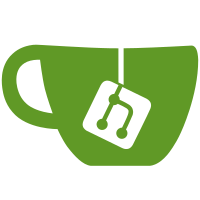
39 changed files with 2294 additions and 1905 deletions
@ -0,0 +1,38 @@
@@ -0,0 +1,38 @@
|
||||
--- |
||||
name: "boost" |
||||
suites: |
||||
- "lucid" |
||||
architectures: |
||||
- "i386" |
||||
packages: |
||||
- "mingw32" |
||||
- "faketime" |
||||
- "zip" |
||||
reference_datetime: "2011-01-30 00:00:00" |
||||
remotes: [] |
||||
files: |
||||
- "boost_1_47_0.tar.bz2" |
||||
script: | |
||||
TMPDIR="$HOME/tmpdir" |
||||
mkdir -p $TMPDIR/bin/$GBUILD_BITS $TMPDIR/include |
||||
tar xjf boost_1_47_0.tar.bz2 |
||||
cd boost_1_47_0 |
||||
echo "using gcc : 4.4 : i586-mingw32msvc-g++ |
||||
: |
||||
<rc>i586-mingw32msvc-windres |
||||
<archiver>i586-mingw32msvc-ar |
||||
<cxxflags>-frandom-seed=boost1 |
||||
;" > user-config.jam |
||||
./bootstrap.sh --without-icu |
||||
./bjam toolset=gcc target-os=windows threadapi=win32 threading=multi variant=release link=static --user-config=user-config.jam --without-mpi --without-python -sNO_BZIP2=1 -sNO_ZLIB=1 --layout=tagged --build-type=complete $MAKEOPTS stage |
||||
for lib in chrono date_time exception filesystem graph iostreams math_c99f math_c99l math_c99 math_tr1f math_tr1l math_tr1 prg_exec_monitor program_options random regex serialization signals system test_exec_monitor thread_win32 unit_test_framework wave wserialization; do |
||||
mkdir $lib |
||||
(cd $lib ; ar xf ../stage/lib/libboost_${lib}-mt-s.a) |
||||
mv $lib $TMPDIR/bin/$GBUILD_BITS |
||||
done |
||||
cp -a boost $TMPDIR/include |
||||
cd $TMPDIR |
||||
export LD_PRELOAD=/usr/lib/faketime/libfaketime.so.1 |
||||
export FAKETIME=$REFERENCE_DATETIME |
||||
zip -r boost-win32-1.47.0-gitian.zip * |
||||
cp boost-win32-1.47.0-gitian.zip $OUTDIR |
@ -0,0 +1,6 @@
@@ -0,0 +1,6 @@
|
||||
|
||||
This is a 'getwork' CPU mining client for bitcoin. |
||||
|
||||
It is pure-python, and therefore very, very slow. The purpose is to |
||||
provide a reference implementation of a miner, for study. |
||||
|
@ -0,0 +1,32 @@
@@ -0,0 +1,32 @@
|
||||
|
||||
# |
||||
# RPC login details |
||||
# |
||||
host=127.0.0.1 |
||||
port=8332 |
||||
|
||||
rpcuser=myusername |
||||
rpcpass=mypass |
||||
|
||||
|
||||
# |
||||
# mining details |
||||
# |
||||
|
||||
threads=4 |
||||
|
||||
# periodic rate for requesting new work, if solution not found |
||||
scantime=60 |
||||
|
||||
|
||||
# |
||||
# misc. |
||||
# |
||||
|
||||
# not really used right now |
||||
logdir=/tmp/pyminer |
||||
|
||||
# set to 1, to enable hashmeter output |
||||
hashmeter=0 |
||||
|
||||
|
@ -0,0 +1,252 @@
@@ -0,0 +1,252 @@
|
||||
#!/usr/bin/python |
||||
# |
||||
# Copyright (c) 2011 The Bitcoin developers |
||||
# Distributed under the MIT/X11 software license, see the accompanying |
||||
# file license.txt or http://www.opensource.org/licenses/mit-license.php. |
||||
# |
||||
|
||||
import time |
||||
import json |
||||
import pprint |
||||
import hashlib |
||||
import struct |
||||
import re |
||||
import base64 |
||||
import httplib |
||||
import sys |
||||
from multiprocessing import Process |
||||
|
||||
ERR_SLEEP = 15 |
||||
MAX_NONCE = 1000000L |
||||
|
||||
settings = {} |
||||
pp = pprint.PrettyPrinter(indent=4) |
||||
|
||||
class BitcoinRPC: |
||||
OBJID = 1 |
||||
|
||||
def __init__(self, host, port, username, password): |
||||
authpair = "%s:%s" % (username, password) |
||||
self.authhdr = "Basic %s" % (base64.b64encode(authpair)) |
||||
self.conn = httplib.HTTPConnection(host, port, False, 30) |
||||
def rpc(self, method, params=None): |
||||
self.OBJID += 1 |
||||
obj = { 'version' : '1.1', |
||||
'method' : method, |
||||
'id' : self.OBJID } |
||||
if params is None: |
||||
obj['params'] = [] |
||||
else: |
||||
obj['params'] = params |
||||
self.conn.request('POST', '/', json.dumps(obj), |
||||
{ 'Authorization' : self.authhdr, |
||||
'Content-type' : 'application/json' }) |
||||
|
||||
resp = self.conn.getresponse() |
||||
if resp is None: |
||||
print "JSON-RPC: no response" |
||||
return None |
||||
|
||||
body = resp.read() |
||||
resp_obj = json.loads(body) |
||||
if resp_obj is None: |
||||
print "JSON-RPC: cannot JSON-decode body" |
||||
return None |
||||
if 'error' in resp_obj and resp_obj['error'] != None: |
||||
return resp_obj['error'] |
||||
if 'result' not in resp_obj: |
||||
print "JSON-RPC: no result in object" |
||||
return None |
||||
|
||||
return resp_obj['result'] |
||||
def getblockcount(self): |
||||
return self.rpc('getblockcount') |
||||
def getwork(self, data=None): |
||||
return self.rpc('getwork', data) |
||||
|
||||
def uint32(x): |
||||
return x & 0xffffffffL |
||||
|
||||
def bytereverse(x): |
||||
return uint32(( ((x) << 24) | (((x) << 8) & 0x00ff0000) | |
||||
(((x) >> 8) & 0x0000ff00) | ((x) >> 24) )) |
||||
|
||||
def bufreverse(in_buf): |
||||
out_words = [] |
||||
for i in range(0, len(in_buf), 4): |
||||
word = struct.unpack('@I', in_buf[i:i+4])[0] |
||||
out_words.append(struct.pack('@I', bytereverse(word))) |
||||
return ''.join(out_words) |
||||
|
||||
def wordreverse(in_buf): |
||||
out_words = [] |
||||
for i in range(0, len(in_buf), 4): |
||||
out_words.append(in_buf[i:i+4]) |
||||
out_words.reverse() |
||||
return ''.join(out_words) |
||||
|
||||
class Miner: |
||||
def __init__(self, id): |
||||
self.id = id |
||||
self.max_nonce = MAX_NONCE |
||||
|
||||
def work(self, datastr, targetstr): |
||||
# decode work data hex string to binary |
||||
static_data = datastr.decode('hex') |
||||
static_data = bufreverse(static_data) |
||||
|
||||
# the first 76b of 80b do not change |
||||
blk_hdr = static_data[:76] |
||||
|
||||
# decode 256-bit target value |
||||
targetbin = targetstr.decode('hex') |
||||
targetbin = targetbin[::-1] # byte-swap and dword-swap |
||||
targetbin_str = targetbin.encode('hex') |
||||
target = long(targetbin_str, 16) |
||||
|
||||
# pre-hash first 76b of block header |
||||
static_hash = hashlib.sha256() |
||||
static_hash.update(blk_hdr) |
||||
|
||||
for nonce in xrange(self.max_nonce): |
||||
|
||||
# encode 32-bit nonce value |
||||
nonce_bin = struct.pack("<I", nonce) |
||||
|
||||
# hash final 4b, the nonce value |
||||
hash1_o = static_hash.copy() |
||||
hash1_o.update(nonce_bin) |
||||
hash1 = hash1_o.digest() |
||||
|
||||
# sha256 hash of sha256 hash |
||||
hash_o = hashlib.sha256() |
||||
hash_o.update(hash1) |
||||
hash = hash_o.digest() |
||||
|
||||
# quick test for winning solution: high 32 bits zero? |
||||
if hash[-4:] != '\0\0\0\0': |
||||
continue |
||||
|
||||
# convert binary hash to 256-bit Python long |
||||
hash = bufreverse(hash) |
||||
hash = wordreverse(hash) |
||||
|
||||
hash_str = hash.encode('hex') |
||||
l = long(hash_str, 16) |
||||
|
||||
# proof-of-work test: hash < target |
||||
if l < target: |
||||
print time.asctime(), "PROOF-OF-WORK found: %064x" % (l,) |
||||
return (nonce + 1, nonce_bin) |
||||
else: |
||||
print time.asctime(), "PROOF-OF-WORK false positive %064x" % (l,) |
||||
# return (nonce + 1, nonce_bin) |
||||
|
||||
return (nonce + 1, None) |
||||
|
||||
def submit_work(self, rpc, original_data, nonce_bin): |
||||
nonce_bin = bufreverse(nonce_bin) |
||||
nonce = nonce_bin.encode('hex') |
||||
solution = original_data[:152] + nonce + original_data[160:256] |
||||
param_arr = [ solution ] |
||||
result = rpc.getwork(param_arr) |
||||
print time.asctime(), "--> Upstream RPC result:", result |
||||
|
||||
def iterate(self, rpc): |
||||
work = rpc.getwork() |
||||
if work is None: |
||||
time.sleep(ERR_SLEEP) |
||||
return |
||||
if 'data' not in work or 'target' not in work: |
||||
time.sleep(ERR_SLEEP) |
||||
return |
||||
|
||||
time_start = time.time() |
||||
|
||||
(hashes_done, nonce_bin) = self.work(work['data'], |
||||
work['target']) |
||||
|
||||
time_end = time.time() |
||||
time_diff = time_end - time_start |
||||
|
||||
self.max_nonce = long( |
||||
(hashes_done * settings['scantime']) / time_diff) |
||||
if self.max_nonce > 0xfffffffaL: |
||||
self.max_nonce = 0xfffffffaL |
||||
|
||||
if settings['hashmeter']: |
||||
print "HashMeter(%d): %d hashes, %.2f Khash/sec" % ( |
||||
self.id, hashes_done, |
||||
(hashes_done / 1000.0) / time_diff) |
||||
|
||||
if nonce_bin is not None: |
||||
self.submit_work(rpc, work['data'], nonce_bin) |
||||
|
||||
def loop(self): |
||||
rpc = BitcoinRPC(settings['host'], settings['port'], |
||||
settings['rpcuser'], settings['rpcpass']) |
||||
if rpc is None: |
||||
return |
||||
|
||||
while True: |
||||
self.iterate(rpc) |
||||
|
||||
def miner_thread(id): |
||||
miner = Miner(id) |
||||
miner.loop() |
||||
|
||||
if __name__ == '__main__': |
||||
if len(sys.argv) != 2: |
||||
print "Usage: pyminer.py CONFIG-FILE" |
||||
sys.exit(1) |
||||
|
||||
f = open(sys.argv[1]) |
||||
for line in f: |
||||
# skip comment lines |
||||
m = re.search('^\s*#', line) |
||||
if m: |
||||
continue |
||||
|
||||
# parse key=value lines |
||||
m = re.search('^(\w+)\s*=\s*(\S.*)$', line) |
||||
if m is None: |
||||
continue |
||||
settings[m.group(1)] = m.group(2) |
||||
f.close() |
||||
|
||||
if 'host' not in settings: |
||||
settings['host'] = '127.0.0.1' |
||||
if 'port' not in settings: |
||||
settings['port'] = 8332 |
||||
if 'threads' not in settings: |
||||
settings['threads'] = 1 |
||||
if 'hashmeter' not in settings: |
||||
settings['hashmeter'] = 0 |
||||
if 'scantime' not in settings: |
||||
settings['scantime'] = 30L |
||||
if 'rpcuser' not in settings or 'rpcpass' not in settings: |
||||
print "Missing username and/or password in cfg file" |
||||
sys.exit(1) |
||||
|
||||
settings['port'] = int(settings['port']) |
||||
settings['threads'] = int(settings['threads']) |
||||
settings['hashmeter'] = int(settings['hashmeter']) |
||||
settings['scantime'] = long(settings['scantime']) |
||||
|
||||
thr_list = [] |
||||
for thr_id in range(settings['threads']): |
||||
p = Process(target=miner_thread, args=(thr_id,)) |
||||
p.start() |
||||
thr_list.append(p) |
||||
time.sleep(1) # stagger threads |
||||
|
||||
print settings['threads'], "mining threads started" |
||||
|
||||
print time.asctime(), "Miner Starts - %s:%s" % (settings['host'], settings['port']) |
||||
try: |
||||
for thr_proc in thr_list: |
||||
thr_proc.join() |
||||
except KeyboardInterrupt: |
||||
pass |
||||
print time.asctime(), "Miner Stops - %s:%s" % (settings['host'], settings['port']) |
||||
|
@ -1,4 +0,0 @@
@@ -1,4 +0,0 @@
|
||||
This folder contains two patches which are applied to wxWidgets |
||||
2.9.1 before building the wxWidgets which is used for release |
||||
versions of bitcoin. They make the GUI show up on newer OSs |
||||
with new libgtks, such as Ubuntu 11.04. |
@ -1,86 +0,0 @@
@@ -1,86 +0,0 @@
|
||||
--- /wxWidgets/trunk/src/gtk/toplevel.cpp (revision 67326)
|
||||
+++ /wxWidgets/trunk/src/gtk/toplevel.cpp (revision 67496)
|
||||
@@ -72,4 +72,8 @@
|
||||
// send any activate events at all |
||||
static int g_sendActivateEvent = -1; |
||||
+
|
||||
+// Whether _NET_REQUEST_FRAME_EXTENTS support is working
|
||||
+// 0 == not tested yet, 1 == working, 2 == broken
|
||||
+static int gs_requestFrameExtentsStatus;
|
||||
|
||||
//----------------------------------------------------------------------------- |
||||
@@ -432,4 +436,12 @@
|
||||
if (event->state == GDK_PROPERTY_NEW_VALUE && event->atom == property) |
||||
{ |
||||
+ if (win->m_netFrameExtentsTimerId)
|
||||
+ {
|
||||
+ // WM support for _NET_REQUEST_FRAME_EXTENTS is working
|
||||
+ gs_requestFrameExtentsStatus = 1;
|
||||
+ g_source_remove(win->m_netFrameExtentsTimerId);
|
||||
+ win->m_netFrameExtentsTimerId = 0;
|
||||
+ }
|
||||
+
|
||||
wxSize decorSize = win->m_decorSize; |
||||
int left, right, top, bottom; |
||||
@@ -439,4 +451,22 @@
|
||||
win->GTKUpdateDecorSize(decorSize); |
||||
} |
||||
+ return false;
|
||||
+}
|
||||
+}
|
||||
+
|
||||
+extern "C" {
|
||||
+static gboolean request_frame_extents_timeout(void* data)
|
||||
+{
|
||||
+ // WM support for _NET_REQUEST_FRAME_EXTENTS is broken
|
||||
+ gs_requestFrameExtentsStatus = 2;
|
||||
+ gdk_threads_enter();
|
||||
+ wxTopLevelWindowGTK* win = static_cast<wxTopLevelWindowGTK*>(data);
|
||||
+ win->m_netFrameExtentsTimerId = 0;
|
||||
+ wxSize decorSize = win->m_decorSize;
|
||||
+ int left, right, top, bottom;
|
||||
+ if (wxGetFrameExtents(gtk_widget_get_window(win->m_widget), &left, &right, &top, &bottom))
|
||||
+ decorSize.Set(left + right, top + bottom);
|
||||
+ win->GTKUpdateDecorSize(decorSize);
|
||||
+ gdk_threads_leave();
|
||||
return false; |
||||
} |
||||
@@ -459,4 +489,5 @@
|
||||
m_deferShowAllowed = true; |
||||
m_updateDecorSize = true; |
||||
+ m_netFrameExtentsTimerId = 0;
|
||||
|
||||
m_urgency_hint = -2; |
||||
@@ -811,5 +842,6 @@
|
||||
if (deferShow) |
||||
{ |
||||
- deferShow = m_deferShowAllowed && !GTK_WIDGET_REALIZED(m_widget);
|
||||
+ deferShow = gs_requestFrameExtentsStatus != 2 &&
|
||||
+ m_deferShowAllowed && !gtk_widget_get_realized(m_widget);
|
||||
if (deferShow) |
||||
{ |
||||
@@ -829,11 +861,4 @@
|
||||
// GetSize()/SetSize() because it makes window bigger between each |
||||
// restore and save. |
||||
- m_updateDecorSize = deferShow;
|
||||
- }
|
||||
- if (deferShow)
|
||||
- {
|
||||
- // Fluxbox support for _NET_REQUEST_FRAME_EXTENTS is broken
|
||||
- const char* name = gdk_x11_screen_get_window_manager_name(screen);
|
||||
- deferShow = strcmp(name, "Fluxbox") != 0;
|
||||
m_updateDecorSize = deferShow; |
||||
} |
||||
@@ -875,4 +900,12 @@
|
||||
(XEvent*)&xevent); |
||||
|
||||
+ if (gs_requestFrameExtentsStatus == 0)
|
||||
+ {
|
||||
+ // if WM does not respond to request within 1 second,
|
||||
+ // we assume support for _NET_REQUEST_FRAME_EXTENTS is not working
|
||||
+ m_netFrameExtentsTimerId =
|
||||
+ g_timeout_add(1000, request_frame_extents_timeout, this);
|
||||
+ }
|
||||
+
|
||||
// defer calling gtk_widget_show() |
||||
m_isShown = true; |
@ -1,9 +0,0 @@
@@ -1,9 +0,0 @@
|
||||
--- /wxWidgets/trunk/include/wx/gtk/toplevel.h (revision 65373)
|
||||
+++ /wxWidgets/trunk/include/wx/gtk/toplevel.h (revision 67496)
|
||||
@@ -114,4 +114,6 @@
|
||||
// wxUSER_ATTENTION_ERROR difference, -2 for no hint, -1 for ERROR hint, rest for GtkTimeout handle. |
||||
int m_urgency_hint; |
||||
+ // timer for detecting WM with broken _NET_REQUEST_FRAME_EXTENTS handling
|
||||
+ unsigned m_netFrameExtentsTimerId;
|
||||
|
||||
// return the size of the window without WM decorations |
@ -0,0 +1,40 @@
@@ -0,0 +1,40 @@
|
||||
--- |
||||
name: "wxwidgets" |
||||
suites: |
||||
- "lucid" |
||||
architectures: |
||||
- "i386" |
||||
packages: |
||||
- "mingw32" |
||||
- "faketime" |
||||
- "zip" |
||||
reference_datetime: "2011-01-30 00:00:00" |
||||
remotes: [] |
||||
files: |
||||
- "wxWidgets-2.9.2.tar.bz2" |
||||
script: | |
||||
INSTDIR="$HOME/install" |
||||
TMPDIR="$HOME/tmpdir" |
||||
export LIBRARY_PATH="$INSTDIR/lib" |
||||
# |
||||
tar xjf wxWidgets-2.9.2.tar.bz2 |
||||
cd wxWidgets-2.9.2 |
||||
CXXFLAGS=-frandom-seed=wx1 ./configure --host=i586-mingw32msvc --build=i686-linux --prefix=$INSTDIR --disable-shared --enable-monolithic --without-libpng --disable-svg |
||||
perl -i -p -e "s/__TIME__/\"$REFERENCE_TIME\"/;s/__DATE__/\"$REFERENCE_DATE\"/" include/wx/chartype.h |
||||
make $MAKEOPTS install |
||||
mkdir $TMPDIR |
||||
cd $TMPDIR |
||||
cp -af $INSTDIR/include . |
||||
mkdir -p $TMPDIR/bin/$GBUILD_BITS |
||||
cd $TMPDIR/bin/$GBUILD_BITS |
||||
cp -af $INSTDIR/lib/wx . |
||||
for lib in wx_mswu; do |
||||
mkdir $lib |
||||
(cd $lib ; ar xf $INSTDIR/lib/lib${lib}-2.9-i586-mingw32msvc.a) |
||||
done |
||||
chmod -R +w $TMPDIR |
||||
cd $TMPDIR |
||||
export LD_PRELOAD=/usr/lib/faketime/libfaketime.so.1 |
||||
export FAKETIME=$REFERENCE_DATETIME |
||||
zip -r wxwidgets-win32-2.9.2-gitian.zip * |
||||
cp wxwidgets-win32-2.9.2-gitian.zip $OUTDIR |
@ -0,0 +1,42 @@
@@ -0,0 +1,42 @@
|
||||
--- |
||||
name: "wxwidgets" |
||||
suites: |
||||
- "lucid" |
||||
architectures: |
||||
- "i386" |
||||
- "amd64" |
||||
packages: |
||||
- "libxxf86vm-dev" |
||||
- "libgtk2.0-dev" |
||||
- "faketime" |
||||
- "zip" |
||||
reference_datetime: "2011-01-30 00:00:00" |
||||
remotes: [] |
||||
files: |
||||
- "wxWidgets-2.9.2.tar.bz2" |
||||
script: | |
||||
INSTDIR="$HOME/install" |
||||
TMPDIR="$HOME/tmpdir" |
||||
export LIBRARY_PATH="$INSTDIR/lib" |
||||
# |
||||
tar xjf wxWidgets-2.9.2.tar.bz2 |
||||
cd wxWidgets-2.9.2 |
||||
./configure --prefix=$INSTDIR --enable-monolithic --disable-shared |
||||
perl -i -p -e "s/__TIME__/\"$REFERENCE_TIME\"/;s/__DATE__/\"$REFERENCE_DATE\"/" include/wx/chartype.h |
||||
make $MAKEOPTS install |
||||
mkdir $TMPDIR |
||||
cd $TMPDIR |
||||
cp -af $INSTDIR/include . |
||||
mkdir -p $TMPDIR/bin/$GBUILD_BITS |
||||
cd $TMPDIR/bin/$GBUILD_BITS |
||||
cp -af $INSTDIR/lib/wx . |
||||
for lib in wxtiff wxregexu wx_gtk2u; do |
||||
mkdir $lib |
||||
(cd $lib ; ar xf $INSTDIR/lib/lib${lib}-2.9.a) |
||||
done |
||||
chmod -R +w $TMPDIR |
||||
cd $TMPDIR |
||||
export LD_PRELOAD=/usr/lib/faketime/libfaketime.so.1 |
||||
export FAKETIME=$REFERENCE_DATETIME |
||||
zip -r wxWidgets-2.9.2-gitian.zip * |
||||
cp wxWidgets-2.9.2-gitian.zip $OUTDIR |
File diff suppressed because it is too large
Load Diff
@ -0,0 +1,312 @@
@@ -0,0 +1,312 @@
|
||||
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
||||
// Copyright (c) 2011 The Bitcoin developers
|
||||
// Distributed under the MIT/X11 software license, see the accompanying
|
||||
// file license.txt or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#include "protocol.h" |
||||
#include "util.h" |
||||
|
||||
#ifndef __WXMSW__ |
||||
# include <arpa/inet.h> |
||||
#endif |
||||
|
||||
// Prototypes from net.h, but that header (currently) stinks, can't #include it without breaking things
|
||||
bool Lookup(const char *pszName, std::vector<CAddress>& vaddr, int nServices, int nMaxSolutions, bool fAllowLookup = false, int portDefault = 0, bool fAllowPort = false); |
||||
bool Lookup(const char *pszName, CAddress& addr, int nServices, bool fAllowLookup = false, int portDefault = 0, bool fAllowPort = false); |
||||
|
||||
static const unsigned char pchIPv4[12] = { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0xff, 0xff }; |
||||
static const char* ppszTypeName[] = |
||||
{ |
||||
"ERROR", |
||||
"tx", |
||||
"block", |
||||
}; |
||||
|
||||
CMessageHeader::CMessageHeader() |
||||
{ |
||||
memcpy(pchMessageStart, ::pchMessageStart, sizeof(pchMessageStart)); |
||||
memset(pchCommand, 0, sizeof(pchCommand)); |
||||
pchCommand[1] = 1; |
||||
nMessageSize = -1; |
||||
nChecksum = 0; |
||||
} |
||||
|
||||
CMessageHeader::CMessageHeader(const char* pszCommand, unsigned int nMessageSizeIn) |
||||
{ |
||||
memcpy(pchMessageStart, ::pchMessageStart, sizeof(pchMessageStart)); |
||||
strncpy(pchCommand, pszCommand, COMMAND_SIZE); |
||||
nMessageSize = nMessageSizeIn; |
||||
nChecksum = 0; |
||||
} |
||||
|
||||
std::string CMessageHeader::GetCommand() const |
||||
{ |
||||
if (pchCommand[COMMAND_SIZE-1] == 0) |
||||
return std::string(pchCommand, pchCommand + strlen(pchCommand)); |
||||
else |
||||
return std::string(pchCommand, pchCommand + COMMAND_SIZE); |
||||
} |
||||
|
||||
bool CMessageHeader::IsValid() const |
||||
{ |
||||
// Check start string
|
||||
if (memcmp(pchMessageStart, ::pchMessageStart, sizeof(pchMessageStart)) != 0) |
||||
return false; |
||||
|
||||
// Check the command string for errors
|
||||
for (const char* p1 = pchCommand; p1 < pchCommand + COMMAND_SIZE; p1++) |
||||
{ |
||||
if (*p1 == 0) |
||||
{ |
||||
// Must be all zeros after the first zero
|
||||
for (; p1 < pchCommand + COMMAND_SIZE; p1++) |
||||
if (*p1 != 0) |
||||
return false; |
||||
} |
||||
else if (*p1 < ' ' || *p1 > 0x7E) |
||||
return false; |
||||
} |
||||
|
||||
// Message size
|
||||
if (nMessageSize > MAX_SIZE) |
||||
{ |
||||
printf("CMessageHeader::IsValid() : (%s, %u bytes) nMessageSize > MAX_SIZE\n", GetCommand().c_str(), nMessageSize); |
||||
return false; |
||||
} |
||||
|
||||
return true; |
||||
} |
||||
|
||||
CAddress::CAddress() |
||||
{ |
||||
Init(); |
||||
} |
||||
|
||||
CAddress::CAddress(unsigned int ipIn, unsigned short portIn, uint64 nServicesIn) |
||||
{ |
||||
Init(); |
||||
ip = ipIn; |
||||
port = htons(portIn == 0 ? GetDefaultPort() : portIn); |
||||
nServices = nServicesIn; |
||||
} |
||||
|
||||
CAddress::CAddress(const struct sockaddr_in& sockaddr, uint64 nServicesIn) |
||||
{ |
||||
Init(); |
||||
ip = sockaddr.sin_addr.s_addr; |
||||
port = sockaddr.sin_port; |
||||
nServices = nServicesIn; |
||||
} |
||||
|
||||
CAddress::CAddress(const char* pszIn, int portIn, bool fNameLookup, uint64 nServicesIn) |
||||
{ |
||||
Init(); |
||||
Lookup(pszIn, *this, nServicesIn, fNameLookup, portIn); |
||||
} |
||||
|
||||
CAddress::CAddress(const char* pszIn, bool fNameLookup, uint64 nServicesIn) |
||||
{ |
||||
Init(); |
||||
Lookup(pszIn, *this, nServicesIn, fNameLookup, 0, true); |
||||
} |
||||
|
||||
CAddress::CAddress(std::string strIn, int portIn, bool fNameLookup, uint64 nServicesIn) |
||||
{ |
||||
Init(); |
||||
Lookup(strIn.c_str(), *this, nServicesIn, fNameLookup, portIn); |
||||
} |
||||
|
||||
CAddress::CAddress(std::string strIn, bool fNameLookup, uint64 nServicesIn) |
||||
{ |
||||
Init(); |
||||
Lookup(strIn.c_str(), *this, nServicesIn, fNameLookup, 0, true); |
||||
} |
||||
|
||||
void CAddress::Init() |
||||
{ |
||||
nServices = NODE_NETWORK; |
||||
memcpy(pchReserved, pchIPv4, sizeof(pchReserved)); |
||||
ip = INADDR_NONE; |
||||
port = htons(GetDefaultPort()); |
||||
nTime = 100000000; |
||||
nLastTry = 0; |
||||
} |
||||
|
||||
bool operator==(const CAddress& a, const CAddress& b) |
||||
{ |
||||
return (memcmp(a.pchReserved, b.pchReserved, sizeof(a.pchReserved)) == 0 && |
||||
a.ip == b.ip && |
||||
a.port == b.port); |
||||
} |
||||
|
||||
bool operator!=(const CAddress& a, const CAddress& b) |
||||
{ |
||||
return (!(a == b)); |
||||
} |
||||
|
||||
bool operator<(const CAddress& a, const CAddress& b) |
||||
{ |
||||
int ret = memcmp(a.pchReserved, b.pchReserved, sizeof(a.pchReserved)); |
||||
if (ret < 0) |
||||
return true; |
||||
else if (ret == 0) |
||||
{ |
||||
if (ntohl(a.ip) < ntohl(b.ip)) |
||||
return true; |
||||
else if (a.ip == b.ip) |
||||
return ntohs(a.port) < ntohs(b.port); |
||||
} |
||||
return false; |
||||
} |
||||
|
||||
std::vector<unsigned char> CAddress::GetKey() const |
||||
{ |
||||
CDataStream ss; |
||||
ss.reserve(18); |
||||
ss << FLATDATA(pchReserved) << ip << port; |
||||
|
||||
#if defined(_MSC_VER) && _MSC_VER < 1300 |
||||
return std::vector<unsigned char>((unsigned char*)&ss.begin()[0], (unsigned char*)&ss.end()[0]); |
||||
#else |
||||
return std::vector<unsigned char>(ss.begin(), ss.end()); |
||||
#endif |
||||
} |
||||
|
||||
struct sockaddr_in CAddress::GetSockAddr() const |
||||
{ |
||||
struct sockaddr_in sockaddr; |
||||
memset(&sockaddr, 0, sizeof(sockaddr)); |
||||
sockaddr.sin_family = AF_INET; |
||||
sockaddr.sin_addr.s_addr = ip; |
||||
sockaddr.sin_port = port; |
||||
return sockaddr; |
||||
} |
||||
|
||||
bool CAddress::IsIPv4() const |
||||
{ |
||||
return (memcmp(pchReserved, pchIPv4, sizeof(pchIPv4)) == 0); |
||||
} |
||||
|
||||
bool CAddress::IsRFC1918() const |
||||
{ |
||||
return IsIPv4() && (GetByte(3) == 10 || |
||||
(GetByte(3) == 192 && GetByte(2) == 168) || |
||||
(GetByte(3) == 172 && |
||||
(GetByte(2) >= 16 && GetByte(2) <= 31))); |
||||
} |
||||
|
||||
bool CAddress::IsRFC3927() const |
||||
{ |
||||
return IsIPv4() && (GetByte(3) == 169 && GetByte(2) == 254); |
||||
} |
||||
|
||||
bool CAddress::IsLocal() const |
||||
{ |
||||
return IsIPv4() && (GetByte(3) == 127 || |
||||
GetByte(3) == 0); |
||||
} |
||||
|
||||
bool CAddress::IsRoutable() const |
||||
{ |
||||
return IsValid() && |
||||
!(IsRFC1918() || IsRFC3927() || IsLocal()); |
||||
} |
||||
|
||||
bool CAddress::IsValid() const |
||||
{ |
||||
// Clean up 3-byte shifted addresses caused by garbage in size field
|
||||
// of addr messages from versions before 0.2.9 checksum.
|
||||
// Two consecutive addr messages look like this:
|
||||
// header20 vectorlen3 addr26 addr26 addr26 header20 vectorlen3 addr26 addr26 addr26...
|
||||
// so if the first length field is garbled, it reads the second batch
|
||||
// of addr misaligned by 3 bytes.
|
||||
if (memcmp(pchReserved, pchIPv4+3, sizeof(pchIPv4)-3) == 0) |
||||
return false; |
||||
|
||||
return (ip != 0 && ip != INADDR_NONE && port != htons(USHRT_MAX)); |
||||
} |
||||
|
||||
unsigned char CAddress::GetByte(int n) const |
||||
{ |
||||
return ((unsigned char*)&ip)[3-n]; |
||||
} |
||||
|
||||
std::string CAddress::ToStringIPPort() const |
||||
{ |
||||
return strprintf("%u.%u.%u.%u:%u", GetByte(3), GetByte(2), GetByte(1), GetByte(0), ntohs(port)); |
||||
} |
||||
|
||||
std::string CAddress::ToStringIP() const |
||||
{ |
||||
return strprintf("%u.%u.%u.%u", GetByte(3), GetByte(2), GetByte(1), GetByte(0)); |
||||
} |
||||
|
||||
std::string CAddress::ToStringPort() const |
||||
{ |
||||
return strprintf("%u", ntohs(port)); |
||||
} |
||||
|
||||
std::string CAddress::ToString() const |
||||
{ |
||||
return strprintf("%u.%u.%u.%u:%u", GetByte(3), GetByte(2), GetByte(1), GetByte(0), ntohs(port)); |
||||
} |
||||
|
||||
void CAddress::print() const |
||||
{ |
||||
printf("CAddress(%s)\n", ToString().c_str()); |
||||
} |
||||
|
||||
CInv::CInv() |
||||
{ |
||||
type = 0; |
||||
hash = 0; |
||||
} |
||||
|
||||
CInv::CInv(int typeIn, const uint256& hashIn) |
||||
{ |
||||
type = typeIn; |
||||
hash = hashIn; |
||||
} |
||||
|
||||
CInv::CInv(const std::string& strType, const uint256& hashIn) |
||||
{ |
||||
int i; |
||||
for (i = 1; i < ARRAYLEN(ppszTypeName); i++) |
||||
{ |
||||
if (strType == ppszTypeName[i]) |
||||
{ |
||||
type = i; |
||||
break; |
||||
} |
||||
} |
||||
if (i == ARRAYLEN(ppszTypeName)) |
||||
throw std::out_of_range(strprintf("CInv::CInv(string, uint256) : unknown type '%s'", strType.c_str())); |
||||
hash = hashIn; |
||||
} |
||||
|
||||
bool operator<(const CInv& a, const CInv& b) |
||||
{ |
||||
return (a.type < b.type || (a.type == b.type && a.hash < b.hash)); |
||||
} |
||||
|
||||
bool CInv::IsKnownType() const |
||||
{ |
||||
return (type >= 1 && type < ARRAYLEN(ppszTypeName)); |
||||
} |
||||
|
||||
const char* CInv::GetCommand() const |
||||
{ |
||||
if (!IsKnownType()) |
||||
throw std::out_of_range(strprintf("CInv::GetCommand() : type=%d unknown type", type)); |
||||
return ppszTypeName[type]; |
||||
} |
||||
|
||||
std::string CInv::ToString() const |
||||
{ |
||||
return strprintf("%s %s", GetCommand(), hash.ToString().substr(0,20).c_str()); |
||||
} |
||||
|
||||
void CInv::print() const |
||||
{ |
||||
printf("CInv(%s)\n", ToString().c_str()); |
||||
} |
@ -0,0 +1,150 @@
@@ -0,0 +1,150 @@
|
||||
// Copyright (c) 2009-2010 Satoshi Nakamoto
|
||||
// Copyright (c) 2011 The Bitcoin developers
|
||||
// Distributed under the MIT/X11 software license, see the accompanying
|
||||
// file license.txt or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#ifndef __cplusplus |
||||
# error This header can only be compiled as C++. |
||||
#endif |
||||
|
||||
#ifndef __INCLUDED_PROTOCOL_H__ |
||||
#define __INCLUDED_PROTOCOL_H__ |
||||
|
||||
#include "serialize.h" |
||||
#include <string> |
||||
#include "uint256.h" |
||||
|
||||
extern bool fTestNet; |
||||
static inline unsigned short GetDefaultPort(const bool testnet = fTestNet) |
||||
{ |
||||
return testnet ? 18333 : 8333; |
||||
} |
||||
|
||||
//
|
||||
// Message header
|
||||
// (4) message start
|
||||
// (12) command
|
||||
// (4) size
|
||||
// (4) checksum
|
||||
|
||||
extern unsigned char pchMessageStart[4]; |
||||
|
||||
class CMessageHeader |
||||
{ |
||||
public: |
||||
CMessageHeader(); |
||||
CMessageHeader(const char* pszCommand, unsigned int nMessageSizeIn); |
||||
|
||||
std::string GetCommand() const; |
||||
bool IsValid() const; |
||||
|
||||
IMPLEMENT_SERIALIZE |
||||
( |
||||
READWRITE(FLATDATA(pchMessageStart)); |
||||
READWRITE(FLATDATA(pchCommand)); |
||||
READWRITE(nMessageSize); |
||||
if (nVersion >= 209) |
||||
READWRITE(nChecksum); |
||||
) |
||||
|
||||
// TODO: make private (improves encapsulation)
|
||||
public: |
||||
enum { COMMAND_SIZE=12 }; |
||||
char pchMessageStart[sizeof(::pchMessageStart)]; |
||||
char pchCommand[COMMAND_SIZE]; |
||||
unsigned int nMessageSize; |
||||
unsigned int nChecksum; |
||||
}; |
||||
|
||||
enum |
||||
{ |
||||
NODE_NETWORK = (1 << 0), |
||||
}; |
||||
|
||||
class CAddress |
||||
{ |
||||
public: |
||||
CAddress(); |
||||
CAddress(unsigned int ipIn, unsigned short portIn=0, uint64 nServicesIn=NODE_NETWORK); |
||||
explicit CAddress(const struct sockaddr_in& sockaddr, uint64 nServicesIn=NODE_NETWORK); |
||||
explicit CAddress(const char* pszIn, int portIn, bool fNameLookup = false, uint64 nServicesIn=NODE_NETWORK); |
||||
explicit CAddress(const char* pszIn, bool fNameLookup = false, uint64 nServicesIn=NODE_NETWORK); |
||||
explicit CAddress(std::string strIn, int portIn, bool fNameLookup = false, uint64 nServicesIn=NODE_NETWORK); |
||||
explicit CAddress(std::string strIn, bool fNameLookup = false, uint64 nServicesIn=NODE_NETWORK); |
||||
|
||||
void Init(); |
||||
|
||||
IMPLEMENT_SERIALIZE |
||||
( |
||||
if (fRead) |
||||
const_cast<CAddress*>(this)->Init(); |
||||
if (nType & SER_DISK) |
||||
READWRITE(nVersion); |
||||
if ((nType & SER_DISK) || (nVersion >= 31402 && !(nType & SER_GETHASH))) |
||||
READWRITE(nTime); |
||||
READWRITE(nServices); |
||||
READWRITE(FLATDATA(pchReserved)); // for IPv6
|
||||
READWRITE(ip); |
||||
READWRITE(port); |
||||
) |
||||
|
||||
friend bool operator==(const CAddress& a, const CAddress& b); |
||||
friend bool operator!=(const CAddress& a, const CAddress& b); |
||||
friend bool operator<(const CAddress& a, const CAddress& b); |
||||
|
||||
std::vector<unsigned char> GetKey() const; |
||||
struct sockaddr_in GetSockAddr() const; |
||||
bool IsIPv4() const; |
||||
bool IsRFC1918() const; |
||||
bool IsRFC3927() const; |
||||
bool IsLocal() const; |
||||
bool IsRoutable() const; |
||||
bool IsValid() const; |
||||
unsigned char GetByte(int n) const; |
||||
std::string ToStringIPPort() const; |
||||
std::string ToStringIP() const; |
||||
std::string ToStringPort() const; |
||||
std::string ToString() const; |
||||
void print() const; |
||||
|
||||
// TODO: make private (improves encapsulation)
|
||||
public: |
||||
uint64 nServices; |
||||
unsigned char pchReserved[12]; |
||||
unsigned int ip; |
||||
unsigned short port; |
||||
|
||||
// disk and network only
|
||||
unsigned int nTime; |
||||
|
||||
// memory only
|
||||
unsigned int nLastTry; |
||||
}; |
||||
|
||||
class CInv |
||||
{ |
||||
public: |
||||
CInv(); |
||||
CInv(int typeIn, const uint256& hashIn); |
||||
CInv(const std::string& strType, const uint256& hashIn); |
||||
|
||||
IMPLEMENT_SERIALIZE |
||||
( |
||||
READWRITE(type); |
||||
READWRITE(hash); |
||||
) |
||||
|
||||
friend bool operator<(const CInv& a, const CInv& b); |
||||
|
||||
bool IsKnownType() const; |
||||
const char* GetCommand() const; |
||||
std::string ToString() const; |
||||
void print() const; |
||||
|
||||
// TODO: make private (improves encapsulation)
|
||||
public: |
||||
int type; |
||||
uint256 hash; |
||||
}; |
||||
|
||||
#endif // __INCLUDED_PROTOCOL_H__
|
Loading…
Reference in new issue