Browse Source
0.154ccc12a54
[qa] Rewrite BIP66 functional tests (Suhas Daftuar)d4f0d87b6
[qa] Rewrite BIP65 functional tests (Suhas Daftuar) Pull request description: After122786d0e0
, BIP65 and BIP66 activate at particular fixed heights (without regard to version numbers of blocks below those heights). Rewrite the functional tests to take this into account, and remove two tests that weren't really testing anything. Moves the rewritten functional tests out of the extended test suite, so that they run in travis regularly. Note: I discovered that the ComparisonTestFramework (which the original versions of these p2p tests were written is, has a bug that caused them to not catch obvious errors, eg if you just comment out setting the script flags for these softforks in ConnectBlock, the versions of these tests in master do not fail(!) -- will separately PR a fix for the comparison test framework). Tree-SHA512: 2108b79951f2e980854d0d14ccc0fd1810a43dd4251fd6e3b203e7f104ea55e00650bb1a60a28c2801b4249f46563a8559ac3e3cd39db220914acfed0b3b163d
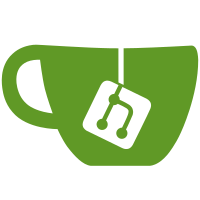
5 changed files with 213 additions and 414 deletions
@ -1,82 +0,0 @@
@@ -1,82 +0,0 @@
|
||||
#!/usr/bin/env python3 |
||||
# Copyright (c) 2015-2016 The Bitcoin Core developers |
||||
# Distributed under the MIT software license, see the accompanying |
||||
# file COPYING or http://www.opensource.org/licenses/mit-license.php. |
||||
"""Test the CHECKLOCKTIMEVERIFY (BIP65) soft-fork logic.""" |
||||
|
||||
from test_framework.test_framework import BitcoinTestFramework |
||||
from test_framework.util import * |
||||
|
||||
class BIP65Test(BitcoinTestFramework): |
||||
def __init__(self): |
||||
super().__init__() |
||||
self.num_nodes = 3 |
||||
self.setup_clean_chain = False |
||||
self.extra_args = [[], ["-blockversion=3"], ["-blockversion=4"]] |
||||
|
||||
def setup_network(self): |
||||
self.setup_nodes() |
||||
connect_nodes(self.nodes[1], 0) |
||||
connect_nodes(self.nodes[2], 0) |
||||
self.sync_all() |
||||
|
||||
def run_test(self): |
||||
cnt = self.nodes[0].getblockcount() |
||||
|
||||
# Mine some old-version blocks |
||||
self.nodes[1].generate(200) |
||||
cnt += 100 |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 100): |
||||
raise AssertionError("Failed to mine 100 version=3 blocks") |
||||
|
||||
# Mine 750 new-version blocks |
||||
for i in range(15): |
||||
self.nodes[2].generate(50) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 850): |
||||
raise AssertionError("Failed to mine 750 version=4 blocks") |
||||
|
||||
# TODO: check that new CHECKLOCKTIMEVERIFY rules are not enforced |
||||
|
||||
# Mine 1 new-version block |
||||
self.nodes[2].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 851): |
||||
raise AssertionError("Failed to mine a version=4 blocks") |
||||
|
||||
# TODO: check that new CHECKLOCKTIMEVERIFY rules are enforced |
||||
|
||||
# Mine 198 new-version blocks |
||||
for i in range(2): |
||||
self.nodes[2].generate(99) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1049): |
||||
raise AssertionError("Failed to mine 198 version=4 blocks") |
||||
|
||||
# Mine 1 old-version block |
||||
self.nodes[1].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1050): |
||||
raise AssertionError("Failed to mine a version=3 block after 949 version=4 blocks") |
||||
|
||||
# Mine 1 new-version blocks |
||||
self.nodes[2].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1051): |
||||
raise AssertionError("Failed to mine a version=4 block") |
||||
|
||||
# Mine 1 old-version blocks. This should fail |
||||
assert_raises_jsonrpc(-1,"CreateNewBlock: TestBlockValidity failed: bad-version(0x00000003)", self.nodes[1].generate, 1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1051): |
||||
raise AssertionError("Accepted a version=3 block after 950 version=4 blocks") |
||||
|
||||
# Mine 1 new-version blocks |
||||
self.nodes[2].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1052): |
||||
raise AssertionError("Failed to mine a version=4 block") |
||||
|
||||
if __name__ == '__main__': |
||||
BIP65Test().main() |
@ -1,81 +0,0 @@
@@ -1,81 +0,0 @@
|
||||
#!/usr/bin/env python3 |
||||
# Copyright (c) 2014-2016 The Bitcoin Core developers |
||||
# Distributed under the MIT software license, see the accompanying |
||||
# file COPYING or http://www.opensource.org/licenses/mit-license.php. |
||||
"""Test the BIP66 changeover logic.""" |
||||
|
||||
from test_framework.test_framework import BitcoinTestFramework |
||||
from test_framework.util import * |
||||
|
||||
class BIP66Test(BitcoinTestFramework): |
||||
def __init__(self): |
||||
super().__init__() |
||||
self.num_nodes = 3 |
||||
self.setup_clean_chain = False |
||||
self.extra_args = [[], ["-blockversion=2"], ["-blockversion=3"]] |
||||
|
||||
def setup_network(self): |
||||
self.setup_nodes() |
||||
connect_nodes(self.nodes[1], 0) |
||||
connect_nodes(self.nodes[2], 0) |
||||
self.sync_all() |
||||
|
||||
def run_test(self): |
||||
cnt = self.nodes[0].getblockcount() |
||||
|
||||
# Mine some old-version blocks |
||||
self.nodes[1].generate(100) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 100): |
||||
raise AssertionError("Failed to mine 100 version=2 blocks") |
||||
|
||||
# Mine 750 new-version blocks |
||||
for i in range(15): |
||||
self.nodes[2].generate(50) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 850): |
||||
raise AssertionError("Failed to mine 750 version=3 blocks") |
||||
|
||||
# TODO: check that new DERSIG rules are not enforced |
||||
|
||||
# Mine 1 new-version block |
||||
self.nodes[2].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 851): |
||||
raise AssertionError("Failed to mine a version=3 blocks") |
||||
|
||||
# TODO: check that new DERSIG rules are enforced |
||||
|
||||
# Mine 198 new-version blocks |
||||
for i in range(2): |
||||
self.nodes[2].generate(99) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1049): |
||||
raise AssertionError("Failed to mine 198 version=3 blocks") |
||||
|
||||
# Mine 1 old-version block |
||||
self.nodes[1].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1050): |
||||
raise AssertionError("Failed to mine a version=2 block after 949 version=3 blocks") |
||||
|
||||
# Mine 1 new-version blocks |
||||
self.nodes[2].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1051): |
||||
raise AssertionError("Failed to mine a version=3 block") |
||||
|
||||
# Mine 1 old-version blocks. This should fail |
||||
assert_raises_jsonrpc(-1, "CreateNewBlock: TestBlockValidity failed: bad-version(0x00000002)", self.nodes[1].generate, 1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1051): |
||||
raise AssertionError("Accepted a version=2 block after 950 version=3 blocks") |
||||
|
||||
# Mine 1 new-version blocks |
||||
self.nodes[2].generate(1) |
||||
self.sync_all() |
||||
if (self.nodes[0].getblockcount() != cnt + 1052): |
||||
raise AssertionError("Failed to mine a version=3 block") |
||||
|
||||
if __name__ == '__main__': |
||||
BIP66Test().main() |
Loading…
Reference in new issue