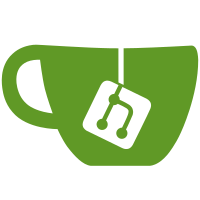
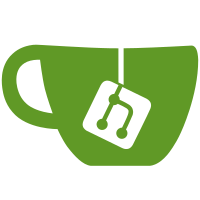
3 changed files with 120 additions and 3 deletions
@ -0,0 +1,91 @@
@@ -0,0 +1,91 @@
|
||||
/* |
||||
* Copyright (c) 2023 New Vector Ltd |
||||
* |
||||
* Licensed under the Apache License, Version 2.0 (the "License"); |
||||
* you may not use this file except in compliance with the License. |
||||
* You may obtain a copy of the License at |
||||
* |
||||
* http://www.apache.org/licenses/LICENSE-2.0 |
||||
* |
||||
* Unless required by applicable law or agreed to in writing, software |
||||
* distributed under the License is distributed on an "AS IS" BASIS, |
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
||||
* See the License for the specific language governing permissions and |
||||
* limitations under the License. |
||||
*/ |
||||
|
||||
package io.element.android.features.login.impl.changeaccountprovider.common |
||||
|
||||
import app.cash.molecule.RecompositionClock |
||||
import app.cash.molecule.moleculeFlow |
||||
import app.cash.turbine.test |
||||
import com.google.common.truth.Truth.assertThat |
||||
import io.element.android.features.login.impl.accountprovider.item.AccountProvider |
||||
import io.element.android.features.login.impl.datasource.AccountProviderDataSource |
||||
import io.element.android.libraries.architecture.Async |
||||
import io.element.android.libraries.matrix.test.A_HOMESERVER |
||||
import io.element.android.libraries.matrix.test.A_HOMESERVER_URL |
||||
import io.element.android.libraries.matrix.test.auth.FakeAuthenticationService |
||||
import kotlinx.coroutines.test.runTest |
||||
import org.junit.Test |
||||
|
||||
class ChangeServerPresenterTest { |
||||
@Test |
||||
fun `present - initial state`() = runTest { |
||||
val presenter = ChangeServerPresenter( |
||||
FakeAuthenticationService(), |
||||
AccountProviderDataSource() |
||||
) |
||||
moleculeFlow(RecompositionClock.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.changeServerAction).isEqualTo(Async.Uninitialized) |
||||
} |
||||
} |
||||
|
||||
@Test |
||||
fun `present - change server ok`() = runTest { |
||||
val authenticationService = FakeAuthenticationService() |
||||
val presenter = ChangeServerPresenter( |
||||
authenticationService, |
||||
AccountProviderDataSource() |
||||
) |
||||
moleculeFlow(RecompositionClock.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.changeServerAction).isEqualTo(Async.Uninitialized) |
||||
authenticationService.givenHomeserver(A_HOMESERVER) |
||||
initialState.eventSink.invoke(ChangeServerEvents.ChangeServer(AccountProvider(A_HOMESERVER_URL))) |
||||
val loadingState = awaitItem() |
||||
assertThat(loadingState.changeServerAction).isInstanceOf(Async.Loading::class.java) |
||||
val successState = awaitItem() |
||||
assertThat(successState.changeServerAction).isEqualTo(Async.Success(Unit)) |
||||
} |
||||
} |
||||
|
||||
@Test |
||||
fun `present - change server error`() = runTest { |
||||
val authenticationService = FakeAuthenticationService() |
||||
val presenter = ChangeServerPresenter( |
||||
authenticationService, |
||||
AccountProviderDataSource() |
||||
) |
||||
moleculeFlow(RecompositionClock.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.changeServerAction).isEqualTo(Async.Uninitialized) |
||||
initialState.eventSink.invoke(ChangeServerEvents.ChangeServer(AccountProvider(A_HOMESERVER_URL))) |
||||
val loadingState = awaitItem() |
||||
assertThat(loadingState.changeServerAction).isInstanceOf(Async.Loading::class.java) |
||||
val failureState = awaitItem() |
||||
assertThat(failureState.changeServerAction).isInstanceOf(Async.Failure::class.java) |
||||
// Clear error |
||||
failureState.eventSink.invoke(ChangeServerEvents.ClearError) |
||||
val finalState = awaitItem() |
||||
assertThat(finalState.changeServerAction).isEqualTo(Async.Uninitialized) |
||||
} |
||||
} |
||||
} |
Loading…
Reference in new issue