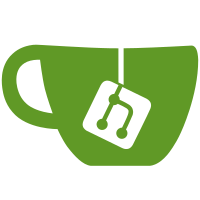
27 changed files with 1486 additions and 137 deletions
@ -0,0 +1,620 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Controller; |
||||||
|
|
||||||
|
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; |
||||||
|
|
||||||
|
use Symfony\Component\Routing\Annotation\Route; |
||||||
|
use Symfony\Component\HttpFoundation\Response; |
||||||
|
use Symfony\Component\HttpFoundation\Request; |
||||||
|
|
||||||
|
use App\Service\ActivityService; |
||||||
|
use App\Service\UserService; |
||||||
|
use App\Service\ArticleService; |
||||||
|
use App\Service\TorrentService; |
||||||
|
|
||||||
|
class ActivityController extends AbstractController |
||||||
|
{ |
||||||
|
public function template( |
||||||
|
$activity, |
||||||
|
ActivityService $activityService, |
||||||
|
UserService $userService, |
||||||
|
ArticleService $articleService, |
||||||
|
TorrentService $torrentService, |
||||||
|
): Response |
||||||
|
{ |
||||||
|
switch ($activity->getEvent()) |
||||||
|
{ |
||||||
|
// User |
||||||
|
case $activity::EVENT_USER_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_APPROVE_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/approve/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_APPROVE_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/approve/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_MODERATOR_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/moderator/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_MODERATOR_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/moderator/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_STATUS_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/status/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_STATUS_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/status/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_STAR_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/star/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_USER_STAR_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/user/star/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'by' => |
||||||
|
[ |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getData()['userId'], |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getData()['userId'] |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
// Torrent |
||||||
|
case $activity::EVENT_TORRENT_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName() |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
/// Torrent Locales |
||||||
|
case $activity::EVENT_TORRENT_LOCALES_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/locales/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'locales' => [ |
||||||
|
'id' => $activity->getData()['torrentLocalesId'], |
||||||
|
'exist' => $torrentService->getTorrentLocales( |
||||||
|
$activity->getData()['torrentLocalesId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_TORRENT_LOCALES_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/locales/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'locales' => [ |
||||||
|
'id' => $activity->getData()['torrentLocalesId'], |
||||||
|
'exist' => $torrentService->getTorrentLocales( |
||||||
|
$activity->getData()['torrentLocalesId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_TORRENT_LOCALES_APPROVE_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/locales/approve/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'locales' => [ |
||||||
|
'id' => $activity->getData()['torrentLocalesId'], |
||||||
|
'exist' => $torrentService->getTorrentLocales( |
||||||
|
$activity->getData()['torrentLocalesId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_TORRENT_LOCALES_APPROVE_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/locales/approve/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'locales' => [ |
||||||
|
'id' => $activity->getData()['torrentLocalesId'], |
||||||
|
'exist' => $torrentService->getTorrentLocales( |
||||||
|
$activity->getData()['torrentLocalesId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
/// Torrent Sensitive |
||||||
|
case $activity::EVENT_TORRENT_SENSITIVE_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/sensitive/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'sensitive' => [ |
||||||
|
'id' => $activity->getData()['torrentSensitiveId'], |
||||||
|
'exist' => $torrentService->getTorrentSensitive( |
||||||
|
$activity->getData()['torrentSensitiveId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_TORRENT_SENSITIVE_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/sensitive/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'sensitive' => [ |
||||||
|
'id' => $activity->getData()['torrentSensitiveId'], |
||||||
|
'exist' => $torrentService->getTorrentSensitive( |
||||||
|
$activity->getData()['torrentSensitiveId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_TORRENT_SENSITIVE_APPROVE_ADD: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/sensitive/approve/add.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'sensitive' => [ |
||||||
|
'id' => $activity->getData()['torrentSensitiveId'], |
||||||
|
'exist' => $torrentService->getTorrentSensitive( |
||||||
|
$activity->getData()['torrentSensitiveId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
case $activity::EVENT_TORRENT_SENSITIVE_APPROVE_DELETE: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/torrent/sensitive/approve/delete.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
], |
||||||
|
'torrent' => |
||||||
|
[ |
||||||
|
'id' => $activity->getTorrentId(), |
||||||
|
'name' => $torrentService->readTorrentFileByTorrentId( |
||||||
|
$activity->getTorrentId() |
||||||
|
)->getName(), |
||||||
|
'sensitive' => [ |
||||||
|
'id' => $activity->getData()['torrentSensitiveId'], |
||||||
|
'exist' => $torrentService->getTorrentSensitive( |
||||||
|
$activity->getData()['torrentSensitiveId'] // could be deleted by moderator, remove links |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
|
||||||
|
break; |
||||||
|
|
||||||
|
// Page |
||||||
|
|
||||||
|
default: |
||||||
|
|
||||||
|
return $this->render( |
||||||
|
'default/activity/event/undefined.html.twig', |
||||||
|
[ |
||||||
|
'added' => $activity->getAdded(), |
||||||
|
'user' => |
||||||
|
[ |
||||||
|
'id' => $activity->getUserId(), |
||||||
|
'identicon' => $userService->identicon( |
||||||
|
$userService->getUser( |
||||||
|
$activity->getUserId() |
||||||
|
)->getAddress() |
||||||
|
) |
||||||
|
] |
||||||
|
] |
||||||
|
); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'added torrent' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'added locales edition' | trans }} |
||||||
|
{% if torrent.locales.exist %} |
||||||
|
<a href="{{ path('torrent_locales_edit', { torrentId : torrent.id, torrentLocalesId : torrent.locales.id }) }}"> |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'approved locales edition' | trans }} |
||||||
|
{% if torrent.locales.exist %} |
||||||
|
<a href="{{ path('torrent_locales_edit', { torrentId : torrent.id, torrentLocalesId : torrent.locales.id }) }}"> |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'disapproved locales edition' | trans }} |
||||||
|
{% if torrent.locales.exist %} |
||||||
|
<a href="{{ path('torrent_locales_edit', { torrentId : torrent.id, torrentLocalesId : torrent.locales.id }) }}"> |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'deleted locales edition' | trans }} |
||||||
|
{% if torrent.locales.exist %} |
||||||
|
<a href="{{ path('torrent_locales_edit', { torrentId : torrent.id, torrentLocalesId : torrent.locales.id }) }}"> |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.locales.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'added sensitive edition' | trans }} |
||||||
|
{% if torrent.sensitive.exist %} |
||||||
|
<a href="{{ path('torrent_sensitive_edit', { torrentId : torrent.id, torrentSensitiveId : torrent.sensitive.id }) }}"> |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'approved sensitive edition' | trans }} |
||||||
|
{% if torrent.sensitive.exist %} |
||||||
|
<a href="{{ path('torrent_sensitive_edit', { torrentId : torrent.id, torrentSensitiveId : torrent.sensitive.id }) }}"> |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'disapproved sensitive edition' | trans }} |
||||||
|
{% if torrent.sensitive.exist %} |
||||||
|
<a href="{{ path('torrent_sensitive_edit', { torrentId : torrent.id, torrentSensitiveId : torrent.sensitive.id }) }}"> |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,18 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
{{ 'deleted sensitive edition' | trans }} |
||||||
|
{% if torrent.sensitive.exist %} |
||||||
|
<a href="{{ path('torrent_sensitive_edit', { torrentId : torrent.id, torrentSensitiveId : torrent.sensitive.id }) }}"> |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
</a> |
||||||
|
{% else %} |
||||||
|
#{{ torrent.sensitive.id }} |
||||||
|
{% endif %} |
||||||
|
{{ 'for torrent' | trans }} |
||||||
|
<a href="{{ path('torrent_info', { torrentId : torrent.id }) }}"> |
||||||
|
{{ torrent.name }} |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,9 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'undefined event' | trans }} |
||||||
|
</span> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,9 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'joined' | trans }} {{ name }} |
||||||
|
</span> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'approved by' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'disapproved by' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'grant moderator permissions to' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'remove moderator permissions from' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'add star to' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'removed star from' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'enable user' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -0,0 +1,12 @@ |
|||||||
|
<a href="{{ path('user_info', { userId : by.user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ by.user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<span class="margin-x-4-px"> |
||||||
|
{{ 'diable user' | trans }} |
||||||
|
</span> |
||||||
|
<a href="{{ path('user_info', { userId : user.id }) }}"> |
||||||
|
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ user.identicon }}" alt="{{ 'identicon' | trans }}" /> |
||||||
|
</a> |
||||||
|
<div class="float-right"> |
||||||
|
{{ added | format_ago }} |
||||||
|
</div> |
@ -1,23 +1,12 @@ |
|||||||
{% extends 'default/layout.html.twig' %} |
{% extends 'default/layout.html.twig' %} |
||||||
{% block title %}{{ 'Last activity'|trans }} - {{ name }}{% endblock %} |
{% block title %}{{ 'Activity' | trans }} - {{ name }}{% endblock %} |
||||||
{% block main_content %} |
{% block main_content %} |
||||||
{% for activity in activities %} |
{% for activity in activities %} |
||||||
<div class="padding-16-px margin-y-8-px border-radius-3-px background-color-night"> |
<div class="padding-16-px margin-y-8-px border-radius-3-px background-color-night"> |
||||||
<div class="row"> |
{{ render(controller( |
||||||
<div class="column width-80"> |
'App\\Controller\\ActivityController::template', |
||||||
<a href="{{ path('user_info', { userId : activity.user.id }) }}"> |
{ activity : activity } |
||||||
<img class="vertical-align-middle border-radius-50 border-color-default border-width-2-px margin-r-8-px" |
)) }} |
||||||
src="{{ activity.user.identicon }}" |
|
||||||
alt="{{ 'identicon'|trans }}" /> |
|
||||||
</a> |
|
||||||
{% if activity.type == 'join' %} |
|
||||||
{{ 'joined'|trans }} {{ name }} |
|
||||||
{% endif %} |
|
||||||
</div> |
|
||||||
<div class="column width-20 text-right padding-y-4-px"> |
|
||||||
{{ activity.added }} |
|
||||||
</div> |
|
||||||
</div> |
|
||||||
</div> |
</div> |
||||||
{% endfor %} |
{% endfor %} |
||||||
{% endblock %} |
{% endblock %} |
Loading…
Reference in new issue