mirror of https://github.com/PurpleI2P/i2pd.git
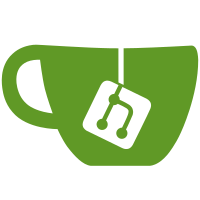
5 changed files with 238 additions and 33 deletions
@ -0,0 +1,33 @@ |
|||||||
|
#include <cryptopp/sha.h> |
||||||
|
#include "Log.h" |
||||||
|
#include "RouterInfo.h" |
||||||
|
#include "LeaseSet.h" |
||||||
|
|
||||||
|
namespace i2p |
||||||
|
{ |
||||||
|
namespace data |
||||||
|
{ |
||||||
|
LeaseSet::LeaseSet (const uint8_t * buf, int len) |
||||||
|
{ |
||||||
|
#pragma pack(1) |
||||||
|
struct H |
||||||
|
{ |
||||||
|
RouterIdentity destination; |
||||||
|
uint8_t encryptionKey[256]; |
||||||
|
uint8_t signingKey[128]; |
||||||
|
uint8_t num; |
||||||
|
}; |
||||||
|
#pragma pack () |
||||||
|
|
||||||
|
const H * header = (const H *)buf; |
||||||
|
CryptoPP::SHA256().CalculateDigest(m_IdentHash, (uint8_t *)&header->destination, sizeof (RouterIdentity)); |
||||||
|
memcpy (m_EncryptionKey, header->encryptionKey, 256); |
||||||
|
LogPrint ("LeaseSet num=", (int)header->num); |
||||||
|
|
||||||
|
for (int i = 0; i < header->num; i++) |
||||||
|
{ |
||||||
|
m_Leases.push_back (*(Lease *)(buf + sizeof (H))); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,46 @@ |
|||||||
|
#ifndef LEASE_SET_H__ |
||||||
|
#define LEASE_SET_H__ |
||||||
|
|
||||||
|
#include <inttypes.h> |
||||||
|
#include <list> |
||||||
|
|
||||||
|
namespace i2p |
||||||
|
{ |
||||||
|
namespace data |
||||||
|
{ |
||||||
|
#pragma pack(1) |
||||||
|
struct Lease |
||||||
|
{ |
||||||
|
uint8_t tunnelGateway[32]; |
||||||
|
uint32_t tunnelID; |
||||||
|
uint64_t endDate; |
||||||
|
}; |
||||||
|
#pragma pack() |
||||||
|
|
||||||
|
class RoutingDestination // TODO: move to separate file later
|
||||||
|
{ |
||||||
|
public: |
||||||
|
virtual const uint8_t * GetIdentHash () const = 0; |
||||||
|
virtual const uint8_t * GetEncryptionPublicKey () const = 0; |
||||||
|
}; |
||||||
|
|
||||||
|
class LeaseSet: public RoutingDestination |
||||||
|
{ |
||||||
|
public: |
||||||
|
|
||||||
|
LeaseSet (const uint8_t * buf, int len); |
||||||
|
|
||||||
|
// implements RoutingDestination
|
||||||
|
const uint8_t * GetIdentHash () const { return m_IdentHash; }; |
||||||
|
const uint8_t * GetEncryptionPublicKey () const { return m_EncryptionKey; }; |
||||||
|
|
||||||
|
private: |
||||||
|
|
||||||
|
std::list<Lease> m_Leases; |
||||||
|
uint8_t m_IdentHash[32]; |
||||||
|
uint8_t m_EncryptionKey[256]; |
||||||
|
}; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
#endif |
Loading…
Reference in new issue