Browse Source
0.159576b01
Enable xvfb in travis to allow running test_bitcoin-qt (Russell Yanofsky)9e6817e
Add new test_bitcoin-qt static library dependencies (Russell Yanofsky)2754ef1
Add simple qt wallet test sending a transaction (Russell Yanofsky)b61b34c
Add braces to if statements in Qt test_main (Russell Yanofsky)cc9503c
Make qt test compatible with TestChain100Setup framework (Russell Yanofsky)91e3035
Make test_bitcoin.cpp compatible with Qt Test framework (Russell Yanofsky) Tree-SHA512: da491181848b8c39138e997ae5ff2df0b16eef2d9cdd0a965229b1a28d4fa862d5f1ef314a1736e5050e88858f329124d15c689659fc6e50fefde769ba24e523
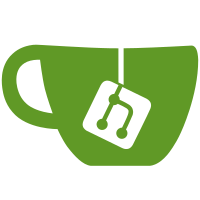
9 changed files with 206 additions and 38 deletions
@ -0,0 +1,104 @@ |
|||||||
|
#include "wallettests.h" |
||||||
|
|
||||||
|
#include "qt/bitcoinamountfield.h" |
||||||
|
#include "qt/optionsmodel.h" |
||||||
|
#include "qt/platformstyle.h" |
||||||
|
#include "qt/qvalidatedlineedit.h" |
||||||
|
#include "qt/sendcoinsdialog.h" |
||||||
|
#include "qt/sendcoinsentry.h" |
||||||
|
#include "qt/transactiontablemodel.h" |
||||||
|
#include "qt/walletmodel.h" |
||||||
|
#include "test/test_bitcoin.h" |
||||||
|
#include "validation.h" |
||||||
|
#include "wallet/wallet.h" |
||||||
|
|
||||||
|
#include <QAbstractButton> |
||||||
|
#include <QApplication> |
||||||
|
#include <QTimer> |
||||||
|
#include <QVBoxLayout> |
||||||
|
|
||||||
|
namespace |
||||||
|
{ |
||||||
|
//! Press "Yes" button in modal send confirmation dialog.
|
||||||
|
void ConfirmSend() |
||||||
|
{ |
||||||
|
QTimer::singleShot(0, Qt::PreciseTimer, []() { |
||||||
|
for (QWidget* widget : QApplication::topLevelWidgets()) { |
||||||
|
if (widget->inherits("SendConfirmationDialog")) { |
||||||
|
SendConfirmationDialog* dialog = qobject_cast<SendConfirmationDialog*>(widget); |
||||||
|
QAbstractButton* button = dialog->button(QMessageBox::Yes); |
||||||
|
button->setEnabled(true); |
||||||
|
button->click(); |
||||||
|
} |
||||||
|
} |
||||||
|
}); |
||||||
|
} |
||||||
|
|
||||||
|
//! Send coins to address and return txid.
|
||||||
|
uint256 SendCoins(CWallet& wallet, SendCoinsDialog& sendCoinsDialog, const CBitcoinAddress& address, CAmount amount) |
||||||
|
{ |
||||||
|
QVBoxLayout* entries = sendCoinsDialog.findChild<QVBoxLayout*>("entries"); |
||||||
|
SendCoinsEntry* entry = qobject_cast<SendCoinsEntry*>(entries->itemAt(0)->widget()); |
||||||
|
entry->findChild<QValidatedLineEdit*>("payTo")->setText(QString::fromStdString(address.ToString())); |
||||||
|
entry->findChild<BitcoinAmountField*>("payAmount")->setValue(amount); |
||||||
|
uint256 txid; |
||||||
|
boost::signals2::scoped_connection c = wallet.NotifyTransactionChanged.connect([&txid](CWallet*, const uint256& hash, ChangeType status) { |
||||||
|
if (status == CT_NEW) txid = hash; |
||||||
|
}); |
||||||
|
ConfirmSend(); |
||||||
|
QMetaObject::invokeMethod(&sendCoinsDialog, "on_sendButton_clicked"); |
||||||
|
return txid; |
||||||
|
} |
||||||
|
|
||||||
|
//! Find index of txid in transaction list.
|
||||||
|
QModelIndex FindTx(const QAbstractItemModel& model, const uint256& txid) |
||||||
|
{ |
||||||
|
QString hash = QString::fromStdString(txid.ToString()); |
||||||
|
int rows = model.rowCount({}); |
||||||
|
for (int row = 0; row < rows; ++row) { |
||||||
|
QModelIndex index = model.index(row, 0, {}); |
||||||
|
if (model.data(index, TransactionTableModel::TxHashRole) == hash) { |
||||||
|
return index; |
||||||
|
} |
||||||
|
} |
||||||
|
return {}; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
//! Simple qt wallet tests.
|
||||||
|
void WalletTests::walletTests() |
||||||
|
{ |
||||||
|
// Set up wallet and chain with 101 blocks (1 mature block for spending).
|
||||||
|
TestChain100Setup test; |
||||||
|
test.CreateAndProcessBlock({}, GetScriptForRawPubKey(test.coinbaseKey.GetPubKey())); |
||||||
|
bitdb.MakeMock(); |
||||||
|
CWallet wallet("wallet_test.dat"); |
||||||
|
bool firstRun; |
||||||
|
wallet.LoadWallet(firstRun); |
||||||
|
{ |
||||||
|
LOCK(wallet.cs_wallet); |
||||||
|
wallet.SetAddressBook(test.coinbaseKey.GetPubKey().GetID(), "", "receive"); |
||||||
|
wallet.AddKeyPubKey(test.coinbaseKey, test.coinbaseKey.GetPubKey()); |
||||||
|
} |
||||||
|
wallet.ScanForWalletTransactions(chainActive.Genesis(), true); |
||||||
|
wallet.SetBroadcastTransactions(true); |
||||||
|
|
||||||
|
// Create widgets for sending coins and listing transactions.
|
||||||
|
std::unique_ptr<const PlatformStyle> platformStyle(PlatformStyle::instantiate("other")); |
||||||
|
SendCoinsDialog sendCoinsDialog(platformStyle.get()); |
||||||
|
OptionsModel optionsModel; |
||||||
|
WalletModel walletModel(platformStyle.get(), &wallet, &optionsModel); |
||||||
|
sendCoinsDialog.setModel(&walletModel); |
||||||
|
|
||||||
|
// Send two transactions, and verify they are added to transaction list.
|
||||||
|
TransactionTableModel* transactionTableModel = walletModel.getTransactionTableModel(); |
||||||
|
QCOMPARE(transactionTableModel->rowCount({}), 101); |
||||||
|
uint256 txid1 = SendCoins(wallet, sendCoinsDialog, CBitcoinAddress(CKeyID()), 5 * COIN); |
||||||
|
uint256 txid2 = SendCoins(wallet, sendCoinsDialog, CBitcoinAddress(CKeyID()), 10 * COIN); |
||||||
|
QCOMPARE(transactionTableModel->rowCount({}), 103); |
||||||
|
QVERIFY(FindTx(*transactionTableModel, txid1).isValid()); |
||||||
|
QVERIFY(FindTx(*transactionTableModel, txid2).isValid()); |
||||||
|
|
||||||
|
bitdb.Flush(true); |
||||||
|
bitdb.Reset(); |
||||||
|
} |
@ -0,0 +1,15 @@ |
|||||||
|
#ifndef BITCOIN_QT_TEST_WALLETTESTS_H |
||||||
|
#define BITCOIN_QT_TEST_WALLETTESTS_H |
||||||
|
|
||||||
|
#include <QObject> |
||||||
|
#include <QTest> |
||||||
|
|
||||||
|
class WalletTests : public QObject |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
private Q_SLOTS: |
||||||
|
void walletTests(); |
||||||
|
}; |
||||||
|
|
||||||
|
#endif // BITCOIN_QT_TEST_WALLETTESTS_H
|
@ -0,0 +1,26 @@ |
|||||||
|
// Copyright (c) 2011-2016 The Bitcoin Core developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#define BOOST_TEST_MODULE Bitcoin Test Suite |
||||||
|
|
||||||
|
#include "net.h" |
||||||
|
|
||||||
|
#include <boost/test/unit_test.hpp> |
||||||
|
|
||||||
|
std::unique_ptr<CConnman> g_connman; |
||||||
|
|
||||||
|
void Shutdown(void* parg) |
||||||
|
{ |
||||||
|
exit(EXIT_SUCCESS); |
||||||
|
} |
||||||
|
|
||||||
|
void StartShutdown() |
||||||
|
{ |
||||||
|
exit(EXIT_SUCCESS); |
||||||
|
} |
||||||
|
|
||||||
|
bool ShutdownRequested() |
||||||
|
{ |
||||||
|
return false; |
||||||
|
} |
Loading…
Reference in new issue