |
||||||
|
|
||||||
|
let photo = media.photo || media.document; |
||||||
|
|
||||||
|
let sizes = photo.sizes || photo.thumbs; |
||||||
|
if(sizes && sizes[0].bytes) { |
||||||
|
appPhotosManager.setAttachmentPreview(sizes[0].bytes, replyMedia, false, true); |
||||||
|
} |
||||||
|
|
||||||
|
appPhotosManager.preloadPhoto(photo, appPhotosManager.choosePhotoSize(photo, 32, 32)) |
||||||
|
.then(() => { |
||||||
|
renderImageFromUrl(replyMedia, photo._ == 'photo' ? photo.url : appPhotosManager.getDocumentCachedThumb(photo.id).url); |
||||||
|
}); |
||||||
|
|
||||||
|
this.content.prepend(this.mediaEl = replyMedia); |
||||||
|
this.container.classList.add('is-media'); |
||||||
|
} |
||||||
|
} else { |
||||||
|
subtitle = subtitle ? RichTextProcessor.wrapEmojiText(subtitle) : ''; |
||||||
|
} |
||||||
|
|
||||||
|
this.title.innerHTML = title; |
||||||
|
this.subtitle.innerHTML = subtitle; |
||||||
|
}); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,27 @@ |
|||||||
|
export default class DivAndCaption<T> { |
||||||
|
public container: HTMLElement; |
||||||
|
public border: HTMLElement; |
||||||
|
public content: HTMLElement; |
||||||
|
public title: HTMLElement; |
||||||
|
public subtitle: HTMLElement; |
||||||
|
|
||||||
|
constructor(protected className: string, public fill: T) { |
||||||
|
this.container = document.createElement('div'); |
||||||
|
this.container.className = className; |
||||||
|
|
||||||
|
this.border = document.createElement('div'); |
||||||
|
this.border.classList.add(className + '-border'); |
||||||
|
|
||||||
|
this.content = document.createElement('div'); |
||||||
|
this.content.classList.add(className + '-content'); |
||||||
|
|
||||||
|
this.title = document.createElement('div'); |
||||||
|
this.title.classList.add(className + '-title'); |
||||||
|
|
||||||
|
this.subtitle = document.createElement('div'); |
||||||
|
this.subtitle.classList.add(className + '-subtitle'); |
||||||
|
|
||||||
|
this.content.append(this.title, this.subtitle); |
||||||
|
this.container.append(this.border, this.content); |
||||||
|
} |
||||||
|
} |
Loading…
Reference in new issue