Browse Source
libtorrent allows setting a custom dispatch handler that is invoked in libtorrent thread when new alerts are incoming. QAlertDispatcher is a class that allows to translate these alerts to UI thread. The concept is very simple: 1. On initialization QAlertDispatcher constructor calls set_alert_dispatch() passing QAlertDispatcher::dispatch as argument. 2. On deinitialization destructor calls set_alert_dispatch() passing a empty function. (line 25) libtorrent handles thos and switches back to queuing alerts in queue. 3. QAlertDispatcher::dispatch() adds alert to queue and notifies UI thread that new alerts are incoming. Enqueuing is done in function enqueueToMainThread(). The invariant of class is the following: if alert queue is not empty, in message loop of UI thread contains a queued invocation of deliverSignal(). 4. When message loop is pumped UI thread execute deliverSignal() function. It emit appropriate signal and if queue is still not empty (for example if slot doesn't grab alerts) rewind enqueuing to main thread. This is a idea. But here is some details. 1. When QAlertDispatcher is destoyed, libtorrent still can call QAlertDispatcher::dispatch a few times after destruction. This is handled by passing a "tag". A tag is a object that references QAlertDispatch. Tag could be invalidated. So on destruction QAlertDispatcher invalidates a tag and then unsubscribes from alerts. When QAlertDispatcher::dispatch is called with invalid tag it simply discard an alert. Therefore we could drop a few alerts during unsubscription. So we unsubscribe only at exit when missing some alerts is not a problem. 2. Another problem is in QBtSession::saveFastResumeData(). It pumps alert queue synchronously. My first attempt was to destroy QAlertDispatcher and then pump libtorrent queue. But as I was afraid of losing alerts I supported synchronous querying of alerts in QAlertDispatcher. (QAlertDispatcher::getPendingAlerts) Conflicts: src/qtlibtorrent/qbtsession.cppadaptive-webui-19844
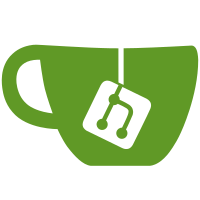
5 changed files with 590 additions and 445 deletions
@ -0,0 +1,93 @@
@@ -0,0 +1,93 @@
|
||||
#include "alertdispatcher.h" |
||||
|
||||
#include <boost/bind.hpp> |
||||
#include <boost/make_shared.hpp> |
||||
#include <QMutexLocker> |
||||
|
||||
QAlertDispatcher::QAlertDispatcher(libtorrent::session *session, QObject* parent) |
||||
: QObject(parent) |
||||
, session(session) |
||||
, current_tag(new QAtomicPointer<QAlertDispatcher>(this)) |
||||
, event_posted(false) { |
||||
session->set_alert_dispatch(boost::bind(&QAlertDispatcher::dispatch, current_tag, _1)); |
||||
} |
||||
|
||||
QAlertDispatcher::~QAlertDispatcher() { |
||||
// When QAlertDispatcher is destoyed, libtorrent still can call
|
||||
// QAlertDispatcher::dispatch a few times after destruction. This is
|
||||
// handled by passing a "tag". A tag is a object that references QAlertDispatch.
|
||||
// Tag could be invalidated. So on destruction QAlertDispatcher invalidates a tag
|
||||
// and then unsubscribes from alerts. When QAlertDispatcher::dispatch is called
|
||||
// with invalid tag it simply discard an alert.
|
||||
|
||||
{ |
||||
QMutexLocker lock(&(alerts_mutex)); |
||||
*current_tag = 0; |
||||
current_tag.clear(); |
||||
} |
||||
|
||||
typedef boost::function<void (std::auto_ptr<libtorrent::alert>)> dispatch_function_t; |
||||
session->set_alert_dispatch(dispatch_function_t()); |
||||
} |
||||
|
||||
void QAlertDispatcher::getPendingAlertsNoWait(std::deque<libtorrent::alert*>& out) { |
||||
Q_ASSERT(out.empty()); |
||||
|
||||
QMutexLocker lock(&(alerts_mutex)); |
||||
std::swap(alerts, out); |
||||
event_posted = false; |
||||
} |
||||
|
||||
void QAlertDispatcher::getPendingAlerts(std::deque<libtorrent::alert*>& out) { |
||||
assert(out.empty()); |
||||
|
||||
QMutexLocker lock(&(alerts_mutex)); |
||||
|
||||
while (alerts.empty()) |
||||
alerts_condvar.wait(&(alerts_mutex)); |
||||
|
||||
std::swap(alerts, out); |
||||
event_posted = false; |
||||
} |
||||
|
||||
void QAlertDispatcher::dispatch(QSharedPointer<QAtomicPointer<QAlertDispatcher> > tag, |
||||
std::auto_ptr<libtorrent::alert> alert_ptr) { |
||||
QAlertDispatcher* that = *tag; |
||||
if (!that) |
||||
return; |
||||
|
||||
QMutexLocker lock(&(that->alerts_mutex)); |
||||
|
||||
that = *tag; |
||||
if (!that) |
||||
return; |
||||
|
||||
bool was_empty = that->alerts.empty(); |
||||
|
||||
that->alerts.push_back(alert_ptr.get()); |
||||
alert_ptr.release(); |
||||
|
||||
if (was_empty) |
||||
that->alerts_condvar.wakeAll(); |
||||
|
||||
that->enqueueToMainThread(); |
||||
|
||||
Q_ASSERT(that->current_tag == tag); |
||||
} |
||||
|
||||
void QAlertDispatcher::enqueueToMainThread() { |
||||
if (!event_posted) { |
||||
event_posted = true; |
||||
QMetaObject::invokeMethod(this, "deliverSignal", Qt::QueuedConnection); |
||||
} |
||||
} |
||||
|
||||
void QAlertDispatcher::deliverSignal() { |
||||
emit alertsReceived(); |
||||
|
||||
QMutexLocker lock(&(alerts_mutex)); |
||||
event_posted = false; |
||||
|
||||
if (!alerts.empty()) |
||||
enqueueToMainThread(); |
||||
} |
@ -0,0 +1,43 @@
@@ -0,0 +1,43 @@
|
||||
#ifndef ALERTDISPATCHER_H |
||||
#define ALERTDISPATCHER_H |
||||
|
||||
#include <QObject> |
||||
#include <QMutex> |
||||
#include <QWaitCondition> |
||||
#include <QAtomicPointer> |
||||
#include <QSharedPointer> |
||||
#include <libtorrent/session.hpp> |
||||
|
||||
class QAlertDispatcher : public QObject |
||||
{ |
||||
Q_OBJECT |
||||
Q_DISABLE_COPY(QAlertDispatcher) |
||||
|
||||
public: |
||||
QAlertDispatcher(libtorrent::session *session, QObject* parent); |
||||
~QAlertDispatcher(); |
||||
|
||||
void getPendingAlertsNoWait(std::deque<libtorrent::alert*>&); |
||||
void getPendingAlerts(std::deque<libtorrent::alert*>&); |
||||
|
||||
signals: |
||||
void alertsReceived(); |
||||
|
||||
private: |
||||
static void dispatch(QSharedPointer<QAtomicPointer<QAlertDispatcher> >, |
||||
std::auto_ptr<libtorrent::alert>); |
||||
void enqueueToMainThread(); |
||||
|
||||
private slots: |
||||
void deliverSignal(); |
||||
|
||||
private: |
||||
libtorrent::session *session; |
||||
QMutex alerts_mutex; |
||||
QWaitCondition alerts_condvar; |
||||
std::deque<libtorrent::alert*> alerts; |
||||
QSharedPointer<QAtomicPointer<QAlertDispatcher> > current_tag; |
||||
bool event_posted; |
||||
}; |
||||
|
||||
#endif // ALERTDISPATCHER_H
|
Loading…
Reference in new issue