Browse Source
There were too many levels of indirection here, and the functionality of walletframe and walletstack can easily be merged. This commit merges the two which cuts a lot of lines of boilerplate code.0.10
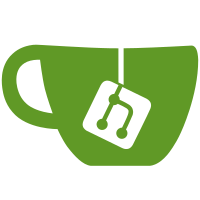
7 changed files with 106 additions and 324 deletions
@ -1,174 +0,0 @@ |
|||||||
/*
|
|
||||||
* Qt4 bitcoin GUI. |
|
||||||
* |
|
||||||
* W.J. van der Laan 2011-2012 |
|
||||||
* The Bitcoin Developers 2011-2013 |
|
||||||
*/ |
|
||||||
#include "walletstack.h" |
|
||||||
#include "walletview.h" |
|
||||||
#include "bitcoingui.h" |
|
||||||
|
|
||||||
#include <QMap> |
|
||||||
#include <QMessageBox> |
|
||||||
|
|
||||||
WalletStack::WalletStack(QWidget *parent) : |
|
||||||
QStackedWidget(parent), |
|
||||||
gui(0), |
|
||||||
clientModel(0), |
|
||||||
bOutOfSync(true) |
|
||||||
{ |
|
||||||
setContentsMargins(0,0,0,0); |
|
||||||
} |
|
||||||
|
|
||||||
WalletStack::~WalletStack() |
|
||||||
{ |
|
||||||
} |
|
||||||
|
|
||||||
bool WalletStack::addWallet(const QString& name, WalletModel *walletModel) |
|
||||||
{ |
|
||||||
if (!gui || !clientModel || !walletModel || mapWalletViews.count(name) > 0) |
|
||||||
return false; |
|
||||||
|
|
||||||
WalletView *walletView = new WalletView(this, gui); |
|
||||||
walletView->setBitcoinGUI(gui); |
|
||||||
walletView->setClientModel(clientModel); |
|
||||||
walletView->setWalletModel(walletModel); |
|
||||||
walletView->showOutOfSyncWarning(bOutOfSync); |
|
||||||
addWidget(walletView); |
|
||||||
mapWalletViews[name] = walletView; |
|
||||||
|
|
||||||
// Ensure a walletView is able to show the main window
|
|
||||||
connect(walletView, SIGNAL(showNormalIfMinimized()), gui, SLOT(showNormalIfMinimized())); |
|
||||||
|
|
||||||
return true; |
|
||||||
} |
|
||||||
|
|
||||||
bool WalletStack::removeWallet(const QString& name) |
|
||||||
{ |
|
||||||
if (mapWalletViews.count(name) == 0) |
|
||||||
return false; |
|
||||||
|
|
||||||
WalletView *walletView = mapWalletViews.take(name); |
|
||||||
removeWidget(walletView); |
|
||||||
return true; |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::removeAllWallets() |
|
||||||
{ |
|
||||||
QMap<QString, WalletView*>::const_iterator i; |
|
||||||
for (i = mapWalletViews.constBegin(); i != mapWalletViews.constEnd(); ++i) |
|
||||||
removeWidget(i.value()); |
|
||||||
mapWalletViews.clear(); |
|
||||||
} |
|
||||||
|
|
||||||
bool WalletStack::handlePaymentRequest(const SendCoinsRecipient &recipient) |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (!walletView) |
|
||||||
return false; |
|
||||||
|
|
||||||
return walletView->handlePaymentRequest(recipient); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::showOutOfSyncWarning(bool fShow) |
|
||||||
{ |
|
||||||
bOutOfSync = fShow; |
|
||||||
QMap<QString, WalletView*>::const_iterator i; |
|
||||||
for (i = mapWalletViews.constBegin(); i != mapWalletViews.constEnd(); ++i) |
|
||||||
i.value()->showOutOfSyncWarning(fShow); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::gotoOverviewPage() |
|
||||||
{ |
|
||||||
QMap<QString, WalletView*>::const_iterator i; |
|
||||||
for (i = mapWalletViews.constBegin(); i != mapWalletViews.constEnd(); ++i) |
|
||||||
i.value()->gotoOverviewPage(); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::gotoHistoryPage() |
|
||||||
{ |
|
||||||
QMap<QString, WalletView*>::const_iterator i; |
|
||||||
for (i = mapWalletViews.constBegin(); i != mapWalletViews.constEnd(); ++i) |
|
||||||
i.value()->gotoHistoryPage(); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::gotoAddressBookPage() |
|
||||||
{ |
|
||||||
QMap<QString, WalletView*>::const_iterator i; |
|
||||||
for (i = mapWalletViews.constBegin(); i != mapWalletViews.constEnd(); ++i) |
|
||||||
i.value()->gotoAddressBookPage(); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::gotoReceiveCoinsPage() |
|
||||||
{ |
|
||||||
QMap<QString, WalletView*>::const_iterator i; |
|
||||||
for (i = mapWalletViews.constBegin(); i != mapWalletViews.constEnd(); ++i) |
|
||||||
i.value()->gotoReceiveCoinsPage(); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::gotoSendCoinsPage(QString addr) |
|
||||||
{ |
|
||||||
QMap<QString, WalletView*>::const_iterator i; |
|
||||||
for (i = mapWalletViews.constBegin(); i != mapWalletViews.constEnd(); ++i) |
|
||||||
i.value()->gotoSendCoinsPage(addr); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::gotoSignMessageTab(QString addr) |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (walletView) |
|
||||||
walletView->gotoSignMessageTab(addr); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::gotoVerifyMessageTab(QString addr) |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (walletView) |
|
||||||
walletView->gotoVerifyMessageTab(addr); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::encryptWallet(bool status) |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (walletView) |
|
||||||
walletView->encryptWallet(status); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::backupWallet() |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (walletView) |
|
||||||
walletView->backupWallet(); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::changePassphrase() |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (walletView) |
|
||||||
walletView->changePassphrase(); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::unlockWallet() |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (walletView) |
|
||||||
walletView->unlockWallet(); |
|
||||||
} |
|
||||||
|
|
||||||
void WalletStack::setEncryptionStatus() |
|
||||||
{ |
|
||||||
WalletView *walletView = (WalletView*)currentWidget(); |
|
||||||
if (walletView) |
|
||||||
walletView->setEncryptionStatus(); |
|
||||||
} |
|
||||||
|
|
||||||
bool WalletStack::setCurrentWallet(const QString& name) |
|
||||||
{ |
|
||||||
if (mapWalletViews.count(name) == 0) |
|
||||||
return false; |
|
||||||
|
|
||||||
WalletView *walletView = mapWalletViews.value(name); |
|
||||||
setCurrentWidget(walletView); |
|
||||||
walletView->setEncryptionStatus(); |
|
||||||
return true; |
|
||||||
} |
|
@ -1,104 +0,0 @@ |
|||||||
/*
|
|
||||||
* Qt4 bitcoin GUI. |
|
||||||
* |
|
||||||
* W.J. van der Laan 2011-2012 |
|
||||||
* The Bitcoin Developers 2011-2013 |
|
||||||
*/ |
|
||||||
#ifndef WALLETSTACK_H |
|
||||||
#define WALLETSTACK_H |
|
||||||
|
|
||||||
#include <QStackedWidget> |
|
||||||
#include <QMap> |
|
||||||
#include <boost/shared_ptr.hpp> |
|
||||||
|
|
||||||
class BitcoinGUI; |
|
||||||
class TransactionTableModel; |
|
||||||
class ClientModel; |
|
||||||
class WalletModel; |
|
||||||
class WalletView; |
|
||||||
class TransactionView; |
|
||||||
class OverviewPage; |
|
||||||
class AddressBookPage; |
|
||||||
class SendCoinsDialog; |
|
||||||
class SendCoinsRecipient; |
|
||||||
class SignVerifyMessageDialog; |
|
||||||
class Notificator; |
|
||||||
class RPCConsole; |
|
||||||
|
|
||||||
class CWalletManager; |
|
||||||
|
|
||||||
QT_BEGIN_NAMESPACE |
|
||||||
class QLabel; |
|
||||||
class QModelIndex; |
|
||||||
QT_END_NAMESPACE |
|
||||||
|
|
||||||
/*
|
|
||||||
WalletStack class. This class is a container for WalletView instances. It takes the place of centralWidget. |
|
||||||
It was added to support multiple wallet functionality. It communicates with both the client and the |
|
||||||
wallet models to give the user an up-to-date view of the current core state. It manages all the wallet views |
|
||||||
it contains and updates them accordingly. |
|
||||||
*/ |
|
||||||
class WalletStack : public QStackedWidget |
|
||||||
{ |
|
||||||
Q_OBJECT |
|
||||||
|
|
||||||
public: |
|
||||||
explicit WalletStack(QWidget *parent = 0); |
|
||||||
~WalletStack(); |
|
||||||
|
|
||||||
void setBitcoinGUI(BitcoinGUI *gui) { this->gui = gui; } |
|
||||||
|
|
||||||
void setClientModel(ClientModel *clientModel) { this->clientModel = clientModel; } |
|
||||||
|
|
||||||
bool addWallet(const QString& name, WalletModel *walletModel); |
|
||||||
bool removeWallet(const QString& name); |
|
||||||
|
|
||||||
void removeAllWallets(); |
|
||||||
|
|
||||||
bool handlePaymentRequest(const SendCoinsRecipient &recipient); |
|
||||||
|
|
||||||
void showOutOfSyncWarning(bool fShow); |
|
||||||
|
|
||||||
private: |
|
||||||
BitcoinGUI *gui; |
|
||||||
ClientModel *clientModel; |
|
||||||
QMap<QString, WalletView*> mapWalletViews; |
|
||||||
|
|
||||||
bool bOutOfSync; |
|
||||||
|
|
||||||
public slots: |
|
||||||
bool setCurrentWallet(const QString& name); |
|
||||||
|
|
||||||
/** Switch to overview (home) page */ |
|
||||||
void gotoOverviewPage(); |
|
||||||
/** Switch to history (transactions) page */ |
|
||||||
void gotoHistoryPage(); |
|
||||||
/** Switch to address book page */ |
|
||||||
void gotoAddressBookPage(); |
|
||||||
/** Switch to receive coins page */ |
|
||||||
void gotoReceiveCoinsPage(); |
|
||||||
/** Switch to send coins page */ |
|
||||||
void gotoSendCoinsPage(QString addr = ""); |
|
||||||
|
|
||||||
/** Show Sign/Verify Message dialog and switch to sign message tab */ |
|
||||||
void gotoSignMessageTab(QString addr = ""); |
|
||||||
/** Show Sign/Verify Message dialog and switch to verify message tab */ |
|
||||||
void gotoVerifyMessageTab(QString addr = ""); |
|
||||||
|
|
||||||
/** Encrypt the wallet */ |
|
||||||
void encryptWallet(bool status); |
|
||||||
/** Backup the wallet */ |
|
||||||
void backupWallet(); |
|
||||||
/** Change encrypted wallet passphrase */ |
|
||||||
void changePassphrase(); |
|
||||||
/** Ask for passphrase to unlock wallet temporarily */ |
|
||||||
void unlockWallet(); |
|
||||||
|
|
||||||
/** Set the encryption status as shown in the UI.
|
|
||||||
@param[in] status current encryption status |
|
||||||
@see WalletModel::EncryptionStatus |
|
||||||
*/ |
|
||||||
void setEncryptionStatus(); |
|
||||||
}; |
|
||||||
|
|
||||||
#endif // WALLETSTACK_H
|
|
Loading…
Reference in new issue