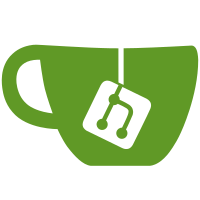
5 changed files with 183 additions and 1 deletions
@ -1,2 +1,31 @@
@@ -1,2 +1,31 @@
|
||||
# clitor |
||||
CLI util to put & get large objects in small block size |
||||
|
||||
CLI util to operate with large objects in small blocks size |
||||
|
||||
## drivers |
||||
|
||||
* [x] [kevacoin](https://github.com/kevacoin-project/kevacoin) |
||||
|
||||
### put |
||||
|
||||
export FS object to blockchain namespace |
||||
|
||||
``` |
||||
php driver/put.php processor filename [length] |
||||
``` |
||||
|
||||
* `processor` - path to kevacoin-cli |
||||
* `filename` - file path to store in blockchain |
||||
* `length` - optional split size, 3072 bytes [max](https://kevacoin.org/faq.html) |
||||
|
||||
### get |
||||
|
||||
import namespace to FS location |
||||
|
||||
``` |
||||
php driver/get.php processor namespace [destination] |
||||
``` |
||||
|
||||
* `processor` - path to kevacoin-cli |
||||
* `namespace` - hash received from the `put` command |
||||
* `destination` - optional file system location, `data/import` by default |
@ -0,0 +1,87 @@
@@ -0,0 +1,87 @@
|
||||
<?php |
||||
|
||||
// Init helper |
||||
function _exec( |
||||
string $processor, |
||||
string $command |
||||
): mixed |
||||
{ |
||||
if (false !== exec(sprintf('%s %s', $processor, $command), $output)) |
||||
{ |
||||
$rows = []; |
||||
|
||||
foreach($output as $row) |
||||
{ |
||||
$rows[] = $row; |
||||
} |
||||
|
||||
if ($result = @json_decode(implode(PHP_EOL, $rows))) |
||||
{ |
||||
return $result; |
||||
} |
||||
} |
||||
|
||||
return false; |
||||
} |
||||
|
||||
// Get last namespace value |
||||
$kevaNS = _exec( |
||||
$argv[1], |
||||
sprintf( |
||||
'%s %s "_KEVA_NS_"', |
||||
'keva_filter', |
||||
$argv[2] |
||||
) |
||||
); |
||||
|
||||
print_r($kevaNS); |
||||
|
||||
$names = []; |
||||
|
||||
foreach ($kevaNS as $ns) |
||||
{ |
||||
$names[$ns->height] = $ns->value; |
||||
} |
||||
|
||||
krsort($names); |
||||
|
||||
// Get namespace content |
||||
$parts = _exec( |
||||
$argv[1], |
||||
sprintf( |
||||
'%s %s "\d+"', |
||||
'keva_filter', |
||||
$argv[2] |
||||
) |
||||
); |
||||
|
||||
print_r($parts); |
||||
|
||||
// Merge content data |
||||
$data = []; |
||||
foreach ($parts as $part) |
||||
{ |
||||
$data[$part->key] = $part->value; |
||||
} |
||||
|
||||
ksort($data); |
||||
|
||||
// Save merged data to destination |
||||
$filename = isset($argv[3]) ? $argv[3] : sprintf( |
||||
'%s/../../data/import/kevacoin.%s.%s', |
||||
__DIR__, |
||||
$argv[2], |
||||
$names[array_key_first($names)] |
||||
); |
||||
|
||||
file_put_contents( |
||||
$filename, |
||||
base64_decode( |
||||
implode('', $data) |
||||
) |
||||
); |
||||
|
||||
echo sprintf( |
||||
'saved to %s' . PHP_EOL, |
||||
$filename |
||||
); |
@ -0,0 +1,64 @@
@@ -0,0 +1,64 @@
|
||||
<?php |
||||
|
||||
// Init helper |
||||
function _exec( |
||||
string $processor, |
||||
string $command |
||||
): mixed |
||||
{ |
||||
if (false !== exec(sprintf('%s %s', $processor, $command), $output)) |
||||
{ |
||||
$rows = []; |
||||
|
||||
foreach($output as $row) |
||||
{ |
||||
$rows[] = $row; |
||||
} |
||||
|
||||
if ($result = @json_decode(implode(PHP_EOL, $rows))) |
||||
{ |
||||
return $result; |
||||
} |
||||
} |
||||
|
||||
return false; |
||||
} |
||||
|
||||
// Create namespace |
||||
$kevaNamespace = _exec( |
||||
$argv[1], |
||||
sprintf( |
||||
'%s %s', |
||||
'keva_namespace', |
||||
basename($argv[2]) |
||||
) |
||||
); |
||||
|
||||
print_r($kevaNamespace); |
||||
|
||||
// Insert content parts |
||||
if (!empty($kevaNamespace->namespaceId)) |
||||
{ |
||||
$parts = str_split( |
||||
base64_encode( |
||||
file_get_contents($argv[2]) |
||||
), |
||||
isset($argv[3]) && $argv[3] <= 3072 ? (int) $argv[3] : 3072 // 3072 bytes limit |
||||
); |
||||
|
||||
foreach ($parts as $key => $value) |
||||
{ |
||||
$kevaPut = _exec( |
||||
$argv[1], |
||||
sprintf( |
||||
'%s %s %s %s', |
||||
'keva_put', |
||||
$kevaNamespace->namespaceId, |
||||
$key, |
||||
$value |
||||
) |
||||
); |
||||
|
||||
print_r($kevaPut); |
||||
} |
||||
} |
Loading…
Reference in new issue