Browse Source
Some browsers do not download files, intended for immediate opening, into a temporary directory, and thus a regular download directories accumulate those unneeded files. The option allows qBittorrent to clean after itself and delete those files whether they were succesfully added or not (user-selectable policy).adaptive-webui-19844
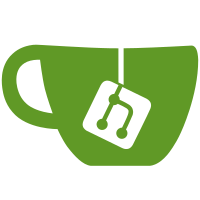
10 changed files with 391 additions and 49 deletions
@ -0,0 +1,100 @@
@@ -0,0 +1,100 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt and libtorrent. |
||||
* Copyright (C) 2016 Eugene Shalygin <eugene.shalygin@gmail.com> |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
*/ |
||||
|
||||
#include "torrentfileguard.h" |
||||
|
||||
#include <QMetaEnum> |
||||
#include "settingsstorage.h" |
||||
#include "utils/fs.h" |
||||
|
||||
namespace |
||||
{ |
||||
const QLatin1String KEY_AUTO_DELETE_ENABLED ("Core/AutoDeleteAddedTorrentFile"); |
||||
} |
||||
|
||||
FileGuard::FileGuard(const QString &path) |
||||
: m_path {path} |
||||
, m_remove {true} |
||||
{ |
||||
} |
||||
|
||||
void FileGuard::setAutoRemove(bool remove) noexcept |
||||
{ |
||||
m_remove = remove; |
||||
} |
||||
|
||||
FileGuard::~FileGuard() |
||||
{ |
||||
if (m_remove && !m_path.isEmpty()) |
||||
Utils::Fs::forceRemove(m_path); // forceRemove() checks for file existence
|
||||
} |
||||
|
||||
TorrentFileGuard::TorrentFileGuard(const QString &path) |
||||
: m_mode {autoDeleteMode()} |
||||
, m_wasAdded {false} |
||||
, m_guard {m_mode != Never ? path : QString()} |
||||
{ |
||||
} |
||||
|
||||
TorrentFileGuard::~TorrentFileGuard() |
||||
{ |
||||
if (!m_wasAdded && (m_mode != Always)) |
||||
m_guard.setAutoRemove(false); |
||||
} |
||||
|
||||
void TorrentFileGuard::markAsAddedToSession() |
||||
{ |
||||
m_wasAdded = true; |
||||
} |
||||
|
||||
void TorrentFileGuard::setAutoRemove(bool remove) |
||||
{ |
||||
m_guard.setAutoRemove(remove); |
||||
} |
||||
|
||||
TorrentFileGuard::AutoDeleteMode TorrentFileGuard::autoDeleteMode() |
||||
{ |
||||
QMetaEnum meta {modeMetaEnum()}; |
||||
return static_cast<AutoDeleteMode>(meta.keyToValue(SettingsStorage::instance()->loadValue( |
||||
KEY_AUTO_DELETE_ENABLED, meta.valueToKey(Never)).toByteArray())); |
||||
} |
||||
|
||||
void TorrentFileGuard::setautoDeleteMode(TorrentFileGuard::AutoDeleteMode mode) |
||||
{ |
||||
QMetaEnum meta {modeMetaEnum()}; |
||||
SettingsStorage::instance()->storeValue(KEY_AUTO_DELETE_ENABLED, meta.valueToKey(mode)); |
||||
} |
||||
|
||||
QMetaEnum TorrentFileGuard::modeMetaEnum() |
||||
{ |
||||
#if QT_VERSION >= QT_VERSION_CHECK(5, 7, 0) |
||||
return QMetaEnum::fromType<AutoDeleteMode>(); |
||||
#else |
||||
return staticMetaObject.enumerator(staticMetaObject.indexOfEnumerator("AutoDeleteMode")); |
||||
#endif |
||||
} |
@ -0,0 +1,95 @@
@@ -0,0 +1,95 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt and libtorrent. |
||||
* Copyright (C) 2016 Eugene Shalygin <eugene.shalygin@gmail.com> |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
*/ |
||||
|
||||
#include <QString> |
||||
#include <QMetaType> |
||||
|
||||
class QMetaEnum; |
||||
/// Utility class to defer file deletion
|
||||
class FileGuard |
||||
{ |
||||
public: |
||||
FileGuard(const QString &path = QString()); |
||||
~FileGuard(); |
||||
|
||||
/// Cancels or re-enables deferred file deletion
|
||||
void setAutoRemove(bool remove) noexcept; |
||||
|
||||
private: |
||||
QString m_path; |
||||
bool m_remove; |
||||
}; |
||||
|
||||
/// Reads settings for .torrent files from preferences
|
||||
/// and sets the file guard up accordingly
|
||||
class TorrentFileGuard |
||||
{ |
||||
Q_GADGET |
||||
|
||||
public: |
||||
TorrentFileGuard(const QString &path = QString()); |
||||
~TorrentFileGuard(); |
||||
|
||||
/// marks the torrent file as loaded (added) into the BitTorrent::Session
|
||||
void markAsAddedToSession(); |
||||
void setAutoRemove(bool remove); |
||||
|
||||
enum AutoDeleteMode // do not change these names: they are stored in config file
|
||||
{ |
||||
Never, |
||||
IfAdded, |
||||
Always |
||||
}; |
||||
|
||||
// static interface to get/set preferences
|
||||
static AutoDeleteMode autoDeleteMode(); |
||||
static void setautoDeleteMode(AutoDeleteMode mode); |
||||
|
||||
private: |
||||
static QMetaEnum modeMetaEnum(); |
||||
#if QT_VERSION < QT_VERSION_CHECK(5, 5, 0) |
||||
Q_ENUMS(AutoDeleteMode) |
||||
#else |
||||
Q_ENUM(AutoDeleteMode) |
||||
#endif |
||||
AutoDeleteMode m_mode; |
||||
bool m_wasAdded; |
||||
// Qt 4 moc has troubles with Q_GADGET: if Q_GADGET is present in a class, moc unconditionally
|
||||
// references in the generated code the statiMetaObject from the class ancestor.
|
||||
// Moreover, if the ancestor class has Q_GADGET but does not have other
|
||||
// Q_ declarations, moc does not generate staticMetaObject for it. These results
|
||||
// in referencing the non existent staticMetaObject and such code fails to compile.
|
||||
// This problem is NOT present in Qt 5.7.0 and maybe in some older Qt 5 versions too
|
||||
// Qt 4.8.7 has it.
|
||||
// Therefore, we can't inherit FileGuard :(
|
||||
FileGuard m_guard; |
||||
}; |
||||
|
||||
#if QT_VERSION < QT_VERSION_CHECK(5, 5, 0) |
||||
Q_DECLARE_METATYPE(TorrentFileGuard::AutoDeleteMode) |
||||
#endif |
Loading…
Reference in new issue