Browse Source
- fixed all issues with rendering, performance has increased dramatically on not-fullscreen and has increased a little in fullscreen - battery life improved as a result - added hebrew language - changed the way history works (now uses SQLite database, it's way better than the android.provider.Browser way) - fixed a couple crashes related to bookmarks and downloads - formatted the code a little better (thanks to Aeefire for the tips, just know I'm taking your advice LOL)master
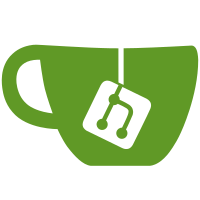
9 changed files with 508 additions and 183 deletions
After Width: | Height: | Size: 2.5 KiB |
@ -0,0 +1,24 @@ |
|||||||
|
<?xml version="1.0" encoding="utf-8"?> |
||||||
|
<!-- |
||||||
|
/* |
||||||
|
** |
||||||
|
** Copyright 2009, The Android Open Source Project |
||||||
|
** |
||||||
|
** Licensed under the Apache License, Version 2.0 (the "License"); |
||||||
|
** you may not use this file except in compliance with the License. |
||||||
|
** You may obtain a copy of the License at |
||||||
|
** |
||||||
|
** http://www.apache.org/licenses/LICENSE-2.0 |
||||||
|
** |
||||||
|
** Unless required by applicable law or agreed to in writing, software |
||||||
|
** distributed under the License is distributed on an "AS IS" BASIS, |
||||||
|
** WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
||||||
|
** See the License for the specific language governing permissions and |
||||||
|
** limitations under the License. |
||||||
|
*/ |
||||||
|
--> |
||||||
|
<animated-rotate xmlns:android="http://schemas.android.com/apk/res/android" |
||||||
|
android:drawable="@drawable/spinner" |
||||||
|
android:pivotX="50%" |
||||||
|
android:pivotY="50%" |
||||||
|
/> |
@ -0,0 +1,26 @@ |
|||||||
|
<?xml version="1.0" encoding="utf-8"?> |
||||||
|
<!-- |
||||||
|
Copyright 2010, The Android Open Source Project |
||||||
|
|
||||||
|
Licensed under the Apache License, Version 2.0 (the "License"); |
||||||
|
you may not use this file except in compliance with the License. |
||||||
|
You may obtain a copy of the License at |
||||||
|
|
||||||
|
http://www.apache.org/licenses/LICENSE-2.0 |
||||||
|
|
||||||
|
Unless required by applicable law or agreed to in writing, software |
||||||
|
distributed under the License is distributed on an "AS IS" BASIS, |
||||||
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
||||||
|
See the License for the specific language governing permissions and |
||||||
|
limitations under the License. |
||||||
|
--> |
||||||
|
<layer-list xmlns:android="http://schemas.android.com/apk/res/android"> |
||||||
|
<item> |
||||||
|
<rotate |
||||||
|
android:drawable="@drawable/spinner" |
||||||
|
android:fromDegrees="720" |
||||||
|
android:pivotX="50%" |
||||||
|
android:pivotY="50%" |
||||||
|
android:toDegrees="0" /> |
||||||
|
</item> |
||||||
|
</layer-list> |
@ -0,0 +1,77 @@ |
|||||||
|
package acr.browser.barebones; |
||||||
|
|
||||||
|
import android.content.Context; |
||||||
|
import android.view.MotionEvent; |
||||||
|
import android.view.View; |
||||||
|
import android.view.animation.Animation; |
||||||
|
import android.webkit.WebView; |
||||||
|
|
||||||
|
public final class AnthonyWebView extends WebView { |
||||||
|
|
||||||
|
boolean move; |
||||||
|
int API = Barebones.API; |
||||||
|
long timeBetweenDownPress = 0; |
||||||
|
int hitTest; |
||||||
|
boolean showFullScreen = Barebones.showFullScreen; |
||||||
|
View uBar = Barebones.uBar; |
||||||
|
boolean uBarShows = Barebones.uBarShows; |
||||||
|
Animation slideUp = Barebones.slideUp; |
||||||
|
Animation slideDown = Barebones.slideDown; |
||||||
|
|
||||||
|
public AnthonyWebView(Context context) { |
||||||
|
super(context); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean onTouchEvent(MotionEvent event) { |
||||||
|
|
||||||
|
switch (event.getAction()) { |
||||||
|
case MotionEvent.ACTION_DOWN: { |
||||||
|
move = false; |
||||||
|
if (API <= 10 && !Barebones.main[Barebones.pageId].hasFocus()) { |
||||||
|
Barebones.main[Barebones.pageId].requestFocus(); |
||||||
|
} |
||||||
|
timeBetweenDownPress = System.currentTimeMillis(); |
||||||
|
break; |
||||||
|
} |
||||||
|
case MotionEvent.ACTION_MOVE: { |
||||||
|
move = true; |
||||||
|
} |
||||||
|
case MotionEvent.ACTION_UP: { |
||||||
|
|
||||||
|
try { |
||||||
|
hitTest = getHitTestResult().getType(); |
||||||
|
} catch (NullPointerException e) { |
||||||
|
} |
||||||
|
if (showFullScreen && hitTest != 9) { |
||||||
|
if (System.currentTimeMillis() - timeBetweenDownPress < 500 |
||||||
|
&& !move) { |
||||||
|
if (!uBarShows) { |
||||||
|
uBar.startAnimation(slideDown); |
||||||
|
uBarShows = true; |
||||||
|
} else if (uBarShows) { |
||||||
|
uBar.startAnimation(slideUp); |
||||||
|
uBarShows = false; |
||||||
|
} |
||||||
|
break; |
||||||
|
|
||||||
|
} else if (Barebones.main[Barebones.pageId].getScrollY() > 5 && uBarShows) { |
||||||
|
uBar.startAnimation(slideUp); |
||||||
|
uBarShows = false; |
||||||
|
break; |
||||||
|
} else if (Barebones.main[Barebones.pageId].getScrollY() < 5 && !uBarShows) { |
||||||
|
|
||||||
|
uBar.startAnimation(slideDown); |
||||||
|
uBarShows = true; |
||||||
|
break; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
default: |
||||||
|
break; |
||||||
|
} |
||||||
|
|
||||||
|
return super.onTouchEvent(event); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,141 @@ |
|||||||
|
package acr.browser.barebones; |
||||||
|
|
||||||
|
import java.util.ArrayList; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
import android.content.ContentValues; |
||||||
|
import android.content.Context; |
||||||
|
import android.database.Cursor; |
||||||
|
import android.database.sqlite.SQLiteDatabase; |
||||||
|
import android.database.sqlite.SQLiteOpenHelper; |
||||||
|
|
||||||
|
public class DatabaseHandler extends SQLiteOpenHelper { |
||||||
|
|
||||||
|
// All Static variables
|
||||||
|
// Database Version
|
||||||
|
private static final int DATABASE_VERSION = 1; |
||||||
|
|
||||||
|
// Database Name
|
||||||
|
private static final String DATABASE_NAME = "historyManager"; |
||||||
|
|
||||||
|
// HistoryItems table name
|
||||||
|
private static final String TABLE_HISTORY = "history"; |
||||||
|
|
||||||
|
// HistoryItems Table Columns names
|
||||||
|
private static final String KEY_ID = "id"; |
||||||
|
private static final String KEY_URL = "url"; |
||||||
|
private static final String KEY_TITLE = "title"; |
||||||
|
|
||||||
|
public DatabaseHandler(Context context) { |
||||||
|
super(context, DATABASE_NAME, null, DATABASE_VERSION); |
||||||
|
} |
||||||
|
|
||||||
|
// Creating Tables
|
||||||
|
@Override |
||||||
|
public void onCreate(SQLiteDatabase db) { |
||||||
|
String CREATE_CONTACTS_TABLE = "CREATE TABLE " + TABLE_HISTORY + "(" |
||||||
|
+ KEY_ID + " INTEGER PRIMARY KEY," + KEY_URL + " TEXT," |
||||||
|
+ KEY_TITLE + " TEXT" +")"; |
||||||
|
db.execSQL(CREATE_CONTACTS_TABLE); |
||||||
|
} |
||||||
|
|
||||||
|
// Upgrading database
|
||||||
|
@Override |
||||||
|
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { |
||||||
|
// Drop older table if existed
|
||||||
|
db.execSQL("DROP TABLE IF EXISTS " + TABLE_HISTORY); |
||||||
|
|
||||||
|
// Create tables again
|
||||||
|
onCreate(db); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* All CRUD(Create, Read, Update, Delete) Operations |
||||||
|
*/ |
||||||
|
|
||||||
|
// Adding new item
|
||||||
|
void addHistoryItem(HistoryItem item) { |
||||||
|
SQLiteDatabase db = this.getWritableDatabase(); |
||||||
|
|
||||||
|
ContentValues values = new ContentValues(); |
||||||
|
values.put(KEY_URL, item.getUrl()); // HistoryItem Name
|
||||||
|
values.put(KEY_TITLE, item.getTitle()); // HistoryItem Phone
|
||||||
|
// Inserting Row
|
||||||
|
db.insert(TABLE_HISTORY, null, values); |
||||||
|
db.close(); // Closing database connection
|
||||||
|
} |
||||||
|
|
||||||
|
// Getting single item
|
||||||
|
HistoryItem getHistoryItem(int id) { |
||||||
|
SQLiteDatabase db = this.getReadableDatabase(); |
||||||
|
|
||||||
|
Cursor cursor = db.query(TABLE_HISTORY, new String[] { KEY_ID, |
||||||
|
KEY_URL, KEY_TITLE}, KEY_ID + "=?", |
||||||
|
new String[] { String.valueOf(id) }, null, null, null, null); |
||||||
|
if (cursor != null) |
||||||
|
cursor.moveToFirst(); |
||||||
|
|
||||||
|
HistoryItem item = new HistoryItem(Integer.parseInt(cursor.getString(0)), |
||||||
|
cursor.getString(1), cursor.getString(2)); |
||||||
|
// return item
|
||||||
|
return item; |
||||||
|
} |
||||||
|
|
||||||
|
// Getting All HistoryItems
|
||||||
|
public List<HistoryItem> getAllHistoryItems() { |
||||||
|
List<HistoryItem> itemList = new ArrayList<HistoryItem>(); |
||||||
|
// Select All Query
|
||||||
|
String selectQuery = "SELECT * FROM " + TABLE_HISTORY; |
||||||
|
|
||||||
|
SQLiteDatabase db = this.getWritableDatabase(); |
||||||
|
Cursor cursor = db.rawQuery(selectQuery, null); |
||||||
|
|
||||||
|
// looping through all rows and adding to list
|
||||||
|
if (cursor.moveToFirst()) { |
||||||
|
do { |
||||||
|
HistoryItem item = new HistoryItem(); |
||||||
|
item.setID(Integer.parseInt(cursor.getString(0))); |
||||||
|
item.setUrl(cursor.getString(1)); |
||||||
|
item.setTitle(cursor.getString(2)); |
||||||
|
// Adding item to list
|
||||||
|
itemList.add(item); |
||||||
|
} while (cursor.moveToNext()); |
||||||
|
} |
||||||
|
|
||||||
|
// return item list
|
||||||
|
return itemList; |
||||||
|
} |
||||||
|
|
||||||
|
// Updating single item
|
||||||
|
public int updateHistoryItem(HistoryItem item) { |
||||||
|
SQLiteDatabase db = this.getWritableDatabase(); |
||||||
|
|
||||||
|
ContentValues values = new ContentValues(); |
||||||
|
values.put(KEY_URL, item.getUrl()); |
||||||
|
values.put(KEY_TITLE, item.getTitle()); |
||||||
|
|
||||||
|
// updating row
|
||||||
|
return db.update(TABLE_HISTORY, values, KEY_ID + " = ?", |
||||||
|
new String[] { String.valueOf(item.getID()) }); |
||||||
|
} |
||||||
|
|
||||||
|
// Deleting single item
|
||||||
|
public void deleteHistoryItem(HistoryItem item) { |
||||||
|
SQLiteDatabase db = this.getWritableDatabase(); |
||||||
|
db.delete(TABLE_HISTORY, KEY_ID + " = ?", |
||||||
|
new String[] { String.valueOf(item.getID()) }); |
||||||
|
db.close(); |
||||||
|
} |
||||||
|
|
||||||
|
// Getting items Count
|
||||||
|
public int getHistoryItemsCount() { |
||||||
|
String countQuery = "SELECT * FROM " + TABLE_HISTORY; |
||||||
|
SQLiteDatabase db = this.getReadableDatabase(); |
||||||
|
Cursor cursor = db.rawQuery(countQuery, null); |
||||||
|
cursor.close(); |
||||||
|
|
||||||
|
// return count
|
||||||
|
return cursor.getCount(); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,57 @@ |
|||||||
|
package acr.browser.barebones; |
||||||
|
|
||||||
|
public class HistoryItem { |
||||||
|
|
||||||
|
|
||||||
|
//private variables
|
||||||
|
int _id; |
||||||
|
String _url; |
||||||
|
String _title; |
||||||
|
|
||||||
|
// Empty constructor
|
||||||
|
public HistoryItem(){ |
||||||
|
|
||||||
|
} |
||||||
|
// constructor
|
||||||
|
public HistoryItem(int id, String url, String title){ |
||||||
|
this._id = id; |
||||||
|
this._url = url; |
||||||
|
this._title = title; |
||||||
|
} |
||||||
|
|
||||||
|
// constructor
|
||||||
|
public HistoryItem(String url, String title){ |
||||||
|
this._url = url; |
||||||
|
this._title = title; |
||||||
|
} |
||||||
|
// getting ID
|
||||||
|
public int getID(){ |
||||||
|
return this._id; |
||||||
|
} |
||||||
|
|
||||||
|
// setting id
|
||||||
|
public void setID(int id){ |
||||||
|
this._id = id; |
||||||
|
} |
||||||
|
|
||||||
|
// getting name
|
||||||
|
public String getUrl(){ |
||||||
|
return this._url; |
||||||
|
} |
||||||
|
|
||||||
|
// setting name
|
||||||
|
public void setUrl(String url){ |
||||||
|
this._url = url; |
||||||
|
} |
||||||
|
|
||||||
|
// getting phone number
|
||||||
|
public String getTitle(){ |
||||||
|
return this._title; |
||||||
|
} |
||||||
|
|
||||||
|
// setting phone number
|
||||||
|
public void setTitle(String title){ |
||||||
|
this._title = title; |
||||||
|
} |
||||||
|
|
||||||
|
} |
Loading…
Reference in new issue