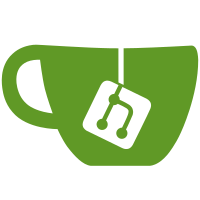
11 changed files with 454 additions and 4 deletions
@ -0,0 +1,43 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<ui version="4.0"> |
||||||
|
<class>KevaBookmarksDialog</class> |
||||||
|
<widget class="QDialog" name="KevaBookmarksDialog"> |
||||||
|
<property name="geometry"> |
||||||
|
<rect> |
||||||
|
<x>0</x> |
||||||
|
<y>0</y> |
||||||
|
<width>800</width> |
||||||
|
<height>400</height> |
||||||
|
</rect> |
||||||
|
</property> |
||||||
|
<property name="windowTitle"> |
||||||
|
<string notr="true">Namespace Bookmarks</string> |
||||||
|
</property> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout"> |
||||||
|
<item> |
||||||
|
<widget class="QTableView" name="namespaceView"> |
||||||
|
<property name="contextMenuPolicy"> |
||||||
|
<enum>Qt::CustomContextMenu</enum> |
||||||
|
</property> |
||||||
|
<property name="tabKeyNavigation"> |
||||||
|
<bool>false</bool> |
||||||
|
</property> |
||||||
|
<property name="sortingEnabled"> |
||||||
|
<bool>true</bool> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QDialogButtonBox" name="buttonBox"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="standardButtons"> |
||||||
|
<set>QDialogButtonBox::Apply|QDialogButtonBox::Cancel</set> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
<resources/> |
||||||
|
</ui> |
@ -0,0 +1,78 @@ |
|||||||
|
// Copyright (c) 2011-2017 The Bitcoin Core developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#include <qt/kevabookmarksdialog.h> |
||||||
|
#include <qt/forms/ui_kevabookmarksdialog.h> |
||||||
|
|
||||||
|
#include <qt/kevabookmarksmodel.h> |
||||||
|
#include <qt/kevadialog.h> |
||||||
|
|
||||||
|
#include <QPushButton> |
||||||
|
#include <QModelIndex> |
||||||
|
|
||||||
|
KevaBookmarksDialog::KevaBookmarksDialog(QWidget *parent) : |
||||||
|
QDialog(parent), |
||||||
|
ui(new Ui::KevaBookmarksDialog) |
||||||
|
{ |
||||||
|
ui->setupUi(this); |
||||||
|
ui->buttonBox->button(QDialogButtonBox::Apply)->setEnabled(false); |
||||||
|
ui->buttonBox->button(QDialogButtonBox::Apply)->setText(tr("Show")); |
||||||
|
connect(ui->buttonBox->button(QDialogButtonBox::Cancel), SIGNAL(clicked()), this, SLOT(reject())); |
||||||
|
connect(ui->buttonBox->button(QDialogButtonBox::Apply), SIGNAL(clicked()), this, SLOT(apply())); |
||||||
|
} |
||||||
|
|
||||||
|
void KevaBookmarksDialog::setModel(WalletModel *_model) |
||||||
|
{ |
||||||
|
this->model = _model; |
||||||
|
|
||||||
|
if(_model && _model->getOptionsModel()) |
||||||
|
{ |
||||||
|
_model->getKevaBookmarksModel()->sort(KevaBookmarksModel::Name, Qt::DescendingOrder); |
||||||
|
QTableView* tableView = ui->namespaceView; |
||||||
|
|
||||||
|
tableView->verticalHeader()->hide(); |
||||||
|
tableView->setHorizontalScrollBarPolicy(Qt::ScrollBarAlwaysOff); |
||||||
|
tableView->setModel(_model->getKevaBookmarksModel()); |
||||||
|
tableView->setAlternatingRowColors(true); |
||||||
|
tableView->setSelectionBehavior(QAbstractItemView::SelectRows); |
||||||
|
tableView->setSelectionMode(QAbstractItemView::ContiguousSelection); |
||||||
|
tableView->horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch); |
||||||
|
|
||||||
|
connect(tableView->selectionModel(), SIGNAL(selectionChanged(QItemSelection,QItemSelection)), |
||||||
|
this, SLOT(namespaceView_selectionChanged())); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
void KevaBookmarksDialog::namespaceView_selectionChanged() |
||||||
|
{ |
||||||
|
bool enable = !ui->namespaceView->selectionModel()->selectedRows().isEmpty(); |
||||||
|
ui->buttonBox->button(QDialogButtonBox::Apply)->setEnabled(enable); |
||||||
|
|
||||||
|
if (enable) { |
||||||
|
selectedIndex = ui->namespaceView->selectionModel()->currentIndex(); |
||||||
|
} else { |
||||||
|
QModelIndex empty; |
||||||
|
selectedIndex = empty; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void KevaBookmarksDialog::apply() |
||||||
|
{ |
||||||
|
QModelIndex idIdx = selectedIndex.sibling(selectedIndex.row(), KevaBookmarksModel::Id); |
||||||
|
QString idStr = idIdx.data(Qt::DisplayRole).toString(); |
||||||
|
KevaDialog* dialog = (KevaDialog*)this->parentWidget(); |
||||||
|
dialog->showNamespace(idStr); |
||||||
|
QDialog::close(); |
||||||
|
} |
||||||
|
|
||||||
|
void KevaBookmarksDialog::reject() |
||||||
|
{ |
||||||
|
QDialog::reject(); |
||||||
|
} |
||||||
|
|
||||||
|
KevaBookmarksDialog::~KevaBookmarksDialog() |
||||||
|
{ |
||||||
|
delete ui; |
||||||
|
} |
@ -0,0 +1,51 @@ |
|||||||
|
// Copyright (c) 2011-2014 The Bitcoin Core developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#ifndef BITCOIN_QT_KEVABOOKMARKSDIALOG_H |
||||||
|
#define BITCOIN_QT_KEVABOOKMARKSDIALOG_H |
||||||
|
|
||||||
|
#include <QObject> |
||||||
|
#include <QString> |
||||||
|
|
||||||
|
#include <QDialog> |
||||||
|
#include <QItemSelection> |
||||||
|
#include <QAbstractItemView> |
||||||
|
|
||||||
|
class WalletModel; |
||||||
|
|
||||||
|
namespace Ui { |
||||||
|
class KevaBookmarksDialog; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** Dialog showing namepsace creation. */ |
||||||
|
class KevaBookmarksDialog : public QDialog |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
enum ColumnWidths { |
||||||
|
ID_COLUMN_WIDTH = 260, |
||||||
|
NAME_COLUMN_WIDTH = 260, |
||||||
|
MINIMUM_COLUMN_WIDTH = 260 |
||||||
|
}; |
||||||
|
|
||||||
|
public: |
||||||
|
explicit KevaBookmarksDialog(QWidget *parent = 0); |
||||||
|
~KevaBookmarksDialog(); |
||||||
|
void setModel(WalletModel *_model); |
||||||
|
|
||||||
|
public Q_SLOTS: |
||||||
|
void apply(); |
||||||
|
void reject(); |
||||||
|
|
||||||
|
private Q_SLOTS: |
||||||
|
void namespaceView_selectionChanged(); |
||||||
|
|
||||||
|
private: |
||||||
|
Ui::KevaBookmarksDialog *ui; |
||||||
|
WalletModel *model; |
||||||
|
QModelIndex selectedIndex; |
||||||
|
}; |
||||||
|
|
||||||
|
#endif // BITCOIN_QT_KEVABOOKMARKSDIALOG_H
|
@ -0,0 +1,152 @@ |
|||||||
|
// Copyright (c) 2011-2017 The Bitcoin Core developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#include <qt/kevabookmarksmodel.h> |
||||||
|
|
||||||
|
#include <qt/bitcoinunits.h> |
||||||
|
#include <qt/guiutil.h> |
||||||
|
#include <qt/optionsmodel.h> |
||||||
|
|
||||||
|
#include <clientversion.h> |
||||||
|
#include <streams.h> |
||||||
|
|
||||||
|
|
||||||
|
KevaBookmarksModel::KevaBookmarksModel(CWallet *wallet, WalletModel *parent) : |
||||||
|
QAbstractTableModel(parent), walletModel(parent) |
||||||
|
{ |
||||||
|
Q_UNUSED(wallet) |
||||||
|
|
||||||
|
/* These columns must match the indices in the ColumnIndex enumeration */ |
||||||
|
columns << tr("Id") << tr("Name"); |
||||||
|
} |
||||||
|
|
||||||
|
KevaBookmarksModel::~KevaBookmarksModel() |
||||||
|
{ |
||||||
|
/* Intentionally left empty */ |
||||||
|
} |
||||||
|
|
||||||
|
int KevaBookmarksModel::rowCount(const QModelIndex &parent) const |
||||||
|
{ |
||||||
|
Q_UNUSED(parent); |
||||||
|
|
||||||
|
return list.length(); |
||||||
|
} |
||||||
|
|
||||||
|
int KevaBookmarksModel::columnCount(const QModelIndex &parent) const |
||||||
|
{ |
||||||
|
Q_UNUSED(parent); |
||||||
|
|
||||||
|
return columns.length(); |
||||||
|
} |
||||||
|
|
||||||
|
QVariant KevaBookmarksModel::data(const QModelIndex &index, int role) const |
||||||
|
{ |
||||||
|
if(!index.isValid() || index.row() >= list.length()) |
||||||
|
return QVariant(); |
||||||
|
|
||||||
|
if (role == Qt::TextColorRole) |
||||||
|
{ |
||||||
|
return QVariant(); |
||||||
|
} |
||||||
|
else if(role == Qt::DisplayRole || role == Qt::EditRole) |
||||||
|
{ |
||||||
|
const BookmarkEntry *rec = &list[index.row()]; |
||||||
|
switch(index.column()) |
||||||
|
{ |
||||||
|
case Id: |
||||||
|
return QString::fromStdString(rec->id); |
||||||
|
case Name: |
||||||
|
return QString::fromStdString(rec->name); |
||||||
|
} |
||||||
|
} |
||||||
|
else if (role == Qt::TextAlignmentRole) |
||||||
|
{ |
||||||
|
return (int)(Qt::AlignLeft|Qt::AlignVCenter); |
||||||
|
} |
||||||
|
return QVariant(); |
||||||
|
} |
||||||
|
|
||||||
|
bool KevaBookmarksModel::setData(const QModelIndex &index, const QVariant &value, int role) |
||||||
|
{ |
||||||
|
return true; |
||||||
|
} |
||||||
|
|
||||||
|
QVariant KevaBookmarksModel::headerData(int section, Qt::Orientation orientation, int role) const |
||||||
|
{ |
||||||
|
if(orientation == Qt::Horizontal) |
||||||
|
{ |
||||||
|
if(role == Qt::DisplayRole && section < columns.size()) |
||||||
|
{ |
||||||
|
return columns[section]; |
||||||
|
} |
||||||
|
} |
||||||
|
return QVariant(); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
QModelIndex KevaBookmarksModel::index(int row, int column, const QModelIndex &parent) const |
||||||
|
{ |
||||||
|
Q_UNUSED(parent); |
||||||
|
|
||||||
|
return createIndex(row, column); |
||||||
|
} |
||||||
|
|
||||||
|
bool KevaBookmarksModel::removeRows(int row, int count, const QModelIndex &parent) |
||||||
|
{ |
||||||
|
Q_UNUSED(parent); |
||||||
|
|
||||||
|
if(count > 0 && row >= 0 && (row+count) <= list.size()) |
||||||
|
{ |
||||||
|
beginRemoveRows(parent, row, row + count - 1); |
||||||
|
list.erase(list.begin() + row, list.begin() + row + count); |
||||||
|
endRemoveRows(); |
||||||
|
return true; |
||||||
|
} else { |
||||||
|
return false; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
Qt::ItemFlags KevaBookmarksModel::flags(const QModelIndex &index) const |
||||||
|
{ |
||||||
|
return Qt::ItemIsSelectable | Qt::ItemIsEnabled; |
||||||
|
} |
||||||
|
|
||||||
|
// actually add to table in GUI
|
||||||
|
void KevaBookmarksModel::setBookmarks(std::vector<BookmarkEntry> vBookmarEntries) |
||||||
|
{ |
||||||
|
// Remove the old ones.
|
||||||
|
removeRows(0, list.size()); |
||||||
|
list.clear(); |
||||||
|
|
||||||
|
for (auto it = vBookmarEntries.begin(); it != vBookmarEntries.end(); it++) { |
||||||
|
beginInsertRows(QModelIndex(), 0, 0); |
||||||
|
list.prepend(*it); |
||||||
|
endInsertRows(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void KevaBookmarksModel::sort(int column, Qt::SortOrder order) |
||||||
|
{ |
||||||
|
qSort(list.begin(), list.end(), BookmarkEntryLessThan(column, order)); |
||||||
|
Q_EMIT dataChanged(index(0, 0, QModelIndex()), index(list.size() - 1, NUMBER_OF_COLUMNS - 1, QModelIndex())); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
bool BookmarkEntryLessThan::operator()(BookmarkEntry &left, BookmarkEntry &right) const |
||||||
|
{ |
||||||
|
BookmarkEntry *pLeft = &left; |
||||||
|
BookmarkEntry *pRight = &right; |
||||||
|
if (order == Qt::DescendingOrder) |
||||||
|
std::swap(pLeft, pRight); |
||||||
|
|
||||||
|
switch(column) |
||||||
|
{ |
||||||
|
case KevaBookmarksModel::Id: |
||||||
|
return pLeft->id < pRight->id; |
||||||
|
case KevaBookmarksModel::Name: |
||||||
|
return pLeft->name < pRight->name; |
||||||
|
default: |
||||||
|
return pLeft->id < pRight->id; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,79 @@ |
|||||||
|
// Copyright (c) 2011-2017 The Bitcoin Core developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#ifndef BITCOIN_QT_KEVABOOKMARKSMODEL_H |
||||||
|
#define BITCOIN_QT_KEVABOOKMARKSMODEL_H |
||||||
|
|
||||||
|
#include <qt/walletmodel.h> |
||||||
|
|
||||||
|
#include <QAbstractTableModel> |
||||||
|
#include <QStringList> |
||||||
|
#include <QDateTime> |
||||||
|
|
||||||
|
class CWallet; |
||||||
|
|
||||||
|
class BookmarkEntry |
||||||
|
{ |
||||||
|
public: |
||||||
|
BookmarkEntry() { } |
||||||
|
|
||||||
|
std::string id; |
||||||
|
std::string name; |
||||||
|
}; |
||||||
|
|
||||||
|
class BookmarkEntryLessThan |
||||||
|
{ |
||||||
|
public: |
||||||
|
BookmarkEntryLessThan(int nColumn, Qt::SortOrder fOrder): |
||||||
|
column(nColumn), order(fOrder) {} |
||||||
|
bool operator()(BookmarkEntry &left, BookmarkEntry &right) const; |
||||||
|
|
||||||
|
private: |
||||||
|
int column; |
||||||
|
Qt::SortOrder order; |
||||||
|
}; |
||||||
|
|
||||||
|
/** Model for list of recently generated payment requests / bitcoin: URIs.
|
||||||
|
* Part of wallet model. |
||||||
|
*/ |
||||||
|
class KevaBookmarksModel: public QAbstractTableModel |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
public: |
||||||
|
explicit KevaBookmarksModel(CWallet *wallet, WalletModel *parent); |
||||||
|
~KevaBookmarksModel(); |
||||||
|
|
||||||
|
enum ColumnIndex { |
||||||
|
Id = 0, |
||||||
|
Name = 1, |
||||||
|
NUMBER_OF_COLUMNS |
||||||
|
}; |
||||||
|
|
||||||
|
/** @name Methods overridden from QAbstractTableModel
|
||||||
|
@{*/ |
||||||
|
int rowCount(const QModelIndex &parent) const; |
||||||
|
int columnCount(const QModelIndex &parent) const; |
||||||
|
QVariant data(const QModelIndex &index, int role) const; |
||||||
|
bool setData(const QModelIndex &index, const QVariant &value, int role); |
||||||
|
QVariant headerData(int section, Qt::Orientation orientation, int role) const; |
||||||
|
QModelIndex index(int row, int column, const QModelIndex &parent) const; |
||||||
|
bool removeRows(int row, int count, const QModelIndex &parent = QModelIndex()); |
||||||
|
Qt::ItemFlags flags(const QModelIndex &index) const; |
||||||
|
/*@}*/ |
||||||
|
|
||||||
|
const BookmarkEntry &entry(int row) const { return list[row]; } |
||||||
|
void setBookmarks(std::vector<BookmarkEntry> vBookmarkEntries); |
||||||
|
|
||||||
|
public Q_SLOTS: |
||||||
|
void sort(int column, Qt::SortOrder order = Qt::AscendingOrder); |
||||||
|
|
||||||
|
private: |
||||||
|
WalletModel *walletModel; |
||||||
|
QStringList columns; |
||||||
|
QList<BookmarkEntry> list; |
||||||
|
int64_t nReceiveRequestsMaxId; |
||||||
|
}; |
||||||
|
|
||||||
|
#endif // BITCOIN_QT_KEVABOOKMARKSMODEL_H
|
Loading…
Reference in new issue