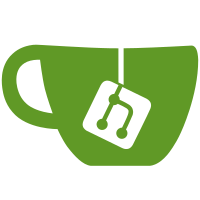
19 changed files with 19 additions and 326 deletions
@ -1,7 +0,0 @@
@@ -1,7 +0,0 @@
|
||||
Makefile |
||||
CmakeCache.txt |
||||
cmake_install.cmake |
||||
CMakeFiles |
||||
*.a |
||||
*.so |
||||
*.dylib |
@ -1,44 +0,0 @@
@@ -1,44 +0,0 @@
|
||||
set(CXXLIB "cnutil") |
||||
|
||||
find_package(Boost COMPONENTS thread system program_options date_time filesystem REQUIRED) |
||||
|
||||
# Flags |
||||
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -std=c++11 -D_GNU_SOURCE") |
||||
|
||||
include_directories(${Boost_INCLUDE_DIRS}) |
||||
include_directories("${MONERO_DIR}/contrib/epee/include") |
||||
include_directories("${MONERO_DIR}/src") |
||||
include_directories("${MONERO_DIR}/external/easylogging++") |
||||
|
||||
link_directories( |
||||
${MONERO_DIR}/src/cryptonote_core |
||||
${MONERO_DIR}/src/cryptonote_basic |
||||
${MONERO_DIR}/src/crypto |
||||
${MONERO_DIR}/src/common |
||||
${MONERO_DIR}/src/ringct |
||||
${MONERO_DIR}/src/blockchain_db |
||||
${MONERO_DIR}/external/easylogging++ |
||||
${MONERO_DIR}/contrib/epee/src |
||||
) |
||||
|
||||
# Build library |
||||
add_library(${CXXLIB} SHARED src/cnutil.cpp) |
||||
|
||||
target_link_libraries(${CXXLIB} |
||||
cryptonote_core |
||||
cryptonote_basic |
||||
cncrypto |
||||
common |
||||
ringct |
||||
blockchain_db |
||||
easylogging |
||||
epee |
||||
) |
||||
|
||||
target_link_libraries(${CXXLIB} |
||||
${Boost_THREAD_LIBRARY} |
||||
${Boost_SYSTEM_LIBRARY} |
||||
${Boost_PROGRAM_OPTIONS_LIBRARY} |
||||
${Boost_DATE_TIME_LIBRARY} |
||||
${Boost_FILESYSTEM_LIBRARY} |
||||
) |
@ -1,28 +0,0 @@
@@ -1,28 +0,0 @@
|
||||
package cnutil |
||||
|
||||
// #cgo CFLAGS: -std=c11 -D_GNU_SOURCE
|
||||
// #cgo LDFLAGS: -L${SRCDIR} -lcnutil -Wl,-rpath ${SRCDIR} -lstdc++
|
||||
// #include <stdlib.h>
|
||||
// #include "src/cnutil.h"
|
||||
import "C" |
||||
import "unsafe" |
||||
|
||||
func ConvertBlob(blob []byte) []byte { |
||||
output := make([]byte, 76) |
||||
out := (*C.char)(unsafe.Pointer(&output[0])) |
||||
|
||||
input := (*C.char)(unsafe.Pointer(&blob[0])) |
||||
|
||||
size := (C.uint32_t)(len(blob)) |
||||
C.convert_blob(input, size, out) |
||||
return output |
||||
} |
||||
|
||||
func ValidateAddress(addr string) bool { |
||||
input := C.CString(addr) |
||||
defer C.free(unsafe.Pointer(input)) |
||||
|
||||
size := (C.uint32_t)(len(addr)) |
||||
result := C.validate_address(input, size) |
||||
return (bool)(result) |
||||
} |
@ -1,55 +0,0 @@
@@ -1,55 +0,0 @@
|
||||
package cnutil |
||||
|
||||
import ( |
||||
"encoding/hex" |
||||
"testing" |
||||
) |
||||
|
||||
func TestConvertBlob(t *testing.T) { |
||||
hashBytes, _ := hex.DecodeString("0100a5d1fca9057dff46d140d453a672437ba0ec7d6a74bc5fa0391f8a918e41fd7ba2cf6fc1af000000000183811401ffc7801405889ec5dc2402e0fe0db63a8e532a7b988e0c32a764e5e8d64d7efac9bc9d24ce32b0984ab93980b09dc2df0102ccd38432501a9182ccc5b44cb47abdddbc9a6321cd581f5f07a7a9195795d5c68080dd9da41702f6e944ee4c6e1eeaed1fa6a3c2480a410e959c6e823b96dad54d31fe223cc0fd80c0a8ca9a3a0286d0e3411670e4c2abe8492c695c66d8262660ee88a0b14a2b03c9180fb6f0d480c0caf384a30202aec5c9b7efe841dd821476e0e06217be13a4c85a83efcf9576314d60130e02e72b0150526f7a381cec33e5827c1848dd80e6eac4b262304ea06b3a43303a4631df28020800000000018ba82000") |
||||
expectedResult, _ := hex.DecodeString("0100a5d1fca9057dff46d140d453a672437ba0ec7d6a74bc5fa0391f8a918e41fd7ba2cf6fc1af00000000e81cb2bf0d2c5054a49bda094c39cb263a9565b9b81cf4c4f848292040419f4a01") |
||||
output := ConvertBlob(hashBytes) |
||||
|
||||
if len(output) != 76 { |
||||
t.Error("Invalid result length") |
||||
} |
||||
ok := true |
||||
for i := range output { |
||||
if expectedResult[i] != output[i] { |
||||
ok = false |
||||
break |
||||
} |
||||
} |
||||
if !ok { |
||||
t.Error("Invalid result") |
||||
} |
||||
} |
||||
|
||||
func TestDecodeAddress(t *testing.T) { |
||||
addy := "45pyCXYn2UBVUmCFjgKr7LF8hCTeGwucWJ2xni7qrbj6GgAZBFY6tANarozZx9DaQqHyuR1AL8HJbRmqwLhUaDpKJW4hqS1" |
||||
if !ValidateAddress(addy) { |
||||
t.Error("Valid address") |
||||
} |
||||
|
||||
addy = "46BeWrHpwXmHDpDEUmZBWZfoQpdc6HaERCNmx1pEYL2rAcuwufPN9rXHHtyUA4QVy66qeFQkn6sfK8aHYjA3jk3o1Bv16em" |
||||
if !ValidateAddress(addy) { |
||||
t.Error("Valid address") |
||||
} |
||||
|
||||
if ValidateAddress("OMG") { |
||||
t.Error("Invalid address") |
||||
} |
||||
} |
||||
|
||||
func BenchmarkConvertBlob(b *testing.B) { |
||||
for i := 0; i < b.N; i++ { |
||||
hashBytes, _ := hex.DecodeString("0100a5d1fca9057dff46d140d453a672437ba0ec7d6a74bc5fa0391f8a918e41fd7ba2cf6fc1af000000000183811401ffc7801405889ec5dc2402e0fe0db63a8e532a7b988e0c32a764e5e8d64d7efac9bc9d24ce32b0984ab93980b09dc2df0102ccd38432501a9182ccc5b44cb47abdddbc9a6321cd581f5f07a7a9195795d5c68080dd9da41702f6e944ee4c6e1eeaed1fa6a3c2480a410e959c6e823b96dad54d31fe223cc0fd80c0a8ca9a3a0286d0e3411670e4c2abe8492c695c66d8262660ee88a0b14a2b03c9180fb6f0d480c0caf384a30202aec5c9b7efe841dd821476e0e06217be13a4c85a83efcf9576314d60130e02e72b0150526f7a381cec33e5827c1848dd80e6eac4b262304ea06b3a43303a4631df28020800000000018ba82000") |
||||
ConvertBlob(hashBytes) |
||||
} |
||||
} |
||||
|
||||
func BenchmarkDecodeAddress(b *testing.B) { |
||||
for i := 0; i < b.N; i++ { |
||||
ValidateAddress("45pyCXYn2UBVUmCFjgKr7LF8hCTeGwucWJ2xni7qrbj6GgAZBFY6tANarozZx9DaQqHyuR1AL8HJbRmqwLhUaDpKJW4hqS1") |
||||
} |
||||
} |
@ -1,27 +0,0 @@
@@ -1,27 +0,0 @@
|
||||
#include <stdint.h> |
||||
#include <string> |
||||
#include "cryptonote_basic/cryptonote_format_utils.h" |
||||
#include "common/base58.h" |
||||
|
||||
using namespace cryptonote; |
||||
|
||||
extern "C" uint32_t convert_blob(const char *blob, size_t len, char *out) { |
||||
std::string input = std::string(blob, len); |
||||
std::string output = ""; |
||||
|
||||
block b = AUTO_VAL_INIT(b); |
||||
if (!parse_and_validate_block_from_blob(input, b)) { |
||||
return 0; |
||||
} |
||||
|
||||
output = get_block_hashing_blob(b); |
||||
output.copy(out, output.length(), 0); |
||||
return output.length(); |
||||
} |
||||
|
||||
extern "C" bool validate_address(const char *addr, size_t len) { |
||||
std::string input = std::string(addr, len); |
||||
std::string output = ""; |
||||
uint64_t prefix; |
||||
return tools::base58::decode_addr(addr, prefix, output); |
||||
} |
@ -1,13 +0,0 @@
@@ -1,13 +0,0 @@
|
||||
#include <stdint.h> |
||||
#include "stdbool.h" |
||||
|
||||
#ifdef __cplusplus |
||||
extern "C" { |
||||
#endif |
||||
|
||||
uint32_t convert_blob(const char *blob, uint32_t len, char *out); |
||||
bool validate_address(const char *addr, uint32_t len); |
||||
|
||||
#ifdef __cplusplus |
||||
} |
||||
#endif |
@ -1,5 +0,0 @@
@@ -1,5 +0,0 @@
|
||||
Makefile |
||||
CmakeCache.txt |
||||
cmake_install.cmake |
||||
CMakeFiles |
||||
libhashing.a |
@ -1,12 +0,0 @@
@@ -1,12 +0,0 @@
|
||||
set(LIB "hashing") |
||||
|
||||
set(CMAKE_C_FLAGS "${CMAKE_C_FLAGS} -std=c11 -D_GNU_SOURCE") |
||||
|
||||
include_directories("${MONERO_DIR}/contrib/epee/include") |
||||
include_directories("${MONERO_DIR}/src") |
||||
|
||||
link_directories(${MONERO_DIR}/src/crypto) |
||||
|
||||
add_library(${LIB} SHARED src/hashing.c) |
||||
|
||||
target_link_libraries(${LIB} cncrypto) |
@ -1,23 +0,0 @@
@@ -1,23 +0,0 @@
|
||||
package hashing |
||||
|
||||
// #cgo CFLAGS: -std=c11 -D_GNU_SOURCE
|
||||
// #cgo LDFLAGS: -L${SRCDIR} -lhashing -Wl,-rpath ${SRCDIR} -lstdc++
|
||||
// #include <stdlib.h>
|
||||
// #include <stdint.h>
|
||||
// #include "src/hashing.h"
|
||||
import "C" |
||||
import "unsafe" |
||||
|
||||
func Hash(blob []byte, fast bool) []byte { |
||||
output := make([]byte, 32) |
||||
if fast { |
||||
C.cryptonight_fast_hash((*C.char)(unsafe.Pointer(&blob[0])), (*C.char)(unsafe.Pointer(&output[0])), (C.uint32_t)(len(blob))) |
||||
} else { |
||||
C.cryptonight_hash((*C.char)(unsafe.Pointer(&blob[0])), (*C.char)(unsafe.Pointer(&output[0])), (C.uint32_t)(len(blob))) |
||||
} |
||||
return output |
||||
} |
||||
|
||||
func FastHash(blob []byte) []byte { |
||||
return Hash(append([]byte{byte(len(blob))}, blob...), true) |
||||
} |
@ -1,82 +0,0 @@
@@ -1,82 +0,0 @@
|
||||
package hashing |
||||
|
||||
import "testing" |
||||
import "log" |
||||
import "encoding/hex" |
||||
|
||||
func TestHash(t *testing.T) { |
||||
blob, _ := hex.DecodeString("01009091e4aa05ff5fe4801727ed0c1b8b339e1a0054d75568fec6ba9c4346e88b10d59edbf6858b2b00008a63b2865b65b84d28bb31feb057b16a21e2eda4bf6cc6377e3310af04debe4a01") |
||||
hashBytes := Hash(blob, false) |
||||
hash := hex.EncodeToString(hashBytes) |
||||
log.Println(hash) |
||||
|
||||
expectedHash := "a70a96f64a266f0f59e4f67c4a92f24fe8237c1349f377fd2720c9e1f2970400" |
||||
|
||||
if hash != expectedHash { |
||||
t.Error("Invalid hash") |
||||
} |
||||
} |
||||
|
||||
func TestHash_fast(t *testing.T) { |
||||
blob, _ := hex.DecodeString("01009091e4aa05ff5fe4801727ed0c1b8b339e1a0054d75568fec6ba9c4346e88b10d59edbf6858b2b00008a63b2865b65b84d28bb31feb057b16a21e2eda4bf6cc6377e3310af04debe4a01") |
||||
hashBytes := Hash(blob, true) |
||||
hash := hex.EncodeToString(hashBytes) |
||||
log.Println(hash) |
||||
|
||||
expectedHash := "7591f4d8ff9d86ea44873e89a5fb6f380f4410be6206030010567ac9d0d4b0e1" |
||||
|
||||
if hash != expectedHash { |
||||
t.Error("Invalid fast hash") |
||||
} |
||||
} |
||||
|
||||
func TestFastHash(t *testing.T) { |
||||
blob, _ := hex.DecodeString("01009091e4aa05ff5fe4801727ed0c1b8b339e1a0054d75568fec6ba9c4346e88b10d59edbf6858b2b00008a63b2865b65b84d28bb31feb057b16a21e2eda4bf6cc6377e3310af04debe4a01") |
||||
hashBytes := FastHash(blob) |
||||
hash := hex.EncodeToString(hashBytes) |
||||
log.Println(hash) |
||||
|
||||
expectedHash := "8706c697d9fc8a48b14ea93a31c6f0750c48683e585ec1a534e9c57c97193fa6" |
||||
|
||||
if hash != expectedHash { |
||||
t.Error("Invalid fast hash") |
||||
} |
||||
} |
||||
|
||||
func BenchmarkHash(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
for i := 0; i < b.N; i++ { |
||||
Hash(blob, false) |
||||
} |
||||
} |
||||
|
||||
func BenchmarkHashParallel(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
b.RunParallel(func(pb *testing.PB) { |
||||
for pb.Next() { |
||||
Hash(blob, false) |
||||
} |
||||
}) |
||||
} |
||||
|
||||
func BenchmarkHash_fast(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
for i := 0; i < b.N; i++ { |
||||
Hash(blob, true) |
||||
} |
||||
} |
||||
|
||||
func BenchmarkFastHash(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
for i := 0; i < b.N; i++ { |
||||
FastHash(blob) |
||||
} |
||||
} |
@ -1,10 +0,0 @@
@@ -1,10 +0,0 @@
|
||||
#include "crypto/hash-ops.h" |
||||
|
||||
void cryptonight_hash(const char* input, char* output, uint32_t len) { |
||||
const int variant = input[0] >= 7 ? input[0] - 6 : 0; |
||||
cn_slow_hash(input, len, output, variant, 0); |
||||
} |
||||
|
||||
void cryptonight_fast_hash(const char* input, char* output, uint32_t len) { |
||||
cn_fast_hash(input, len, output); |
||||
} |
@ -1,2 +0,0 @@
@@ -1,2 +0,0 @@
|
||||
void cryptonight_hash(const char* input, char* output, uint32_t len); |
||||
void cryptonight_fast_hash(const char* input, char* output, uint32_t len); |
Loading…
Reference in new issue