mirror of https://github.com/YGGverse/Yoda.git
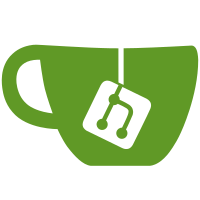
5 changed files with 111 additions and 62 deletions
@ -1,46 +1,30 @@
@@ -1,46 +1,30 @@
|
||||
mod action; |
||||
mod app; |
||||
mod profile; |
||||
|
||||
use crate::profile::Profile; |
||||
use app::App; |
||||
use gtk::glib::{user_config_dir, ExitCode}; |
||||
use sqlite::Connection; |
||||
use std::{fs::create_dir_all, rc::Rc, sync::RwLock}; |
||||
use gtk::glib::ExitCode; |
||||
use std::rc::Rc; |
||||
|
||||
const VENDOR: &str = "YGGverse"; |
||||
const APP_ID: &str = "Yoda"; // env!("CARGO_PKG_NAME");
|
||||
const APP_ID: &str = "Yoda"; |
||||
const BRANCH: &str = "master"; |
||||
|
||||
fn main() -> ExitCode { |
||||
// Init profile path
|
||||
let mut profile_path = user_config_dir(); |
||||
|
||||
profile_path.push(VENDOR); |
||||
profile_path.push(APP_ID); |
||||
profile_path.push(BRANCH); |
||||
profile_path.push(format!( |
||||
match gtk::init() { |
||||
Ok(_) => App::new(Rc::new(Profile::new( |
||||
VENDOR, |
||||
APP_ID, |
||||
BRANCH, |
||||
format!( |
||||
"{}.{}", |
||||
env!("CARGO_PKG_VERSION_MAJOR"), |
||||
env!("CARGO_PKG_VERSION_MINOR") |
||||
)); // @TODO remove after auto-migrate feature implementation
|
||||
|
||||
if let Err(e) = create_dir_all(&profile_path) { |
||||
panic!("Failed to create profile directory: {e}") |
||||
} |
||||
|
||||
// Init profile database path
|
||||
let mut profile_database_path = profile_path.clone(); |
||||
|
||||
profile_database_path.push("profile.sqlite3"); |
||||
|
||||
// Init database connection
|
||||
let profile_database_connection = match Connection::open(profile_database_path) { |
||||
Ok(connection) => Rc::new(RwLock::new(connection)), |
||||
Err(e) => panic!("Failed to connect profile database: {e}"), |
||||
}; |
||||
|
||||
// Init GTK, start application
|
||||
match gtk::init() { |
||||
Ok(_) => App::new(profile_database_connection, profile_path).run(), // @TODO common struct for profile data
|
||||
) |
||||
.as_str(), |
||||
))) |
||||
.run(), |
||||
Err(_) => ExitCode::FAILURE, |
||||
} |
||||
} |
||||
|
@ -0,0 +1,53 @@
@@ -0,0 +1,53 @@
|
||||
mod database; |
||||
pub use database::Database; |
||||
|
||||
use gtk::glib::user_config_dir; |
||||
use std::{ |
||||
fs::create_dir_all, |
||||
path::{Path, PathBuf}, |
||||
}; |
||||
|
||||
const DB_NAME: &str = "profile.sqlite3"; |
||||
|
||||
pub struct Profile { |
||||
database: Database, |
||||
config_path: PathBuf, |
||||
} |
||||
|
||||
impl Profile { |
||||
// Constructors
|
||||
|
||||
pub fn new(vendor: &str, app_id: &str, branch: &str, version: &str) -> Self { |
||||
// Init profile path
|
||||
let mut config_path = user_config_dir(); |
||||
|
||||
config_path.push(vendor); |
||||
config_path.push(app_id); |
||||
config_path.push(branch); |
||||
config_path.push(version); // @TODO remove after auto-migrate feature implementation
|
||||
|
||||
if let Err(e) = create_dir_all(&config_path) { |
||||
panic!("Failed to create profile directory: {e}") |
||||
} |
||||
|
||||
// Init database path
|
||||
let mut database_path = config_path.clone(); |
||||
database_path.push(DB_NAME); |
||||
|
||||
// Result
|
||||
Self { |
||||
database: Database::new(database_path.as_path()), |
||||
config_path, |
||||
} |
||||
} |
||||
|
||||
// Getters
|
||||
|
||||
pub fn database(&self) -> &Database { |
||||
&self.database |
||||
} |
||||
|
||||
pub fn config_path(&self) -> &Path { |
||||
self.config_path.as_path() |
||||
} |
||||
} |
@ -0,0 +1,25 @@
@@ -0,0 +1,25 @@
|
||||
use sqlite::Connection; |
||||
use std::{path::Path, rc::Rc, sync::RwLock}; |
||||
|
||||
pub struct Database { |
||||
connection: Rc<RwLock<Connection>>, |
||||
} |
||||
|
||||
impl Database { |
||||
// Constructors
|
||||
|
||||
pub fn new(path: &Path) -> Self { |
||||
Self { |
||||
connection: match Connection::open(path) { |
||||
Ok(connection) => Rc::new(RwLock::new(connection)), |
||||
Err(e) => panic!("Failed to connect profile database: {e}"), |
||||
}, |
||||
} |
||||
} |
||||
|
||||
// Getters
|
||||
|
||||
pub fn connection(&self) -> &Rc<RwLock<Connection>> { |
||||
&self.connection |
||||
} |
||||
} |
Loading…
Reference in new issue