mirror of https://github.com/PurpleI2P/i2pd.git
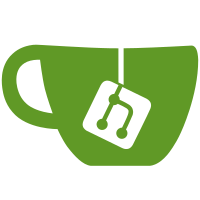
5 changed files with 211 additions and 102 deletions
@ -1,81 +1,81 @@ |
|||||||
#include "I2PEndian.h" |
//#include "I2PEndian.h"
|
||||||
|
//
|
||||||
// http://habrahabr.ru/post/121811/
|
//// http://habrahabr.ru/post/121811/
|
||||||
// http://codepad.org/2ycmkz2y
|
//// http://codepad.org/2ycmkz2y
|
||||||
|
//
|
||||||
#include "LittleBigEndian.h" |
//#include "LittleBigEndian.h"
|
||||||
|
//
|
||||||
uint16_t htobe16(uint16_t int16) |
//uint16_t htobe16(uint16_t int16)
|
||||||
{ |
//{
|
||||||
BigEndian<uint16_t> u16(int16); |
// BigEndian<uint16_t> u16(int16);
|
||||||
return u16.raw_value; |
// return u16.raw_value;
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint32_t htobe32(uint32_t int32) |
//uint32_t htobe32(uint32_t int32)
|
||||||
{ |
//{
|
||||||
BigEndian<uint32_t> u32(int32); |
// BigEndian<uint32_t> u32(int32);
|
||||||
return u32.raw_value; |
// return u32.raw_value;
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint64_t htobe64(uint64_t int64) |
//uint64_t htobe64(uint64_t int64)
|
||||||
{ |
//{
|
||||||
BigEndian<uint64_t> u64(int64); |
// BigEndian<uint64_t> u64(int64);
|
||||||
return u64.raw_value; |
// return u64.raw_value;
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint16_t be16toh(uint16_t big16) |
//uint16_t be16toh(uint16_t big16)
|
||||||
{ |
//{
|
||||||
LittleEndian<uint16_t> u16(big16); |
// LittleEndian<uint16_t> u16(big16);
|
||||||
return u16.raw_value; |
// return u16.raw_value;
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint32_t be32toh(uint32_t big32) |
//uint32_t be32toh(uint32_t big32)
|
||||||
{ |
//{
|
||||||
LittleEndian<uint32_t> u32(big32); |
// LittleEndian<uint32_t> u32(big32);
|
||||||
return u32.raw_value; |
// return u32.raw_value;
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint64_t be64toh(uint64_t big64) |
//uint64_t be64toh(uint64_t big64)
|
||||||
{ |
//{
|
||||||
LittleEndian<uint64_t> u64(big64); |
// LittleEndian<uint64_t> u64(big64);
|
||||||
return u64.raw_value; |
// return u64.raw_value;
|
||||||
} |
//}
|
||||||
|
//
|
||||||
/* it can be used in Windows 8
|
///* it can be used in Windows 8
|
||||||
#include <Winsock2.h> |
//#include <Winsock2.h>
|
||||||
|
//
|
||||||
uint16_t htobe16(uint16_t int16) |
//uint16_t htobe16(uint16_t int16)
|
||||||
{ |
//{
|
||||||
return htons(int16); |
// return htons(int16);
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint32_t htobe32(uint32_t int32) |
//uint32_t htobe32(uint32_t int32)
|
||||||
{ |
//{
|
||||||
return htonl(int32); |
// return htonl(int32);
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint64_t htobe64(uint64_t int64) |
//uint64_t htobe64(uint64_t int64)
|
||||||
{ |
//{
|
||||||
// http://msdn.microsoft.com/en-us/library/windows/desktop/jj710199%28v=vs.85%29.aspx
|
// // http://msdn.microsoft.com/en-us/library/windows/desktop/jj710199%28v=vs.85%29.aspx
|
||||||
//return htonll(int64);
|
// //return htonll(int64);
|
||||||
return 0; |
// return 0;
|
||||||
} |
//}
|
||||||
|
//
|
||||||
|
//
|
||||||
uint16_t be16toh(uint16_t big16) |
//uint16_t be16toh(uint16_t big16)
|
||||||
{ |
//{
|
||||||
return ntohs(big16); |
// return ntohs(big16);
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint32_t be32toh(uint32_t big32) |
//uint32_t be32toh(uint32_t big32)
|
||||||
{ |
//{
|
||||||
return ntohl(big32); |
// return ntohl(big32);
|
||||||
} |
//}
|
||||||
|
//
|
||||||
uint64_t be64toh(uint64_t big64) |
//uint64_t be64toh(uint64_t big64)
|
||||||
{ |
//{
|
||||||
// http://msdn.microsoft.com/en-us/library/windows/desktop/jj710199%28v=vs.85%29.aspx
|
// // http://msdn.microsoft.com/en-us/library/windows/desktop/jj710199%28v=vs.85%29.aspx
|
||||||
//return ntohll(big64);
|
// //return ntohll(big64);
|
||||||
return 0; |
// return 0;
|
||||||
} |
//}
|
||||||
*/ |
//*/
|
@ -0,0 +1,115 @@ |
|||||||
|
#ifndef PORTABLE_ENDIAN_H__ |
||||||
|
#define PORTABLE_ENDIAN_H__ |
||||||
|
|
||||||
|
#if (defined(_WIN16) || defined(_WIN32) || defined(_WIN64)) && !defined(__WINDOWS__) |
||||||
|
|
||||||
|
# define __WINDOWS__ |
||||||
|
|
||||||
|
#endif |
||||||
|
|
||||||
|
#if defined(__linux__) || defined(__CYGWIN__) |
||||||
|
|
||||||
|
# include <endian.h> |
||||||
|
|
||||||
|
#elif defined(__APPLE__) |
||||||
|
|
||||||
|
# include <libkern/OSByteOrder.h> |
||||||
|
|
||||||
|
# define htobe16 OSSwapHostToBigInt16 |
||||||
|
# define htole16 OSSwapHostToLittleInt16 |
||||||
|
# define be16toh OSSwapBigToHostInt16 |
||||||
|
# define le16toh OSSwapLittleToHostInt16 |
||||||
|
|
||||||
|
# define htobe32 OSSwapHostToBigInt32 |
||||||
|
# define htole32 OSSwapHostToLittleInt32 |
||||||
|
# define be32toh OSSwapBigToHostInt32 |
||||||
|
# define le32toh OSSwapLittleToHostInt32 |
||||||
|
|
||||||
|
# define htobe64 OSSwapHostToBigInt64 |
||||||
|
# define htole64 OSSwapHostToLittleInt64 |
||||||
|
# define be64toh OSSwapBigToHostInt64 |
||||||
|
# define le64toh OSSwapLittleToHostInt64 |
||||||
|
|
||||||
|
# define __BYTE_ORDER BYTE_ORDER |
||||||
|
# define __BIG_ENDIAN BIG_ENDIAN |
||||||
|
# define __LITTLE_ENDIAN LITTLE_ENDIAN |
||||||
|
# define __PDP_ENDIAN PDP_ENDIAN |
||||||
|
|
||||||
|
#elif defined(__OpenBSD__) |
||||||
|
|
||||||
|
# include <sys/endian.h> |
||||||
|
|
||||||
|
#elif defined(__NetBSD__) || defined(__FreeBSD__) || defined(__DragonFly__) |
||||||
|
|
||||||
|
# include <sys/endian.h> |
||||||
|
|
||||||
|
# define be16toh betoh16 |
||||||
|
# define le16toh letoh16 |
||||||
|
|
||||||
|
# define be32toh betoh32 |
||||||
|
# define le32toh letoh32 |
||||||
|
|
||||||
|
# define be64toh betoh64 |
||||||
|
# define le64toh letoh64 |
||||||
|
|
||||||
|
#elif defined(__WINDOWS__) |
||||||
|
|
||||||
|
#define INCL_EXTRA_HTON_FUNCTIONS |
||||||
|
#define NOMINMAX |
||||||
|
# include <winsock2.h> |
||||||
|
#undef NOMINMAX |
||||||
|
//# include <sys/param.h>
|
||||||
|
|
||||||
|
# if BYTE_ORDER == LITTLE_ENDIAN |
||||||
|
|
||||||
|
# define htobe16 htons |
||||||
|
# define htole16(x) (x) |
||||||
|
# define be16toh ntohs |
||||||
|
# define le16toh(x) (x) |
||||||
|
|
||||||
|
# define htobe32 htonl |
||||||
|
# define htole32(x) (x) |
||||||
|
# define be32toh ntohl |
||||||
|
# define le32toh(x) (x) |
||||||
|
|
||||||
|
# define htobe64 htonll |
||||||
|
# define htole64(x) (x) |
||||||
|
# define be64toh ntohll |
||||||
|
# define le64toh(x) (x) |
||||||
|
|
||||||
|
# elif BYTE_ORDER == BIG_ENDIAN |
||||||
|
|
||||||
|
/* that would be xbox 360 */ |
||||||
|
# define htobe16(x) (x) |
||||||
|
# define htole16(x) __builtin_bswap16(x) |
||||||
|
# define be16toh(x) (x) |
||||||
|
# define le16toh(x) __builtin_bswap16(x) |
||||||
|
|
||||||
|
# define htobe32(x) (x) |
||||||
|
# define htole32(x) __builtin_bswap32(x) |
||||||
|
# define be32toh(x) (x) |
||||||
|
# define le32toh(x) __builtin_bswap32(x) |
||||||
|
|
||||||
|
# define htobe64(x) (x) |
||||||
|
# define htole64(x) __builtin_bswap64(x) |
||||||
|
# define be64toh(x) (x) |
||||||
|
# define le64toh(x) __builtin_bswap64(x) |
||||||
|
|
||||||
|
# else |
||||||
|
|
||||||
|
# error byte order not supported |
||||||
|
|
||||||
|
# endif |
||||||
|
|
||||||
|
# define __BYTE_ORDER BYTE_ORDER |
||||||
|
# define __BIG_ENDIAN BIG_ENDIAN |
||||||
|
# define __LITTLE_ENDIAN LITTLE_ENDIAN |
||||||
|
# define __PDP_ENDIAN PDP_ENDIAN |
||||||
|
|
||||||
|
#else |
||||||
|
|
||||||
|
# error platform not supported |
||||||
|
|
||||||
|
#endif |
||||||
|
|
||||||
|
#endif |
Loading…
Reference in new issue