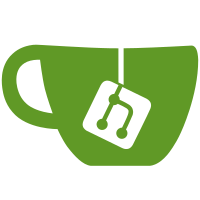
22 changed files with 1006 additions and 199 deletions
@ -0,0 +1,30 @@
@@ -0,0 +1,30 @@
|
||||
package acr.browser.lightning.activity; |
||||
|
||||
import android.support.annotation.Nullable; |
||||
|
||||
import acr.browser.lightning.browser.BookmarksView; |
||||
|
||||
/** |
||||
* The UI model representing the current folder shown |
||||
* by the {@link BookmarksView}. |
||||
* <p> |
||||
* Created by anthonycr on 5/7/17. |
||||
*/ |
||||
public class BookmarkUiModel { |
||||
|
||||
@Nullable private String mCurrentFolder; |
||||
|
||||
public void setCurrentFolder(@Nullable String folder) { |
||||
mCurrentFolder = folder; |
||||
} |
||||
|
||||
public boolean isRootFolder() { |
||||
return mCurrentFolder == null; |
||||
} |
||||
|
||||
@Nullable |
||||
public String getCurrentFolder() { |
||||
return mCurrentFolder; |
||||
} |
||||
|
||||
} |
@ -0,0 +1,375 @@
@@ -0,0 +1,375 @@
|
||||
package acr.browser.lightning.database.bookmark; |
||||
|
||||
import android.app.Application; |
||||
import android.content.ContentValues; |
||||
import android.database.Cursor; |
||||
import android.database.DatabaseUtils; |
||||
import android.database.sqlite.SQLiteDatabase; |
||||
import android.database.sqlite.SQLiteOpenHelper; |
||||
import android.support.annotation.NonNull; |
||||
import android.support.annotation.Nullable; |
||||
import android.text.TextUtils; |
||||
|
||||
import com.anthonycr.bonsai.Completable; |
||||
import com.anthonycr.bonsai.CompletableAction; |
||||
import com.anthonycr.bonsai.CompletableSubscriber; |
||||
import com.anthonycr.bonsai.Single; |
||||
import com.anthonycr.bonsai.SingleAction; |
||||
import com.anthonycr.bonsai.SingleSubscriber; |
||||
|
||||
import java.util.ArrayList; |
||||
import java.util.List; |
||||
|
||||
import javax.inject.Inject; |
||||
import javax.inject.Singleton; |
||||
|
||||
import acr.browser.lightning.R; |
||||
import acr.browser.lightning.constant.Constants; |
||||
import acr.browser.lightning.database.HistoryItem; |
||||
|
||||
/** |
||||
* The disk backed bookmark database. |
||||
* <p> |
||||
* Created by anthonycr on 5/6/17. |
||||
*/ |
||||
@Singleton |
||||
public class BookmarkDatabase extends SQLiteOpenHelper implements BookmarkModel { |
||||
|
||||
private static final String TAG = "BookmarkDatabase"; |
||||
|
||||
// Database Version
|
||||
private static final int DATABASE_VERSION = 1; |
||||
|
||||
// Database Name
|
||||
private static final String DATABASE_NAME = "bookmarkManager"; |
||||
|
||||
// HistoryItems table name
|
||||
private static final String TABLE_BOOKMARK = "bookmark"; |
||||
|
||||
// HistoryItems Table Columns names
|
||||
private static final String KEY_ID = "id"; |
||||
private static final String KEY_URL = "url"; |
||||
private static final String KEY_TITLE = "title"; |
||||
private static final String KEY_FOLDER = "folder"; |
||||
private static final String KEY_POSITION = "position"; |
||||
|
||||
|
||||
@NonNull private final String DEFAULT_BOOKMARK_TITLE; |
||||
|
||||
@Nullable private SQLiteDatabase mDatabase; |
||||
|
||||
@Inject |
||||
public BookmarkDatabase(@NonNull Application application) { |
||||
super(application, DATABASE_NAME, null, DATABASE_VERSION); |
||||
DEFAULT_BOOKMARK_TITLE = application.getString(R.string.untitled); |
||||
} |
||||
|
||||
@NonNull |
||||
private SQLiteDatabase lazyDatabase() { |
||||
if (mDatabase == null) { |
||||
mDatabase = getWritableDatabase(); |
||||
} |
||||
|
||||
return mDatabase; |
||||
} |
||||
|
||||
// Creating Tables
|
||||
@Override |
||||
public void onCreate(@NonNull SQLiteDatabase db) { |
||||
String CREATE_BOOKMARK_TABLE = "CREATE TABLE " + |
||||
DatabaseUtils.sqlEscapeString(TABLE_BOOKMARK) + '(' + |
||||
DatabaseUtils.sqlEscapeString(KEY_ID) + " INTEGER PRIMARY KEY," + |
||||
DatabaseUtils.sqlEscapeString(KEY_URL) + " TEXT," + |
||||
DatabaseUtils.sqlEscapeString(KEY_TITLE) + " TEXT," + |
||||
DatabaseUtils.sqlEscapeString(KEY_FOLDER) + " TEXT," + |
||||
DatabaseUtils.sqlEscapeString(KEY_POSITION) + " INTEGER" + ')'; |
||||
db.execSQL(CREATE_BOOKMARK_TABLE); |
||||
} |
||||
|
||||
// Upgrading database
|
||||
@Override |
||||
public void onUpgrade(@NonNull SQLiteDatabase db, int oldVersion, int newVersion) { |
||||
// Drop older table if it exists
|
||||
db.execSQL("DROP TABLE IF EXISTS " + DatabaseUtils.sqlEscapeString(TABLE_BOOKMARK)); |
||||
// Create tables again
|
||||
onCreate(db); |
||||
} |
||||
|
||||
@NonNull |
||||
private static ContentValues bindBookmarkToContentValues(@NonNull HistoryItem historyItem) { |
||||
ContentValues contentValues = new ContentValues(4); |
||||
contentValues.put(KEY_TITLE, historyItem.getTitle()); |
||||
contentValues.put(KEY_URL, historyItem.getUrl()); |
||||
contentValues.put(KEY_FOLDER, historyItem.getFolder()); |
||||
contentValues.put(KEY_POSITION, historyItem.getPosition()); |
||||
|
||||
return contentValues; |
||||
} |
||||
|
||||
@NonNull |
||||
private static HistoryItem bindCursorToHistoryItem(@NonNull Cursor cursor) { |
||||
HistoryItem bookmark = new HistoryItem(); |
||||
|
||||
bookmark.setImageId(R.drawable.ic_bookmark); |
||||
bookmark.setUrl(cursor.getString(cursor.getColumnIndex(KEY_URL))); |
||||
bookmark.setTitle(cursor.getString(cursor.getColumnIndex(KEY_TITLE))); |
||||
bookmark.setFolder(cursor.getString(cursor.getColumnIndex(KEY_FOLDER))); |
||||
bookmark.setPosition(cursor.getInt(cursor.getColumnIndex(KEY_POSITION))); |
||||
|
||||
return bookmark; |
||||
} |
||||
|
||||
@NonNull |
||||
private static List<HistoryItem> bindCursorToHistoryItemList(@NonNull Cursor cursor) { |
||||
List<HistoryItem> bookmarks = new ArrayList<>(); |
||||
|
||||
while (cursor.moveToNext()) { |
||||
bookmarks.add(bindCursorToHistoryItem(cursor)); |
||||
} |
||||
|
||||
cursor.close(); |
||||
|
||||
return bookmarks; |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<HistoryItem> findBookmarkForUrl(@NonNull final String url) { |
||||
return Single.create(new SingleAction<HistoryItem>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<HistoryItem> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_BOOKMARK, null, KEY_URL + "=?", new String[]{url}, null, null, null, "1"); |
||||
|
||||
if (cursor.moveToFirst()) { |
||||
subscriber.onItem(bindCursorToHistoryItem(cursor)); |
||||
} |
||||
|
||||
cursor.close(); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<Boolean> isBookmark(@NonNull final String url) { |
||||
return Single.create(new SingleAction<Boolean>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<Boolean> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_BOOKMARK, null, KEY_URL + "=?", new String[]{url}, null, null, null, "1"); |
||||
|
||||
subscriber.onItem(cursor.moveToFirst()); |
||||
|
||||
cursor.close(); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<Boolean> addBookmarkIfNotExists(@NonNull final HistoryItem item) { |
||||
return Single.create(new SingleAction<Boolean>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<Boolean> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_BOOKMARK, null, KEY_URL + "=?", new String[]{item.getUrl()}, null, null, null, "1"); |
||||
|
||||
if (cursor.moveToFirst()) { |
||||
cursor.close(); |
||||
subscriber.onItem(false); |
||||
subscriber.onComplete(); |
||||
return; |
||||
} |
||||
|
||||
cursor.close(); |
||||
|
||||
long id = lazyDatabase().insert(TABLE_BOOKMARK, null, bindBookmarkToContentValues(item)); |
||||
|
||||
subscriber.onItem(id != -1); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Completable addBookmarkList(@NonNull final List<HistoryItem> bookmarkItems) { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull CompletableSubscriber subscriber) { |
||||
lazyDatabase().beginTransaction(); |
||||
|
||||
for (HistoryItem item : bookmarkItems) { |
||||
addBookmarkIfNotExists(item).subscribe(); |
||||
} |
||||
|
||||
lazyDatabase().setTransactionSuccessful(); |
||||
lazyDatabase().endTransaction(); |
||||
|
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<Boolean> deleteBookmark(@NonNull final HistoryItem bookmark) { |
||||
return Single.create(new SingleAction<Boolean>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<Boolean> subscriber) { |
||||
int rows = lazyDatabase().delete(TABLE_BOOKMARK, KEY_URL + "=?", new String[]{bookmark.getUrl()}); |
||||
|
||||
subscriber.onItem(rows > 0); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Completable renameFolder(@NonNull final String oldName, @NonNull final String newName) { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull CompletableSubscriber subscriber) { |
||||
ContentValues contentValues = new ContentValues(1); |
||||
contentValues.put(KEY_FOLDER, newName); |
||||
|
||||
lazyDatabase().update(TABLE_BOOKMARK, contentValues, KEY_FOLDER + "=?", new String[]{oldName}); |
||||
|
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Completable deleteFolder(@NonNull final String folderToDelete) { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull CompletableSubscriber subscriber) { |
||||
renameFolder(folderToDelete, "").subscribe(); |
||||
|
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Completable deleteAllBookmarks() { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull CompletableSubscriber subscriber) { |
||||
lazyDatabase().delete(TABLE_BOOKMARK, null, null); |
||||
|
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Completable editBookmark(@NonNull final HistoryItem oldBookmark, @NonNull final HistoryItem newBookmark) { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull CompletableSubscriber subscriber) { |
||||
if (newBookmark.getTitle().isEmpty()) { |
||||
newBookmark.setTitle(DEFAULT_BOOKMARK_TITLE); |
||||
} |
||||
ContentValues contentValues = bindBookmarkToContentValues(newBookmark); |
||||
|
||||
lazyDatabase().update(TABLE_BOOKMARK, contentValues, KEY_URL + "=?", new String[]{oldBookmark.getUrl()}); |
||||
|
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<List<HistoryItem>> getAllBookmarks() { |
||||
return Single.create(new SingleAction<List<HistoryItem>>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<List<HistoryItem>> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_BOOKMARK, null, null, null, null, null, null); |
||||
|
||||
subscriber.onItem(bindCursorToHistoryItemList(cursor)); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<List<HistoryItem>> getBookmarksFromFolder(@Nullable final String folder) { |
||||
return Single.create(new SingleAction<List<HistoryItem>>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<List<HistoryItem>> subscriber) { |
||||
String finalFolder = folder != null ? folder : ""; |
||||
Cursor cursor = lazyDatabase().query(TABLE_BOOKMARK, null, KEY_FOLDER + "=?", new String[]{finalFolder}, null, null, null); |
||||
|
||||
subscriber.onItem(bindCursorToHistoryItemList(cursor)); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<List<HistoryItem>> getFolders() { |
||||
return Single.create(new SingleAction<List<HistoryItem>>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<List<HistoryItem>> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(true, TABLE_BOOKMARK, new String[]{KEY_FOLDER}, null, null, null, null, null, null); |
||||
|
||||
List<HistoryItem> folders = new ArrayList<>(); |
||||
while (cursor.moveToNext()) { |
||||
String folderName = cursor.getString(cursor.getColumnIndex(KEY_FOLDER)); |
||||
if (TextUtils.isEmpty(folderName)) { |
||||
continue; |
||||
} |
||||
|
||||
final HistoryItem folder = new HistoryItem(); |
||||
folder.setIsFolder(true); |
||||
folder.setTitle(folderName); |
||||
folder.setImageId(R.drawable.ic_folder); |
||||
folder.setUrl(Constants.FOLDER + folderName); |
||||
|
||||
folders.add(folder); |
||||
} |
||||
|
||||
cursor.close(); |
||||
|
||||
subscriber.onItem(folders); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<List<String>> getFolderNames() { |
||||
return Single.create(new SingleAction<List<String>>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<List<String>> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(true, TABLE_BOOKMARK, new String[]{KEY_FOLDER}, null, null, null, null, null, null); |
||||
|
||||
List<String> folders = new ArrayList<>(); |
||||
while (cursor.moveToNext()) { |
||||
String folderName = cursor.getString(cursor.getColumnIndex(KEY_FOLDER)); |
||||
if (TextUtils.isEmpty(folderName)) { |
||||
continue; |
||||
} |
||||
|
||||
folders.add(folderName); |
||||
} |
||||
|
||||
cursor.close(); |
||||
|
||||
subscriber.onItem(folders); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,166 @@
@@ -0,0 +1,166 @@
|
||||
package acr.browser.lightning.database.bookmark; |
||||
|
||||
import android.content.Context; |
||||
import android.os.Environment; |
||||
import android.support.annotation.NonNull; |
||||
import android.support.annotation.WorkerThread; |
||||
import android.util.Log; |
||||
|
||||
import com.anthonycr.bonsai.Completable; |
||||
import com.anthonycr.bonsai.CompletableAction; |
||||
import com.anthonycr.bonsai.CompletableSubscriber; |
||||
import com.anthonycr.bonsai.Single; |
||||
import com.anthonycr.bonsai.SingleAction; |
||||
import com.anthonycr.bonsai.SingleSubscriber; |
||||
|
||||
import org.json.JSONException; |
||||
import org.json.JSONObject; |
||||
|
||||
import java.io.BufferedReader; |
||||
import java.io.BufferedWriter; |
||||
import java.io.File; |
||||
import java.io.FileReader; |
||||
import java.io.FileWriter; |
||||
import java.io.IOException; |
||||
import java.io.InputStream; |
||||
import java.io.InputStreamReader; |
||||
import java.util.ArrayList; |
||||
import java.util.List; |
||||
|
||||
import acr.browser.lightning.R; |
||||
import acr.browser.lightning.database.HistoryItem; |
||||
import acr.browser.lightning.utils.Preconditions; |
||||
import acr.browser.lightning.utils.Utils; |
||||
|
||||
/** |
||||
* The class responsible for importing and exporting |
||||
* bookmarks in the JSON format. |
||||
* <p> |
||||
* Created by anthonycr on 5/7/17. |
||||
*/ |
||||
public class BookmarkExporter { |
||||
|
||||
private static final String TAG = "BookmarkExporter"; |
||||
|
||||
private static final String KEY_URL = "url"; |
||||
private static final String KEY_TITLE = "title"; |
||||
private static final String KEY_FOLDER = "folder"; |
||||
private static final String KEY_ORDER = "order"; |
||||
|
||||
@NonNull |
||||
public static List<HistoryItem> importBookmarksFromAssets(@NonNull Context context) { |
||||
List<HistoryItem> bookmarks = new ArrayList<>(); |
||||
BufferedReader bookmarksReader = null; |
||||
InputStream inputStream = null; |
||||
try { |
||||
inputStream = context.getResources().openRawResource(R.raw.default_bookmarks); |
||||
//noinspection IOResourceOpenedButNotSafelyClosed
|
||||
bookmarksReader = new BufferedReader(new InputStreamReader(inputStream)); |
||||
String line; |
||||
while ((line = bookmarksReader.readLine()) != null) { |
||||
try { |
||||
JSONObject object = new JSONObject(line); |
||||
HistoryItem item = new HistoryItem(); |
||||
item.setTitle(object.getString(KEY_TITLE)); |
||||
final String url = object.getString(KEY_URL); |
||||
item.setUrl(url); |
||||
item.setFolder(object.getString(KEY_FOLDER)); |
||||
item.setPosition(object.getInt(KEY_ORDER)); |
||||
item.setImageId(R.drawable.ic_bookmark); |
||||
bookmarks.add(item); |
||||
} catch (JSONException e) { |
||||
Log.e(TAG, "Can't parse line " + line, e); |
||||
} |
||||
} |
||||
} catch (IOException e) { |
||||
Log.e(TAG, "Error reading the bookmarks file", e); |
||||
} finally { |
||||
Utils.close(bookmarksReader); |
||||
Utils.close(inputStream); |
||||
} |
||||
|
||||
return bookmarks; |
||||
} |
||||
|
||||
@NonNull |
||||
public static Completable exportBookmarksToFile(@NonNull final List<HistoryItem> bookmarkList, |
||||
@NonNull final File file) { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull final CompletableSubscriber subscriber) { |
||||
Preconditions.checkNonNull(bookmarkList); |
||||
BufferedWriter bookmarkWriter = null; |
||||
try { |
||||
//noinspection IOResourceOpenedButNotSafelyClosed
|
||||
bookmarkWriter = new BufferedWriter(new FileWriter(file, false)); |
||||
|
||||
JSONObject object = new JSONObject(); |
||||
for (HistoryItem item : bookmarkList) { |
||||
object.put(KEY_TITLE, item.getTitle()); |
||||
object.put(KEY_URL, item.getUrl()); |
||||
object.put(KEY_FOLDER, item.getFolder()); |
||||
object.put(KEY_ORDER, item.getPosition()); |
||||
bookmarkWriter.write(object.toString()); |
||||
bookmarkWriter.newLine(); |
||||
} |
||||
subscriber.onComplete(); |
||||
} catch (@NonNull IOException | JSONException e) { |
||||
subscriber.onError(e); |
||||
} finally { |
||||
Utils.close(bookmarkWriter); |
||||
} |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
public static Single<List<HistoryItem>> importBookmarksFromFile(@NonNull final File file) { |
||||
return Single.create(new SingleAction<List<HistoryItem>>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<List<HistoryItem>> subscriber) { |
||||
BufferedReader bookmarksReader = null; |
||||
try { |
||||
//noinspection IOResourceOpenedButNotSafelyClosed
|
||||
bookmarksReader = new BufferedReader(new FileReader(file)); |
||||
String line; |
||||
|
||||
List<HistoryItem> bookmarks = new ArrayList<>(); |
||||
while ((line = bookmarksReader.readLine()) != null) { |
||||
JSONObject object = new JSONObject(line); |
||||
HistoryItem item = new HistoryItem(); |
||||
item.setTitle(object.getString(KEY_TITLE)); |
||||
item.setUrl(object.getString(KEY_URL)); |
||||
item.setFolder(object.getString(KEY_FOLDER)); |
||||
item.setPosition(object.getInt(KEY_ORDER)); |
||||
bookmarks.add(item); |
||||
} |
||||
|
||||
subscriber.onItem(bookmarks); |
||||
subscriber.onComplete(); |
||||
} catch (IOException | JSONException e) { |
||||
subscriber.onError(e); |
||||
} finally { |
||||
Utils.close(bookmarksReader); |
||||
} |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@WorkerThread |
||||
@NonNull |
||||
public static File createNewExportFile() { |
||||
File bookmarksExport = new File( |
||||
Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS), |
||||
"BookmarksExport.txt"); |
||||
int counter = 0; |
||||
while (bookmarksExport.exists()) { |
||||
counter++; |
||||
bookmarksExport = new File( |
||||
Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS), |
||||
"BookmarksExport-" + counter + ".txt"); |
||||
} |
||||
|
||||
return bookmarksExport; |
||||
} |
||||
|
||||
} |
@ -0,0 +1,60 @@
@@ -0,0 +1,60 @@
|
||||
package acr.browser.lightning.database.bookmark; |
||||
|
||||
import android.support.annotation.NonNull; |
||||
import android.support.annotation.Nullable; |
||||
|
||||
import com.anthonycr.bonsai.Completable; |
||||
import com.anthonycr.bonsai.Single; |
||||
|
||||
import java.io.File; |
||||
import java.util.List; |
||||
|
||||
import acr.browser.lightning.database.HistoryItem; |
||||
|
||||
/** |
||||
* The interface that should be used to |
||||
* communicate with the bookmark database. |
||||
* <p> |
||||
* Created by anthonycr on 5/6/17. |
||||
*/ |
||||
public interface BookmarkModel { |
||||
|
||||
@NonNull |
||||
Single<HistoryItem> findBookmarkForUrl(@NonNull String url); |
||||
|
||||
@NonNull |
||||
Single<Boolean> isBookmark(@NonNull String url); |
||||
|
||||
@NonNull |
||||
Single<Boolean> addBookmarkIfNotExists(@NonNull HistoryItem item); |
||||
|
||||
@NonNull |
||||
Completable addBookmarkList(@NonNull List<HistoryItem> bookmarkItems); |
||||
|
||||
@NonNull |
||||
Single<Boolean> deleteBookmark(@NonNull HistoryItem bookmark); |
||||
|
||||
@NonNull |
||||
Completable renameFolder(@NonNull String oldName, @NonNull String newName); |
||||
|
||||
@NonNull |
||||
Completable deleteFolder(@NonNull String folderToDelete); |
||||
|
||||
@NonNull |
||||
Completable deleteAllBookmarks(); |
||||
|
||||
@NonNull |
||||
Completable editBookmark(@NonNull HistoryItem oldBookmark, @NonNull HistoryItem newBookmark); |
||||
|
||||
@NonNull |
||||
Single<List<HistoryItem>> getAllBookmarks(); |
||||
|
||||
@NonNull |
||||
Single<List<HistoryItem>> getBookmarksFromFolder(@Nullable String folder); |
||||
|
||||
@NonNull |
||||
Single<List<HistoryItem>> getFolders(); |
||||
|
||||
@NonNull |
||||
Single<List<String>> getFolderNames(); |
||||
} |
Loading…
Reference in new issue