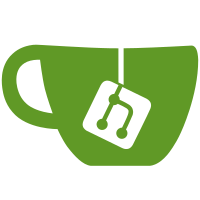
43 changed files with 597 additions and 597 deletions
@ -0,0 +1,23 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Repository; |
||||||
|
|
||||||
|
use App\Entity\ArticleDescription; |
||||||
|
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||||
|
use Doctrine\Persistence\ManagerRegistry; |
||||||
|
|
||||||
|
/** |
||||||
|
* @extends ServiceEntityRepository<ArticleDescription> |
||||||
|
* |
||||||
|
* @method ArticleDescription|null find($id, $lockMode = null, $lockVersion = null) |
||||||
|
* @method ArticleDescription|null findOneBy(array $criteria, array $orderBy = null) |
||||||
|
* @method ArticleDescription[] findAll() |
||||||
|
* @method ArticleDescription[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||||
|
*/ |
||||||
|
class ArticleDescriptionRepository extends ServiceEntityRepository |
||||||
|
{ |
||||||
|
public function __construct(ManagerRegistry $registry) |
||||||
|
{ |
||||||
|
parent::__construct($registry, ArticleDescription::class); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Repository; |
||||||
|
|
||||||
|
use App\Entity\Article; |
||||||
|
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||||
|
use Doctrine\Persistence\ManagerRegistry; |
||||||
|
|
||||||
|
/** |
||||||
|
* @extends ServiceEntityRepository<Article> |
||||||
|
* |
||||||
|
* @method Article|null find($id, $lockMode = null, $lockVersion = null) |
||||||
|
* @method Article|null findOneBy(array $criteria, array $orderBy = null) |
||||||
|
* @method Article[] findAll() |
||||||
|
* @method Article[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||||
|
*/ |
||||||
|
class ArticleRepository extends ServiceEntityRepository |
||||||
|
{ |
||||||
|
public function __construct(ManagerRegistry $registry) |
||||||
|
{ |
||||||
|
parent::__construct($registry, Article::class); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Repository; |
||||||
|
|
||||||
|
use App\Entity\ArticleSensitive; |
||||||
|
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||||
|
use Doctrine\Persistence\ManagerRegistry; |
||||||
|
|
||||||
|
/** |
||||||
|
* @extends ServiceEntityRepository<ArticleSensitive> |
||||||
|
* |
||||||
|
* @method ArticleSensitive|null find($id, $lockMode = null, $lockVersion = null) |
||||||
|
* @method ArticleSensitive|null findOneBy(array $criteria, array $orderBy = null) |
||||||
|
* @method ArticleSensitive[] findAll() |
||||||
|
* @method ArticleSensitive[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||||
|
*/ |
||||||
|
class ArticleSensitiveRepository extends ServiceEntityRepository |
||||||
|
{ |
||||||
|
public function __construct(ManagerRegistry $registry) |
||||||
|
{ |
||||||
|
parent::__construct($registry, ArticleSensitive::class); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Repository; |
||||||
|
|
||||||
|
use App\Entity\ArticleTitle; |
||||||
|
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||||
|
use Doctrine\Persistence\ManagerRegistry; |
||||||
|
|
||||||
|
/** |
||||||
|
* @extends ServiceEntityRepository<ArticleTitle> |
||||||
|
* |
||||||
|
* @method ArticleTitle|null find($id, $lockMode = null, $lockVersion = null) |
||||||
|
* @method ArticleTitle|null findOneBy(array $criteria, array $orderBy = null) |
||||||
|
* @method ArticleTitle[] findAll() |
||||||
|
* @method ArticleTitle[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||||
|
*/ |
||||||
|
class ArticleTitleRepository extends ServiceEntityRepository |
||||||
|
{ |
||||||
|
public function __construct(ManagerRegistry $registry) |
||||||
|
{ |
||||||
|
parent::__construct($registry, ArticleTitle::class); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Repository; |
||||||
|
|
||||||
|
use App\Entity\ArticleTorrents; |
||||||
|
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||||
|
use Doctrine\Persistence\ManagerRegistry; |
||||||
|
|
||||||
|
/** |
||||||
|
* @extends ServiceEntityRepository<ArticleTorrents> |
||||||
|
* |
||||||
|
* @method ArticleTorrents|null find($id, $lockMode = null, $lockVersion = null) |
||||||
|
* @method ArticleTorrents|null findOneBy(array $criteria, array $orderBy = null) |
||||||
|
* @method ArticleTorrents[] findAll() |
||||||
|
* @method ArticleTorrents[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||||
|
*/ |
||||||
|
class ArticleTorrentsRepository extends ServiceEntityRepository |
||||||
|
{ |
||||||
|
public function __construct(ManagerRegistry $registry) |
||||||
|
{ |
||||||
|
parent::__construct($registry, ArticleTorrents::class); |
||||||
|
} |
||||||
|
} |
@ -1,23 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace App\Repository; |
|
||||||
|
|
||||||
use App\Entity\PageDescription; |
|
||||||
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
|
||||||
use Doctrine\Persistence\ManagerRegistry; |
|
||||||
|
|
||||||
/** |
|
||||||
* @extends ServiceEntityRepository<PageDescription> |
|
||||||
* |
|
||||||
* @method PageDescription|null find($id, $lockMode = null, $lockVersion = null) |
|
||||||
* @method PageDescription|null findOneBy(array $criteria, array $orderBy = null) |
|
||||||
* @method PageDescription[] findAll() |
|
||||||
* @method PageDescription[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
|
||||||
*/ |
|
||||||
class PageDescriptionRepository extends ServiceEntityRepository |
|
||||||
{ |
|
||||||
public function __construct(ManagerRegistry $registry) |
|
||||||
{ |
|
||||||
parent::__construct($registry, PageDescription::class); |
|
||||||
} |
|
||||||
} |
|
@ -1,23 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace App\Repository; |
|
||||||
|
|
||||||
use App\Entity\Page; |
|
||||||
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
|
||||||
use Doctrine\Persistence\ManagerRegistry; |
|
||||||
|
|
||||||
/** |
|
||||||
* @extends ServiceEntityRepository<Page> |
|
||||||
* |
|
||||||
* @method Page|null find($id, $lockMode = null, $lockVersion = null) |
|
||||||
* @method Page|null findOneBy(array $criteria, array $orderBy = null) |
|
||||||
* @method Page[] findAll() |
|
||||||
* @method Page[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
|
||||||
*/ |
|
||||||
class PageRepository extends ServiceEntityRepository |
|
||||||
{ |
|
||||||
public function __construct(ManagerRegistry $registry) |
|
||||||
{ |
|
||||||
parent::__construct($registry, Page::class); |
|
||||||
} |
|
||||||
} |
|
@ -1,23 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace App\Repository; |
|
||||||
|
|
||||||
use App\Entity\PageSensitive; |
|
||||||
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
|
||||||
use Doctrine\Persistence\ManagerRegistry; |
|
||||||
|
|
||||||
/** |
|
||||||
* @extends ServiceEntityRepository<PageSensitive> |
|
||||||
* |
|
||||||
* @method PageSensitive|null find($id, $lockMode = null, $lockVersion = null) |
|
||||||
* @method PageSensitive|null findOneBy(array $criteria, array $orderBy = null) |
|
||||||
* @method PageSensitive[] findAll() |
|
||||||
* @method PageSensitive[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
|
||||||
*/ |
|
||||||
class PageSensitiveRepository extends ServiceEntityRepository |
|
||||||
{ |
|
||||||
public function __construct(ManagerRegistry $registry) |
|
||||||
{ |
|
||||||
parent::__construct($registry, PageSensitive::class); |
|
||||||
} |
|
||||||
} |
|
@ -1,23 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace App\Repository; |
|
||||||
|
|
||||||
use App\Entity\PageTitle; |
|
||||||
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
|
||||||
use Doctrine\Persistence\ManagerRegistry; |
|
||||||
|
|
||||||
/** |
|
||||||
* @extends ServiceEntityRepository<PageTitle> |
|
||||||
* |
|
||||||
* @method PageTitle|null find($id, $lockMode = null, $lockVersion = null) |
|
||||||
* @method PageTitle|null findOneBy(array $criteria, array $orderBy = null) |
|
||||||
* @method PageTitle[] findAll() |
|
||||||
* @method PageTitle[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
|
||||||
*/ |
|
||||||
class PageTitleRepository extends ServiceEntityRepository |
|
||||||
{ |
|
||||||
public function __construct(ManagerRegistry $registry) |
|
||||||
{ |
|
||||||
parent::__construct($registry, PageTitle::class); |
|
||||||
} |
|
||||||
} |
|
@ -1,23 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace App\Repository; |
|
||||||
|
|
||||||
use App\Entity\PageTorrents; |
|
||||||
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
|
||||||
use Doctrine\Persistence\ManagerRegistry; |
|
||||||
|
|
||||||
/** |
|
||||||
* @extends ServiceEntityRepository<PageTorrents> |
|
||||||
* |
|
||||||
* @method PageTorrents|null find($id, $lockMode = null, $lockVersion = null) |
|
||||||
* @method PageTorrents|null findOneBy(array $criteria, array $orderBy = null) |
|
||||||
* @method PageTorrents[] findAll() |
|
||||||
* @method PageTorrents[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
|
||||||
*/ |
|
||||||
class PageTorrentsRepository extends ServiceEntityRepository |
|
||||||
{ |
|
||||||
public function __construct(ManagerRegistry $registry) |
|
||||||
{ |
|
||||||
parent::__construct($registry, PageTorrents::class); |
|
||||||
} |
|
||||||
} |
|
@ -0,0 +1,196 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Service; |
||||||
|
|
||||||
|
use App\Entity\Article; |
||||||
|
use App\Entity\ArticleTitle; |
||||||
|
use App\Entity\ArticleDescription; |
||||||
|
use App\Entity\ArticleTorrents; |
||||||
|
use App\Entity\ArticleSensitive; |
||||||
|
|
||||||
|
use App\Repository\ArticleRepository; |
||||||
|
use App\Repository\ArticleTitleRepository; |
||||||
|
use App\Repository\ArticleDescriptionRepository; |
||||||
|
use App\Repository\ArticleSensitiveRepository; |
||||||
|
use App\Repository\ArticleTorrentsRepository; |
||||||
|
|
||||||
|
use Doctrine\ORM\EntityManagerInterface; |
||||||
|
|
||||||
|
class ArticleService |
||||||
|
{ |
||||||
|
private EntityManagerInterface $entityManager; |
||||||
|
private ParameterBagInterface $parameterBagInterface; |
||||||
|
|
||||||
|
public function __construct( |
||||||
|
EntityManagerInterface $entityManager, |
||||||
|
) |
||||||
|
{ |
||||||
|
$this->entityManager = $entityManager; |
||||||
|
} |
||||||
|
|
||||||
|
public function submit( |
||||||
|
int $added, |
||||||
|
int $userId, |
||||||
|
string $locale, |
||||||
|
string $title, |
||||||
|
string $description, |
||||||
|
array $torrents, |
||||||
|
bool $sensitive, |
||||||
|
bool $approved |
||||||
|
): ?Article |
||||||
|
{ |
||||||
|
$article = $this->addArticle(); |
||||||
|
|
||||||
|
if (!empty($title)) |
||||||
|
{ |
||||||
|
$articleTitle = $this->addArticleTitle( |
||||||
|
$article->getId(), |
||||||
|
$userId, |
||||||
|
$added, |
||||||
|
$locale, |
||||||
|
$title, |
||||||
|
$approved |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
if (!empty($description)) |
||||||
|
{ |
||||||
|
$articleDescription = $this->addArticleDescription( |
||||||
|
$article->getId(), |
||||||
|
$userId, |
||||||
|
$added, |
||||||
|
$locale, |
||||||
|
$description, |
||||||
|
$approved |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
if (!empty($torrents)) |
||||||
|
{ |
||||||
|
$articleTorrents = $this->addArticleTorrents( |
||||||
|
$article->getId(), |
||||||
|
$userId, |
||||||
|
$added, |
||||||
|
$locale, |
||||||
|
$torrents, |
||||||
|
$approved |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
// @TODO |
||||||
|
$articleSensitive = $this->addArticleSensitive( |
||||||
|
$article->getId(), |
||||||
|
$userId, |
||||||
|
$added, |
||||||
|
$locale, |
||||||
|
$description, |
||||||
|
$approved |
||||||
|
); |
||||||
|
|
||||||
|
return $article; |
||||||
|
} |
||||||
|
|
||||||
|
public function addArticle(): ?Article |
||||||
|
{ |
||||||
|
$article = new Article(); |
||||||
|
|
||||||
|
$this->entityManager->persist($article); |
||||||
|
$this->entityManager->flush(); |
||||||
|
|
||||||
|
return $article; |
||||||
|
} |
||||||
|
|
||||||
|
public function addArticleTitle( |
||||||
|
int $articleId, |
||||||
|
int $userId, |
||||||
|
int $added, |
||||||
|
string $locale, |
||||||
|
string $value, |
||||||
|
bool $approved |
||||||
|
): ?ArticleTitle |
||||||
|
{ |
||||||
|
$articleTitle = new ArticleTitle(); |
||||||
|
|
||||||
|
$articleTitle->setArticleId($articleId); |
||||||
|
$articleTitle->setUserId($userId); |
||||||
|
$articleTitle->setLocale($locale); |
||||||
|
$articleTitle->setValue($value); |
||||||
|
$articleTitle->setAdded($added); |
||||||
|
$articleTitle->setApproved($approved); |
||||||
|
|
||||||
|
$this->entityManager->persist($articleTitle); |
||||||
|
$this->entityManager->flush(); |
||||||
|
|
||||||
|
return $articleTitle; |
||||||
|
} |
||||||
|
|
||||||
|
public function addArticleDescription( |
||||||
|
int $articleId, |
||||||
|
int $userId, |
||||||
|
int $added, |
||||||
|
string $locale, |
||||||
|
string $value, |
||||||
|
bool $approved |
||||||
|
): ?ArticleDescription |
||||||
|
{ |
||||||
|
$articleDescription = new ArticleDescription(); |
||||||
|
|
||||||
|
$articleDescription->setArticleId($articleId); |
||||||
|
$articleDescription->setUserId($userId); |
||||||
|
$articleDescription->setAdded($added); |
||||||
|
$articleDescription->setLocale($locale); |
||||||
|
$articleDescription->setValue($value); |
||||||
|
$articleDescription->setApproved($approved); |
||||||
|
|
||||||
|
$this->entityManager->persist($articleDescription); |
||||||
|
$this->entityManager->flush(); |
||||||
|
|
||||||
|
return $articleDescription; |
||||||
|
} |
||||||
|
|
||||||
|
public function addArticleTorrents( |
||||||
|
int $articleId, |
||||||
|
int $userId, |
||||||
|
int $added, |
||||||
|
array $torrentsId, |
||||||
|
bool $approved |
||||||
|
): ?ArticleTorrents |
||||||
|
{ |
||||||
|
$articleTorrents = new ArticleTorrents(); |
||||||
|
|
||||||
|
$articleTorrents->setArticleId($articleId); |
||||||
|
$articleTorrents->setUserId($userId); |
||||||
|
$articleTorrents->setAdded($added); |
||||||
|
$articleTorrents->setTorrentsId($torrentsId); |
||||||
|
$articleTorrents->setApproved($approved); |
||||||
|
|
||||||
|
$this->entityManager->persist($articleTorrents); |
||||||
|
$this->entityManager->flush(); |
||||||
|
|
||||||
|
return $articleTorrents; |
||||||
|
} |
||||||
|
|
||||||
|
public function addArticleSensitive( |
||||||
|
int $articleId, |
||||||
|
int $userId, |
||||||
|
int $added, |
||||||
|
string $locale, |
||||||
|
string $value, |
||||||
|
bool $approved |
||||||
|
): ?ArticleSensitive |
||||||
|
{ |
||||||
|
$articleSensitive = new ArticleSensitive(); |
||||||
|
|
||||||
|
$articleSensitive->setArticleId($articleId); |
||||||
|
$articleSensitive->setUserId($userId); |
||||||
|
$articleSensitive->setAdded($added); |
||||||
|
$articleSensitive->setLocale($locale); |
||||||
|
$articleSensitive->setValue($value); |
||||||
|
$articleSensitive->setApproved($approved); |
||||||
|
|
||||||
|
$this->entityManager->persist($articleSensitive); |
||||||
|
$this->entityManager->flush(); |
||||||
|
|
||||||
|
return $articleSensitive; |
||||||
|
} |
||||||
|
} |
@ -1,196 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace App\Service; |
|
||||||
|
|
||||||
use App\Entity\Page; |
|
||||||
use App\Entity\PageTitle; |
|
||||||
use App\Entity\PageDescription; |
|
||||||
use App\Entity\PageTorrents; |
|
||||||
use App\Entity\PageSensitive; |
|
||||||
|
|
||||||
use App\Repository\PageRepository; |
|
||||||
use App\Repository\PageTitleRepository; |
|
||||||
use App\Repository\PageDescriptionRepository; |
|
||||||
use App\Repository\PageSensitiveRepository; |
|
||||||
use App\Repository\PageTorrentsRepository; |
|
||||||
|
|
||||||
use Doctrine\ORM\EntityManagerInterface; |
|
||||||
|
|
||||||
class PageService |
|
||||||
{ |
|
||||||
private EntityManagerInterface $entityManager; |
|
||||||
private ParameterBagInterface $parameterBagInterface; |
|
||||||
|
|
||||||
public function __construct( |
|
||||||
EntityManagerInterface $entityManager, |
|
||||||
) |
|
||||||
{ |
|
||||||
$this->entityManager = $entityManager; |
|
||||||
} |
|
||||||
|
|
||||||
public function submit( |
|
||||||
int $added, |
|
||||||
int $userId, |
|
||||||
string $locale, |
|
||||||
string $title, |
|
||||||
string $description, |
|
||||||
array $torrents, |
|
||||||
bool $sensitive, |
|
||||||
bool $approved |
|
||||||
): ?Page |
|
||||||
{ |
|
||||||
$page = $this->addPage(); |
|
||||||
|
|
||||||
if (!empty($title)) |
|
||||||
{ |
|
||||||
$pageTitle = $this->addPageTitle( |
|
||||||
$page->getId(), |
|
||||||
$userId, |
|
||||||
$added, |
|
||||||
$locale, |
|
||||||
$title, |
|
||||||
$approved |
|
||||||
); |
|
||||||
} |
|
||||||
|
|
||||||
if (!empty($description)) |
|
||||||
{ |
|
||||||
$pageDescription = $this->addPageDescription( |
|
||||||
$page->getId(), |
|
||||||
$userId, |
|
||||||
$added, |
|
||||||
$locale, |
|
||||||
$description, |
|
||||||
$approved |
|
||||||
); |
|
||||||
} |
|
||||||
|
|
||||||
if (!empty($torrents)) |
|
||||||
{ |
|
||||||
$pageTorrents = $this->addPageTorrents( |
|
||||||
$page->getId(), |
|
||||||
$userId, |
|
||||||
$added, |
|
||||||
$locale, |
|
||||||
$torrents, |
|
||||||
$approved |
|
||||||
); |
|
||||||
} |
|
||||||
|
|
||||||
// @TODO |
|
||||||
$pageSensitive = $this->addPageSensitive( |
|
||||||
$page->getId(), |
|
||||||
$userId, |
|
||||||
$added, |
|
||||||
$locale, |
|
||||||
$description, |
|
||||||
$approved |
|
||||||
); |
|
||||||
|
|
||||||
return $page; |
|
||||||
} |
|
||||||
|
|
||||||
public function addPage(): ?Page |
|
||||||
{ |
|
||||||
$page = new Page(); |
|
||||||
|
|
||||||
$this->entityManager->persist($page); |
|
||||||
$this->entityManager->flush(); |
|
||||||
|
|
||||||
return $page; |
|
||||||
} |
|
||||||
|
|
||||||
public function addPageTitle( |
|
||||||
int $pageId, |
|
||||||
int $userId, |
|
||||||
int $added, |
|
||||||
string $locale, |
|
||||||
string $value, |
|
||||||
bool $approved |
|
||||||
): ?PageTitle |
|
||||||
{ |
|
||||||
$pageTitle = new PageTitle(); |
|
||||||
|
|
||||||
$pageTitle->setPageId($pageId); |
|
||||||
$pageTitle->setUserId($userId); |
|
||||||
$pageTitle->setLocale($locale); |
|
||||||
$pageTitle->setValue($value); |
|
||||||
$pageTitle->setAdded($added); |
|
||||||
$pageTitle->setApproved($approved); |
|
||||||
|
|
||||||
$this->entityManager->persist($pageTitle); |
|
||||||
$this->entityManager->flush(); |
|
||||||
|
|
||||||
return $pageTitle; |
|
||||||
} |
|
||||||
|
|
||||||
public function addPageDescription( |
|
||||||
int $pageId, |
|
||||||
int $userId, |
|
||||||
int $added, |
|
||||||
string $locale, |
|
||||||
string $value, |
|
||||||
bool $approved |
|
||||||
): ?PageDescription |
|
||||||
{ |
|
||||||
$pageDescription = new PageDescription(); |
|
||||||
|
|
||||||
$pageDescription->setPageId($pageId); |
|
||||||
$pageDescription->setUserId($userId); |
|
||||||
$pageDescription->setAdded($added); |
|
||||||
$pageDescription->setLocale($locale); |
|
||||||
$pageDescription->setValue($value); |
|
||||||
$pageDescription->setApproved($approved); |
|
||||||
|
|
||||||
$this->entityManager->persist($pageDescription); |
|
||||||
$this->entityManager->flush(); |
|
||||||
|
|
||||||
return $pageDescription; |
|
||||||
} |
|
||||||
|
|
||||||
public function addPageTorrents( |
|
||||||
int $pageId, |
|
||||||
int $userId, |
|
||||||
int $added, |
|
||||||
array $torrentsId, |
|
||||||
bool $approved |
|
||||||
): ?PageTorrents |
|
||||||
{ |
|
||||||
$pageTorrents = new PageTorrents(); |
|
||||||
|
|
||||||
$pageTorrents->setPageId($pageId); |
|
||||||
$pageTorrents->setUserId($userId); |
|
||||||
$pageTorrents->setAdded($added); |
|
||||||
$pageTorrents->setTorrentsId($torrentsId); |
|
||||||
$pageTorrents->setApproved($approved); |
|
||||||
|
|
||||||
$this->entityManager->persist($pageTorrents); |
|
||||||
$this->entityManager->flush(); |
|
||||||
|
|
||||||
return $pageTorrents; |
|
||||||
} |
|
||||||
|
|
||||||
public function addPageSensitive( |
|
||||||
int $pageId, |
|
||||||
int $userId, |
|
||||||
int $added, |
|
||||||
string $locale, |
|
||||||
string $value, |
|
||||||
bool $approved |
|
||||||
): ?PageSensitive |
|
||||||
{ |
|
||||||
$pageSensitive = new PageSensitive(); |
|
||||||
|
|
||||||
$pageSensitive->setPageId($pageId); |
|
||||||
$pageSensitive->setUserId($userId); |
|
||||||
$pageSensitive->setAdded($added); |
|
||||||
$pageSensitive->setLocale($locale); |
|
||||||
$pageSensitive->setValue($value); |
|
||||||
$pageSensitive->setApproved($approved); |
|
||||||
|
|
||||||
$this->entityManager->persist($pageSensitive); |
|
||||||
$this->entityManager->flush(); |
|
||||||
|
|
||||||
return $pageSensitive; |
|
||||||
} |
|
||||||
} |
|
@ -1,11 +1,11 @@ |
|||||||
{% extends 'default/layout.html.twig' %} |
{% extends 'default/layout.html.twig' %} |
||||||
{% block title %}{{'Submit page'|trans }} - {{ name }}{% endblock %} |
{% block title %}{{'Submit article'|trans }} - {{ name }}{% endblock %} |
||||||
{% block main_content %} |
{% block main_content %} |
||||||
<div class="padding-24-px margin-y-8-px border-radius-3-px background-color-night"> |
<div class="padding-24-px margin-y-8-px border-radius-3-px background-color-night"> |
||||||
<div class="margin-b-24-px padding-b-16-px border-bottom-default"> |
<div class="margin-b-24-px padding-b-16-px border-bottom-default"> |
||||||
<h1>{{'Submit page'|trans }}</h1> |
<h1>{{'Submit article'|trans }}</h1> |
||||||
</div> |
</div> |
||||||
<form name="submit" method="post" enctype="multipart/form-data" action="{{ path('page_submit') }}"> |
<form name="submit" method="post" enctype="multipart/form-data" action="{{ path('article_submit') }}"> |
||||||
<div class="margin-b-16-px"> |
<div class="margin-b-16-px"> |
||||||
<label for="locale"> |
<label for="locale"> |
||||||
{{'Content language'|trans }} |
{{'Content language'|trans }} |
Loading…
Reference in new issue