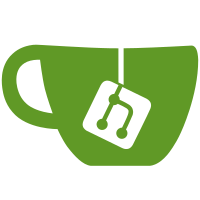
11 changed files with 4363 additions and 19 deletions
@ -0,0 +1,256 @@
@@ -0,0 +1,256 @@
|
||||
/***
|
||||
* |
||||
* Copyright (c) 1996-2002, Valve LLC. All rights reserved. |
||||
* |
||||
* This product contains software technology licensed from Id |
||||
* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc. |
||||
* All Rights Reserved. |
||||
* |
||||
* Use, distribution, and modification of this source code and/or resulting |
||||
* object code is restricted to non-commercial enhancements to products from |
||||
* Valve LLC. All other use, distribution, or modification is prohibited |
||||
* without written permission from Valve LLC. |
||||
* |
||||
****/ |
||||
|
||||
#include "hud.h" |
||||
#include "cl_util.h" |
||||
#include "parsemsg.h" |
||||
|
||||
#include "const.h" |
||||
#include "entity_state.h" |
||||
#include "cl_entity.h" |
||||
#include "entity_types.h" |
||||
#include "usercmd.h" |
||||
#include "pm_defs.h" |
||||
#include "pm_materials.h" |
||||
#include "ref_params.h" |
||||
#include <string.h> |
||||
#include "vgui_viewport.h" |
||||
#include "vgui_ScorePanel.h" |
||||
|
||||
#define RED_FLAG_STOLE 1 |
||||
#define BLUE_FLAG_STOLE 2 |
||||
#define RED_FLAG_LOST 3 |
||||
#define BLUE_FLAG_LOST 4 |
||||
#define RED_FLAG_ATBASE 5 |
||||
#define BLUE_FLAG_ATBASE 6 |
||||
|
||||
#define ITEM_RUNE1_FLAG 1 |
||||
#define ITEM_RUNE2_FLAG 2 |
||||
#define ITEM_RUNE3_FLAG 3 |
||||
#define ITEM_RUNE4_FLAG 4 |
||||
|
||||
DECLARE_MESSAGE(m_FlagStat, FlagStat) |
||||
DECLARE_MESSAGE(m_FlagStat, RuneStat) |
||||
DECLARE_MESSAGE(m_FlagStat, FlagCarrier) |
||||
|
||||
int CHudFlagStatus::Init(void) |
||||
{ |
||||
HOOK_MESSAGE( FlagStat ); |
||||
HOOK_MESSAGE( RuneStat ); |
||||
HOOK_MESSAGE( FlagCarrier ); |
||||
|
||||
m_iFlags |= HUD_ACTIVE; |
||||
|
||||
gHUD.AddHudElem(this); |
||||
|
||||
Reset(); |
||||
|
||||
return 1; |
||||
}; |
||||
|
||||
int CHudFlagStatus::VidInit(void) |
||||
{ |
||||
m_iBlueAtBaseIndex = gHUD.GetSpriteIndex( "blue_atbase" ); |
||||
m_iBlueLostIndex = gHUD.GetSpriteIndex( "blue_lost" ); |
||||
m_iBlueStolenIndex = gHUD.GetSpriteIndex( "blue_stolen" ); |
||||
|
||||
m_iRedAtBaseIndex = gHUD.GetSpriteIndex( "red_atbase" ); |
||||
m_iRedLostIndex = gHUD.GetSpriteIndex( "red_lost" ); |
||||
m_iRedStolenIndex = gHUD.GetSpriteIndex( "red_stolen" ); |
||||
|
||||
m_iRune1Index = gHUD.GetSpriteIndex( "rune1" ); |
||||
m_iRune2Index = gHUD.GetSpriteIndex( "rune2" ); |
||||
m_iRune3Index = gHUD.GetSpriteIndex( "rune3" ); |
||||
m_iRune4Index = gHUD.GetSpriteIndex( "rune4" ); |
||||
|
||||
m_hBlueAtBase = gHUD.GetSprite( m_iBlueAtBaseIndex ); |
||||
m_hBlueLost = gHUD.GetSprite( m_iBlueLostIndex ); |
||||
m_hBlueStolen = gHUD.GetSprite( m_iBlueStolenIndex ); |
||||
|
||||
m_hRedAtBase = gHUD.GetSprite( m_iRedAtBaseIndex ); |
||||
m_hRedLost = gHUD.GetSprite( m_iRedLostIndex ); |
||||
m_hRedStolen = gHUD.GetSprite( m_iRedStolenIndex ); |
||||
|
||||
m_hRune1 = gHUD.GetSprite( m_iRune1Index ); |
||||
m_hRune2 = gHUD.GetSprite( m_iRune2Index ); |
||||
m_hRune3 = gHUD.GetSprite( m_iRune3Index ); |
||||
m_hRune4 = gHUD.GetSprite( m_iRune4Index ); |
||||
|
||||
// Load sprites here
|
||||
m_iBlueFlagIndex = gHUD.GetSpriteIndex( "b_flag_c" ); |
||||
m_iRedFlagIndex = gHUD.GetSpriteIndex( "r_flag_c" ); |
||||
|
||||
m_hBlueFlag = gHUD.GetSprite( m_iBlueFlagIndex ); |
||||
m_hRedFlag = gHUD.GetSprite( m_iRedFlagIndex ); |
||||
|
||||
return 1; |
||||
} |
||||
|
||||
void CHudFlagStatus :: Reset( void ) |
||||
{ |
||||
return; |
||||
} |
||||
|
||||
|
||||
int CHudFlagStatus ::Draw(float flTime ) |
||||
{ |
||||
|
||||
if ( !iDrawStatus ) |
||||
return 1; |
||||
|
||||
int x, y; |
||||
int r,g,b; |
||||
|
||||
r = g = b = 255; |
||||
|
||||
x = 20; |
||||
y = ( ScreenHeight - gHUD.m_iFontHeight ) - ( gHUD.m_iFontHeight / 2 ) - 40; |
||||
|
||||
|
||||
switch ( iBlueFlagStatus ) |
||||
{ |
||||
case BLUE_FLAG_STOLE: |
||||
SPR_Set( m_hBlueStolen, r, g, b ); |
||||
SPR_DrawHoles( 1, x, y, NULL ); |
||||
break; |
||||
case BLUE_FLAG_LOST: |
||||
SPR_Set( m_hBlueLost, r, g, b ); |
||||
SPR_DrawHoles( 1, x, y, NULL ); |
||||
break; |
||||
case BLUE_FLAG_ATBASE: |
||||
SPR_Set( m_hBlueAtBase, r, g, b ); |
||||
SPR_DrawHoles( 1, x, y, NULL ); |
||||
break; |
||||
} |
||||
|
||||
x = 50; |
||||
|
||||
if ( iBlueTeamScore < 10) |
||||
{ |
||||
x += 3; |
||||
gHUD.DrawHudNumber( x, y + 4, DHN_DRAWZERO, iBlueTeamScore, 255, 255, 255 ); |
||||
} |
||||
else if ( iBlueTeamScore >= 10 && iBlueTeamScore < 100 ) |
||||
gHUD.DrawHudNumber( x, y + 4, DHN_2DIGITS | DHN_DRAWZERO, iBlueTeamScore, 255, 255, 255 ); |
||||
|
||||
x = 20; |
||||
y = ( ScreenHeight - gHUD.m_iFontHeight ) - ( gHUD.m_iFontHeight / 2 ) - 75; |
||||
|
||||
switch ( iRedFlagStatus ) |
||||
{ |
||||
case RED_FLAG_STOLE: |
||||
SPR_Set( m_hRedStolen, r, g, b ); |
||||
SPR_DrawHoles( 1, x, y, NULL ); |
||||
break; |
||||
case RED_FLAG_LOST: |
||||
SPR_Set( m_hRedLost, r, g, b ); |
||||
SPR_DrawHoles( 1, x, y, NULL ); |
||||
break; |
||||
case RED_FLAG_ATBASE: |
||||
SPR_Set( m_hRedAtBase, r, g, b ); |
||||
SPR_DrawHoles( 1, x, y, NULL ); |
||||
break; |
||||
} |
||||
|
||||
x = 50; |
||||
if ( iRedTeamScore < 10) |
||||
{ |
||||
x += 3; |
||||
gHUD.DrawHudNumber( x, y + 4, DHN_DRAWZERO, iRedTeamScore, 255, 255, 255 ); |
||||
} |
||||
else if ( iBlueTeamScore >= 10 && iBlueTeamScore < 100 ) |
||||
gHUD.DrawHudNumber( x, y + 4, DHN_2DIGITS | DHN_DRAWZERO, iRedTeamScore, 255, 255, 255 ); |
||||
|
||||
x = 20; |
||||
y = ( ScreenHeight - gHUD.m_iFontHeight ) - ( gHUD.m_iFontHeight / 2 ) - 110; |
||||
|
||||
switch ( m_iRuneStat ) |
||||
{ |
||||
case ITEM_RUNE1_FLAG: |
||||
SPR_Set( m_hRune1, r, g, b ); |
||||
SPR_Draw( 1, x, y, NULL ); |
||||
break; |
||||
|
||||
case ITEM_RUNE2_FLAG: |
||||
SPR_Set( m_hRune2, r, g, b ); |
||||
SPR_Draw( 1, x, y, NULL ); |
||||
break; |
||||
|
||||
case ITEM_RUNE3_FLAG: |
||||
SPR_Set( m_hRune3, r, g, b ); |
||||
SPR_Draw( 1, x, y, NULL ); |
||||
break; |
||||
|
||||
case ITEM_RUNE4_FLAG: |
||||
SPR_Set( m_hRune4, r, g, b ); |
||||
SPR_Draw( 1, x, y, NULL ); |
||||
break; |
||||
} |
||||
|
||||
return 1; |
||||
} |
||||
|
||||
int CHudFlagStatus::MsgFunc_FlagStat(const char *pszName, int iSize, void *pbuf) |
||||
{ |
||||
BEGIN_READ( pbuf, iSize ); |
||||
|
||||
iDrawStatus = READ_BYTE(); |
||||
iRedFlagStatus = READ_BYTE(); |
||||
iBlueFlagStatus = READ_BYTE(); |
||||
|
||||
iRedTeamScore = READ_BYTE(); |
||||
iBlueTeamScore = READ_BYTE(); |
||||
|
||||
return 1; |
||||
} |
||||
|
||||
int CHudFlagStatus::MsgFunc_RuneStat(const char *pszName, int iSize, void *pbuf) |
||||
{ |
||||
BEGIN_READ( pbuf, iSize ); |
||||
|
||||
m_iRuneStat = READ_BYTE(); |
||||
|
||||
return 1; |
||||
} |
||||
|
||||
int CHudFlagStatus::MsgFunc_FlagCarrier(const char *pszName, int iSize, void *pbuf) |
||||
{ |
||||
BEGIN_READ( pbuf, iSize ); |
||||
|
||||
int index = READ_BYTE(); |
||||
|
||||
bool bRedFlag = false; |
||||
bool bBlueFlag = false; |
||||
|
||||
g_PlayerExtraInfo[ index ].iHasFlag = READ_BYTE(); |
||||
|
||||
for ( int i = 1; i < MAX_PLAYERS + 1; i++ ) |
||||
{ |
||||
if ( g_PlayerExtraInfo[ i ].iHasFlag ) |
||||
{ |
||||
if ( g_PlayerExtraInfo[ i ].teamnumber == 1 ) |
||||
bRedFlag = true; |
||||
else if ( g_PlayerExtraInfo[ i ].teamnumber == 2 ) |
||||
bBlueFlag = true; |
||||
} |
||||
} |
||||
|
||||
if ( !bRedFlag ) |
||||
gViewPort->m_pScoreBoard->m_pImages[ 5 ]->setVisible( false ); |
||||
if ( !bBlueFlag ) |
||||
gViewPort->m_pScoreBoard->m_pImages[ 4 ]->setVisible( false ); |
||||
|
||||
return 1; |
||||
} |
@ -0,0 +1,195 @@
@@ -0,0 +1,195 @@
|
||||
/***
|
||||
* |
||||
* Copyright (c) 1996-2002, Valve LLC. All rights reserved. |
||||
* |
||||
* This product contains software technology licensed from Id |
||||
* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc. |
||||
* All Rights Reserved. |
||||
* |
||||
* Use, distribution, and modification of this source code and/or resulting |
||||
* object code is restricted to non-commercial enhancements to products from |
||||
* Valve LLC. All other use, distribution, or modification is prohibited |
||||
* without written permission from Valve LLC. |
||||
* |
||||
****/ |
||||
|
||||
#include "hud.h" |
||||
#include "cl_util.h" |
||||
#include "parsemsg.h" |
||||
|
||||
#include "const.h" |
||||
#include "entity_state.h" |
||||
#include "cl_entity.h" |
||||
#include "entity_types.h" |
||||
#include "usercmd.h" |
||||
#include "pm_defs.h" |
||||
#include "pm_materials.h" |
||||
#include "ref_params.h" |
||||
#include <string.h> |
||||
|
||||
|
||||
#define MAX_BONUS 10 |
||||
|
||||
#define RED_FLAG_STOLEN 1 |
||||
#define BLUE_FLAG_STOLEN 2 |
||||
#define RED_FLAG_CAPTURED 3 |
||||
#define BLUE_FLAG_CAPTURED 4 |
||||
#define RED_FLAG_RETURNED_PLAYER 5 |
||||
#define BLUE_FLAG_RETURNED_PLAYER 6 |
||||
#define RED_FLAG_RETURNED 7 |
||||
#define BLUE_FLAG_RETURNED 8 |
||||
#define RED_FLAG_LOST_HUD 9 |
||||
#define BLUE_FLAG_LOST_HUD 10 |
||||
|
||||
|
||||
|
||||
char *sBonusStrings[] = |
||||
{ |
||||
"", |
||||
"\\w stole the \\rRED\\w Flag!", |
||||
"\\w stole the \\bBLUE\\w Flag!", |
||||
"\\w captured the \\rRED\\w Flag", |
||||
"\\w captured the \\bBLUE\\w Flag", |
||||
"\\w returned the \\rRED\\w Flag", |
||||
"\\w returned the \\bBLUE\\w Flag", |
||||
"\\wThe \\rRED\\w Flag has Returned", |
||||
"\\wThe \\bBLUE\\w Flag has Returned", |
||||
"\\w lost the \\rRED\\w Flag!", |
||||
"\\w lost the \\bBLUE\\w Flag!", |
||||
}; |
||||
|
||||
DECLARE_MESSAGE(m_Bonus, Bonus) |
||||
|
||||
struct bonus_info_t |
||||
{ |
||||
int iSlot; |
||||
int iType; |
||||
bool bActive; |
||||
float flBonusTime; |
||||
char sPlayerName[64]; |
||||
}; |
||||
|
||||
bonus_info_t g_PlayerBonus[MAX_BONUS+1]; |
||||
|
||||
int CHudBonus::Init(void) |
||||
{ |
||||
HOOK_MESSAGE( Bonus ); |
||||
|
||||
m_iFlags |= HUD_ACTIVE; |
||||
|
||||
gHUD.AddHudElem(this); |
||||
|
||||
Reset(); |
||||
|
||||
return 1; |
||||
}; |
||||
|
||||
int CHudBonus::VidInit(void) |
||||
{ |
||||
return 1; |
||||
} |
||||
|
||||
void CHudBonus :: Reset( void ) |
||||
{ |
||||
m_iFlags |= HUD_ACTIVE; |
||||
|
||||
for ( int reset = 0; reset < MAX_BONUS; reset++) |
||||
{ |
||||
g_PlayerBonus[ reset ].flBonusTime = 0.0; |
||||
g_PlayerBonus[ reset ].iSlot = 0; |
||||
g_PlayerBonus[ reset ].iType = 0; |
||||
g_PlayerBonus[ reset ].bActive = false; |
||||
m_bUsedSlot[ reset ] = false; |
||||
strcpy ( g_PlayerBonus[ reset ].sPlayerName, "" ); |
||||
} |
||||
} |
||||
|
||||
int CHudBonus ::Draw(float flTime ) |
||||
{ |
||||
for (int index = 1; index < MAX_BONUS + 1; index++) |
||||
{ |
||||
//Just activated
|
||||
if ( g_PlayerBonus[ index ].bActive && !g_PlayerBonus[ index ].flBonusTime ) |
||||
{ |
||||
g_PlayerBonus[ index ].flBonusTime = flTime + 5.0; |
||||
|
||||
for ( int i = 1; i < MAX_BONUS + 1; i++ ) |
||||
{ |
||||
if ( m_bUsedSlot[ i ] == false ) //found one thats not used
|
||||
{ |
||||
m_bUsedSlot[ i ] = true; //use it!
|
||||
g_PlayerBonus[ index ].iSlot = i; |
||||
break; |
||||
} |
||||
} |
||||
} |
||||
|
||||
if ( g_PlayerBonus[ index ].flBonusTime > flTime ) |
||||
{ |
||||
int YPos; |
||||
int iMod = gHUD.ReturnStringPixelLength( "\\w\\r\\w" ); |
||||
|
||||
YPos = ( ( ScreenHeight - gHUD.m_iFontHeight ) - ( gHUD.m_iFontHeight / 2 ) + 3 ) - ( 30 * g_PlayerBonus[ index ].iSlot ); |
||||
|
||||
int XPos = 75; |
||||
|
||||
char szText[256]; |
||||
|
||||
strcpy ( szText, g_PlayerBonus[ index ].sPlayerName ); |
||||
strcat ( szText, sBonusStrings[ g_PlayerBonus[ index ].iType ] ); |
||||
|
||||
if ( gHUD.m_FlagStat.iBlueTeamScore >= 10 ) |
||||
gHUD.DrawHudStringCTF( XPos + 20, YPos, 640, szText, 255, 255, 255 ); |
||||
else |
||||
gHUD.DrawHudStringCTF( XPos , YPos, 320, szText, 255, 255, 255 ); |
||||
|
||||
} |
||||
|
||||
if ( g_PlayerBonus[ index ].flBonusTime < flTime ) |
||||
{ |
||||
g_PlayerBonus[ index ].bActive = false; |
||||
m_bUsedSlot[ g_PlayerBonus[ index ].iSlot ] = false; |
||||
g_PlayerBonus[ index ].iSlot = 0; |
||||
strcpy ( g_PlayerBonus[ index ].sPlayerName, "" ); |
||||
} |
||||
} |
||||
|
||||
return 1; |
||||
} |
||||
|
||||
int CHudBonus::MsgFunc_Bonus(const char *pszName, int iSize, void *pbuf) |
||||
{ |
||||
BEGIN_READ( pbuf, iSize ); |
||||
|
||||
for ( int index = 1; index < MAX_BONUS + 1; index++) |
||||
{ |
||||
//Find wich one is not used
|
||||
if ( g_PlayerBonus[ index ].bActive == false ) |
||||
break; //Not using this one?, then we shall use this.
|
||||
} |
||||
|
||||
g_PlayerBonus[ index ].bActive = true; |
||||
g_PlayerBonus[ index ].flBonusTime = 0.0; |
||||
g_PlayerBonus[ index ].iType = READ_BYTE(); |
||||
strcpy ( g_PlayerBonus[ index ].sPlayerName, READ_STRING() ); |
||||
|
||||
switch ( g_PlayerBonus[ index ].iType ) |
||||
{ |
||||
case RED_FLAG_STOLEN: |
||||
case BLUE_FLAG_STOLEN: |
||||
PlaySound( "ctf/flagtk.wav", 1 ); |
||||
break; |
||||
case RED_FLAG_CAPTURED: |
||||
case BLUE_FLAG_CAPTURED: |
||||
PlaySound( "ctf/flagcap.wav", 1 ); |
||||
break; |
||||
case RED_FLAG_RETURNED_PLAYER: |
||||
case BLUE_FLAG_RETURNED_PLAYER: |
||||
case RED_FLAG_RETURNED: |
||||
case BLUE_FLAG_RETURNED: |
||||
PlaySound( "ctf/flagret.wav", 1 ); |
||||
break; |
||||
} |
||||
|
||||
return 1; |
||||
} |
File diff suppressed because it is too large
Load Diff
@ -0,0 +1,232 @@
@@ -0,0 +1,232 @@
|
||||
/***
|
||||
* |
||||
* Copyright (c) 1996-2002, Valve LLC. All rights reserved. |
||||
* |
||||
* This product contains software technology licensed from Id |
||||
* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc. |
||||
* All Rights Reserved. |
||||
* |
||||
* Use, distribution, and modification of this source code and/or resulting |
||||
* object code is restricted to non-commercial enhancements to products from |
||||
* Valve LLC. All other use, distribution, or modification is prohibited |
||||
* without written permission from Valve LLC. |
||||
* |
||||
****/ |
||||
|
||||
#define BLUE 2 |
||||
#define RED 1 |
||||
|
||||
|
||||
#include "voice_gamemgr.h" |
||||
|
||||
|
||||
|
||||
//=========================================================
|
||||
// Flags
|
||||
//=========================================================
|
||||
class CItemFlag : public CBaseEntity |
||||
{ |
||||
public: |
||||
void Spawn( void ); |
||||
|
||||
BOOL Dropped; |
||||
float m_flDroppedTime; |
||||
|
||||
void EXPORT FlagThink( void ); |
||||
|
||||
private: |
||||
void Precache ( void ); |
||||
void Capture(CBasePlayer *pPlayer, int iTeam ); |
||||
void ResetFlag( int iTeam ); |
||||
void Materialize( void ); |
||||
void EXPORT FlagTouch( CBaseEntity *pOther ); |
||||
// BOOL MyTouch( CBasePlayer *pPlayer );
|
||||
|
||||
}; |
||||
|
||||
class CCarriedFlag : public CBaseEntity |
||||
{ |
||||
public: |
||||
void Spawn( void ); |
||||
|
||||
CBasePlayer *Owner; |
||||
|
||||
int m_iOwnerOldVel; |
||||
|
||||
private: |
||||
void Precache ( void ); |
||||
void EXPORT FlagThink( void ); |
||||
}; |
||||
|
||||
|
||||
class CResistRune : public CBaseEntity |
||||
{ |
||||
private: |
||||
|
||||
void EXPORT RuneRespawn ( void ); |
||||
|
||||
public: |
||||
|
||||
void EXPORT RuneTouch ( CBaseEntity *pOther ); |
||||
void Spawn( void ); |
||||
|
||||
void EXPORT MakeTouchable ( void ); |
||||
|
||||
int m_iRuneFlag; |
||||
bool m_bTouchable; |
||||
bool dropped; |
||||
}; |
||||
|
||||
class CStrengthRune : public CBaseEntity |
||||
{ |
||||
|
||||
private: |
||||
void EXPORT RuneRespawn ( void ); |
||||
|
||||
public: |
||||
|
||||
void EXPORT RuneTouch ( CBaseEntity *pOther ); |
||||
void Spawn( void ); |
||||
|
||||
void EXPORT MakeTouchable ( void ); |
||||
|
||||
int m_iRuneFlag; |
||||
bool m_bTouchable; |
||||
bool dropped; |
||||
}; |
||||
|
||||
|
||||
class CHasteRune : public CBaseEntity |
||||
{ |
||||
|
||||
private: |
||||
void EXPORT RuneRespawn ( void ); |
||||
public: |
||||
|
||||
void EXPORT RuneTouch ( CBaseEntity *pOther ); |
||||
|
||||
void EXPORT MakeTouchable ( void ); |
||||
void Spawn( void ); |
||||
|
||||
int m_iRuneFlag; |
||||
bool m_bTouchable; |
||||
bool dropped; |
||||
}; |
||||
|
||||
|
||||
class CRegenRune : public CBaseEntity |
||||
{ |
||||
|
||||
private: |
||||
void EXPORT RuneRespawn ( void ); |
||||
|
||||
public: |
||||
|
||||
void EXPORT RuneTouch ( CBaseEntity *pOther ); |
||||
void Spawn( void ); |
||||
|
||||
void EXPORT MakeTouchable ( void ); |
||||
|
||||
int m_iRuneFlag; |
||||
bool m_bTouchable; |
||||
bool dropped; |
||||
}; |
||||
|
||||
class CGrapple : public CBaseEntity |
||||
{ |
||||
public: |
||||
|
||||
//Yes, I have no imagination so I use standard touch, spawn and think function names.
|
||||
//Sue me! =P.
|
||||
void Spawn ( void ); |
||||
void EXPORT OnAirThink ( void ); |
||||
void EXPORT GrappleTouch ( CBaseEntity *pOther ); |
||||
void Reset_Grapple ( void ); |
||||
void EXPORT Grapple_Track ( void ); |
||||
|
||||
float m_flNextIdleTime; |
||||
|
||||
}; |
||||
|
||||
|
||||
|
||||
#define STEAL_SOUND 1 |
||||
#define CAPTURE_SOUND 2 |
||||
#define RETURN_SOUND 3 |
||||
|
||||
#define RED_FLAG_STOLEN 1 |
||||
#define BLUE_FLAG_STOLEN 2 |
||||
#define RED_FLAG_CAPTURED 3 |
||||
#define BLUE_FLAG_CAPTURED 4 |
||||
#define RED_FLAG_RETURNED_PLAYER 5 |
||||
#define BLUE_FLAG_RETURNED_PLAYER 6 |
||||
#define RED_FLAG_RETURNED 7 |
||||
#define BLUE_FLAG_RETURNED 8 |
||||
#define RED_FLAG_LOST 9 |
||||
#define BLUE_FLAG_LOST 10 |
||||
|
||||
#define RED_FLAG_STOLEN 1 |
||||
#define BLUE_FLAG_STOLEN 2 |
||||
#define RED_FLAG_DROPPED 3 |
||||
#define BLUE_FLAG_DROPPED 4 |
||||
#define RED_FLAG_ATBASE 5 |
||||
#define BLUE_FLAG_ATBASE 6 |
||||
|
||||
|
||||
#define MAX_TEAMNAME_LENGTH 16 |
||||
#define MAX_TEAMS 32 |
||||
|
||||
#define TEAMPLAY_TEAMLISTLENGTH MAX_TEAMS*MAX_TEAMNAME_LENGTH |
||||
|
||||
class CThreeWave : public CHalfLifeMultiplay |
||||
{ |
||||
public: |
||||
CThreeWave(); |
||||
|
||||
virtual BOOL ClientConnected( edict_t *pEntity, const char *pszName, const char *pszAddress, char szRejectReason[ 128 ] ); |
||||
virtual BOOL ClientCommand( CBasePlayer *pPlayer, const char *pcmd ); |
||||
virtual void ClientUserInfoChanged( CBasePlayer *pPlayer, char *infobuffer ); |
||||
virtual BOOL IsTeamplay( void ); |
||||
virtual BOOL FPlayerCanTakeDamage( CBasePlayer *pPlayer, CBaseEntity *pAttacker ); |
||||
virtual int PlayerRelationship( CBaseEntity *pPlayer, CBaseEntity *pTarget ); |
||||
virtual const char *GetTeamID( CBaseEntity *pEntity ); |
||||
virtual BOOL ShouldAutoAim( CBasePlayer *pPlayer, edict_t *target ); |
||||
virtual int IPointsForKill( CBasePlayer *pAttacker, CBasePlayer *pKilled ); |
||||
virtual void InitHUD( CBasePlayer *pl ); |
||||
virtual void DeathNotice( CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pevInflictor ); |
||||
virtual const char *GetGameDescription( void ) { return "3Wave CTF"; } // this is the game name that gets seen in the server browser
|
||||
virtual void UpdateGameMode( CBasePlayer *pPlayer ); // the client needs to be informed of the current game mode
|
||||
virtual void PlayerKilled( CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pInflictor ); |
||||
virtual void Think ( void ); |
||||
virtual int GetTeamIndex( const char *pTeamName ); |
||||
virtual const char *GetIndexedTeamName( int teamIndex ); |
||||
virtual BOOL IsValidTeam( const char *pTeamName ); |
||||
virtual void ChangePlayerTeam( CBasePlayer *pPlayer, int iTeam ); |
||||
virtual void PlayerSpawn( CBasePlayer *pPlayer ); |
||||
void JoinTeam ( CBasePlayer *pPlayer, int iTeam ); |
||||
int TeamWithFewestPlayers ( void ); |
||||
virtual void ClientDisconnected( edict_t *pClient ); |
||||
void GetFlagStatus( CBasePlayer *pPlayer ); |
||||
|
||||
virtual edict_t *GetPlayerSpawnSpot( CBasePlayer *pPlayer ); |
||||
|
||||
virtual void PlayerThink( CBasePlayer *pPlayer ); |
||||
|
||||
void PlayerTakeDamage( CBasePlayer *pPlayer , CBaseEntity *pAttacker ); |
||||
|
||||
int iBlueFlagStatus; |
||||
int iRedFlagStatus; |
||||
|
||||
int iBlueTeamScore; |
||||
int iRedTeamScore; |
||||
|
||||
float m_flFlagStatusTime; |
||||
|
||||
private: |
||||
void RecountTeams( void ); |
||||
|
||||
BOOL m_DisableDeathMessages; |
||||
BOOL m_DisableDeathPenalty; |
||||
BOOL m_teamLimit; // This means the server set only some teams as valid
|
||||
char m_szTeamList[TEAMPLAY_TEAMLISTLENGTH]; |
||||
}; |
Loading…
Reference in new issue