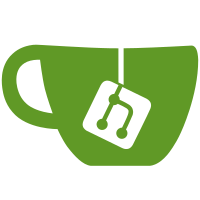
7 changed files with 212 additions and 218 deletions
@ -1,109 +0,0 @@
@@ -1,109 +0,0 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?> |
||||
<ui version="4.0"> |
||||
<class>addPeerDialog</class> |
||||
<widget class="QDialog" name="addPeerDialog"> |
||||
<property name="geometry"> |
||||
<rect> |
||||
<x>0</x> |
||||
<y>0</y> |
||||
<width>400</width> |
||||
<height>112</height> |
||||
</rect> |
||||
</property> |
||||
<property name="sizePolicy"> |
||||
<sizepolicy hsizetype="Fixed" vsizetype="Fixed"> |
||||
<horstretch>0</horstretch> |
||||
<verstretch>0</verstretch> |
||||
</sizepolicy> |
||||
</property> |
||||
<property name="windowTitle"> |
||||
<string>Peer addition</string> |
||||
</property> |
||||
<layout class="QVBoxLayout" name="verticalLayout_3"> |
||||
<item> |
||||
<layout class="QHBoxLayout" name="horizontalLayout"> |
||||
<item> |
||||
<layout class="QVBoxLayout" name="verticalLayout"> |
||||
<item> |
||||
<widget class="QLabel" name="label"> |
||||
<property name="font"> |
||||
<font> |
||||
<weight>75</weight> |
||||
<bold>true</bold> |
||||
</font> |
||||
</property> |
||||
<property name="text"> |
||||
<string>IP</string> |
||||
</property> |
||||
<property name="alignment"> |
||||
<set>Qt::AlignCenter</set> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QLineEdit" name="lineIP"/> |
||||
</item> |
||||
</layout> |
||||
</item> |
||||
<item> |
||||
<layout class="QVBoxLayout" name="verticalLayout_2"> |
||||
<item> |
||||
<widget class="QLabel" name="label_2"> |
||||
<property name="font"> |
||||
<font> |
||||
<weight>75</weight> |
||||
<bold>true</bold> |
||||
</font> |
||||
</property> |
||||
<property name="text"> |
||||
<string>Port</string> |
||||
</property> |
||||
<property name="alignment"> |
||||
<set>Qt::AlignCenter</set> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QSpinBox" name="spinPort"> |
||||
<property name="minimumSize"> |
||||
<size> |
||||
<width>60</width> |
||||
<height>0</height> |
||||
</size> |
||||
</property> |
||||
<property name="maximumSize"> |
||||
<size> |
||||
<width>70</width> |
||||
<height>16777215</height> |
||||
</size> |
||||
</property> |
||||
<property name="minimum"> |
||||
<number>1000</number> |
||||
</property> |
||||
<property name="maximum"> |
||||
<number>65535</number> |
||||
</property> |
||||
<property name="value"> |
||||
<number>6881</number> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
</layout> |
||||
</item> |
||||
</layout> |
||||
</item> |
||||
<item> |
||||
<widget class="QDialogButtonBox" name="buttonBox"> |
||||
<property name="orientation"> |
||||
<enum>Qt::Horizontal</enum> |
||||
</property> |
||||
<property name="standardButtons"> |
||||
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
</layout> |
||||
</widget> |
||||
<resources/> |
||||
<connections/> |
||||
</ui> |
@ -1,81 +0,0 @@
@@ -1,81 +0,0 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt and libtorrent. |
||||
* Copyright (C) 2006 Christophe Dumez |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
* |
||||
* Contact : chris@qbittorrent.org |
||||
*/ |
||||
|
||||
#include <QRegExp> |
||||
#include <QMessageBox> |
||||
|
||||
#include "core/bittorrent/peerinfo.h" |
||||
#include "peeraddition.h" |
||||
|
||||
PeerAdditionDlg::PeerAdditionDlg(QWidget *parent) |
||||
: QDialog(parent) |
||||
{ |
||||
setupUi(this); |
||||
connect(buttonBox, SIGNAL(rejected()), this, SLOT(reject())); |
||||
connect(buttonBox, SIGNAL(accepted()), this, SLOT(validateInput())); |
||||
} |
||||
|
||||
QHostAddress PeerAdditionDlg::getAddress() const |
||||
{ |
||||
return QHostAddress(lineIP->text()); |
||||
} |
||||
|
||||
|
||||
ushort PeerAdditionDlg::getPort() const |
||||
{ |
||||
return spinPort->value(); |
||||
} |
||||
|
||||
|
||||
BitTorrent::PeerAddress PeerAdditionDlg::askForPeerAddress() |
||||
{ |
||||
BitTorrent::PeerAddress addr; |
||||
|
||||
PeerAdditionDlg dlg; |
||||
if (dlg.exec() == QDialog::Accepted) { |
||||
addr.ip = dlg.getAddress(); |
||||
if (addr.ip.isNull()) |
||||
qDebug("Unable to parse the provided IP."); |
||||
else |
||||
qDebug("Provided IP is correct"); |
||||
addr.port = dlg.getPort(); |
||||
} |
||||
|
||||
return addr; |
||||
} |
||||
|
||||
|
||||
void PeerAdditionDlg::validateInput() |
||||
{ |
||||
if (getAddress().isNull()) |
||||
QMessageBox::warning(this, tr("Invalid IP"), tr("The IP you provided is invalid."), QMessageBox::Ok); |
||||
else |
||||
accept(); |
||||
} |
@ -0,0 +1,103 @@
@@ -0,0 +1,103 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt4 and libtorrent. |
||||
* Copyright (C) 2006 Christophe Dumez |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
* |
||||
* Contact : chris@qbittorrent.org |
||||
*/ |
||||
|
||||
#include <QMessageBox> |
||||
#include <QHostAddress> |
||||
|
||||
#include "peersadditiondlg.h" |
||||
|
||||
PeersAdditionDlg::PeersAdditionDlg(QWidget *parent) |
||||
: QDialog(parent) |
||||
{ |
||||
setupUi(this); |
||||
connect(buttonBox, SIGNAL(accepted()), this, SLOT(validateInput())); |
||||
|
||||
#if (QT_VERSION >= QT_VERSION_CHECK(5, 0, 0)) |
||||
label_format->hide(); |
||||
peers_txt->setPlaceholderText("Format: IPv4:port / [IPv6]:port"); |
||||
#endif |
||||
} |
||||
|
||||
QList<BitTorrent::PeerAddress> PeersAdditionDlg::askForPeers() |
||||
{ |
||||
PeersAdditionDlg dlg; |
||||
dlg.exec(); |
||||
return dlg.m_peersList; |
||||
} |
||||
|
||||
void PeersAdditionDlg::validateInput() |
||||
{ |
||||
if (peers_txt->toPlainText().trimmed().isEmpty()) { |
||||
QMessageBox::warning(this, tr("No peer entered"), |
||||
tr("Please type at least one peer."), |
||||
QMessageBox::Ok); |
||||
return; |
||||
} |
||||
foreach (const QString &peer, peers_txt->toPlainText().trimmed().split("\n")) { |
||||
BitTorrent::PeerAddress addr = parsePeer(peer); |
||||
if (!addr.ip.isNull()) { |
||||
m_peersList.append(addr); |
||||
} |
||||
else { |
||||
QMessageBox::warning(this, tr("Invalid peer"), |
||||
tr("The peer %1 is invalid.").arg(peer), |
||||
QMessageBox::Ok); |
||||
m_peersList.clear(); |
||||
return; |
||||
} |
||||
} |
||||
accept(); |
||||
} |
||||
|
||||
BitTorrent::PeerAddress PeersAdditionDlg::parsePeer(QString peer) |
||||
{ |
||||
BitTorrent::PeerAddress addr; |
||||
QStringList ipPort; |
||||
|
||||
if (peer[0] == '[' && peer.indexOf("]:") != -1) // IPv6
|
||||
ipPort = peer.remove(QChar('[')).split("]:"); |
||||
else if (peer.indexOf(":") != -1) // IPv4
|
||||
ipPort = peer.split(":"); |
||||
else |
||||
return addr; |
||||
|
||||
QHostAddress ip(ipPort[0]); |
||||
if (ip.isNull()) |
||||
return addr; |
||||
|
||||
bool ok; |
||||
int port = ipPort[1].toInt(&ok); |
||||
if (!ok || port < 1 || port > 65535) |
||||
return addr; |
||||
|
||||
addr.ip = ip; |
||||
addr.port = port; |
||||
return addr; |
||||
} |
@ -0,0 +1,75 @@
@@ -0,0 +1,75 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?> |
||||
<ui version="4.0"> |
||||
<class>addPeersDialog</class> |
||||
<widget class="QDialog" name="addPeersDialog"> |
||||
<property name="geometry"> |
||||
<rect> |
||||
<x>0</x> |
||||
<y>0</y> |
||||
<width>367</width> |
||||
<height>274</height> |
||||
</rect> |
||||
</property> |
||||
<property name="windowTitle"> |
||||
<string>Add Peers</string> |
||||
</property> |
||||
<layout class="QVBoxLayout" name="verticalLayout"> |
||||
<item> |
||||
<widget class="QLabel" name="label"> |
||||
<property name="text"> |
||||
<string>List of peers to add (one per line):</string> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QTextEdit" name="peers_txt"> |
||||
<property name="lineWrapMode"> |
||||
<enum>QTextEdit::NoWrap</enum> |
||||
</property> |
||||
<property name="acceptRichText"> |
||||
<bool>false</bool> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QLabel" name="label_format"> |
||||
<property name="text"> |
||||
<string>Format: IPv4:port / [IPv6]:port</string> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QDialogButtonBox" name="buttonBox"> |
||||
<property name="enabled"> |
||||
<bool>true</bool> |
||||
</property> |
||||
<property name="orientation"> |
||||
<enum>Qt::Horizontal</enum> |
||||
</property> |
||||
<property name="standardButtons"> |
||||
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
</layout> |
||||
</widget> |
||||
<resources/> |
||||
<connections> |
||||
<connection> |
||||
<sender>buttonBox</sender> |
||||
<signal>rejected()</signal> |
||||
<receiver>addPeersDialog</receiver> |
||||
<slot>reject()</slot> |
||||
<hints> |
||||
<hint type="sourcelabel"> |
||||
<x>183</x> |
||||
<y>251</y> |
||||
</hint> |
||||
<hint type="destinationlabel"> |
||||
<x>183</x> |
||||
<y>136</y> |
||||
</hint> |
||||
</hints> |
||||
</connection> |
||||
</connections> |
||||
</ui> |
Loading…
Reference in new issue