Browse Source
- if 10.8, use user notification center, if <10.8, use growl Signed-off-by: Jonas Schnelli <jonas.schnelli@include7.ch>0.10
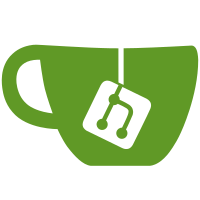
5 changed files with 128 additions and 21 deletions
@ -0,0 +1,25 @@ |
|||||||
|
#ifndef MACNOTIFICATIONHANDLER_H |
||||||
|
#define MACNOTIFICATIONHANDLER_H |
||||||
|
#include <QObject> |
||||||
|
|
||||||
|
/** Macintosh-specific notification handler (supports UserNotificationCenter and Growl).
|
||||||
|
*/ |
||||||
|
class MacNotificationHandler : public QObject |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
public: |
||||||
|
/** shows a 10.8+ UserNotification in the UserNotificationCenter
|
||||||
|
*/ |
||||||
|
void showNotification(const QString &title, const QString &text); |
||||||
|
|
||||||
|
/** executes AppleScript */ |
||||||
|
void sendAppleScript(const QString &script); |
||||||
|
|
||||||
|
/** check if OS can handle UserNotifications */ |
||||||
|
bool hasUserNotificationCenterSupport(void); |
||||||
|
static MacNotificationHandler *instance(); |
||||||
|
}; |
||||||
|
|
||||||
|
|
||||||
|
#endif // MACNOTIFICATIONHANDLER_H
|
@ -0,0 +1,65 @@ |
|||||||
|
#include "macnotificationhandler.h" |
||||||
|
|
||||||
|
#undef slots |
||||||
|
#include <Cocoa/Cocoa.h> |
||||||
|
|
||||||
|
void MacNotificationHandler::showNotification(const QString &title, const QString &text) |
||||||
|
{ |
||||||
|
// check if users OS has support for NSUserNotification |
||||||
|
if(this->hasUserNotificationCenterSupport()) { |
||||||
|
// okay, seems like 10.8+ |
||||||
|
QByteArray utf8 = title.toUtf8(); |
||||||
|
char* cString = (char *)utf8.constData(); |
||||||
|
NSString *titleMac = [[NSString alloc] initWithUTF8String:cString]; |
||||||
|
|
||||||
|
utf8 = text.toUtf8(); |
||||||
|
cString = (char *)utf8.constData(); |
||||||
|
NSString *textMac = [[NSString alloc] initWithUTF8String:cString]; |
||||||
|
|
||||||
|
// do everything weak linked (because we will keep <10.8 compatibility) |
||||||
|
id userNotification = [[NSClassFromString(@"NSUserNotification") alloc] init]; |
||||||
|
[userNotification performSelector:@selector(setTitle:) withObject:titleMac]; |
||||||
|
[userNotification performSelector:@selector(setInformativeText:) withObject:textMac]; |
||||||
|
|
||||||
|
id notificationCenterInstance = [NSClassFromString(@"NSUserNotificationCenter") performSelector:@selector(defaultUserNotificationCenter)]; |
||||||
|
[notificationCenterInstance performSelector:@selector(deliverNotification:) withObject:userNotification]; |
||||||
|
|
||||||
|
[titleMac release]; |
||||||
|
[textMac release]; |
||||||
|
[userNotification release]; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
// sendAppleScript just take a QString and executes it as apple script |
||||||
|
void MacNotificationHandler::sendAppleScript(const QString &script) |
||||||
|
{ |
||||||
|
QByteArray utf8 = script.toUtf8(); |
||||||
|
char* cString = (char *)utf8.constData(); |
||||||
|
NSString *scriptApple = [[NSString alloc] initWithUTF8String:cString]; |
||||||
|
|
||||||
|
NSAppleScript *as = [[NSAppleScript alloc] initWithSource:scriptApple]; |
||||||
|
NSDictionary *err = nil; |
||||||
|
[as executeAndReturnError:&err]; |
||||||
|
[as release]; |
||||||
|
[scriptApple release]; |
||||||
|
} |
||||||
|
|
||||||
|
bool MacNotificationHandler::hasUserNotificationCenterSupport(void) |
||||||
|
{ |
||||||
|
Class possibleClass = NSClassFromString(@"NSUserNotificationCenter"); |
||||||
|
|
||||||
|
// check if users OS has support for NSUserNotification |
||||||
|
if(possibleClass!=nil) { |
||||||
|
return true; |
||||||
|
} |
||||||
|
return false; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
MacNotificationHandler *MacNotificationHandler::instance() |
||||||
|
{ |
||||||
|
static MacNotificationHandler *s_instance = NULL; |
||||||
|
if (!s_instance) |
||||||
|
s_instance = new MacNotificationHandler(); |
||||||
|
return s_instance; |
||||||
|
} |
Loading…
Reference in new issue