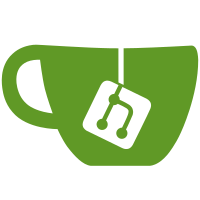
7 changed files with 495 additions and 104 deletions
@ -0,0 +1,85 @@
@@ -0,0 +1,85 @@
|
||||
{% extends 'default/layout.html.twig' %} |
||||
{% block title %}{{'Torrent'|trans }} #{{ torrentId }} - {{'Edit sensitive status'|trans }} - {{ name }}{% endblock %} |
||||
{% block main_content %} |
||||
<div class="padding-24-px margin-y-8-px border-radius-3-px background-color-night"> |
||||
<div class="margin-b-24-px padding-b-16-px border-bottom-default"> |
||||
<h1> |
||||
{{'Edit sensitive status for torrent'|trans }} |
||||
<a href="{{ path('torrent_info', { torrentId : torrentId }) }}">#{{ torrentId }}</a> |
||||
</h1> |
||||
</div> |
||||
<form name="sensitive" method="post" action="{{ path('torrent_sensitive_edit', { torrentId : torrentId }) }}"> |
||||
<div class="margin-y-16-px"> |
||||
<input type="checkbox" name="sensitive" id="sensitive" value="true" {% if form.sensitive.attribute.value %}checked="checked"{% endif %} /> |
||||
<label for="sensitive"> |
||||
{{'Sensitive'|trans }} |
||||
</label> |
||||
<sub class="opacity-0 parent-hover-opacity-09" title="{{ form.sensitive.attribute.placeholder }}"> |
||||
<svg xmlns="http://www.w3.org/2000/svg" width="13" height="13" fill="currentColor" viewBox="0 0 16 16"> |
||||
<path d="M8 16A8 8 0 1 0 8 0a8 8 0 0 0 0 16zm.93-9.412-1 4.705c-.07.34.029.533.304.533.194 0 .487-.07.686-.246l-.088.416c-.287.346-.92.598-1.465.598-.703 0-1.002-.422-.808-1.319l.738-3.468c.064-.293.006-.399-.287-.47l-.451-.081.082-.381 2.29-.287zM8 5.5a1 1 0 1 1 0-2 1 1 0 0 1 0 2z"/> |
||||
</svg> |
||||
</sub> |
||||
</div> |
||||
<div class="text-right"> |
||||
<a class="margin-r-8-px" href="{{ path('torrent_info', { torrentId : torrentId }) }}"> |
||||
{{'cancel'|trans }} |
||||
</a> |
||||
<input class="button-green" type="submit" value="{{'Submit'|trans }}" /> |
||||
</div> |
||||
</form> |
||||
</div> |
||||
{% for edition in editions %} |
||||
<div class="padding-x-24-px padding-y-16-px margin-y-8-px border-radius-3-px {% if edition.active %}background-color-night-light{% else %}background-color-night{% endif %} "> |
||||
{% if edition.active %} |
||||
{{ edition.added | format_ago }} |
||||
{% else %} |
||||
<a href="{{ path('torrent_sensitive_edit', { torrentId : torrentId, torrentSensitiveId : edition.id }) }}"> |
||||
{{ edition.added | format_ago }} |
||||
</a> |
||||
{% endif %} |
||||
{{ 'by'|trans }} |
||||
<a href="{{ path('user_info', { id : edition.user.id }) }}"> |
||||
<img class="border-radius-50 border-color-default vertical-align-middle" src="{{ edition.user.identicon }}" alt="{{'identicon'|trans }}" /> |
||||
</a> |
||||
<div class="float-right"> |
||||
{% if session.moderator or session.owner %} |
||||
<a class="margin-r-8-px text-color-red" href="{{ path('torrent_sensitive_delete', { torrentId : torrentId, torrentSensitiveId : edition.id }) }}" title="{{ 'Delete' | trans }}"> |
||||
<svg xmlns="http://www.w3.org/2000/svg" width="16" height="16" fill="currentColor" class="bi bi-x-circle" viewBox="0 0 16 16"> |
||||
<path d="M8 15A7 7 0 1 1 8 1a7 7 0 0 1 0 14zm0 1A8 8 0 1 0 8 0a8 8 0 0 0 0 16z"/> |
||||
<path d="M4.646 4.646a.5.5 0 0 1 .708 0L8 7.293l2.646-2.647a.5.5 0 0 1 .708.708L8.707 8l2.647 2.646a.5.5 0 0 1-.708.708L8 8.707l-2.646 2.647a.5.5 0 0 1-.708-.708L7.293 8 4.646 5.354a.5.5 0 0 1 0-.708z"/> |
||||
</svg> |
||||
</a> |
||||
{% endif %} |
||||
{% if edition.approved %} |
||||
{% if session.moderator %} |
||||
<a href="{{ path('torrent_sensitive_approve', { torrentId : torrentId, torrentSensitiveId : edition.id }) }}" title="{{ 'Approved' | trans }}"> |
||||
<svg xmlns="http://www.w3.org/2000/svg" width="16" height="16" fill="currentColor" viewBox="0 0 16 16"> |
||||
<path d="M16 8A8 8 0 1 1 0 8a8 8 0 0 1 16 0zm-3.97-3.03a.75.75 0 0 0-1.08.022L7.477 9.417 5.384 7.323a.75.75 0 0 0-1.06 1.06L6.97 11.03a.75.75 0 0 0 1.079-.02l3.992-4.99a.75.75 0 0 0-.01-1.05z"/> |
||||
</svg> |
||||
</a> |
||||
{% else %} |
||||
<span title="{{ 'Approved' | trans }}"> |
||||
<svg xmlns="http://www.w3.org/2000/svg" width="16" height="16" fill="currentColor" viewBox="0 0 16 16"> |
||||
<path d="M16 8A8 8 0 1 1 0 8a8 8 0 0 1 16 0zm-3.97-3.03a.75.75 0 0 0-1.08.022L7.477 9.417 5.384 7.323a.75.75 0 0 0-1.06 1.06L6.97 11.03a.75.75 0 0 0 1.079-.02l3.992-4.99a.75.75 0 0 0-.01-1.05z"/> |
||||
</svg> |
||||
</span> |
||||
{% endif %} |
||||
{% else %} |
||||
{% if session.moderator %} |
||||
<a href="{{ path('torrent_sensitive_approve', { torrentId : torrentId, torrentSensitiveId : edition.id }) }}" title="{{ 'Approved' | trans }}"> |
||||
<svg xmlns="http://www.w3.org/2000/svg" width="16" height="16" fill="currentColor" class="bi bi-hourglass" viewBox="0 0 16 16"> |
||||
<path d="M2 1.5a.5.5 0 0 1 .5-.5h11a.5.5 0 0 1 0 1h-1v1a4.5 4.5 0 0 1-2.557 4.06c-.29.139-.443.377-.443.59v.7c0 .213.154.451.443.59A4.5 4.5 0 0 1 12.5 13v1h1a.5.5 0 0 1 0 1h-11a.5.5 0 1 1 0-1h1v-1a4.5 4.5 0 0 1 2.557-4.06c.29-.139.443-.377.443-.59v-.7c0-.213-.154-.451-.443-.59A4.5 4.5 0 0 1 3.5 3V2h-1a.5.5 0 0 1-.5-.5zm2.5.5v1a3.5 3.5 0 0 0 1.989 3.158c.533.256 1.011.791 1.011 1.491v.702c0 .7-.478 1.235-1.011 1.491A3.5 3.5 0 0 0 4.5 13v1h7v-1a3.5 3.5 0 0 0-1.989-3.158C8.978 9.586 8.5 9.052 8.5 8.351v-.702c0-.7.478-1.235 1.011-1.491A3.5 3.5 0 0 0 11.5 3V2h-7z"/> |
||||
</svg> |
||||
</a> |
||||
{% else %} |
||||
<span title="{{ 'Waiting for approve' | trans }}"> |
||||
<svg xmlns="http://www.w3.org/2000/svg" width="16" height="16" fill="currentColor" class="bi bi-hourglass" viewBox="0 0 16 16"> |
||||
<path d="M2 1.5a.5.5 0 0 1 .5-.5h11a.5.5 0 0 1 0 1h-1v1a4.5 4.5 0 0 1-2.557 4.06c-.29.139-.443.377-.443.59v.7c0 .213.154.451.443.59A4.5 4.5 0 0 1 12.5 13v1h1a.5.5 0 0 1 0 1h-11a.5.5 0 1 1 0-1h1v-1a4.5 4.5 0 0 1 2.557-4.06c.29-.139.443-.377.443-.59v-.7c0-.213-.154-.451-.443-.59A4.5 4.5 0 0 1 3.5 3V2h-1a.5.5 0 0 1-.5-.5zm2.5.5v1a3.5 3.5 0 0 0 1.989 3.158c.533.256 1.011.791 1.011 1.491v.702c0 .7-.478 1.235-1.011 1.491A3.5 3.5 0 0 0 4.5 13v1h7v-1a3.5 3.5 0 0 0-1.989-3.158C8.978 9.586 8.5 9.052 8.5 8.351v-.702c0-.7.478-1.235 1.011-1.491A3.5 3.5 0 0 0 11.5 3V2h-7z"/> |
||||
</svg> |
||||
</span> |
||||
{% endif %} |
||||
{% endif %} |
||||
</div> |
||||
</div> |
||||
{% endfor %} |
||||
{% endblock %} |
Loading…
Reference in new issue