mirror of https://github.com/PurpleI2P/i2pd.git
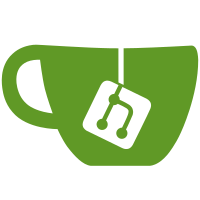
12 changed files with 360 additions and 43 deletions
@ -1,4 +1,66 @@ |
|||||||
#ifndef DAEMONQT_H |
#ifndef DAEMONQT_H |
||||||
#define DAEMONQT_H |
#define DAEMONQT_H |
||||||
|
|
||||||
|
#include <QObject> |
||||||
|
#include <QThread> |
||||||
|
|
||||||
|
namespace i2p |
||||||
|
{ |
||||||
|
namespace util |
||||||
|
{ |
||||||
|
namespace DaemonQt |
||||||
|
{ |
||||||
|
class Worker : public QObject |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
public slots: |
||||||
|
void startDaemon(); |
||||||
|
void restartDaemon(); |
||||||
|
void stopDaemon(); |
||||||
|
|
||||||
|
signals: |
||||||
|
void resultReady(); |
||||||
|
}; |
||||||
|
|
||||||
|
class DaemonQTImpl |
||||||
|
{ |
||||||
|
public: |
||||||
|
typedef void (*runningChangedCallback)(); |
||||||
|
|
||||||
|
/**
|
||||||
|
* @brief init |
||||||
|
* @param argc |
||||||
|
* @param argv |
||||||
|
* @return success |
||||||
|
*/ |
||||||
|
bool static init(int argc, char* argv[]); |
||||||
|
void static deinit(); |
||||||
|
void static start(); |
||||||
|
void static stop(); |
||||||
|
void static restart(); |
||||||
|
void static setRunningCallback(runningChangedCallback cb); |
||||||
|
bool static isRunning(); |
||||||
|
private: |
||||||
|
void static setRunning(bool running); |
||||||
|
}; |
||||||
|
|
||||||
|
class Controller : public QObject |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
QThread workerThread; |
||||||
|
public: |
||||||
|
Controller(); |
||||||
|
~Controller(); |
||||||
|
public slots: |
||||||
|
void handleResults(){} |
||||||
|
signals: |
||||||
|
void startDaemon(); |
||||||
|
void stopDaemon(); |
||||||
|
void restartDaemon(); |
||||||
|
}; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
#endif // DAEMONQT_H
|
#endif // DAEMONQT_H
|
||||||
|
@ -0,0 +1,24 @@ |
|||||||
|
#if 0 |
||||||
|
#include "i2pd_qt_gui.h" |
||||||
|
#include <QApplication> |
||||||
|
#include <QMessageBox> |
||||||
|
#include "mainwindow.h" |
||||||
|
#include "DaemonQT.h" |
||||||
|
|
||||||
|
int runGUI( int argc, char* argv[] ) { |
||||||
|
QApplication app(argc, argv); |
||||||
|
bool daemonInitSuccess = i2p::util::DaemonQTImpl::init(argc, argv); |
||||||
|
if(!daemonInitSuccess) { |
||||||
|
QMessageBox::critical(0, "Error", "Daemon init failed"); |
||||||
|
return 1; |
||||||
|
} |
||||||
|
MainWindow w; |
||||||
|
w.show (); |
||||||
|
i2p::util::DaemonQTImpl::start(); |
||||||
|
int result = app.exec(); |
||||||
|
//QMessageBox::information(&w, "Debug", "exec finished");
|
||||||
|
i2p::util::DaemonQTImpl::stop(); |
||||||
|
//QMessageBox::information(&w, "Debug", "demon stopped");
|
||||||
|
return result; |
||||||
|
} |
||||||
|
#endif |
@ -1,4 +1,6 @@ |
|||||||
#ifndef IQPD_QT_GUI_H |
#ifndef IQPD_QT_GUI_H |
||||||
#define IQPD_QT_GUI_H |
#define IQPD_QT_GUI_H |
||||||
|
|
||||||
|
int runGUI( int argc, char* argv[] ); |
||||||
|
|
||||||
#endif // IQPD_QT_GUI_H
|
#endif // IQPD_QT_GUI_H
|
||||||
|
@ -1,14 +1,55 @@ |
|||||||
#include "mainwindow.h" |
#include "mainwindow.h" |
||||||
#include "ui_mainwindow.h" |
//#include "ui_mainwindow.h"
|
||||||
|
#include <QMessageBox> |
||||||
|
|
||||||
MainWindow::MainWindow(QWidget *parent) : |
MainWindow::MainWindow(QWidget *parent) : |
||||||
QMainWindow(parent), |
QMainWindow(parent)/*,
|
||||||
ui(new Ui::MainWindow) |
ui(new Ui::MainWindow)*/ |
||||||
{ |
{ |
||||||
ui->setupUi(this); |
//ui->setupUi(this);
|
||||||
|
if (objectName().isEmpty()) |
||||||
|
setObjectName(QStringLiteral("MainWindow")); |
||||||
|
resize(800, 480); |
||||||
|
centralWidget = new QWidget(this); |
||||||
|
centralWidget->setObjectName(QStringLiteral("centralWidget")); |
||||||
|
verticalLayoutWidget = new QWidget(centralWidget); |
||||||
|
verticalLayoutWidget->setObjectName(QStringLiteral("verticalLayoutWidget")); |
||||||
|
//verticalLayoutWidget->setGeometry(QRect(10, 20, 771, 441));
|
||||||
|
verticalLayout1 = new QVBoxLayout(verticalLayoutWidget); |
||||||
|
verticalLayout1->setSpacing(6); |
||||||
|
verticalLayout1->setContentsMargins(11, 11, 11, 11); |
||||||
|
verticalLayout1->setObjectName(QStringLiteral("verticalLayout1")); |
||||||
|
verticalLayout1->setContentsMargins(0, 0, 0, 0); |
||||||
|
quitButton = new QPushButton(verticalLayoutWidget); |
||||||
|
quitButton->setObjectName(QStringLiteral("quitButton")); |
||||||
|
QSizePolicy sizePolicy(QSizePolicy::MinimumExpanding, QSizePolicy::Fixed); |
||||||
|
sizePolicy.setHorizontalStretch(0); |
||||||
|
sizePolicy.setVerticalStretch(0); |
||||||
|
sizePolicy.setHeightForWidth(quitButton->sizePolicy().hasHeightForWidth()); |
||||||
|
quitButton->setSizePolicy(sizePolicy); |
||||||
|
|
||||||
|
verticalLayout1->addWidget(quitButton); |
||||||
|
|
||||||
|
setCentralWidget(centralWidget); |
||||||
|
|
||||||
|
setWindowTitle(QApplication::translate("MainWindow", "MainWindow", 0)); |
||||||
|
quitButton->setText(QApplication::translate("MainWindow", "Quit", 0)); |
||||||
|
|
||||||
|
QObject::connect(quitButton, SIGNAL(released()), this, SLOT(handleQuitButton())); |
||||||
|
|
||||||
|
//QMetaObject::connectSlotsByName(this);
|
||||||
|
} |
||||||
|
|
||||||
|
void MainWindow::handleQuitButton() { |
||||||
|
qDebug("Quit pressed. Hiding the main window"); |
||||||
|
close(); |
||||||
|
QApplication::instance()->quit(); |
||||||
} |
} |
||||||
|
|
||||||
MainWindow::~MainWindow() |
MainWindow::~MainWindow() |
||||||
{ |
{ |
||||||
delete ui; |
qDebug("Destroying main window"); |
||||||
|
//QMessageBox::information(0, "Debug", "mw destructor 1");
|
||||||
|
//delete ui;
|
||||||
|
//QMessageBox::information(0, "Debug", "mw destructor 2");
|
||||||
} |
} |
||||||
|
Loading…
Reference in new issue