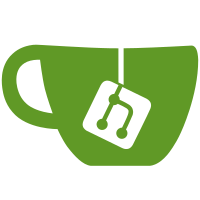
15 changed files with 278 additions and 335 deletions
After Width: | Height: | Size: 1.5 KiB |
After Width: | Height: | Size: 1.6 KiB |
After Width: | Height: | Size: 1.4 KiB |
After Width: | Height: | Size: 1.5 KiB |
@ -1,92 +0,0 @@ |
|||||||
/* |
|
||||||
* MIT License |
|
||||||
* Copyright (c) 2008 Christophe Dumez <chris@qbittorrent.org> |
|
||||||
* |
|
||||||
* Permission is hereby granted, free of charge, to any person obtaining a copy |
|
||||||
* of this software and associated documentation files (the "Software"), to deal |
|
||||||
* in the Software without restriction, including without limitation the rights |
|
||||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
|
||||||
* copies of the Software, and to permit persons to whom the Software is |
|
||||||
* furnished to do so, subject to the following conditions: |
|
||||||
* |
|
||||||
* The above copyright notice and this permission notice shall be included in |
|
||||||
* all copies or substantial portions of the Software. |
|
||||||
* |
|
||||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
||||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|
||||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
|
||||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|
||||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
|
||||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN |
|
||||||
* THE SOFTWARE. |
|
||||||
*/ |
|
||||||
|
|
||||||
/* |
|
||||||
* Original code from http://www.silverscripting.com/mootabs/ |
|
||||||
* Edited by Christophe Dumez |
|
||||||
*/ |
|
||||||
|
|
||||||
.toolbarTabs { |
|
||||||
padding: 0 5px 2px 2px; |
|
||||||
background: url(../images/skin/tabs.gif) repeat-x; |
|
||||||
background-position: left -70px; |
|
||||||
overflow: visible; |
|
||||||
} |
|
||||||
|
|
||||||
.mootabs_title { |
|
||||||
padding-top: 1px; |
|
||||||
list-style: none; |
|
||||||
margin: 0; |
|
||||||
padding: 0; |
|
||||||
line-height: 16px; |
|
||||||
font-size: 11px; |
|
||||||
} |
|
||||||
|
|
||||||
.mootabs_title li { |
|
||||||
display: block; |
|
||||||
float: left; |
|
||||||
margin: 0 0 5px 0; |
|
||||||
cursor: pointer; |
|
||||||
background: url(../images/skin/tabs.gif) repeat-x; |
|
||||||
background-position: left -35px; |
|
||||||
|
|
||||||
} |
|
||||||
|
|
||||||
.mootabs_title li.active { |
|
||||||
background: url(../images/skin/tabs.gif) repeat-x; |
|
||||||
background-position: left 0; |
|
||||||
} |
|
||||||
|
|
||||||
.mootabs_title li a { |
|
||||||
display: block; |
|
||||||
margin-left: 8px; |
|
||||||
padding: 6px 16px 5px 10px; |
|
||||||
text-align: center; |
|
||||||
font-weight: normal; |
|
||||||
color: #141414; |
|
||||||
background: url(../images/skin/tabs.gif) repeat-x; |
|
||||||
background-position: right -35px; |
|
||||||
} |
|
||||||
|
|
||||||
.mootabs_title li.active a { |
|
||||||
color: #141414; |
|
||||||
font-weight: bold; |
|
||||||
background: url(../images/skin/tabs.gif) repeat-x; |
|
||||||
background-position: right 0; |
|
||||||
} |
|
||||||
|
|
||||||
.mootabs_panel { |
|
||||||
display: none; |
|
||||||
background-color: #ddd; |
|
||||||
position: relative; |
|
||||||
width: 100%; |
|
||||||
top: -1px; |
|
||||||
clear: both; |
|
||||||
overflow: auto; |
|
||||||
|
|
||||||
} |
|
||||||
|
|
||||||
.mootabs_panel.active { |
|
||||||
background-color: #e6e6e6; |
|
||||||
display: block; |
|
||||||
} |
|
@ -0,0 +1,155 @@ |
|||||||
|
var ContextMenu = new Class({ |
||||||
|
|
||||||
|
//implements
|
||||||
|
Implements: [Options,Events], |
||||||
|
|
||||||
|
//options
|
||||||
|
options: { |
||||||
|
actions: {}, |
||||||
|
menu: 'contextmenu', |
||||||
|
stopEvent: true, |
||||||
|
targets: 'body', |
||||||
|
trigger: 'contextmenu', |
||||||
|
offsets: { x:0, y:0 }, |
||||||
|
onShow: $empty, |
||||||
|
onHide: $empty, |
||||||
|
onClick: $empty, |
||||||
|
fadeSpeed: 200 |
||||||
|
}, |
||||||
|
|
||||||
|
//initialization
|
||||||
|
initialize: function(options) { |
||||||
|
//set options
|
||||||
|
this.setOptions(options) |
||||||
|
|
||||||
|
//option diffs menu
|
||||||
|
this.menu = $(this.options.menu); |
||||||
|
this.targets = $$(this.options.targets); |
||||||
|
|
||||||
|
//fx
|
||||||
|
this.fx = new Fx.Tween(this.menu, { property: 'opacity', duration:this.options.fadeSpeed }); |
||||||
|
|
||||||
|
//hide and begin the listener
|
||||||
|
this.hide().startListener(); |
||||||
|
|
||||||
|
//hide the menu
|
||||||
|
this.menu.setStyles({ 'position':'absolute','top':'-900000px', 'display':'block' }); |
||||||
|
}, |
||||||
|
|
||||||
|
addTarget: function(t) { |
||||||
|
this.targets[this.targets.length] = t; |
||||||
|
t.addEvent(this.options.trigger,function(e) { |
||||||
|
//enabled?
|
||||||
|
if(!this.options.disabled) { |
||||||
|
//prevent default, if told to
|
||||||
|
if(this.options.stopEvent) { e.stop(); } |
||||||
|
//record this as the trigger
|
||||||
|
this.options.element = $(t); |
||||||
|
//position the menu
|
||||||
|
this.menu.setStyles({ |
||||||
|
top: (e.page.y + this.options.offsets.y), |
||||||
|
left: (e.page.x + this.options.offsets.x), |
||||||
|
position: 'absolute', |
||||||
|
'z-index': '2000' |
||||||
|
}); |
||||||
|
//show the menu
|
||||||
|
this.show(); |
||||||
|
} |
||||||
|
}.bind(this)); |
||||||
|
}, |
||||||
|
|
||||||
|
//get things started
|
||||||
|
startListener: function() { |
||||||
|
/* all elements */ |
||||||
|
this.targets.each(function(el) { |
||||||
|
/* show the menu */ |
||||||
|
el.addEvent(this.options.trigger,function(e) { |
||||||
|
//enabled?
|
||||||
|
if(!this.options.disabled) { |
||||||
|
//prevent default, if told to
|
||||||
|
if(this.options.stopEvent) { e.stop(); } |
||||||
|
//record this as the trigger
|
||||||
|
this.options.element = $(el); |
||||||
|
//position the menu
|
||||||
|
this.menu.setStyles({ |
||||||
|
top: (e.page.y + this.options.offsets.y), |
||||||
|
left: (e.page.x + this.options.offsets.x), |
||||||
|
position: 'absolute', |
||||||
|
'z-index': '2000' |
||||||
|
}); |
||||||
|
//show the menu
|
||||||
|
this.show(); |
||||||
|
} |
||||||
|
}.bind(this)); |
||||||
|
},this); |
||||||
|
|
||||||
|
/* menu items */ |
||||||
|
this.menu.getElements('a').each(function(item) { |
||||||
|
item.addEvent('click',function(e) { |
||||||
|
if(!item.hasClass('disabled')) { |
||||||
|
this.execute(item.get('href').split('#')[1],$(this.options.element)); |
||||||
|
this.fireEvent('click',[item,e]); |
||||||
|
} |
||||||
|
}.bind(this)); |
||||||
|
},this); |
||||||
|
|
||||||
|
//hide on body click
|
||||||
|
$(document.body).addEvent('click', function() { |
||||||
|
this.hide(); |
||||||
|
}.bind(this)); |
||||||
|
}, |
||||||
|
|
||||||
|
//show menu
|
||||||
|
show: function(trigger) { |
||||||
|
//this.menu.fade('in');
|
||||||
|
this.fx.start(1); |
||||||
|
this.fireEvent('show'); |
||||||
|
this.shown = true; |
||||||
|
return this; |
||||||
|
}, |
||||||
|
|
||||||
|
//hide the menu
|
||||||
|
hide: function(trigger) { |
||||||
|
if(this.shown) |
||||||
|
{ |
||||||
|
this.fx.start(0); |
||||||
|
//this.menu.fade('out');
|
||||||
|
this.fireEvent('hide'); |
||||||
|
this.shown = false; |
||||||
|
} |
||||||
|
return this; |
||||||
|
}, |
||||||
|
|
||||||
|
//disable an item
|
||||||
|
disableItem: function(item) { |
||||||
|
this.menu.getElements('a[href$=' + item + ']').addClass('disabled'); |
||||||
|
return this; |
||||||
|
}, |
||||||
|
|
||||||
|
//enable an item
|
||||||
|
enableItem: function(item) { |
||||||
|
this.menu.getElements('a[href$=' + item + ']').removeClass('disabled'); |
||||||
|
return this; |
||||||
|
}, |
||||||
|
|
||||||
|
//diable the entire menu
|
||||||
|
disable: function() { |
||||||
|
this.options.disabled = true; |
||||||
|
return this; |
||||||
|
}, |
||||||
|
|
||||||
|
//enable the entire menu
|
||||||
|
enable: function() { |
||||||
|
this.options.disabled = false; |
||||||
|
return this; |
||||||
|
}, |
||||||
|
|
||||||
|
//execute an action
|
||||||
|
execute: function(action,element) { |
||||||
|
if(this.options.actions[action]) { |
||||||
|
this.options.actions[action](element,this); |
||||||
|
} |
||||||
|
return this; |
||||||
|
} |
||||||
|
|
||||||
|
}); |
@ -1,223 +0,0 @@ |
|||||||
/* |
|
||||||
* MIT License |
|
||||||
* Copyright (c) 2008 Christophe Dumez <chris@qbittorrent.org> |
|
||||||
* |
|
||||||
* Permission is hereby granted, free of charge, to any person obtaining a copy |
|
||||||
* of this software and associated documentation files (the "Software"), to deal |
|
||||||
* in the Software without restriction, including without limitation the rights |
|
||||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
|
||||||
* copies of the Software, and to permit persons to whom the Software is |
|
||||||
* furnished to do so, subject to the following conditions: |
|
||||||
* |
|
||||||
* The above copyright notice and this permission notice shall be included in |
|
||||||
* all copies or substantial portions of the Software. |
|
||||||
* |
|
||||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
||||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|
||||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
|
||||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|
||||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
|
||||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN |
|
||||||
* THE SOFTWARE. |
|
||||||
*/ |
|
||||||
|
|
||||||
/* |
|
||||||
* Original code from http://www.silverscripting.com/mootabs/
|
|
||||||
* Ported to Mootools 1.2 by Christophe Dumez |
|
||||||
*/ |
|
||||||
|
|
||||||
var mootabs = new Class({ |
|
||||||
|
|
||||||
Implements: [Options], |
|
||||||
|
|
||||||
options: { |
|
||||||
width: '300px', |
|
||||||
height: '200px', |
|
||||||
changeTransition: Fx.Transitions.Bounce.easeOut, |
|
||||||
duration: 1000, |
|
||||||
mouseOverClass: 'over', |
|
||||||
activateOnLoad: 'first', |
|
||||||
useAjax: false, |
|
||||||
ajaxUrl: '', |
|
||||||
ajaxOptions: {method:'get', evalScripts: true}, |
|
||||||
ajaxLoadingText: 'Loading...' |
|
||||||
}, |
|
||||||
|
|
||||||
initialize: function(element, options) { |
|
||||||
|
|
||||||
if(options) this.setOptions(options); |
|
||||||
|
|
||||||
this.el = $(element); |
|
||||||
this.elid = element; |
|
||||||
|
|
||||||
this.el.setStyles({ |
|
||||||
height: this.options.height, |
|
||||||
width: this.options.width |
|
||||||
}); |
|
||||||
|
|
||||||
this.titles = $$('#' + this.elid + ' ul li'); |
|
||||||
this.panelHeight = this.el.getSize().y - (this.titles[0].getSize().y + 4); |
|
||||||
this.panels = $$('#' + this.elid + ' .mootabs_panel'); |
|
||||||
|
|
||||||
|
|
||||||
this.panels.setStyle('height', this.panelHeight); |
|
||||||
|
|
||||||
this.titles.each(function(item) { |
|
||||||
item.addEvent('click', function(){ |
|
||||||
if(item != this.activeTitle) |
|
||||||
{ |
|
||||||
item.removeClass(this.options.mouseOverClass); |
|
||||||
this.activate(item); |
|
||||||
} |
|
||||||
|
|
||||||
}.bind(this)); |
|
||||||
|
|
||||||
item.addEvent('mouseover', function() { |
|
||||||
if(item != this.activeTitle) |
|
||||||
{ |
|
||||||
item.addClass(this.options.mouseOverClass); |
|
||||||
} |
|
||||||
}.bind(this)); |
|
||||||
|
|
||||||
item.addEvent('mouseout', function() { |
|
||||||
if(item != this.activeTitle) |
|
||||||
{ |
|
||||||
item.removeClass(this.options.mouseOverClass); |
|
||||||
} |
|
||||||
}.bind(this)); |
|
||||||
}.bind(this)); |
|
||||||
|
|
||||||
|
|
||||||
if(this.options.activateOnLoad != 'none') |
|
||||||
{ |
|
||||||
if(this.options.activateOnLoad == 'first') |
|
||||||
{ |
|
||||||
this.activate(this.titles[0], true); |
|
||||||
} |
|
||||||
else |
|
||||||
{ |
|
||||||
this.activate(this.options.activateOnLoad, true); |
|
||||||
} |
|
||||||
} |
|
||||||
}, |
|
||||||
|
|
||||||
activate: function(tab, skipAnim){ |
|
||||||
if(! $defined(skipAnim)) |
|
||||||
{ |
|
||||||
skipAnim = false; |
|
||||||
} |
|
||||||
if($type(tab) == 'string') |
|
||||||
{ |
|
||||||
myTab = $$('#' + this.elid + ' ul li').filter('[title=' + tab + ']')[0]; |
|
||||||
tab = myTab; |
|
||||||
} |
|
||||||
|
|
||||||
if($type(tab) == 'element') |
|
||||||
{ |
|
||||||
var newTab = tab.getProperty('title'); |
|
||||||
|
|
||||||
this.panels.removeClass('active'); |
|
||||||
|
|
||||||
this.activePanel = this.panels.filter('#' + newTab)[0]; |
|
||||||
|
|
||||||
this.activePanel.addClass('active'); |
|
||||||
|
|
||||||
if(this.options.changeTransition != 'none' && skipAnim==false) |
|
||||||
{ |
|
||||||
this.panels.filter('#' + newTab).setStyle('height', 0); |
|
||||||
var changeEffect = new Fx.Elements(this.panels.filter('#' + newTab), {duration: this.options.duration, transition: this.options.changeTransition}); |
|
||||||
changeEffect.start({ |
|
||||||
'0': { |
|
||||||
'height': [0, this.panelHeight] |
|
||||||
} |
|
||||||
}); |
|
||||||
} |
|
||||||
|
|
||||||
this.titles.removeClass('active'); |
|
||||||
|
|
||||||
tab.addClass('active'); |
|
||||||
|
|
||||||
this.activeTitle = tab; |
|
||||||
|
|
||||||
if(this.options.useAjax) |
|
||||||
{ |
|
||||||
this._getContent(); |
|
||||||
} |
|
||||||
} |
|
||||||
}, |
|
||||||
|
|
||||||
_getContent: function(){ |
|
||||||
this.activePanel.setHTML(this.options.ajaxLoadingText); |
|
||||||
var newOptions = { |
|
||||||
url: this.options.ajaxUrl + '?tab=' + this.activeTitle.getProperty('title'), |
|
||||||
update: this.activePanel.getProperty('id') |
|
||||||
}; |
|
||||||
this.options.ajaxOptions = $merge(this.options.ajaxOptions, newOptions); |
|
||||||
var tabRequest = new Request.HTML(this.options.ajaxOptions); |
|
||||||
tabRequest.send(); |
|
||||||
}, |
|
||||||
|
|
||||||
addTab: function(title, label, content){ |
|
||||||
//the new title
|
|
||||||
var newTitle = new Element('li', { |
|
||||||
'title': title |
|
||||||
}); |
|
||||||
newTitle.appendText(label); |
|
||||||
this.titles.include(newTitle); |
|
||||||
$$('#' + this.elid + ' ul').adopt(newTitle); |
|
||||||
newTitle.addEvent('click', function() { |
|
||||||
this.activate(newTitle); |
|
||||||
}.bind(this)); |
|
||||||
|
|
||||||
newTitle.addEvent('mouseover', function() { |
|
||||||
if(newTitle != this.activeTitle) |
|
||||||
{ |
|
||||||
newTitle.addClass(this.options.mouseOverClass); |
|
||||||
} |
|
||||||
}.bind(this)); |
|
||||||
newTitle.addEvent('mouseout', function() { |
|
||||||
if(newTitle != this.activeTitle) |
|
||||||
{ |
|
||||||
newTitle.removeClass(this.options.mouseOverClass); |
|
||||||
} |
|
||||||
}.bind(this)); |
|
||||||
//the new panel
|
|
||||||
var newPanel = new Element('div', { |
|
||||||
'style': {'height': this.options.panelHeight}, |
|
||||||
'id': title, |
|
||||||
'class': 'mootabs_panel' |
|
||||||
}); |
|
||||||
if(!this.options.useAjax) |
|
||||||
{ |
|
||||||
newPanel.setHTML(content); |
|
||||||
} |
|
||||||
this.panels.include(newPanel); |
|
||||||
this.el.adopt(newPanel); |
|
||||||
}, |
|
||||||
|
|
||||||
removeTab: function(title){ |
|
||||||
if(this.activeTitle.title == title) |
|
||||||
{ |
|
||||||
this.activate(this.titles[0]); |
|
||||||
} |
|
||||||
$$('#' + this.elid + ' ul li').filter('[title=' + title + ']')[0].remove(); |
|
||||||
|
|
||||||
$$('#' + this.elid + ' .mootabs_panel').filter('#' + title)[0].remove(); |
|
||||||
}, |
|
||||||
|
|
||||||
next: function(){ |
|
||||||
var nextTab = this.activeTitle.getNext(); |
|
||||||
if(!nextTab) { |
|
||||||
nextTab = this.titles[0]; |
|
||||||
} |
|
||||||
this.activate(nextTab); |
|
||||||
}, |
|
||||||
|
|
||||||
previous: function(){ |
|
||||||
var previousTab = this.activeTitle.getPrevious(); |
|
||||||
if(!previousTab) { |
|
||||||
previousTab = this.titles[this.titles.length - 1]; |
|
||||||
} |
|
||||||
this.activate(previousTab); |
|
||||||
} |
|
||||||
}); |
|
Loading…
Reference in new issue