mirror of https://github.com/PurpleI2P/pyseeder
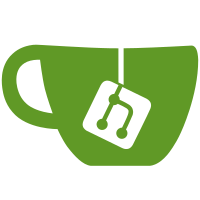
8 changed files with 137 additions and 70 deletions
@ -0,0 +1,52 @@
@@ -0,0 +1,52 @@
|
||||
"""Action functions for argparser""" |
||||
import pyseeder.transport |
||||
import pyseeder.actions |
||||
from pyseeder.utils import PyseederException, check_readable, check_writable |
||||
|
||||
def keygen(args): |
||||
"""Sub-command to generate keys""" |
||||
for f in [args.cert, args.private_key]: check_writable(f) |
||||
|
||||
from pyseeder.crypto import keygen |
||||
from getpass import getpass |
||||
priv_key_password = getpass("Set private key password: ").encode("utf-8") |
||||
keygen(args.cert, args.private_key, priv_key_password, args.signer_id) |
||||
|
||||
def reseed(args): |
||||
"""Sub-command to generate reseed file""" |
||||
check_writable(args.outfile) |
||||
for f in [args.netdb, args.private_key]: check_readable(f) |
||||
|
||||
from pyseeder.su3file import SU3File |
||||
priv_key_password = input().encode("utf-8") |
||||
su3file = SU3File(args.signer_id) |
||||
su3file.reseed(args.netdb) |
||||
su3file.write(args.outfile, args.private_key, priv_key_password) |
||||
|
||||
def transport_pull(args): |
||||
"""Sub-command for downloading su3 file""" |
||||
import random |
||||
random.shuffle(args.urls) |
||||
|
||||
for u in args.urls: |
||||
if pyseeder.transport.download(u, args.outfile): |
||||
return True |
||||
|
||||
raise PyseederException("Failed to download su3 file") |
||||
|
||||
def transport_push(args): |
||||
"""Sub-command for uploading su3 file with transports""" |
||||
check_readable(args.config) |
||||
|
||||
import configparser |
||||
config = configparser.ConfigParser() |
||||
config.read(args.config) |
||||
pyseeder.transport.upload(args.file, config) |
||||
|
||||
def serve(args): |
||||
"""Sub-command to start HTTPS reseed server""" |
||||
for f in [args.private_key, args.cert, args.file]: check_readable(f) |
||||
|
||||
import pyseeder.server |
||||
pyseeder.server.run_server(args.host, args.port, args.private_key, |
||||
args.cert, args.file) |
@ -0,0 +1,34 @@
@@ -0,0 +1,34 @@
|
||||
import http.server |
||||
import urllib.parse |
||||
import ssl |
||||
|
||||
class ReseedHandler(http.server.SimpleHTTPRequestHandler): |
||||
"""Handles reseeding requests""" |
||||
i2pseeds_file = "" |
||||
server_version = "Pyseeder Server" |
||||
sys_version = "" |
||||
|
||||
def do_GET(self): |
||||
path = urllib.parse.urlparse(self.path).path |
||||
if path == "/i2pseeds.su3": |
||||
self.send_response(200) |
||||
self.send_header("Content-Type", "application/octet-stream") |
||||
self.end_headers() |
||||
with open(self.i2pseeds_file, 'rb') as f: |
||||
self.wfile.write(f.read()) |
||||
else: |
||||
self.send_error(404, "Not found") |
||||
|
||||
def run_server(host, port, priv_key, cert, i2pseeds_file): |
||||
"""Start HTTPS server""" |
||||
Handler = ReseedHandler |
||||
Handler.i2pseeds_file = i2pseeds_file |
||||
|
||||
httpd = http.server.HTTPServer((host, int(port)), Handler) |
||||
httpd.socket = ssl.wrap_socket(httpd.socket, server_side=True, |
||||
keyfile=priv_key, certfile=cert, ssl_version=ssl.PROTOCOL_TLSv1) |
||||
|
||||
try: |
||||
httpd.serve_forever() |
||||
except KeyboardInterrupt: |
||||
exit() |
@ -1,7 +1,18 @@
@@ -1,7 +1,18 @@
|
||||
"""Various code""" |
||||
import os |
||||
|
||||
class PyseederException(Exception): |
||||
pass |
||||
|
||||
class TransportException(PyseederException): |
||||
pass |
||||
|
||||
def check_readable(f): |
||||
"""Checks if path exists and readable""" |
||||
if not os.path.exists(f) or not os.access(f, os.R_OK): |
||||
raise PyseederException("Error accessing path: {}".format(f)) |
||||
|
||||
def check_writable(f): |
||||
"""Checks if path is writable""" |
||||
if not os.access(os.path.dirname(f) or ".", os.W_OK): |
||||
raise PyseederException("Path is not writable: {}".format(f)) |
||||
|
Loading…
Reference in new issue