Browse Source
50cc6ab Merge pull request #178 941e221 Add tests for handling of the nonce function in signing. 10c81ff Merge pull request #177 7688e34 Add magnitude limits to secp256k1_fe_verify to ensure that it's own tests function correctly. 4ee4f7a Merge pull request #176 70ae0d2 Use secp256k1_fe_equal_var in secp256k1_fe_sqrt_var. 7767b4d Merge pull request #175 9ab9335 Add a reference consistency test to ge_tests. 60571c6 Rework group tests d26e26f Avoid constructing an invalid signature with probability 1:2^256. b450c34 Merge pull request #163 d57cae9 Merge pull request #154 49ee0db Add _normalizes_to_zero_var variant eed599d Add _fe_normalizes_to_zero method d7174ed Weak normalization for secp256k1_fe_equal 0295f0a weak normalization bbd5ba7 Use rfc6979 as default nonce generation function b37fbc2 Implement SHA256 / HMAC-SHA256 / RFC6979. c6e7f4e [API BREAK] Use a nonce-generation function instead of a nonce cf0c48b Merge pull request #169 603c33b Make signing fail if a too small buffer is passed. 6d16606 Merge pull request #168 7277fd7 Remove GMP field implementation e99c4c4 Merge pull request #123 13278f6 Add explanation about how inversion can be avoided ce7eb6f Optimize verification: avoid field inverse a098f78 Merge pull request #160 38acd01 Merge pull request #165 6a59012 Make git ignore bench_recover when configured with benchmark enabled 1ba4a60 Configure options reorganization 3c0f246 Merge pull request #157 808dd9b Merge pull request #156 8dc75e9 Merge pull request #158 28ade27 build: nuke bashisms 5190079 build: use subdir-objects for automake 8336040 build: disable benchmark by default git-subtree-dir: src/secp256k1 git-subtree-split: 50cc6ab0625efda6dddf1dc86c1e2671f069b0d80.14
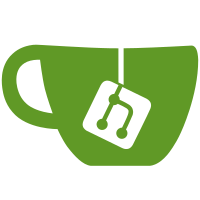
23 changed files with 1172 additions and 529 deletions
@ -1,18 +0,0 @@
@@ -1,18 +0,0 @@
|
||||
/**********************************************************************
|
||||
* Copyright (c) 2013, 2014 Pieter Wuille * |
||||
* Distributed under the MIT software license, see the accompanying * |
||||
* file COPYING or http://www.opensource.org/licenses/mit-license.php.*
|
||||
**********************************************************************/ |
||||
|
||||
#ifndef _SECP256K1_FIELD_REPR_ |
||||
#define _SECP256K1_FIELD_REPR_ |
||||
|
||||
#include <gmp.h> |
||||
|
||||
#define FIELD_LIMBS ((256 + GMP_NUMB_BITS - 1) / GMP_NUMB_BITS) |
||||
|
||||
typedef struct { |
||||
mp_limb_t n[FIELD_LIMBS+1]; |
||||
} secp256k1_fe_t; |
||||
|
||||
#endif |
@ -1,184 +0,0 @@
@@ -1,184 +0,0 @@
|
||||
/**********************************************************************
|
||||
* Copyright (c) 2013, 2014 Pieter Wuille * |
||||
* Distributed under the MIT software license, see the accompanying * |
||||
* file COPYING or http://www.opensource.org/licenses/mit-license.php.*
|
||||
**********************************************************************/ |
||||
|
||||
#ifndef _SECP256K1_FIELD_REPR_IMPL_H_ |
||||
#define _SECP256K1_FIELD_REPR_IMPL_H_ |
||||
|
||||
#include <stdio.h> |
||||
#include <string.h> |
||||
#include "num.h" |
||||
#include "field.h" |
||||
|
||||
static mp_limb_t secp256k1_field_p[FIELD_LIMBS]; |
||||
static mp_limb_t secp256k1_field_pc[(33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS]; |
||||
|
||||
static void secp256k1_fe_inner_start(void) { |
||||
for (int i=0; i<(33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS; i++) |
||||
secp256k1_field_pc[i] = 0; |
||||
secp256k1_field_pc[0] += 0x3D1UL; |
||||
secp256k1_field_pc[32/GMP_NUMB_BITS] += (((mp_limb_t)1) << (32 % GMP_NUMB_BITS)); |
||||
for (int i=0; i<FIELD_LIMBS; i++) { |
||||
secp256k1_field_p[i] = 0; |
||||
} |
||||
mpn_sub(secp256k1_field_p, secp256k1_field_p, FIELD_LIMBS, secp256k1_field_pc, (33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS); |
||||
} |
||||
|
||||
static void secp256k1_fe_inner_stop(void) { |
||||
} |
||||
|
||||
static void secp256k1_fe_normalize(secp256k1_fe_t *r) { |
||||
if (r->n[FIELD_LIMBS] != 0) { |
||||
#if (GMP_NUMB_BITS >= 40) |
||||
mp_limb_t carry = mpn_add_1(r->n, r->n, FIELD_LIMBS, 0x1000003D1ULL * r->n[FIELD_LIMBS]); |
||||
mpn_add_1(r->n, r->n, FIELD_LIMBS, 0x1000003D1ULL * carry); |
||||
#else |
||||
mp_limb_t carry = mpn_add_1(r->n, r->n, FIELD_LIMBS, 0x3D1UL * r->n[FIELD_LIMBS]) + |
||||
mpn_add_1(r->n+(32/GMP_NUMB_BITS), r->n+(32/GMP_NUMB_BITS), FIELD_LIMBS-(32/GMP_NUMB_BITS), r->n[FIELD_LIMBS] << (32 % GMP_NUMB_BITS)); |
||||
mpn_add_1(r->n, r->n, FIELD_LIMBS, 0x3D1UL * carry); |
||||
mpn_add_1(r->n+(32/GMP_NUMB_BITS), r->n+(32/GMP_NUMB_BITS), FIELD_LIMBS-(32/GMP_NUMB_BITS), carry << (32%GMP_NUMB_BITS)); |
||||
#endif |
||||
r->n[FIELD_LIMBS] = 0; |
||||
} |
||||
if (mpn_cmp(r->n, secp256k1_field_p, FIELD_LIMBS) >= 0) |
||||
mpn_sub(r->n, r->n, FIELD_LIMBS, secp256k1_field_p, FIELD_LIMBS); |
||||
} |
||||
|
||||
static void secp256k1_fe_normalize_var(secp256k1_fe_t *r) { |
||||
secp256k1_fe_normalize(r); |
||||
} |
||||
|
||||
SECP256K1_INLINE static void secp256k1_fe_set_int(secp256k1_fe_t *r, int a) { |
||||
r->n[0] = a; |
||||
for (int i=1; i<FIELD_LIMBS+1; i++) |
||||
r->n[i] = 0; |
||||
} |
||||
|
||||
SECP256K1_INLINE static void secp256k1_fe_clear(secp256k1_fe_t *r) { |
||||
for (int i=0; i<FIELD_LIMBS+1; i++) |
||||
r->n[i] = 0; |
||||
} |
||||
|
||||
SECP256K1_INLINE static int secp256k1_fe_is_zero(const secp256k1_fe_t *a) { |
||||
int ret = 1; |
||||
for (int i=0; i<FIELD_LIMBS+1; i++) |
||||
ret &= (a->n[i] == 0); |
||||
return ret; |
||||
} |
||||
|
||||
SECP256K1_INLINE static int secp256k1_fe_is_odd(const secp256k1_fe_t *a) { |
||||
return a->n[0] & 1; |
||||
} |
||||
|
||||
SECP256K1_INLINE static int secp256k1_fe_equal(const secp256k1_fe_t *a, const secp256k1_fe_t *b) { |
||||
int ret = 1; |
||||
for (int i=0; i<FIELD_LIMBS+1; i++) |
||||
ret &= (a->n[i] == b->n[i]); |
||||
return ret; |
||||
} |
||||
|
||||
SECP256K1_INLINE static int secp256k1_fe_cmp_var(const secp256k1_fe_t *a, const secp256k1_fe_t *b) { |
||||
for (int i=FIELD_LIMBS; i>=0; i--) { |
||||
if (a->n[i] > b->n[i]) return 1; |
||||
if (a->n[i] < b->n[i]) return -1; |
||||
} |
||||
return 0; |
||||
} |
||||
|
||||
static int secp256k1_fe_set_b32(secp256k1_fe_t *r, const unsigned char *a) { |
||||
for (int i=0; i<FIELD_LIMBS+1; i++) |
||||
r->n[i] = 0; |
||||
for (int i=0; i<256; i++) { |
||||
int limb = i/GMP_NUMB_BITS; |
||||
int shift = i%GMP_NUMB_BITS; |
||||
r->n[limb] |= (mp_limb_t)((a[31-i/8] >> (i%8)) & 0x1) << shift; |
||||
} |
||||
return (mpn_cmp(r->n, secp256k1_field_p, FIELD_LIMBS) < 0); |
||||
} |
||||
|
||||
/** Convert a field element to a 32-byte big endian value. Requires the input to be normalized */ |
||||
static void secp256k1_fe_get_b32(unsigned char *r, const secp256k1_fe_t *a) { |
||||
for (int i=0; i<32; i++) { |
||||
int c = 0; |
||||
for (int j=0; j<8; j++) { |
||||
int limb = (8*i+j)/GMP_NUMB_BITS; |
||||
int shift = (8*i+j)%GMP_NUMB_BITS; |
||||
c |= ((a->n[limb] >> shift) & 0x1) << j; |
||||
} |
||||
r[31-i] = c; |
||||
} |
||||
} |
||||
|
||||
SECP256K1_INLINE static void secp256k1_fe_negate(secp256k1_fe_t *r, const secp256k1_fe_t *a, int m) { |
||||
(void)m; |
||||
*r = *a; |
||||
secp256k1_fe_normalize(r); |
||||
for (int i=0; i<FIELD_LIMBS; i++) |
||||
r->n[i] = ~(r->n[i]); |
||||
#if (GMP_NUMB_BITS >= 33) |
||||
mpn_sub_1(r->n, r->n, FIELD_LIMBS, 0x1000003D0ULL); |
||||
#else |
||||
mpn_sub_1(r->n, r->n, FIELD_LIMBS, 0x3D0UL); |
||||
mpn_sub_1(r->n+(32/GMP_NUMB_BITS), r->n+(32/GMP_NUMB_BITS), FIELD_LIMBS-(32/GMP_NUMB_BITS), 0x1UL << (32%GMP_NUMB_BITS)); |
||||
#endif |
||||
} |
||||
|
||||
SECP256K1_INLINE static void secp256k1_fe_mul_int(secp256k1_fe_t *r, int a) { |
||||
mpn_mul_1(r->n, r->n, FIELD_LIMBS+1, a); |
||||
} |
||||
|
||||
SECP256K1_INLINE static void secp256k1_fe_add(secp256k1_fe_t *r, const secp256k1_fe_t *a) { |
||||
mpn_add(r->n, r->n, FIELD_LIMBS+1, a->n, FIELD_LIMBS+1); |
||||
} |
||||
|
||||
static void secp256k1_fe_reduce(secp256k1_fe_t *r, mp_limb_t *tmp) { |
||||
/** <A1 A2 A3 A4> <B1 B2 B3 B4>
|
||||
* B1 B2 B3 B4 |
||||
* + C * A1 A2 A3 A4 |
||||
* + A1 A2 A3 A4 |
||||
*/ |
||||
|
||||
#if (GMP_NUMB_BITS >= 33) |
||||
mp_limb_t o = mpn_addmul_1(tmp, tmp+FIELD_LIMBS, FIELD_LIMBS, 0x1000003D1ULL); |
||||
#else |
||||
mp_limb_t o = mpn_addmul_1(tmp, tmp+FIELD_LIMBS, FIELD_LIMBS, 0x3D1UL) + |
||||
mpn_addmul_1(tmp+(32/GMP_NUMB_BITS), tmp+FIELD_LIMBS, FIELD_LIMBS-(32/GMP_NUMB_BITS), 0x1UL << (32%GMP_NUMB_BITS)); |
||||
#endif |
||||
mp_limb_t q[1+(33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS]; |
||||
q[(33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS] = mpn_mul_1(q, secp256k1_field_pc, (33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS, o); |
||||
#if (GMP_NUMB_BITS <= 32) |
||||
mp_limb_t o2 = tmp[2*FIELD_LIMBS-(32/GMP_NUMB_BITS)] << (32%GMP_NUMB_BITS); |
||||
q[(33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS] += mpn_addmul_1(q, secp256k1_field_pc, (33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS, o2); |
||||
#endif |
||||
r->n[FIELD_LIMBS] = mpn_add(r->n, tmp, FIELD_LIMBS, q, 1+(33+GMP_NUMB_BITS-1)/GMP_NUMB_BITS); |
||||
} |
||||
|
||||
static void secp256k1_fe_mul(secp256k1_fe_t *r, const secp256k1_fe_t *a, const secp256k1_fe_t * SECP256K1_RESTRICT b) { |
||||
VERIFY_CHECK(r != b); |
||||
secp256k1_fe_t ac = *a; |
||||
secp256k1_fe_t bc = *b; |
||||
secp256k1_fe_normalize(&ac); |
||||
secp256k1_fe_normalize(&bc); |
||||
mp_limb_t tmp[2*FIELD_LIMBS]; |
||||
mpn_mul_n(tmp, ac.n, bc.n, FIELD_LIMBS); |
||||
secp256k1_fe_reduce(r, tmp); |
||||
} |
||||
|
||||
static void secp256k1_fe_sqr(secp256k1_fe_t *r, const secp256k1_fe_t *a) { |
||||
secp256k1_fe_t ac = *a; |
||||
secp256k1_fe_normalize(&ac); |
||||
mp_limb_t tmp[2*FIELD_LIMBS]; |
||||
mpn_sqr(tmp, ac.n, FIELD_LIMBS); |
||||
secp256k1_fe_reduce(r, tmp); |
||||
} |
||||
|
||||
static void secp256k1_fe_cmov(secp256k1_fe_t *r, const secp256k1_fe_t *a, int flag) { |
||||
mp_limb_t mask0 = flag + ~((mp_limb_t)0), mask1 = ~mask0; |
||||
for (int i = 0; i <= FIELD_LIMBS; i++) { |
||||
r->n[i] = (r->n[i] & mask0) | (a->n[i] & mask1); |
||||
} |
||||
} |
||||
|
||||
#endif |
@ -0,0 +1,41 @@
@@ -0,0 +1,41 @@
|
||||
/**********************************************************************
|
||||
* Copyright (c) 2014 Pieter Wuille * |
||||
* Distributed under the MIT software license, see the accompanying * |
||||
* file COPYING or http://www.opensource.org/licenses/mit-license.php.*
|
||||
**********************************************************************/ |
||||
|
||||
#ifndef _SECP256K1_HASH_ |
||||
#define _SECP256K1_HASH_ |
||||
|
||||
#include <stdlib.h> |
||||
#include <stdint.h> |
||||
|
||||
typedef struct { |
||||
uint32_t s[32]; |
||||
unsigned char buf[64]; |
||||
size_t bytes; |
||||
} secp256k1_sha256_t; |
||||
|
||||
static void secp256k1_sha256_initialize(secp256k1_sha256_t *hash); |
||||
static void secp256k1_sha256_write(secp256k1_sha256_t *hash, const unsigned char *data, size_t size); |
||||
static void secp256k1_sha256_finalize(secp256k1_sha256_t *hash, unsigned char *out32); |
||||
|
||||
typedef struct { |
||||
secp256k1_sha256_t inner, outer; |
||||
} secp256k1_hmac_sha256_t; |
||||
|
||||
static void secp256k1_hmac_sha256_initialize(secp256k1_hmac_sha256_t *hash, const unsigned char *key, size_t size); |
||||
static void secp256k1_hmac_sha256_write(secp256k1_hmac_sha256_t *hash, const unsigned char *data, size_t size); |
||||
static void secp256k1_hmac_sha256_finalize(secp256k1_hmac_sha256_t *hash, unsigned char *out32); |
||||
|
||||
typedef struct { |
||||
unsigned char v[32]; |
||||
unsigned char k[32]; |
||||
int retry; |
||||
} secp256k1_rfc6979_hmac_sha256_t; |
||||
|
||||
static void secp256k1_rfc6979_hmac_sha256_initialize(secp256k1_rfc6979_hmac_sha256_t *rng, const unsigned char *key, size_t keylen, const unsigned char *msg, size_t msglen); |
||||
static void secp256k1_rfc6979_hmac_sha256_generate(secp256k1_rfc6979_hmac_sha256_t *rng, unsigned char *out, size_t outlen); |
||||
static void secp256k1_rfc6979_hmac_sha256_finalize(secp256k1_rfc6979_hmac_sha256_t *rng); |
||||
|
||||
#endif |
@ -0,0 +1,291 @@
@@ -0,0 +1,291 @@
|
||||
/**********************************************************************
|
||||
* Copyright (c) 2014 Pieter Wuille * |
||||
* Distributed under the MIT software license, see the accompanying * |
||||
* file COPYING or http://www.opensource.org/licenses/mit-license.php.*
|
||||
**********************************************************************/ |
||||
|
||||
#ifndef _SECP256K1_HASH_IMPL_H_ |
||||
#define _SECP256K1_HASH_IMPL_H_ |
||||
|
||||
#include "hash.h" |
||||
|
||||
#include <stdlib.h> |
||||
#include <stdint.h> |
||||
|
||||
#define Ch(x,y,z) ((z) ^ ((x) & ((y) ^ (z)))) |
||||
#define Maj(x,y,z) (((x) & (y)) | ((z) & ((x) | (y)))) |
||||
#define Sigma0(x) (((x) >> 2 | (x) << 30) ^ ((x) >> 13 | (x) << 19) ^ ((x) >> 22 | (x) << 10)) |
||||
#define Sigma1(x) (((x) >> 6 | (x) << 26) ^ ((x) >> 11 | (x) << 21) ^ ((x) >> 25 | (x) << 7)) |
||||
#define sigma0(x) (((x) >> 7 | (x) << 25) ^ ((x) >> 18 | (x) << 14) ^ ((x) >> 3)) |
||||
#define sigma1(x) (((x) >> 17 | (x) << 15) ^ ((x) >> 19 | (x) << 13) ^ ((x) >> 10)) |
||||
|
||||
#define Round(a,b,c,d,e,f,g,h,k,w) do { \ |
||||
uint32_t t1 = (h) + Sigma1(e) + Ch((e), (f), (g)) + (k) + (w); \ |
||||
uint32_t t2 = Sigma0(a) + Maj((a), (b), (c)); \ |
||||
(d) += t1; \ |
||||
(h) = t1 + t2; \ |
||||
} while(0) |
||||
|
||||
#define ReadBE32(p) (((uint32_t)((p)[0])) << 24 | ((uint32_t)((p)[1])) << 16 | ((uint32_t)((p)[2])) << 8 | ((uint32_t)((p)[3]))) |
||||
#define WriteBE32(p, v) do { (p)[0] = (v) >> 24; (p)[1] = (v) >> 16; (p)[2] = (v) >> 8; (p)[3] = (v); } while(0) |
||||
|
||||
static void secp256k1_sha256_initialize(secp256k1_sha256_t *hash) { |
||||
hash->s[0] = 0x6a09e667ul; |
||||
hash->s[1] = 0xbb67ae85ul; |
||||
hash->s[2] = 0x3c6ef372ul; |
||||
hash->s[3] = 0xa54ff53aul; |
||||
hash->s[4] = 0x510e527ful; |
||||
hash->s[5] = 0x9b05688cul; |
||||
hash->s[6] = 0x1f83d9abul; |
||||
hash->s[7] = 0x5be0cd19ul; |
||||
hash->bytes = 0; |
||||
} |
||||
|
||||
/** Perform one SHA-256 transformation, processing a 64-byte chunk. */ |
||||
static void secp256k1_sha256_transform(uint32_t* s, const unsigned char* chunk) { |
||||
uint32_t a = s[0], b = s[1], c = s[2], d = s[3], e = s[4], f = s[5], g = s[6], h = s[7]; |
||||
uint32_t w0, w1, w2, w3, w4, w5, w6, w7, w8, w9, w10, w11, w12, w13, w14, w15; |
||||
|
||||
Round(a, b, c, d, e, f, g, h, 0x428a2f98, w0 = ReadBE32(chunk + 0)); |
||||
Round(h, a, b, c, d, e, f, g, 0x71374491, w1 = ReadBE32(chunk + 4)); |
||||
Round(g, h, a, b, c, d, e, f, 0xb5c0fbcf, w2 = ReadBE32(chunk + 8)); |
||||
Round(f, g, h, a, b, c, d, e, 0xe9b5dba5, w3 = ReadBE32(chunk + 12)); |
||||
Round(e, f, g, h, a, b, c, d, 0x3956c25b, w4 = ReadBE32(chunk + 16)); |
||||
Round(d, e, f, g, h, a, b, c, 0x59f111f1, w5 = ReadBE32(chunk + 20)); |
||||
Round(c, d, e, f, g, h, a, b, 0x923f82a4, w6 = ReadBE32(chunk + 24)); |
||||
Round(b, c, d, e, f, g, h, a, 0xab1c5ed5, w7 = ReadBE32(chunk + 28)); |
||||
Round(a, b, c, d, e, f, g, h, 0xd807aa98, w8 = ReadBE32(chunk + 32)); |
||||
Round(h, a, b, c, d, e, f, g, 0x12835b01, w9 = ReadBE32(chunk + 36)); |
||||
Round(g, h, a, b, c, d, e, f, 0x243185be, w10 = ReadBE32(chunk + 40)); |
||||
Round(f, g, h, a, b, c, d, e, 0x550c7dc3, w11 = ReadBE32(chunk + 44)); |
||||
Round(e, f, g, h, a, b, c, d, 0x72be5d74, w12 = ReadBE32(chunk + 48)); |
||||
Round(d, e, f, g, h, a, b, c, 0x80deb1fe, w13 = ReadBE32(chunk + 52)); |
||||
Round(c, d, e, f, g, h, a, b, 0x9bdc06a7, w14 = ReadBE32(chunk + 56)); |
||||
Round(b, c, d, e, f, g, h, a, 0xc19bf174, w15 = ReadBE32(chunk + 60)); |
||||
|
||||
Round(a, b, c, d, e, f, g, h, 0xe49b69c1, w0 += sigma1(w14) + w9 + sigma0(w1)); |
||||
Round(h, a, b, c, d, e, f, g, 0xefbe4786, w1 += sigma1(w15) + w10 + sigma0(w2)); |
||||
Round(g, h, a, b, c, d, e, f, 0x0fc19dc6, w2 += sigma1(w0) + w11 + sigma0(w3)); |
||||
Round(f, g, h, a, b, c, d, e, 0x240ca1cc, w3 += sigma1(w1) + w12 + sigma0(w4)); |
||||
Round(e, f, g, h, a, b, c, d, 0x2de92c6f, w4 += sigma1(w2) + w13 + sigma0(w5)); |
||||
Round(d, e, f, g, h, a, b, c, 0x4a7484aa, w5 += sigma1(w3) + w14 + sigma0(w6)); |
||||
Round(c, d, e, f, g, h, a, b, 0x5cb0a9dc, w6 += sigma1(w4) + w15 + sigma0(w7)); |
||||
Round(b, c, d, e, f, g, h, a, 0x76f988da, w7 += sigma1(w5) + w0 + sigma0(w8)); |
||||
Round(a, b, c, d, e, f, g, h, 0x983e5152, w8 += sigma1(w6) + w1 + sigma0(w9)); |
||||
Round(h, a, b, c, d, e, f, g, 0xa831c66d, w9 += sigma1(w7) + w2 + sigma0(w10)); |
||||
Round(g, h, a, b, c, d, e, f, 0xb00327c8, w10 += sigma1(w8) + w3 + sigma0(w11)); |
||||
Round(f, g, h, a, b, c, d, e, 0xbf597fc7, w11 += sigma1(w9) + w4 + sigma0(w12)); |
||||
Round(e, f, g, h, a, b, c, d, 0xc6e00bf3, w12 += sigma1(w10) + w5 + sigma0(w13)); |
||||
Round(d, e, f, g, h, a, b, c, 0xd5a79147, w13 += sigma1(w11) + w6 + sigma0(w14)); |
||||
Round(c, d, e, f, g, h, a, b, 0x06ca6351, w14 += sigma1(w12) + w7 + sigma0(w15)); |
||||
Round(b, c, d, e, f, g, h, a, 0x14292967, w15 += sigma1(w13) + w8 + sigma0(w0)); |
||||
|
||||
Round(a, b, c, d, e, f, g, h, 0x27b70a85, w0 += sigma1(w14) + w9 + sigma0(w1)); |
||||
Round(h, a, b, c, d, e, f, g, 0x2e1b2138, w1 += sigma1(w15) + w10 + sigma0(w2)); |
||||
Round(g, h, a, b, c, d, e, f, 0x4d2c6dfc, w2 += sigma1(w0) + w11 + sigma0(w3)); |
||||
Round(f, g, h, a, b, c, d, e, 0x53380d13, w3 += sigma1(w1) + w12 + sigma0(w4)); |
||||
Round(e, f, g, h, a, b, c, d, 0x650a7354, w4 += sigma1(w2) + w13 + sigma0(w5)); |
||||
Round(d, e, f, g, h, a, b, c, 0x766a0abb, w5 += sigma1(w3) + w14 + sigma0(w6)); |
||||
Round(c, d, e, f, g, h, a, b, 0x81c2c92e, w6 += sigma1(w4) + w15 + sigma0(w7)); |
||||
Round(b, c, d, e, f, g, h, a, 0x92722c85, w7 += sigma1(w5) + w0 + sigma0(w8)); |
||||
Round(a, b, c, d, e, f, g, h, 0xa2bfe8a1, w8 += sigma1(w6) + w1 + sigma0(w9)); |
||||
Round(h, a, b, c, d, e, f, g, 0xa81a664b, w9 += sigma1(w7) + w2 + sigma0(w10)); |
||||
Round(g, h, a, b, c, d, e, f, 0xc24b8b70, w10 += sigma1(w8) + w3 + sigma0(w11)); |
||||
Round(f, g, h, a, b, c, d, e, 0xc76c51a3, w11 += sigma1(w9) + w4 + sigma0(w12)); |
||||
Round(e, f, g, h, a, b, c, d, 0xd192e819, w12 += sigma1(w10) + w5 + sigma0(w13)); |
||||
Round(d, e, f, g, h, a, b, c, 0xd6990624, w13 += sigma1(w11) + w6 + sigma0(w14)); |
||||
Round(c, d, e, f, g, h, a, b, 0xf40e3585, w14 += sigma1(w12) + w7 + sigma0(w15)); |
||||
Round(b, c, d, e, f, g, h, a, 0x106aa070, w15 += sigma1(w13) + w8 + sigma0(w0)); |
||||
|
||||
Round(a, b, c, d, e, f, g, h, 0x19a4c116, w0 += sigma1(w14) + w9 + sigma0(w1)); |
||||
Round(h, a, b, c, d, e, f, g, 0x1e376c08, w1 += sigma1(w15) + w10 + sigma0(w2)); |
||||
Round(g, h, a, b, c, d, e, f, 0x2748774c, w2 += sigma1(w0) + w11 + sigma0(w3)); |
||||
Round(f, g, h, a, b, c, d, e, 0x34b0bcb5, w3 += sigma1(w1) + w12 + sigma0(w4)); |
||||
Round(e, f, g, h, a, b, c, d, 0x391c0cb3, w4 += sigma1(w2) + w13 + sigma0(w5)); |
||||
Round(d, e, f, g, h, a, b, c, 0x4ed8aa4a, w5 += sigma1(w3) + w14 + sigma0(w6)); |
||||
Round(c, d, e, f, g, h, a, b, 0x5b9cca4f, w6 += sigma1(w4) + w15 + sigma0(w7)); |
||||
Round(b, c, d, e, f, g, h, a, 0x682e6ff3, w7 += sigma1(w5) + w0 + sigma0(w8)); |
||||
Round(a, b, c, d, e, f, g, h, 0x748f82ee, w8 += sigma1(w6) + w1 + sigma0(w9)); |
||||
Round(h, a, b, c, d, e, f, g, 0x78a5636f, w9 += sigma1(w7) + w2 + sigma0(w10)); |
||||
Round(g, h, a, b, c, d, e, f, 0x84c87814, w10 += sigma1(w8) + w3 + sigma0(w11)); |
||||
Round(f, g, h, a, b, c, d, e, 0x8cc70208, w11 += sigma1(w9) + w4 + sigma0(w12)); |
||||
Round(e, f, g, h, a, b, c, d, 0x90befffa, w12 += sigma1(w10) + w5 + sigma0(w13)); |
||||
Round(d, e, f, g, h, a, b, c, 0xa4506ceb, w13 += sigma1(w11) + w6 + sigma0(w14)); |
||||
Round(c, d, e, f, g, h, a, b, 0xbef9a3f7, w14 + sigma1(w12) + w7 + sigma0(w15)); |
||||
Round(b, c, d, e, f, g, h, a, 0xc67178f2, w15 + sigma1(w13) + w8 + sigma0(w0)); |
||||
|
||||
s[0] += a; |
||||
s[1] += b; |
||||
s[2] += c; |
||||
s[3] += d; |
||||
s[4] += e; |
||||
s[5] += f; |
||||
s[6] += g; |
||||
s[7] += h; |
||||
} |
||||
|
||||
static void secp256k1_sha256_write(secp256k1_sha256_t *hash, const unsigned char *data, size_t len) { |
||||
const unsigned char* end = data + len; |
||||
size_t bufsize = hash->bytes % 64; |
||||
if (bufsize && bufsize + len >= 64) { |
||||
// Fill the buffer, and process it.
|
||||
memcpy(hash->buf + bufsize, data, 64 - bufsize); |
||||
hash->bytes += 64 - bufsize; |
||||
data += 64 - bufsize; |
||||
secp256k1_sha256_transform(hash->s, hash->buf); |
||||
bufsize = 0; |
||||
} |
||||
while (end >= data + 64) { |
||||
// Process full chunks directly from the source.
|
||||
secp256k1_sha256_transform(hash->s, data); |
||||
hash->bytes += 64; |
||||
data += 64; |
||||
} |
||||
if (end > data) { |
||||
// Fill the buffer with what remains.
|
||||
memcpy(hash->buf + bufsize, data, end - data); |
||||
hash->bytes += end - data; |
||||
} |
||||
} |
||||
|
||||
static void secp256k1_sha256_finalize(secp256k1_sha256_t *hash, unsigned char *out32) { |
||||
static const unsigned char pad[64] = {0x80, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}; |
||||
unsigned char sizedesc[8]; |
||||
WriteBE32(sizedesc, hash->bytes >> 29); |
||||
WriteBE32(sizedesc + 4, hash->bytes << 3); |
||||
secp256k1_sha256_write(hash, pad, 1 + ((119 - (hash->bytes % 64)) % 64)); |
||||
secp256k1_sha256_write(hash, sizedesc, 8); |
||||
WriteBE32(out32, hash->s[0]); |
||||
hash->s[0] = 0; |
||||
WriteBE32(out32 + 4, hash->s[1]); |
||||
hash->s[1] = 0; |
||||
WriteBE32(out32 + 8, hash->s[2]); |
||||
hash->s[2] = 0; |
||||
WriteBE32(out32 + 12, hash->s[3]); |
||||
hash->s[3] = 0; |
||||
WriteBE32(out32 + 16, hash->s[4]); |
||||
hash->s[4] = 0; |
||||
WriteBE32(out32 + 20, hash->s[5]); |
||||
hash->s[5] = 0; |
||||
WriteBE32(out32 + 24, hash->s[6]); |
||||
hash->s[6] = 0; |
||||
WriteBE32(out32 + 28, hash->s[7]); |
||||
hash->s[7] = 0; |
||||
} |
||||
|
||||
static void secp256k1_hmac_sha256_initialize(secp256k1_hmac_sha256_t *hash, const unsigned char *key, size_t keylen) { |
||||
unsigned char rkey[64]; |
||||
if (keylen <= 64) { |
||||
memcpy(rkey, key, keylen); |
||||
memset(rkey + keylen, 0, 64 - keylen); |
||||
} else { |
||||
secp256k1_sha256_t sha256; |
||||
secp256k1_sha256_initialize(&sha256); |
||||
secp256k1_sha256_write(&sha256, key, keylen); |
||||
secp256k1_sha256_finalize(&sha256, rkey); |
||||
memset(rkey + 32, 0, 32); |
||||
} |
||||
|
||||
secp256k1_sha256_initialize(&hash->outer); |
||||
for (int n = 0; n < 64; n++) |
||||
rkey[n] ^= 0x5c; |
||||
secp256k1_sha256_write(&hash->outer, rkey, 64); |
||||
|
||||
secp256k1_sha256_initialize(&hash->inner); |
||||
for (int n = 0; n < 64; n++) |
||||
rkey[n] ^= 0x5c ^ 0x36; |
||||
secp256k1_sha256_write(&hash->inner, rkey, 64); |
||||
memset(rkey, 0, 64); |
||||
} |
||||
|
||||
static void secp256k1_hmac_sha256_write(secp256k1_hmac_sha256_t *hash, const unsigned char *data, size_t size) { |
||||
secp256k1_sha256_write(&hash->inner, data, size); |
||||
} |
||||
|
||||
static void secp256k1_hmac_sha256_finalize(secp256k1_hmac_sha256_t *hash, unsigned char *out32) { |
||||
unsigned char temp[32]; |
||||
secp256k1_sha256_finalize(&hash->inner, temp); |
||||
secp256k1_sha256_write(&hash->outer, temp, 32); |
||||
memset(temp, 0, 32); |
||||
secp256k1_sha256_finalize(&hash->outer, out32); |
||||
} |
||||
|
||||
|
||||
static void secp256k1_rfc6979_hmac_sha256_initialize(secp256k1_rfc6979_hmac_sha256_t *rng, const unsigned char *key, size_t keylen, const unsigned char *msg, size_t msglen) { |
||||
static const unsigned char zero[1] = {0x00}; |
||||
static const unsigned char one[1] = {0x01}; |
||||
|
||||
memset(rng->v, 0x01, 32); |
||||
memset(rng->k, 0x00, 32); |
||||
|
||||
secp256k1_hmac_sha256_t hmac; |
||||
secp256k1_hmac_sha256_initialize(&hmac, rng->k, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, rng->v, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, zero, 1); |
||||
secp256k1_hmac_sha256_write(&hmac, key, keylen); |
||||
secp256k1_hmac_sha256_write(&hmac, msg, msglen); |
||||
secp256k1_hmac_sha256_finalize(&hmac, rng->k); |
||||
secp256k1_hmac_sha256_initialize(&hmac, rng->k, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, rng->v, 32); |
||||
secp256k1_hmac_sha256_finalize(&hmac, rng->v); |
||||
|
||||
secp256k1_hmac_sha256_initialize(&hmac, rng->k, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, rng->v, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, one, 1); |
||||
secp256k1_hmac_sha256_write(&hmac, key, keylen); |
||||
secp256k1_hmac_sha256_write(&hmac, msg, msglen); |
||||
secp256k1_hmac_sha256_finalize(&hmac, rng->k); |
||||
secp256k1_hmac_sha256_initialize(&hmac, rng->k, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, rng->v, 32); |
||||
secp256k1_hmac_sha256_finalize(&hmac, rng->v); |
||||
rng->retry = 0; |
||||
} |
||||
|
||||
static void secp256k1_rfc6979_hmac_sha256_generate(secp256k1_rfc6979_hmac_sha256_t *rng, unsigned char *out, size_t outlen) { |
||||
static const unsigned char zero[1] = {0x00}; |
||||
if (rng->retry) { |
||||
secp256k1_hmac_sha256_t hmac; |
||||
secp256k1_hmac_sha256_initialize(&hmac, rng->k, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, rng->v, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, zero, 1); |
||||
secp256k1_hmac_sha256_finalize(&hmac, rng->k); |
||||
secp256k1_hmac_sha256_initialize(&hmac, rng->k, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, rng->v, 32); |
||||
secp256k1_hmac_sha256_finalize(&hmac, rng->v); |
||||
} |
||||
|
||||
while (outlen > 0) { |
||||
secp256k1_hmac_sha256_t hmac; |
||||
secp256k1_hmac_sha256_initialize(&hmac, rng->k, 32); |
||||
secp256k1_hmac_sha256_write(&hmac, rng->v, 32); |
||||
secp256k1_hmac_sha256_finalize(&hmac, rng->v); |
||||
int now = outlen; |
||||
if (now > 32) { |
||||
now = 32; |
||||
} |
||||
memcpy(out, rng->v, now); |
||||
out += now; |
||||
outlen -= now; |
||||
} |
||||
|
||||
rng->retry = 1; |
||||
} |
||||
|
||||
static void secp256k1_rfc6979_hmac_sha256_finalize(secp256k1_rfc6979_hmac_sha256_t *rng) { |
||||
memset(rng->k, 0, 32); |
||||
memset(rng->v, 0, 32); |
||||
rng->retry = 0; |
||||
} |
||||
|
||||
|
||||
#undef Round |
||||
#undef sigma0 |
||||
#undef sigma1 |
||||
#undef Sigma0 |
||||
#undef Sigma1 |
||||
#undef Ch |
||||
#undef Maj |
||||
#undef ReadBE32 |
||||
#undef WriteBE32 |
||||
|
||||
#endif |
Loading…
Reference in new issue