mirror of https://github.com/YGGverse/Yoda.git
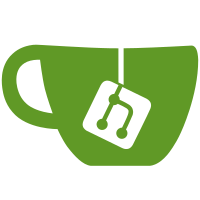
3 changed files with 195 additions and 0 deletions
@ -0,0 +1,77 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace Yggverse\Yoda\Abstract\Model; |
||||||
|
|
||||||
|
use \Exception; |
||||||
|
use \OpenSSLAsymmetricKey; |
||||||
|
use \OpenSSLCertificate; |
||||||
|
use \OpenSSLCertificateSigningRequest; |
||||||
|
|
||||||
|
abstract class Identity implements \Yggverse\Yoda\Interface\Model\Identity |
||||||
|
{ |
||||||
|
// Generate a new private key |
||||||
|
public static function new( |
||||||
|
int $bits = self::PRIVATE_KEY_BITS, |
||||||
|
int $type = self::PRIVATE_KEY_TYPE |
||||||
|
): OpenSSLAsymmetricKey |
||||||
|
{ |
||||||
|
$key = openssl_pkey_new( |
||||||
|
[ |
||||||
|
'private_key_bits' => $bits, |
||||||
|
'private_key_type' => $type |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
if ($key) |
||||||
|
{ |
||||||
|
return $key; |
||||||
|
} |
||||||
|
|
||||||
|
throw new Exception; |
||||||
|
} |
||||||
|
|
||||||
|
// Generate a new certificate signing request (CSR) |
||||||
|
public static function csr( |
||||||
|
OpenSSLAsymmetricKey $key |
||||||
|
): OpenSSLCertificateSigningRequest |
||||||
|
{ |
||||||
|
$csr = openssl_csr_new( |
||||||
|
[ |
||||||
|
// 'commonName' => $commonName @TODO |
||||||
|
], |
||||||
|
$key |
||||||
|
); |
||||||
|
|
||||||
|
if ($csr) |
||||||
|
{ |
||||||
|
return $csr; |
||||||
|
} |
||||||
|
|
||||||
|
throw new Exception; |
||||||
|
} |
||||||
|
|
||||||
|
// Sign the CSR |
||||||
|
public static function sign( |
||||||
|
OpenSSLCertificateSigningRequest $csr, |
||||||
|
OpenSSLCertificate|OpenSSLAsymmetricKey|array|string $key, |
||||||
|
OpenSSLCertificate|string|null $crt = null, // self-signed |
||||||
|
int $days = self::CSR_SIGN_DAYS |
||||||
|
): OpenSSLCertificate |
||||||
|
{ |
||||||
|
$x509 = openssl_csr_sign( |
||||||
|
$csr, |
||||||
|
$crt, |
||||||
|
$key, |
||||||
|
$days |
||||||
|
); |
||||||
|
|
||||||
|
if ($x509) |
||||||
|
{ |
||||||
|
return $x509; |
||||||
|
} |
||||||
|
|
||||||
|
throw new Exception; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,40 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace Yggverse\Yoda\Interface\Model; |
||||||
|
|
||||||
|
use \OpenSSLAsymmetricKey; |
||||||
|
use \OpenSSLCertificate; |
||||||
|
use \OpenSSLCertificateSigningRequest; |
||||||
|
|
||||||
|
/* |
||||||
|
* Certificate-based Identity API |
||||||
|
* |
||||||
|
*/ |
||||||
|
interface Identity |
||||||
|
{ |
||||||
|
public const CSR_SIGN_DAYS = 365; |
||||||
|
|
||||||
|
public const PRIVATE_KEY_BITS = 2048; |
||||||
|
public const PRIVATE_KEY_TYPE = OPENSSL_KEYTYPE_RSA; |
||||||
|
|
||||||
|
// Generate new private key |
||||||
|
public static function new( |
||||||
|
int $bits = self::PRIVATE_KEY_BITS, |
||||||
|
int $type = self::PRIVATE_KEY_TYPE |
||||||
|
): OpenSSLAsymmetricKey; |
||||||
|
|
||||||
|
// Generate certificate signing request (CSR) |
||||||
|
public static function csr( |
||||||
|
OpenSSLAsymmetricKey $key |
||||||
|
): OpenSSLCertificateSigningRequest; |
||||||
|
|
||||||
|
// Sign the CSR |
||||||
|
public static function sign( |
||||||
|
OpenSSLCertificateSigningRequest $csr, |
||||||
|
OpenSSLCertificate|OpenSSLAsymmetricKey|array|string $key, |
||||||
|
OpenSSLCertificate|string|null $crt = null, // self-signed |
||||||
|
int $days = self::CSR_SIGN_DAYS |
||||||
|
): OpenSSLCertificate; |
||||||
|
} |
@ -0,0 +1,78 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace Yggverse\Yoda\Model\Identity; |
||||||
|
|
||||||
|
use \Exception; |
||||||
|
use \OpenSSLAsymmetricKey; |
||||||
|
use \OpenSSLCertificate; |
||||||
|
|
||||||
|
class Gemini extends \Yggverse\Yoda\Abstract\Model\Identity |
||||||
|
{ |
||||||
|
// Update defaults |
||||||
|
public const CSR_SIGN_DAYS = 365 * 1965; // @TODO |
||||||
|
|
||||||
|
// Init identity variables |
||||||
|
protected OpenSSLAsymmetricKey $_key; |
||||||
|
protected OpenSSLCertificate $_crt; |
||||||
|
|
||||||
|
// Init new identity |
||||||
|
public function __construct( |
||||||
|
?OpenSSLAsymmetricKey $key = null, |
||||||
|
?OpenSSLCertificate $crt = null |
||||||
|
) { |
||||||
|
// Init private key |
||||||
|
$this->_key = $key ? $key : self::new(); |
||||||
|
|
||||||
|
// Init self-signed certificate |
||||||
|
$this->_crt = $crt ? $crt : self::sign( |
||||||
|
self::csr( |
||||||
|
$this->_key |
||||||
|
), |
||||||
|
$this->_key, |
||||||
|
null, |
||||||
|
self::CSR_SIGN_DAYS |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
// Get certificate |
||||||
|
public function crt( |
||||||
|
?OpenSSLCertificate $crt = null |
||||||
|
): string |
||||||
|
{ |
||||||
|
$pem = ''; |
||||||
|
|
||||||
|
$result = openssl_x509_export( |
||||||
|
$crt ? $crt : $this->_crt, |
||||||
|
$pem |
||||||
|
); |
||||||
|
|
||||||
|
if ($result) |
||||||
|
{ |
||||||
|
return $pem; |
||||||
|
} |
||||||
|
|
||||||
|
throw new Exception; |
||||||
|
} |
||||||
|
|
||||||
|
// Get private key |
||||||
|
public function key( |
||||||
|
?OpenSSLAsymmetricKey $key = null |
||||||
|
): string |
||||||
|
{ |
||||||
|
$pem = ''; |
||||||
|
|
||||||
|
$result = openssl_pkey_export( |
||||||
|
$key ? $key : $this->_key, |
||||||
|
$pem |
||||||
|
); |
||||||
|
|
||||||
|
if ($result) |
||||||
|
{ |
||||||
|
return $pem; |
||||||
|
} |
||||||
|
|
||||||
|
throw new Exception; |
||||||
|
} |
||||||
|
} |
Loading…
Reference in new issue