mirror of https://github.com/YGGverse/YGGstate.git
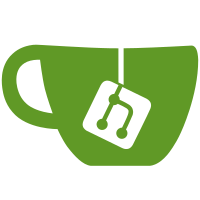
8 changed files with 28 additions and 144 deletions
@ -1,5 +1,9 @@ |
|||||||
.vscode |
/.vscode/ |
||||||
.ftpignore |
/vendor/ |
||||||
|
|
||||||
/config/app.php |
|
||||||
/database/yggstate.mwb.bak |
/database/yggstate.mwb.bak |
||||||
|
|
||||||
|
/src/config/app.php |
||||||
|
|
||||||
|
.ftpignore |
||||||
|
composer.lock |
||||||
|
@ -0,0 +1,17 @@ |
|||||||
|
{ |
||||||
|
"name": "yggverse/yggstate", |
||||||
|
"description": "Yggdrasil Network Analytics", |
||||||
|
"type": "project", |
||||||
|
"require": { |
||||||
|
"php": ">=8.1", |
||||||
|
"yggverse/yggdrasilctl": ">=0.1.0", |
||||||
|
"yggverse/parser": ">=0.1.0" |
||||||
|
}, |
||||||
|
"license": "MIT", |
||||||
|
"autoload": { |
||||||
|
"psr-4": { |
||||||
|
"Yggverse\\Yggstate\\": "src/" |
||||||
|
} |
||||||
|
}, |
||||||
|
"minimum-stability": "alpha" |
||||||
|
} |
@ -1,82 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
class URL { |
|
||||||
|
|
||||||
public static function is(string $url) : bool { |
|
||||||
|
|
||||||
return filter_var($url, FILTER_VALIDATE_URL); |
|
||||||
} |
|
||||||
|
|
||||||
public static function parse(string $url) : mixed { |
|
||||||
|
|
||||||
$result = (object) |
|
||||||
[ |
|
||||||
'host' => (object) |
|
||||||
[ |
|
||||||
'url' => null, |
|
||||||
'scheme' => null, |
|
||||||
'name' => null, |
|
||||||
'port' => null, |
|
||||||
], |
|
||||||
'page' => (object) |
|
||||||
[ |
|
||||||
'url' => null, |
|
||||||
'uri' => null, |
|
||||||
'path' => null, |
|
||||||
'query' => null, |
|
||||||
] |
|
||||||
]; |
|
||||||
|
|
||||||
// Validate URL |
|
||||||
if (!self::is($url)) { |
|
||||||
|
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
// Parse host |
|
||||||
if ($scheme = parse_url($url, PHP_URL_SCHEME)) { |
|
||||||
|
|
||||||
$result->host->url = $scheme . '://'; |
|
||||||
$result->host->scheme = $scheme; |
|
||||||
|
|
||||||
} else { |
|
||||||
|
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
if ($host = parse_url($url, PHP_URL_HOST)) { |
|
||||||
|
|
||||||
$result->host->url .= $host; |
|
||||||
$result->host->name = $host; |
|
||||||
|
|
||||||
} else { |
|
||||||
|
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
if ($port = parse_url($url, PHP_URL_PORT)) { |
|
||||||
|
|
||||||
$result->host->url .= ':' . $port; |
|
||||||
$result->host->port = $port; |
|
||||||
|
|
||||||
// port is optional |
|
||||||
} |
|
||||||
|
|
||||||
// Parse page |
|
||||||
if ($path = parse_url($url, PHP_URL_PATH)) { |
|
||||||
|
|
||||||
$result->page->uri = $path; |
|
||||||
$result->page->path = $path; |
|
||||||
} |
|
||||||
|
|
||||||
if ($query = parse_url($url, PHP_URL_QUERY)) { |
|
||||||
|
|
||||||
$result->page->uri .= '?' . $query; |
|
||||||
$result->page->query = '?' . $query; |
|
||||||
} |
|
||||||
|
|
||||||
$result->page->url = $result->host->url . $result->page->uri; |
|
||||||
|
|
||||||
return $result; |
|
||||||
} |
|
||||||
} |
|
@ -1,55 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
class Yggdrasil { |
|
||||||
|
|
||||||
private static function _exec(string $cmd) : mixed { |
|
||||||
|
|
||||||
if (false !== exec('yggdrasilctl -json getPeers', $output)) { |
|
||||||
|
|
||||||
$rows = []; |
|
||||||
|
|
||||||
foreach($output as $row){ |
|
||||||
|
|
||||||
$rows[] = $row; |
|
||||||
} |
|
||||||
|
|
||||||
if ($result = @json_decode(implode(PHP_EOL, $rows))) { |
|
||||||
|
|
||||||
return $result; |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
public static function getPeers() : mixed { |
|
||||||
|
|
||||||
if (false === $result = self::_exec('yggdrasilctl -json getPeers')) { |
|
||||||
|
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
if (empty($result->peers)) { |
|
||||||
|
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
foreach ((object) $result->peers as $peer) { |
|
||||||
|
|
||||||
switch (false) { |
|
||||||
|
|
||||||
case isset($peer->bytes_recvd): |
|
||||||
case isset($peer->bytes_sent): |
|
||||||
case isset($peer->remote): |
|
||||||
case isset($peer->port): |
|
||||||
case isset($peer->key): |
|
||||||
case isset($peer->uptime): |
|
||||||
case !empty($peer->coords): |
|
||||||
|
|
||||||
return false; |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return $result->peers; |
|
||||||
} |
|
||||||
} |
|
Loading…
Reference in new issue