mirror of https://github.com/YGGverse/Yoda.git
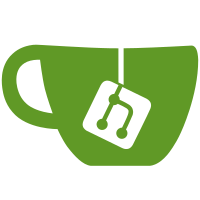
5 changed files with 233 additions and 5 deletions
@ -0,0 +1,76 @@
@@ -0,0 +1,76 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Yoda\Entity\Browser\Menu; |
||||
|
||||
use \GtkMenu; |
||||
use \GtkMenuItem; |
||||
|
||||
use \Yggverse\Yoda\Entity\Browser\Menu; |
||||
|
||||
class Help |
||||
{ |
||||
// GTK |
||||
public GtkMenuItem $gtk; |
||||
|
||||
// Dependencies |
||||
public Menu $menu; |
||||
|
||||
// Requirements |
||||
public Help\About $about; |
||||
public Help\Gemlog $gemlog; |
||||
public Help\Issue $issue; |
||||
|
||||
// Defaults |
||||
public const LABEL = 'Help'; |
||||
|
||||
public function __construct( |
||||
Menu $menu |
||||
) { |
||||
// Init dependencies |
||||
$this->menu = $menu; |
||||
|
||||
// Init menu item |
||||
$this->gtk = GtkMenuItem::new_with_label( |
||||
$this::LABEL |
||||
); |
||||
|
||||
// Init submenu container |
||||
$tab = new GtkMenu; |
||||
|
||||
// Init about menu item |
||||
$this->about = new Help\About( |
||||
$this |
||||
); |
||||
|
||||
$tab->append( |
||||
$this->about->gtk |
||||
); |
||||
|
||||
// Init gemlog menu item |
||||
$this->gemlog = new Help\Gemlog( |
||||
$this |
||||
); |
||||
|
||||
$tab->append( |
||||
$this->gemlog->gtk |
||||
); |
||||
|
||||
// Init issue menu item |
||||
$this->issue = new Help\Issue( |
||||
$this |
||||
); |
||||
|
||||
$tab->append( |
||||
$this->issue->gtk |
||||
); |
||||
|
||||
$this->gtk->set_submenu( |
||||
$tab |
||||
); |
||||
|
||||
// Render |
||||
$this->gtk->show(); |
||||
} |
||||
} |
@ -0,0 +1,46 @@
@@ -0,0 +1,46 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Yoda\Entity\Browser\Menu\Help; |
||||
|
||||
use \Gtk; |
||||
use \GtkMenuItem; |
||||
|
||||
use \Yggverse\Yoda\Entity\Browser\Menu\Help; |
||||
|
||||
class About |
||||
{ |
||||
// GTK |
||||
public GtkMenuItem $gtk; |
||||
|
||||
// Dependencies |
||||
public Help $help; |
||||
|
||||
// Defaults |
||||
public const LABEL = 'About'; |
||||
|
||||
public function __construct( |
||||
Help $help |
||||
) { |
||||
// Init dependencies |
||||
$this->help = $help; |
||||
|
||||
// Init menu item |
||||
$this->gtk = GtkMenuItem::new_with_label( |
||||
$this::LABEL |
||||
); |
||||
|
||||
// Render |
||||
$this->gtk->show(); |
||||
|
||||
// Int events |
||||
$this->gtk->connect( |
||||
'activate', |
||||
function() |
||||
{ |
||||
// @TODO |
||||
} |
||||
); |
||||
} |
||||
} |
@ -0,0 +1,49 @@
@@ -0,0 +1,49 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Yoda\Entity\Browser\Menu\Help; |
||||
|
||||
use \Gtk; |
||||
use \GtkMenuItem; |
||||
|
||||
use \Yggverse\Yoda\Entity\Browser\Menu\Help; |
||||
|
||||
class Gemlog |
||||
{ |
||||
// GTK |
||||
public GtkMenuItem $gtk; |
||||
|
||||
// Dependencies |
||||
public Help $help; |
||||
|
||||
// Defaults |
||||
public const LABEL = 'Gemlog'; |
||||
public const URL = 'gemini://yggverse.cities.yesterweb.org'; |
||||
|
||||
public function __construct( |
||||
Help $help |
||||
) { |
||||
// Init dependencies |
||||
$this->help = $help; |
||||
|
||||
// Init menu item |
||||
$this->gtk = GtkMenuItem::new_with_label( |
||||
$this::LABEL |
||||
); |
||||
|
||||
// Render |
||||
$this->gtk->show(); |
||||
|
||||
// Int events |
||||
$this->gtk->connect( |
||||
'activate', |
||||
function() |
||||
{ |
||||
$this->help->menu->browser->container->tab->append( |
||||
self::URL |
||||
); |
||||
} |
||||
); |
||||
} |
||||
} |
@ -0,0 +1,47 @@
@@ -0,0 +1,47 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Yoda\Entity\Browser\Menu\Help; |
||||
|
||||
use \Gtk; |
||||
use \GtkMenuItem; |
||||
|
||||
use \Yggverse\Yoda\Entity\Browser\Menu\Help; |
||||
|
||||
class Issue |
||||
{ |
||||
// GTK |
||||
public GtkMenuItem $gtk; |
||||
|
||||
// Dependencies |
||||
public Help $help; |
||||
|
||||
// Defaults |
||||
public const LABEL = 'Report issue'; |
||||
public const URL = 'https://github.com/YGGverse/Yoda'; |
||||
|
||||
public function __construct( |
||||
Help $help |
||||
) { |
||||
// Init dependencies |
||||
$this->help = $help; |
||||
|
||||
// Init menu item |
||||
$this->gtk = GtkMenuItem::new_with_label( |
||||
$this::LABEL |
||||
); |
||||
|
||||
// Render |
||||
$this->gtk->show(); |
||||
|
||||
// Int events |
||||
$this->gtk->connect( |
||||
'activate', |
||||
function() |
||||
{ |
||||
// @TODO self::URL |
||||
} |
||||
); |
||||
} |
||||
} |
Loading…
Reference in new issue