mirror of https://github.com/YGGverse/Yoda.git
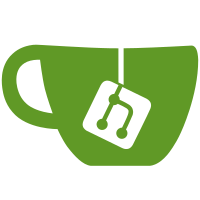
7 changed files with 70 additions and 134 deletions
@ -1,46 +0,0 @@
@@ -1,46 +0,0 @@
|
||||
mod description; |
||||
mod title; |
||||
|
||||
use description::Description; |
||||
use title::Title; |
||||
|
||||
use gtk::{glib::GString, prelude::BoxExt, Align, Box, Orientation}; |
||||
|
||||
pub struct Subject { |
||||
gobject: Box, |
||||
title: Title, |
||||
description: Description, |
||||
} |
||||
|
||||
impl Subject { |
||||
// Construct
|
||||
pub fn new() -> Self { |
||||
let title = Title::new(); |
||||
let description = Description::new(); |
||||
|
||||
let gobject = Box::builder() |
||||
.orientation(Orientation::Vertical) |
||||
.valign(Align::Center) |
||||
.build(); |
||||
|
||||
gobject.append(title.gobject()); |
||||
gobject.append(description.gobject()); |
||||
|
||||
Self { |
||||
gobject, |
||||
title, |
||||
description, |
||||
} |
||||
} |
||||
|
||||
// Actions
|
||||
pub fn update(&self, title: Option<GString>, description: Option<GString>) { |
||||
self.title.update(title); |
||||
self.description.update(description); |
||||
} |
||||
|
||||
// Getters
|
||||
pub fn gobject(&self) -> &Box { |
||||
&self.gobject |
||||
} |
||||
} |
@ -1,35 +0,0 @@
@@ -1,35 +0,0 @@
|
||||
use gtk::glib::GString; |
||||
use gtk::prelude::WidgetExt; |
||||
use gtk::{pango::EllipsizeMode, Label}; |
||||
|
||||
pub struct Description { |
||||
gobject: Label, |
||||
} |
||||
|
||||
impl Description { |
||||
// Construct
|
||||
pub fn new() -> Self { |
||||
let gobject = Label::builder() |
||||
.css_classes(["subtitle"]) |
||||
.single_line_mode(true) |
||||
.ellipsize(EllipsizeMode::End) |
||||
.visible(false) |
||||
.build(); |
||||
|
||||
Self { gobject } |
||||
} |
||||
|
||||
// Actions
|
||||
pub fn update(&self, text: Option<GString>) { |
||||
match text { |
||||
Some(value) => self.gobject.set_text(&value), |
||||
None => self.gobject.set_text(""), // @TODO
|
||||
}; |
||||
self.gobject.set_visible(!self.gobject.text().is_empty()); |
||||
} |
||||
|
||||
// Getters
|
||||
pub fn gobject(&self) -> &Label { |
||||
&self.gobject |
||||
} |
||||
} |
@ -1,41 +0,0 @@
@@ -1,41 +0,0 @@
|
||||
use gtk::{glib::GString, pango::EllipsizeMode, Label}; |
||||
|
||||
const DEFAULT_TEXT: &str = "Yoda"; // @TODO
|
||||
|
||||
pub struct Title { |
||||
gobject: Label, |
||||
} |
||||
|
||||
impl Title { |
||||
// Construct
|
||||
pub fn new() -> Self { |
||||
let gobject = gtk::Label::builder() |
||||
.css_classes(["title"]) |
||||
.single_line_mode(true) |
||||
.ellipsize(EllipsizeMode::End) |
||||
.label(DEFAULT_TEXT) |
||||
.build(); |
||||
|
||||
Self { gobject } |
||||
} |
||||
|
||||
// Actions
|
||||
pub fn update(&self, text: Option<GString>) { |
||||
let mut name = Vec::new(); |
||||
|
||||
if let Some(value) = text { |
||||
if !value.is_empty() { |
||||
name.push(value); |
||||
} |
||||
} |
||||
|
||||
name.push(GString::from(DEFAULT_TEXT)); |
||||
|
||||
self.gobject.set_text(&name.join(" - ")); |
||||
} |
||||
|
||||
// Getters
|
||||
pub fn gobject(&self) -> &Label { |
||||
&self.gobject |
||||
} |
||||
} |
@ -0,0 +1,44 @@
@@ -0,0 +1,44 @@
|
||||
use adw::WindowTitle; |
||||
|
||||
const DEFAULT_TITLE: &str = "Yoda"; // @TODO
|
||||
const DEFAULT_SUBTITLE: &str = ""; |
||||
|
||||
pub struct Title { |
||||
gobject: WindowTitle, |
||||
} |
||||
|
||||
impl Title { |
||||
// Construct
|
||||
pub fn new() -> Self { |
||||
Self { |
||||
gobject: WindowTitle::new(DEFAULT_TITLE, DEFAULT_SUBTITLE), |
||||
} |
||||
} |
||||
|
||||
// Actions
|
||||
pub fn update(&self, title: Option<&str>, subtitle: Option<&str>) { |
||||
// Update title
|
||||
let mut name = Vec::new(); |
||||
|
||||
if let Some(value) = title { |
||||
if !value.is_empty() { |
||||
name.push(value); |
||||
} |
||||
} |
||||
|
||||
name.push(DEFAULT_TITLE); |
||||
|
||||
self.gobject.set_title(&name.join(" - ")); |
||||
|
||||
// Update subtitle
|
||||
self.gobject.set_subtitle(&match subtitle { |
||||
Some(value) => value, |
||||
None => "", // @TODO
|
||||
}); |
||||
} |
||||
|
||||
// Getters
|
||||
pub fn gobject(&self) -> &WindowTitle { |
||||
&self.gobject |
||||
} |
||||
} |
Loading…
Reference in new issue