mirror of https://github.com/YGGverse/titan-II.git
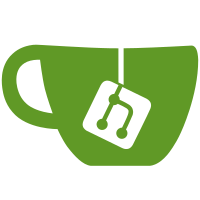
5 changed files with 121 additions and 8 deletions
@ -1,3 +1,37 @@
@@ -1,3 +1,37 @@
|
||||
# Titan II |
||||
|
||||
Gemini Protocol library for PHP. |
||||
Gemini Protocol library for PHP. |
||||
|
||||
## Basic Implentation |
||||
|
||||
``` |
||||
<?php |
||||
|
||||
use TitanII\Request; |
||||
use TitanII\Response; |
||||
use TitanII\Server; |
||||
|
||||
$server = new Server(); |
||||
|
||||
$server->setCert('cert.pem'); |
||||
$server->setKey('key.rsa'); |
||||
|
||||
$server->setHandler(function (Request $request): Response { |
||||
$response = new Response(); |
||||
|
||||
$response->setCode(20); |
||||
$response->setMeta("text/plain"); |
||||
$response->setContent("Hello World!"); |
||||
|
||||
return $response; |
||||
}); |
||||
|
||||
$server->start(); |
||||
``` |
||||
|
||||
## Instructions |
||||
|
||||
1. run `cd test/certs; openssl req -x509 -newkey rsa:4096 -keyout key.rsa -out cert.pem -days 3650 -nodes -subj "/CN=127.0.0.1"` |
||||
2. run `composer install` |
||||
3. run `cd ..; php server.php` |
||||
4. Open `gemini://127.0.0.1` |
Loading…
Reference in new issue