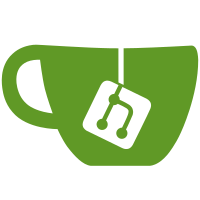
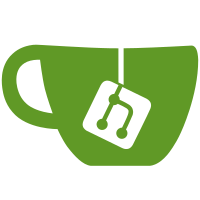
13 changed files with 8 additions and 288 deletions
Before Width: | Height: | Size: 400 B |
@ -1,64 +0,0 @@
@@ -1,64 +0,0 @@
|
||||
#VERSION: 1.32 |
||||
#AUTHORS: BTDigg team (research@btdigg.org) |
||||
# Contributors: Diego de las Heras (ngosang@hotmail.es) |
||||
|
||||
# GNU GENERAL PUBLIC LICENSE |
||||
# Version 3, 29 June 2007 |
||||
# |
||||
# <http://www.gnu.org/licenses/> |
||||
# |
||||
# This program is free software: you can redistribute it and/or modify |
||||
# it under the terms of the GNU General Public License as published by |
||||
# the Free Software Foundation, either version 3 of the License, or |
||||
# (at your option) any later version. |
||||
# |
||||
# This program is distributed in the hope that it will be useful, |
||||
# but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
# GNU General Public License for more details. |
||||
|
||||
from novaprinter import prettyPrinter |
||||
from helpers import retrieve_url, download_file |
||||
import urllib |
||||
import sys |
||||
|
||||
class btdigg(object): |
||||
url = 'https://btdigg.org' |
||||
name = 'BTDigg' |
||||
|
||||
supported_categories = {'all': ''} |
||||
|
||||
def __init__(self): |
||||
pass |
||||
|
||||
def search(self, what, cat='all'): |
||||
req = urllib.unquote(what) |
||||
i = 0 |
||||
results = 0 |
||||
while i < 3: |
||||
data = retrieve_url('https://api.btdigg.org/api/public-8e9a50f8335b964f/s01?%s' % urllib.urlencode(dict(q = req, p = i))) |
||||
for line in data.splitlines(): |
||||
if line.startswith('#'): |
||||
continue |
||||
|
||||
info_hash, name, files, size, dl, seen = line.strip().split('\t')[:6] |
||||
name = name.replace('|', '') |
||||
|
||||
res = dict(link = 'magnet:?xt=urn:btih:%s&dn=%s' % (info_hash, urllib.quote(name.encode('utf8'))), |
||||
name = name, |
||||
size = size, |
||||
seeds = int(dl), |
||||
leech = int(dl), |
||||
engine_url = self.url, |
||||
desc_link = '%s/search?%s' % (self.url, urllib.urlencode(dict(info_hash = info_hash, q = req)))) |
||||
|
||||
prettyPrinter(res) |
||||
results += 1 |
||||
|
||||
if results == 0: |
||||
break |
||||
i += 1 |
||||
|
||||
if __name__ == "__main__": |
||||
s = btdigg() |
||||
s.search(sys.argv[1]) |
Before Width: | Height: | Size: 619 B |
@ -1,70 +0,0 @@
@@ -1,70 +0,0 @@
|
||||
#VERSION: 1.28 |
||||
#AUTHORS: Christophe Dumez (chris@qbittorrent.org) |
||||
#CONTRIBUTORS: Diego de las Heras (ngosang@hotmail.es) |
||||
|
||||
# Redistribution and use in source and binary forms, with or without |
||||
# modification, are permitted provided that the following conditions are met: |
||||
# |
||||
# * Redistributions of source code must retain the above copyright notice, |
||||
# this list of conditions and the following disclaimer. |
||||
# * Redistributions in binary form must reproduce the above copyright |
||||
# notice, this list of conditions and the following disclaimer in the |
||||
# documentation and/or other materials provided with the distribution. |
||||
# * Neither the name of the author nor the names of its contributors may be |
||||
# used to endorse or promote products derived from this software without |
||||
# specific prior written permission. |
||||
# |
||||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" |
||||
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE |
||||
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE |
||||
# ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE |
||||
# LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR |
||||
# CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF |
||||
# SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS |
||||
# INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN |
||||
# CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) |
||||
# ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE |
||||
# POSSIBILITY OF SUCH DAMAGE. |
||||
|
||||
from novaprinter import prettyPrinter |
||||
from helpers import retrieve_url, download_file |
||||
import json |
||||
|
||||
class kickasstorrents(object): |
||||
url = 'https://kat.cr' |
||||
name = 'Kickass Torrents' |
||||
supported_categories = {'all': '', 'movies': 'Movies', 'tv': 'TV', 'music': 'Music', 'games': 'Games', 'software': 'Applications'} |
||||
|
||||
def __init__(self): |
||||
pass |
||||
|
||||
def download_torrent(self, info): |
||||
print download_file(info, info) |
||||
|
||||
def search(self, what, cat='all'): |
||||
i = 1 |
||||
while True and i < 11: |
||||
json_data = retrieve_url(self.url+'/json.php?q=%s&page=%d'%(what, i)) |
||||
try: |
||||
json_dict = json.loads(json_data) |
||||
except: |
||||
i += 1 |
||||
continue |
||||
if int(json_dict['total_results']) <= 0: |
||||
return |
||||
for r in json_dict['list']: |
||||
try: |
||||
if cat != 'all' and self.supported_categories[cat] != r['category']: |
||||
continue |
||||
res_dict = dict() |
||||
res_dict['name'] = r['title'] |
||||
res_dict['size'] = str(r['size']) |
||||
res_dict['seeds'] = r['seeds'] |
||||
res_dict['leech'] = r['leechs'] |
||||
res_dict['link'] = r['torrentLink'] |
||||
res_dict['desc_link'] = r['link'].replace('http://', 'https://') |
||||
res_dict['engine_url'] = self.url |
||||
prettyPrinter(res_dict) |
||||
except: |
||||
pass |
||||
i += 1 |
@ -1,10 +1,8 @@
@@ -1,10 +1,8 @@
|
||||
btdigg: 1.32 |
||||
demonoid: 1.21 |
||||
extratorrent: 2.04 |
||||
kickasstorrents: 1.28 |
||||
legittorrents: 2.01 |
||||
mininova: 2.02 |
||||
piratebay: 2.15 |
||||
torlock: 2.0 |
||||
torrentreactor: 1.42 |
||||
torrentz: 2.20 |
||||
torrentz: 2.21 |
||||
|
Before Width: | Height: | Size: 400 B |
@ -1,64 +0,0 @@
@@ -1,64 +0,0 @@
|
||||
#VERSION: 1.32 |
||||
#AUTHORS: BTDigg team (research@btdigg.org) |
||||
# Contributors: Diego de las Heras (ngosang@hotmail.es) |
||||
|
||||
# GNU GENERAL PUBLIC LICENSE |
||||
# Version 3, 29 June 2007 |
||||
# |
||||
# <http://www.gnu.org/licenses/> |
||||
# |
||||
# This program is free software: you can redistribute it and/or modify |
||||
# it under the terms of the GNU General Public License as published by |
||||
# the Free Software Foundation, either version 3 of the License, or |
||||
# (at your option) any later version. |
||||
# |
||||
# This program is distributed in the hope that it will be useful, |
||||
# but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
# GNU General Public License for more details. |
||||
|
||||
from novaprinter import prettyPrinter |
||||
from helpers import retrieve_url, download_file |
||||
import urllib |
||||
import sys |
||||
|
||||
class btdigg(object): |
||||
url = 'https://btdigg.org' |
||||
name = 'BTDigg' |
||||
|
||||
supported_categories = {'all': ''} |
||||
|
||||
def __init__(self): |
||||
pass |
||||
|
||||
def search(self, what, cat='all'): |
||||
req = urllib.parse.unquote(what) |
||||
i = 0 |
||||
results = 0 |
||||
while i < 3: |
||||
data = retrieve_url('https://api.btdigg.org/api/public-8e9a50f8335b964f/s01?%s' % urllib.parse.urlencode(dict(q = req, p = i))) |
||||
for line in data.splitlines(): |
||||
if line.startswith('#'): |
||||
continue |
||||
|
||||
info_hash, name, files, size, dl, seen = line.strip().split('\t')[:6] |
||||
name = name.replace('|', '') |
||||
|
||||
res = dict(link = 'magnet:?xt=urn:btih:%s&dn=%s' % (info_hash, urllib.parse.quote(name)), |
||||
name = name, |
||||
size = size, |
||||
seeds = int(dl), |
||||
leech = int(dl), |
||||
engine_url = self.url, |
||||
desc_link = '%s/search?%s' % (self.url, urllib.parse.urlencode(dict(info_hash = info_hash, q = req)))) |
||||
|
||||
prettyPrinter(res) |
||||
results += 1 |
||||
|
||||
if results == 0: |
||||
break |
||||
i += 1 |
||||
|
||||
if __name__ == "__main__": |
||||
s = btdigg() |
||||
s.search(sys.argv[1]) |
Before Width: | Height: | Size: 619 B |
@ -1,70 +0,0 @@
@@ -1,70 +0,0 @@
|
||||
#VERSION: 1.28 |
||||
#AUTHORS: Christophe Dumez (chris@qbittorrent.org) |
||||
#CONTRIBUTORS: Diego de las Heras (ngosang@hotmail.es) |
||||
|
||||
# Redistribution and use in source and binary forms, with or without |
||||
# modification, are permitted provided that the following conditions are met: |
||||
# |
||||
# * Redistributions of source code must retain the above copyright notice, |
||||
# this list of conditions and the following disclaimer. |
||||
# * Redistributions in binary form must reproduce the above copyright |
||||
# notice, this list of conditions and the following disclaimer in the |
||||
# documentation and/or other materials provided with the distribution. |
||||
# * Neither the name of the author nor the names of its contributors may be |
||||
# used to endorse or promote products derived from this software without |
||||
# specific prior written permission. |
||||
# |
||||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" |
||||
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE |
||||
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE |
||||
# ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE |
||||
# LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR |
||||
# CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF |
||||
# SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS |
||||
# INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN |
||||
# CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) |
||||
# ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE |
||||
# POSSIBILITY OF SUCH DAMAGE. |
||||
|
||||
from novaprinter import prettyPrinter |
||||
from helpers import retrieve_url, download_file |
||||
import json |
||||
|
||||
class kickasstorrents(object): |
||||
url = 'https://kat.cr' |
||||
name = 'Kickass Torrents' |
||||
supported_categories = {'all': '', 'movies': 'Movies', 'tv': 'TV', 'music': 'Music', 'games': 'Games', 'software': 'Applications'} |
||||
|
||||
def __init__(self): |
||||
pass |
||||
|
||||
def download_torrent(self, info): |
||||
print(download_file(info, info)) |
||||
|
||||
def search(self, what, cat='all'): |
||||
i = 1 |
||||
while True and i < 11: |
||||
json_data = retrieve_url(self.url+'/json.php?q=%s&page=%d'%(what, i)) |
||||
try: |
||||
json_dict = json.loads(json_data) |
||||
except: |
||||
i += 1 |
||||
continue |
||||
if int(json_dict['total_results']) <= 0: |
||||
return |
||||
for r in json_dict['list']: |
||||
try: |
||||
if cat != 'all' and self.supported_categories[cat] != r['category']: |
||||
continue |
||||
res_dict = dict() |
||||
res_dict['name'] = r['title'] |
||||
res_dict['size'] = str(r['size']) |
||||
res_dict['seeds'] = r['seeds'] |
||||
res_dict['leech'] = r['leechs'] |
||||
res_dict['link'] = r['torrentLink'] |
||||
res_dict['desc_link'] = r['link'].replace('http://', 'https://') |
||||
res_dict['engine_url'] = self.url |
||||
prettyPrinter(res_dict) |
||||
except: |
||||
pass |
||||
i += 1 |
Loading…
Reference in new issue