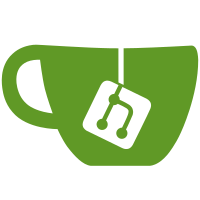
5 changed files with 288 additions and 26 deletions
@ -0,0 +1,65 @@
@@ -0,0 +1,65 @@
|
||||
<?php |
||||
|
||||
namespace App\Entity; |
||||
|
||||
use App\Repository\UserStarRepository; |
||||
use Doctrine\ORM\Mapping as ORM; |
||||
|
||||
#[ORM\Entity(repositoryClass: UserStarRepository::class)] |
||||
class UserStar |
||||
{ |
||||
#[ORM\Id] |
||||
#[ORM\GeneratedValue] |
||||
#[ORM\Column] |
||||
private ?int $id = null; |
||||
|
||||
#[ORM\Column] |
||||
private ?int $userId = null; |
||||
|
||||
#[ORM\Column] |
||||
private ?int $userIdTarget = null; |
||||
|
||||
#[ORM\Column] |
||||
private ?int $added = null; |
||||
|
||||
public function getId(): ?int |
||||
{ |
||||
return $this->id; |
||||
} |
||||
|
||||
public function getUserId(): ?int |
||||
{ |
||||
return $this->userId; |
||||
} |
||||
|
||||
public function setUserId(int $userId): static |
||||
{ |
||||
$this->userId = $userId; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getUserIdTarget(): ?int |
||||
{ |
||||
return $this->userIdTarget; |
||||
} |
||||
|
||||
public function setUserIdTarget(int $userIdTarget): static |
||||
{ |
||||
$this->userIdTarget = $userIdTarget; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getAdded(): ?int |
||||
{ |
||||
return $this->added; |
||||
} |
||||
|
||||
public function setAdded(int $added): static |
||||
{ |
||||
$this->added = $added; |
||||
|
||||
return $this; |
||||
} |
||||
} |
@ -0,0 +1,52 @@
@@ -0,0 +1,52 @@
|
||||
<?php |
||||
|
||||
namespace App\Repository; |
||||
|
||||
use App\Entity\UserStar; |
||||
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||
use Doctrine\Persistence\ManagerRegistry; |
||||
|
||||
/** |
||||
* @extends ServiceEntityRepository<UserStar> |
||||
* |
||||
* @method UserStar|null find($id, $lockMode = null, $lockVersion = null) |
||||
* @method UserStar|null findOneBy(array $criteria, array $orderBy = null) |
||||
* @method UserStar[] findAll() |
||||
* @method UserStar[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||
*/ |
||||
class UserStarRepository extends ServiceEntityRepository |
||||
{ |
||||
public function __construct(ManagerRegistry $registry) |
||||
{ |
||||
parent::__construct($registry, UserStar::class); |
||||
} |
||||
|
||||
public function findUserStar( |
||||
int $userId, |
||||
int $userIdTarget |
||||
): ?UserStar |
||||
{ |
||||
return $this->createQueryBuilder('us') |
||||
->where('us.userId = :userId') |
||||
->andWhere('us.userIdTarget = :userIdTarget') |
||||
->setParameter('userId', $userId) |
||||
->setParameter('userIdTarget', $userIdTarget) |
||||
->setMaxResults(1) |
||||
->getQuery() |
||||
->getOneOrNullResult() |
||||
; |
||||
} |
||||
|
||||
public function findUserStarsTotalByUserIdTarget( |
||||
int $userIdTarget |
||||
): int |
||||
{ |
||||
return $this->createQueryBuilder('us') |
||||
->select('count(us.userId)') |
||||
->where('us.userIdTarget = :userIdTarget') |
||||
->setParameter('userIdTarget', $userIdTarget) |
||||
->getQuery() |
||||
->getSingleScalarResult() |
||||
; |
||||
} |
||||
} |
Loading…
Reference in new issue