Browse Source
84973d3 Merge #454: Remove residual parts from the schnorr expirement. 5e95bf2 Remove residual parts from the schnorr expirement. cbc20b8 Merge #452: Minor optimizations to _scalar_inverse to save 4M 4cc8f52 Merge #437: Unroll secp256k1_fe_(get|set)_b32 to make them much faster. 465159c Further shorten the addition chain for scalar inversion. a2b6b19 Fix benchmark print_number infinite loop. 8b7680a Unroll secp256k1_fe_(get|set)_b32 for 10x26. aa84990 Unroll secp256k1_fe_(get|set)_b32 for 5x52. cf12fa1 Minor optimizations to _scalar_inverse to save 4M 1199492 Merge #408: Add `secp256k1_ec_pubkey_negate` and `secp256k1_ec_privkey_negate` 6af0871 Merge #441: secp256k1_context_randomize: document. ab31a52 Merge #444: test: Use checked_alloc eda5c1a Merge #449: Remove executable bit from secp256k1.c 51b77ae Remove executable bit from secp256k1.c 5eb030c test: Use checked_alloc 72d952c FIXUP: Missing "is" 70ff29b secp256k1_context_randomize: document. 9d560f9 Merge #428: Exhaustive recovery 8e48aa6 Add `secp256k1_ec_pubkey_negate` and `secp256k1_ec_privkey_negate` 2cee5fd exhaustive tests: add recovery module 678b0e5 exhaustive tests: remove erroneous comment from ecdsa_sig_sign 03ff8c2 group_impl.h: remove unused `secp256k1_ge_set_infinity` function a724d72 configure: add --enable-coverage to set options for coverage analysis b595163 recovery: add tests to cover API misusage 6f8ae2f ecdh: test NULL-checking of arguments 25e3cfb ecdsa_impl: replace scalar if-checks with VERIFY_CHECKs in ecdsa_sig_sign git-subtree-dir: src/secp256k1 git-subtree-split: 84973d393ac240a90b2e1a6538c5368202bc22240.15
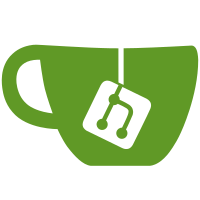
18 changed files with 623 additions and 278 deletions
@ -1,73 +0,0 @@ |
|||||||
/**********************************************************************
|
|
||||||
* Copyright (c) 2014 Pieter Wuille * |
|
||||||
* Distributed under the MIT software license, see the accompanying * |
|
||||||
* file COPYING or http://www.opensource.org/licenses/mit-license.php.*
|
|
||||||
**********************************************************************/ |
|
||||||
|
|
||||||
#include <stdio.h> |
|
||||||
#include <string.h> |
|
||||||
|
|
||||||
#include "include/secp256k1.h" |
|
||||||
#include "include/secp256k1_schnorr.h" |
|
||||||
#include "util.h" |
|
||||||
#include "bench.h" |
|
||||||
|
|
||||||
typedef struct { |
|
||||||
unsigned char key[32]; |
|
||||||
unsigned char sig[64]; |
|
||||||
unsigned char pubkey[33]; |
|
||||||
size_t pubkeylen; |
|
||||||
} benchmark_schnorr_sig_t; |
|
||||||
|
|
||||||
typedef struct { |
|
||||||
secp256k1_context *ctx; |
|
||||||
unsigned char msg[32]; |
|
||||||
benchmark_schnorr_sig_t sigs[64]; |
|
||||||
int numsigs; |
|
||||||
} benchmark_schnorr_verify_t; |
|
||||||
|
|
||||||
static void benchmark_schnorr_init(void* arg) { |
|
||||||
int i, k; |
|
||||||
benchmark_schnorr_verify_t* data = (benchmark_schnorr_verify_t*)arg; |
|
||||||
|
|
||||||
for (i = 0; i < 32; i++) { |
|
||||||
data->msg[i] = 1 + i; |
|
||||||
} |
|
||||||
for (k = 0; k < data->numsigs; k++) { |
|
||||||
secp256k1_pubkey pubkey; |
|
||||||
for (i = 0; i < 32; i++) { |
|
||||||
data->sigs[k].key[i] = 33 + i + k; |
|
||||||
} |
|
||||||
secp256k1_schnorr_sign(data->ctx, data->sigs[k].sig, data->msg, data->sigs[k].key, NULL, NULL); |
|
||||||
data->sigs[k].pubkeylen = 33; |
|
||||||
CHECK(secp256k1_ec_pubkey_create(data->ctx, &pubkey, data->sigs[k].key)); |
|
||||||
CHECK(secp256k1_ec_pubkey_serialize(data->ctx, data->sigs[k].pubkey, &data->sigs[k].pubkeylen, &pubkey, SECP256K1_EC_COMPRESSED)); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
static void benchmark_schnorr_verify(void* arg) { |
|
||||||
int i; |
|
||||||
benchmark_schnorr_verify_t* data = (benchmark_schnorr_verify_t*)arg; |
|
||||||
|
|
||||||
for (i = 0; i < 20000 / data->numsigs; i++) { |
|
||||||
secp256k1_pubkey pubkey; |
|
||||||
data->sigs[0].sig[(i >> 8) % 64] ^= (i & 0xFF); |
|
||||||
CHECK(secp256k1_ec_pubkey_parse(data->ctx, &pubkey, data->sigs[0].pubkey, data->sigs[0].pubkeylen)); |
|
||||||
CHECK(secp256k1_schnorr_verify(data->ctx, data->sigs[0].sig, data->msg, &pubkey) == ((i & 0xFF) == 0)); |
|
||||||
data->sigs[0].sig[(i >> 8) % 64] ^= (i & 0xFF); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
int main(void) { |
|
||||||
benchmark_schnorr_verify_t data; |
|
||||||
|
|
||||||
data.ctx = secp256k1_context_create(SECP256K1_CONTEXT_SIGN | SECP256K1_CONTEXT_VERIFY); |
|
||||||
|
|
||||||
data.numsigs = 1; |
|
||||||
run_benchmark("schnorr_verify", benchmark_schnorr_verify, benchmark_schnorr_init, NULL, &data, 10, 20000); |
|
||||||
|
|
||||||
secp256k1_context_destroy(data.ctx); |
|
||||||
return 0; |
|
||||||
} |
|
Loading…
Reference in new issue