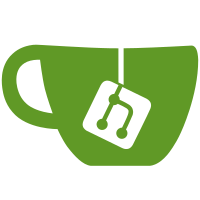
3 changed files with 0 additions and 406 deletions
@ -1,235 +0,0 @@
@@ -1,235 +0,0 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Gemini\Gemtext; |
||||
|
||||
class Body |
||||
{ |
||||
private array $_lines = []; |
||||
|
||||
public function __construct(string $gemtext) |
||||
{ |
||||
foreach ((array) explode(PHP_EOL, $gemtext) as $index => $line) |
||||
{ |
||||
$this->_lines[$index] = $line; |
||||
} |
||||
} |
||||
|
||||
public function getLine(int $index): ?int |
||||
{ |
||||
return isset($this->_lines[$index]) ? $this->_lines[$index] : null; |
||||
} |
||||
|
||||
public function getLines(): array |
||||
{ |
||||
return $this->_lines; |
||||
} |
||||
|
||||
public function getH1(): array |
||||
{ |
||||
$matches = []; |
||||
|
||||
foreach ($this->_lines as $index => $line) |
||||
{ |
||||
if (preg_match('/^#([^#]+)/', trim($line), $match)) |
||||
{ |
||||
$matches[$index] = trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
} |
||||
|
||||
return $matches; |
||||
} |
||||
|
||||
public function getH2(): array |
||||
{ |
||||
$matches = []; |
||||
|
||||
foreach ($this->_lines as $index => $line) |
||||
{ |
||||
if (preg_match('/^##([^#]+)/', trim($line), $match)) |
||||
{ |
||||
$matches[$index] = trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
} |
||||
|
||||
return $matches; |
||||
} |
||||
|
||||
public function getH3(): array |
||||
{ |
||||
$matches = []; |
||||
|
||||
foreach ($this->_lines as $index => $line) |
||||
{ |
||||
if (preg_match('/^###([^#]+)/', trim($line), $match)) |
||||
{ |
||||
$matches[$index] = trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
} |
||||
|
||||
return $matches; |
||||
} |
||||
|
||||
public function getLinks(): array |
||||
{ |
||||
$matches = []; |
||||
|
||||
foreach ($this->_lines as $index => $line) |
||||
{ |
||||
if (preg_match('/^=>(.*)/', trim($line), $match)) |
||||
{ |
||||
$matches[$index] = trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
} |
||||
|
||||
return $matches; |
||||
} |
||||
|
||||
public function getQuote(): array |
||||
{ |
||||
$matches = []; |
||||
|
||||
foreach ($this->_lines as $index => $line) |
||||
{ |
||||
if (preg_match('/^>(.*)/', trim($line), $match)) |
||||
{ |
||||
$matches[$index] = trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
} |
||||
|
||||
return $matches; |
||||
} |
||||
|
||||
public function getCode(): array |
||||
{ |
||||
$matches = []; |
||||
|
||||
foreach ($this->_lines as $index => $line) |
||||
{ |
||||
if (preg_match('/^```(.*)/', trim($line), $match)) |
||||
{ |
||||
$matches[$index] = empty($match[1]) ? null : trim($match[1]); |
||||
} |
||||
} |
||||
|
||||
return $matches; |
||||
} |
||||
|
||||
public function findLinks(string $protocol = 'gemini'): array |
||||
{ |
||||
$matches = []; |
||||
|
||||
foreach ($this->_lines as $index => $line) |
||||
{ |
||||
if (preg_match('/' . $protocol . ':\/\/(.*)[\s\S\'"]*/', trim($line), $match)) |
||||
{ |
||||
$matches[$index] = |
||||
sprintf( |
||||
'%s://%s', |
||||
$protocol, |
||||
trim( |
||||
$match[1] |
||||
) |
||||
); |
||||
} |
||||
} |
||||
|
||||
return $matches; |
||||
} |
||||
|
||||
public function skipTags(array $tags = []): string |
||||
{ |
||||
$lines = []; |
||||
|
||||
foreach ($this->_lines as $line) |
||||
{ |
||||
$line = trim( |
||||
$line |
||||
); |
||||
|
||||
if ($tags) |
||||
{ |
||||
foreach ($tags as $tag) |
||||
{ |
||||
if(!in_array($tag, ['#', '##', '###', '=>', '*', '```'])) |
||||
{ |
||||
continue; |
||||
} |
||||
|
||||
switch (true) |
||||
{ |
||||
case str_starts_with($line, '#'): |
||||
|
||||
$line = preg_replace( |
||||
sprintf( |
||||
'/^%s([^#]+)/ui', |
||||
$tag |
||||
), |
||||
'$1', |
||||
$line |
||||
); |
||||
|
||||
break; |
||||
|
||||
case str_starts_with($line, '*'): |
||||
|
||||
$line = preg_replace( |
||||
'/^\*(.*)/ui', |
||||
'$1', |
||||
$line |
||||
); |
||||
|
||||
break; |
||||
|
||||
default: |
||||
|
||||
$line = preg_replace( |
||||
sprintf( |
||||
'/^%s(.*)/ui', |
||||
$tag |
||||
), |
||||
'$1', |
||||
$line |
||||
); |
||||
} |
||||
} |
||||
} |
||||
|
||||
else |
||||
{ |
||||
$line = preg_replace( |
||||
[ |
||||
'/^#([^#]+)/ui', |
||||
'/^##([^#]+)/ui', |
||||
'/^###([^#]+)/ui', |
||||
'/^=>(.*)/ui', |
||||
'/^\*(.*)/ui', |
||||
'/^```(.*)/ui', |
||||
], |
||||
'$1', |
||||
$line |
||||
); |
||||
} |
||||
|
||||
$lines[] = trim( |
||||
$line |
||||
); |
||||
} |
||||
|
||||
return implode( |
||||
PHP_EOL, |
||||
$lines |
||||
); |
||||
} |
||||
} |
@ -1,69 +0,0 @@
@@ -1,69 +0,0 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Gemini\Gemtext; |
||||
|
||||
class Link |
||||
{ |
||||
private string $_line; |
||||
|
||||
public function __construct(string $line) |
||||
{ |
||||
$this->_line = preg_replace( |
||||
'/^\s*=>(.*)/', |
||||
'$1', |
||||
trim( |
||||
$line |
||||
) |
||||
); |
||||
} |
||||
|
||||
public function getAddress(): ?string |
||||
{ |
||||
if (preg_match('/^\s*([^\s]+)/', trim($this->_line), $match)) |
||||
{ |
||||
return trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
|
||||
return null; |
||||
} |
||||
|
||||
public function getDate(?int &$timestamp = null): ?string |
||||
{ |
||||
if (preg_match('/\s([\d]+-[\d+]+-[\d]+)\s/', trim($this->_line), $match)) |
||||
{ |
||||
if ($result = strtotime($match[1])) |
||||
{ |
||||
$timestamp = $result; |
||||
|
||||
return trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
} |
||||
|
||||
return null; |
||||
} |
||||
|
||||
public function getAlt(): ?string |
||||
{ |
||||
if (preg_match('/\s[\d]+-[\d+]+-[\d]+\s(.*)$/', trim($this->_line), $match)) |
||||
{ |
||||
return trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
|
||||
else if (preg_match('/\s(.*)$/', trim($this->_line), $match)) |
||||
{ |
||||
return trim( |
||||
$match[1] |
||||
); |
||||
} |
||||
|
||||
return null; |
||||
} |
||||
} |
Loading…
Reference in new issue