mirror of https://github.com/YGGverse/net-php.git
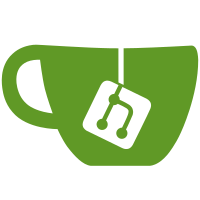
2 changed files with 216 additions and 0 deletions
@ -0,0 +1,185 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace Yggverse\Net; |
||||||
|
|
||||||
|
class Resolve |
||||||
|
{ |
||||||
|
private array $_providers = |
||||||
|
[ |
||||||
|
'1.1.1.1', |
||||||
|
'8.8.8.8' |
||||||
|
]; |
||||||
|
|
||||||
|
private array $_records = |
||||||
|
[ |
||||||
|
'A', |
||||||
|
'AAAA' |
||||||
|
]; |
||||||
|
|
||||||
|
private int $_timeout = 5; |
||||||
|
|
||||||
|
private bool $_shuffle = false; |
||||||
|
|
||||||
|
public function __construct( |
||||||
|
?array $records = null, |
||||||
|
?array $providers = null, |
||||||
|
?int $timeout = null, |
||||||
|
bool $shuffle = false |
||||||
|
) { |
||||||
|
if ($records) |
||||||
|
{ |
||||||
|
$this->setRecords( |
||||||
|
$records |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
if ($providers) |
||||||
|
{ |
||||||
|
$this->setProviders( |
||||||
|
$providers |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
if ($timeout) |
||||||
|
{ |
||||||
|
$this->setTimeout( |
||||||
|
$timeout |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
$this->setShuffle( |
||||||
|
$shuffle |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
public function getRecords(): array |
||||||
|
{ |
||||||
|
return $this->_records; |
||||||
|
} |
||||||
|
|
||||||
|
public function setRecords(array $records): void |
||||||
|
{ |
||||||
|
foreach ($records as $record) |
||||||
|
{ |
||||||
|
$this->addRecord( |
||||||
|
$record |
||||||
|
); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public function addRecord(string $record): void |
||||||
|
{ |
||||||
|
$this->_records[] = $record; |
||||||
|
|
||||||
|
$this->_records = array_unique( |
||||||
|
$this->_records |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
public function getProviders(): array |
||||||
|
{ |
||||||
|
return $this->_providers; |
||||||
|
} |
||||||
|
|
||||||
|
public function setProviders(array $providers): void |
||||||
|
{ |
||||||
|
foreach ($providers as $provider) |
||||||
|
{ |
||||||
|
$this->addProvider( |
||||||
|
$provider |
||||||
|
); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public function addProvider(string $provider): void |
||||||
|
{ |
||||||
|
$this->_providers[] = $provider; |
||||||
|
|
||||||
|
$this->_providers = array_unique( |
||||||
|
$this->_providers |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
public function setTimeout( |
||||||
|
int $timeout |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_timeout = $timeout; |
||||||
|
} |
||||||
|
|
||||||
|
public function getTimeout(): int |
||||||
|
{ |
||||||
|
return $this->_timeout; |
||||||
|
} |
||||||
|
|
||||||
|
public function setShuffle( |
||||||
|
bool $shuffle |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_shuffle = $shuffle; |
||||||
|
} |
||||||
|
|
||||||
|
public function isShuffle(): bool |
||||||
|
{ |
||||||
|
return $this->_shuffle; |
||||||
|
} |
||||||
|
|
||||||
|
public function address( |
||||||
|
string $url, |
||||||
|
array &$result = [], |
||||||
|
array &$error = [] |
||||||
|
): ?\Yggverse\Net\Address |
||||||
|
{ |
||||||
|
$address = new \Yggverse\Net\Address( |
||||||
|
$url |
||||||
|
); |
||||||
|
|
||||||
|
foreach ($this->_providers as $provider) |
||||||
|
{ |
||||||
|
foreach ( |
||||||
|
\Yggverse\Net\Dig::records( |
||||||
|
$address->getHost(), |
||||||
|
$this->_records, |
||||||
|
$result, |
||||||
|
$error, |
||||||
|
$provider, |
||||||
|
$this->_timeout |
||||||
|
) as $record => $data |
||||||
|
) { |
||||||
|
|
||||||
|
if ($this->_shuffle) |
||||||
|
{ |
||||||
|
shuffle( |
||||||
|
$data |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
foreach ($data as $host) |
||||||
|
{ |
||||||
|
if (false !== filter_var($host, FILTER_VALIDATE_IP, FILTER_FLAG_IPV6)) |
||||||
|
{ |
||||||
|
$resolved->setHost( |
||||||
|
sprintf( |
||||||
|
'[%s]', |
||||||
|
$host |
||||||
|
) |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
else |
||||||
|
{ |
||||||
|
$address->setHost( |
||||||
|
$host |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
return $address; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
return null; |
||||||
|
} |
||||||
|
} |
Loading…
Reference in new issue