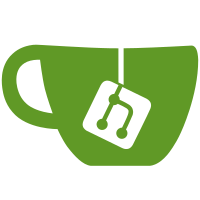
23 changed files with 695 additions and 333 deletions
@ -0,0 +1,60 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<ui version="4.0"> |
||||||
|
<class>KevaEditBookmarkDialog</class> |
||||||
|
<widget class="QDialog" name="KevaEditBookmarkDialog"> |
||||||
|
<property name="geometry"> |
||||||
|
<rect> |
||||||
|
<x>0</x> |
||||||
|
<y>0</y> |
||||||
|
<width>400</width> |
||||||
|
<height>100</height> |
||||||
|
</rect> |
||||||
|
</property> |
||||||
|
<property name="windowTitle"> |
||||||
|
<string notr="true">Bookmark Name</string> |
||||||
|
</property> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout_2"> |
||||||
|
<item> |
||||||
|
<layout class="QGridLayout" name="gridLayout"> |
||||||
|
<item row="2" column="0"> |
||||||
|
<widget class="QLabel" name="label_2"> |
||||||
|
<property name="toolTip"> |
||||||
|
<string>Bookmark name.</string> |
||||||
|
</property> |
||||||
|
<property name="text"> |
||||||
|
<string>Name:</string> |
||||||
|
</property> |
||||||
|
<property name="alignment"> |
||||||
|
<set>Qt::AlignRight|Qt::AlignTrailing|Qt::AlignVCenter</set> |
||||||
|
</property> |
||||||
|
<property name="buddy"> |
||||||
|
<cstring>bookmarkName</cstring> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item row="2" column="2"> |
||||||
|
<widget class="QLineEdit" name="bookmarkName"> |
||||||
|
<property name="toolTip"> |
||||||
|
<string>This pane allows change of bookmark name</string> |
||||||
|
</property> |
||||||
|
<property name="readOnly"> |
||||||
|
<bool>false</bool> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QDialogButtonBox" name="buttonBox"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="standardButtons"> |
||||||
|
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Save</set> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
<resources/> |
||||||
|
</ui> |
@ -0,0 +1,53 @@ |
|||||||
|
// Copyright (c) 2011-2017 The Bitcoin Core developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#include <qt/kevaeditbookmarkdialog.h> |
||||||
|
#include <qt/forms/ui_kevaeditbookmarkdialog.h> |
||||||
|
#include <qt/kevabookmarksdialog.h> |
||||||
|
|
||||||
|
#include <qt/kevatablemodel.h> |
||||||
|
#include <qt/kevadialog.h> |
||||||
|
|
||||||
|
#include <QPushButton> |
||||||
|
#include <QModelIndex> |
||||||
|
|
||||||
|
KevaEditBookmarkDialog::KevaEditBookmarkDialog(QWidget *parent, const QString& id, const QString& name) : |
||||||
|
QDialog(parent), id(id), name(name), |
||||||
|
ui(new Ui::KevaEditBookmarkDialog) |
||||||
|
{ |
||||||
|
ui->setupUi(this); |
||||||
|
ui->bookmarkName->setText(name); |
||||||
|
this->parentDialog = (KevaBookmarksDialog*)parent; |
||||||
|
|
||||||
|
connect(ui->buttonBox->button(QDialogButtonBox::Cancel), SIGNAL(clicked()), this, SLOT(close())); |
||||||
|
connect(ui->buttonBox->button(QDialogButtonBox::Save), SIGNAL(clicked()), this, SLOT(save())); |
||||||
|
connect(ui->bookmarkName, SIGNAL(textChanged(const QString &)), this, SLOT(onNameChanged(const QString &))); |
||||||
|
ui->buttonBox->button(QDialogButtonBox::Save)->setEnabled(false); |
||||||
|
} |
||||||
|
|
||||||
|
void KevaEditBookmarkDialog::onNameChanged(const QString & name) |
||||||
|
{ |
||||||
|
int length = name.length(); |
||||||
|
bool enabled = length > 0; |
||||||
|
ui->buttonBox->button(QDialogButtonBox::Save)->setEnabled(enabled); |
||||||
|
this->name = name; |
||||||
|
} |
||||||
|
|
||||||
|
void KevaEditBookmarkDialog::save() |
||||||
|
{ |
||||||
|
KevaDialog* dialog = (KevaDialog*)this->parentWidget(); |
||||||
|
QString bookmarkText = ui->bookmarkName->text(); |
||||||
|
this->parentDialog->saveName(id, name); |
||||||
|
QDialog::close(); |
||||||
|
} |
||||||
|
|
||||||
|
void KevaEditBookmarkDialog::close() |
||||||
|
{ |
||||||
|
QDialog::close(); |
||||||
|
} |
||||||
|
|
||||||
|
KevaEditBookmarkDialog::~KevaEditBookmarkDialog() |
||||||
|
{ |
||||||
|
delete ui; |
||||||
|
} |
@ -0,0 +1,41 @@ |
|||||||
|
// Copyright (c) 2011-2014 The Bitcoin Core developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#ifndef BITCOIN_QT_KEVAEDITBOOKMARKDIALOG_H |
||||||
|
#define BITCOIN_QT_KEVAEDITBOOKMARKDIALOG_H |
||||||
|
|
||||||
|
#include <QObject> |
||||||
|
#include <QString> |
||||||
|
|
||||||
|
#include <QDialog> |
||||||
|
|
||||||
|
namespace Ui { |
||||||
|
class KevaEditBookmarkDialog; |
||||||
|
} |
||||||
|
|
||||||
|
class KevaBookmarksDialog; |
||||||
|
|
||||||
|
|
||||||
|
/** Dialog showing namepsace creation. */ |
||||||
|
class KevaEditBookmarkDialog : public QDialog |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
public: |
||||||
|
explicit KevaEditBookmarkDialog(QWidget *parent, const QString& id, const QString& name); |
||||||
|
~KevaEditBookmarkDialog(); |
||||||
|
|
||||||
|
public Q_SLOTS: |
||||||
|
void save(); |
||||||
|
void close(); |
||||||
|
void onNameChanged(const QString & ns); |
||||||
|
|
||||||
|
private: |
||||||
|
Ui::KevaEditBookmarkDialog *ui; |
||||||
|
QString id; |
||||||
|
QString name; |
||||||
|
KevaBookmarksDialog* parentDialog; |
||||||
|
}; |
||||||
|
|
||||||
|
#endif // BITCOIN_QT_KEVAEDITBOOKMARKDIALOG_H
|
Before Width: | Height: | Size: 6.3 KiB After Width: | Height: | Size: 5.5 KiB |
Before Width: | Height: | Size: 10 KiB After Width: | Height: | Size: 3.5 KiB |
Before Width: | Height: | Size: 63 KiB After Width: | Height: | Size: 38 KiB |
Loading…
Reference in new issue