mirror of https://github.com/kevachat/npsapp.git
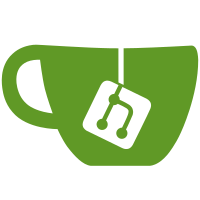
6 changed files with 357 additions and 367 deletions
@ -0,0 +1,262 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Kevachat\Npsapp\Server; |
||||||
|
|
||||||
|
use \Ratchet\MessageComponentInterface; |
||||||
|
|
||||||
|
class Ratchet implements MessageComponentInterface |
||||||
|
{ |
||||||
|
private \Kevachat\Kevacoin\Client $_kevacoin; |
||||||
|
|
||||||
|
private object $_config; |
||||||
|
|
||||||
|
public function __construct( |
||||||
|
object $config |
||||||
|
) { |
||||||
|
// Init config |
||||||
|
$this->_config = $config; |
||||||
|
|
||||||
|
// Init KevaCoin |
||||||
|
$this->_kevacoin = new \Kevachat\Kevacoin\Client( |
||||||
|
$this->_config->kevacoin->server->protocol, |
||||||
|
$this->_config->kevacoin->server->host, |
||||||
|
$this->_config->kevacoin->server->port, |
||||||
|
$this->_config->kevacoin->server->username, |
||||||
|
$this->_config->kevacoin->server->password |
||||||
|
); |
||||||
|
|
||||||
|
// Validate funds |
||||||
|
if ((float) $this->_kevacoin->getBalance($this->_config->kevacoin->wallet->account) <= 0) |
||||||
|
{ |
||||||
|
throw new \Exception(); // @TODO |
||||||
|
} |
||||||
|
|
||||||
|
// Dump event on enabled |
||||||
|
if ($this->_config->nps->event->init->debug->enabled) |
||||||
|
{ |
||||||
|
print( |
||||||
|
str_ireplace( |
||||||
|
[ |
||||||
|
'{time}', |
||||||
|
'{host}', |
||||||
|
'{port}', |
||||||
|
'{keva}' |
||||||
|
], |
||||||
|
[ |
||||||
|
(string) date('c'), |
||||||
|
(string) $this->_config->nps->server->host, |
||||||
|
(string) $this->_config->nps->server->port, |
||||||
|
(float) $this->_kevacoin->getBalance( |
||||||
|
$this->_config->kevacoin->wallet->account |
||||||
|
) |
||||||
|
], |
||||||
|
$this->_config->nps->event->init->debug->template |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public function onOpen( |
||||||
|
\Ratchet\ConnectionInterface $connection |
||||||
|
) { |
||||||
|
// Init config namespace |
||||||
|
$config = $this->_config->nps; |
||||||
|
|
||||||
|
// Build captcha |
||||||
|
$captcha = new \Gregwar\Captcha\CaptchaBuilder( |
||||||
|
null, |
||||||
|
new \Gregwar\Captcha\PhraseBuilder( |
||||||
|
$config->captcha->length, |
||||||
|
$config->captcha->chars |
||||||
|
) |
||||||
|
); |
||||||
|
|
||||||
|
$captcha->setBackgroundColor( |
||||||
|
$config->captcha->background->r, |
||||||
|
$config->captcha->background->g, |
||||||
|
$config->captcha->background->b |
||||||
|
); |
||||||
|
|
||||||
|
$captcha->build( |
||||||
|
$config->captcha->dimensions->width, |
||||||
|
$config->captcha->dimensions->height |
||||||
|
); |
||||||
|
|
||||||
|
// Convert captcha image to ASCII response |
||||||
|
$image = new \Ixnode\PhpCliImage\CliImage( |
||||||
|
$captcha->get(), |
||||||
|
$config->captcha->ascii->width |
||||||
|
); |
||||||
|
|
||||||
|
// Send response |
||||||
|
$connection->send( |
||||||
|
sprintf( |
||||||
|
implode( |
||||||
|
PHP_EOL, |
||||||
|
$config->event->open->response |
||||||
|
) . PHP_EOL . $image->getAsciiString() . PHP_EOL |
||||||
|
) |
||||||
|
); |
||||||
|
|
||||||
|
// Keep captcha phrase in connection |
||||||
|
$connection->captcha = $captcha->getPhrase(); |
||||||
|
|
||||||
|
// Init connection confirmed |
||||||
|
$connection->confirmed = false; |
||||||
|
|
||||||
|
// Debug open event on enabled |
||||||
|
if ($config->event->open->debug->enabled) |
||||||
|
{ |
||||||
|
// Print debug from template |
||||||
|
print( |
||||||
|
str_ireplace( |
||||||
|
[ |
||||||
|
'{time}', |
||||||
|
'{host}', |
||||||
|
'{crid}', |
||||||
|
'{code}' |
||||||
|
], |
||||||
|
[ |
||||||
|
(string) date('c'), |
||||||
|
(string) $connection->remoteAddress, |
||||||
|
(string) $connection->resourceId, |
||||||
|
(string) $connection->captcha |
||||||
|
], |
||||||
|
$config->event->open->debug->template |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public function onMessage( |
||||||
|
\Ratchet\ConnectionInterface $connection, |
||||||
|
$request |
||||||
|
) { |
||||||
|
// Init config namespace |
||||||
|
$config = $this->_config->nps->event->message; |
||||||
|
|
||||||
|
// Captcha request first for unconfirmed connections |
||||||
|
if (!$connection->confirmed) |
||||||
|
{ |
||||||
|
// Request match captcha |
||||||
|
if ($request == $connection->captcha) |
||||||
|
{ |
||||||
|
$connection->confirmed = true; |
||||||
|
|
||||||
|
$connection->send( |
||||||
|
implode( |
||||||
|
PHP_EOL, |
||||||
|
$config->response->captcha->success |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
// Captcha request invalid |
||||||
|
else |
||||||
|
{ |
||||||
|
$connection->confirmed = false; |
||||||
|
|
||||||
|
$connection->send( |
||||||
|
implode( |
||||||
|
PHP_EOL, |
||||||
|
$config->response->captcha->failure |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
|
||||||
|
// Drop connection or do something else.. |
||||||
|
$connection->close(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
// @TODO compose request to KevaCoin, return transaction ID |
||||||
|
else |
||||||
|
{ |
||||||
|
// Save massage to kevacoin |
||||||
|
/* |
||||||
|
$connection->send( |
||||||
|
implode( |
||||||
|
PHP_EOL, |
||||||
|
$config->response->captcha->failure |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
*/ |
||||||
|
} |
||||||
|
|
||||||
|
// Debug message event on enabled |
||||||
|
if ($config->debug->enabled) |
||||||
|
{ |
||||||
|
print( |
||||||
|
str_ireplace( |
||||||
|
[ |
||||||
|
'{time}', |
||||||
|
'{host}', |
||||||
|
'{crid}', |
||||||
|
'{code}', |
||||||
|
'{sent}', |
||||||
|
'{size}' |
||||||
|
], |
||||||
|
[ |
||||||
|
(string) date('c'), |
||||||
|
(string) $connection->remoteAddress, |
||||||
|
(string) $connection->resourceId, |
||||||
|
(string) $connection->captcha, |
||||||
|
(string) str_replace('%', '%%', $request), |
||||||
|
(string) mb_strlen($request) |
||||||
|
], |
||||||
|
$config->debug->template |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public function onClose( |
||||||
|
\Ratchet\ConnectionInterface $connection |
||||||
|
) { |
||||||
|
if ($this->_config->nps->event->close->debug->enabled) |
||||||
|
{ |
||||||
|
print( |
||||||
|
str_ireplace( |
||||||
|
[ |
||||||
|
'{time}', |
||||||
|
'{host}', |
||||||
|
'{crid}' |
||||||
|
], |
||||||
|
[ |
||||||
|
(string) date('c'), |
||||||
|
(string) $connection->remoteAddress, |
||||||
|
(string) $connection->resourceId |
||||||
|
], |
||||||
|
$this->_config->nps->event->close->debug->template |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public function onError( |
||||||
|
\Ratchet\ConnectionInterface $connection, |
||||||
|
\Exception $exception |
||||||
|
) { |
||||||
|
if ($this->_config->nps->event->close->debug->enabled) |
||||||
|
{ |
||||||
|
print( |
||||||
|
str_ireplace( |
||||||
|
[ |
||||||
|
'{time}', |
||||||
|
'{host}', |
||||||
|
'{crid}', |
||||||
|
'{info}' |
||||||
|
], |
||||||
|
[ |
||||||
|
(string) date('c'), |
||||||
|
(string) $connection->remoteAddress, |
||||||
|
(string) $connection->resourceId, |
||||||
|
(string) str_replace('%', '%%', $exception->getMessage()) |
||||||
|
], |
||||||
|
$this->_config->nps->event->error->debug->template |
||||||
|
) . PHP_EOL |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
$connection->close(); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,30 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
// Load dependencies |
||||||
|
require_once __DIR__ . |
||||||
|
DIRECTORY_SEPARATOR . '..'. |
||||||
|
DIRECTORY_SEPARATOR . 'vendor' . |
||||||
|
DIRECTORY_SEPARATOR . 'autoload.php'; |
||||||
|
|
||||||
|
// Init config |
||||||
|
$config = json_decode( |
||||||
|
file_get_contents( |
||||||
|
__DIR__ . |
||||||
|
DIRECTORY_SEPARATOR . '..'. |
||||||
|
DIRECTORY_SEPARATOR . 'config'. |
||||||
|
DIRECTORY_SEPARATOR . ( |
||||||
|
isset($argv[1]) ? $argv[1] : 'example.json' |
||||||
|
) |
||||||
|
) |
||||||
|
); if (!$config) throw new \Exception(); |
||||||
|
|
||||||
|
// Start server |
||||||
|
$server = \Ratchet\Server\IoServer::factory( |
||||||
|
new \Kevachat\Npsapp\Server\Ratchet( |
||||||
|
$config |
||||||
|
), |
||||||
|
$config->nps->server->port, |
||||||
|
$config->nps->server->host |
||||||
|
); |
||||||
|
|
||||||
|
$server->run(); |
@ -1,310 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
// Load dependencies |
|
||||||
require_once __DIR__ . |
|
||||||
DIRECTORY_SEPARATOR . '..'. |
|
||||||
DIRECTORY_SEPARATOR . 'vendor' . |
|
||||||
DIRECTORY_SEPARATOR . 'autoload.php'; |
|
||||||
|
|
||||||
// Init config |
|
||||||
$config = json_decode( |
|
||||||
file_get_contents( |
|
||||||
__DIR__ . |
|
||||||
DIRECTORY_SEPARATOR . '..'. |
|
||||||
DIRECTORY_SEPARATOR . 'config'. |
|
||||||
DIRECTORY_SEPARATOR . ( |
|
||||||
isset($argv[1]) ? $argv[1] : 'example.json' |
|
||||||
) |
|
||||||
) |
|
||||||
); if (!$config) exit; |
|
||||||
|
|
||||||
// Init KevaCoin |
|
||||||
$kevacoin = new \Kevachat\Kevacoin\Client( |
|
||||||
$config->kevacoin->server->protocol, |
|
||||||
$config->kevacoin->server->host, |
|
||||||
$config->kevacoin->server->port, |
|
||||||
$config->kevacoin->server->username, |
|
||||||
$config->kevacoin->server->password |
|
||||||
); |
|
||||||
|
|
||||||
if ((float) $kevacoin->getBalance($config->kevacoin->wallet->account) <= 0) exit; |
|
||||||
|
|
||||||
// Init session |
|
||||||
$session = []; |
|
||||||
|
|
||||||
// Init server |
|
||||||
$server = new \Yggverse\Nps\Server( |
|
||||||
$config->nps->server->host, |
|
||||||
$config->nps->server->port, |
|
||||||
$config->nps->server->size, |
|
||||||
$config->nps->server->line |
|
||||||
); |
|
||||||
|
|
||||||
// Init welcome function |
|
||||||
$server->setWelcome( |
|
||||||
function ( |
|
||||||
string $connect |
|
||||||
): ?string |
|
||||||
{ |
|
||||||
global $config, |
|
||||||
$session; |
|
||||||
|
|
||||||
// Cleanup expired sessions |
|
||||||
foreach ($session as $key => $value) |
|
||||||
{ |
|
||||||
if ($value['time'] + $config->nps->session->timeout < time()) |
|
||||||
{ |
|
||||||
unset( |
|
||||||
$session[$key] |
|
||||||
); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// Build connection URL #72811 |
|
||||||
$url = sprintf( |
|
||||||
'nex://%s', |
|
||||||
$connect |
|
||||||
); |
|
||||||
|
|
||||||
// Init new session |
|
||||||
$session[$connect] = |
|
||||||
[ |
|
||||||
'time' => time(), |
|
||||||
'host' => parse_url( |
|
||||||
$url, |
|
||||||
PHP_URL_HOST |
|
||||||
), |
|
||||||
'port' => parse_url( |
|
||||||
$url, |
|
||||||
PHP_URL_PORT |
|
||||||
), |
|
||||||
'code' => null |
|
||||||
]; |
|
||||||
|
|
||||||
// Build captcha |
|
||||||
$captcha = new \Gregwar\Captcha\CaptchaBuilder( |
|
||||||
null, |
|
||||||
new \Gregwar\Captcha\PhraseBuilder( |
|
||||||
$config->nps->session->captcha->length, |
|
||||||
$config->nps->session->captcha->chars |
|
||||||
) |
|
||||||
); |
|
||||||
|
|
||||||
$captcha->setBackgroundColor( |
|
||||||
$config->nps->session->captcha->background->r, |
|
||||||
$config->nps->session->captcha->background->g, |
|
||||||
$config->nps->session->captcha->background->b |
|
||||||
); |
|
||||||
|
|
||||||
$captcha->build( |
|
||||||
$config->nps->session->captcha->dimensions->width, |
|
||||||
$config->nps->session->captcha->dimensions->height |
|
||||||
); |
|
||||||
|
|
||||||
// Set captcha value to the session code |
|
||||||
$session[$connect]['code'] = $captcha->getPhrase(); |
|
||||||
|
|
||||||
// Create ASCII confirmation code |
|
||||||
$image = new \Ixnode\PhpCliImage\CliImage( |
|
||||||
$captcha->get(), |
|
||||||
$config->nps->session->captcha->ascii->width |
|
||||||
); |
|
||||||
|
|
||||||
// Debug request on enabled |
|
||||||
if ($config->nps->action->welcome->debug->enabled) |
|
||||||
{ |
|
||||||
// Print debug from template |
|
||||||
print( |
|
||||||
str_ireplace( |
|
||||||
[ |
|
||||||
'{time}', |
|
||||||
'{host}', |
|
||||||
'{port}', |
|
||||||
'{code}' |
|
||||||
], |
|
||||||
[ |
|
||||||
(string) date('c'), |
|
||||||
(string) $session[$connect]['host'], |
|
||||||
(string) $session[$connect]['port'], |
|
||||||
(string) $session[$connect]['code'] |
|
||||||
], |
|
||||||
$config->nps->action->welcome->debug->template |
|
||||||
) . PHP_EOL |
|
||||||
); |
|
||||||
} |
|
||||||
|
|
||||||
return sprintf( |
|
||||||
implode( |
|
||||||
PHP_EOL, |
|
||||||
$config->nps->action->welcome->message |
|
||||||
) . PHP_EOL . $image->getAsciiString() . PHP_EOL |
|
||||||
); |
|
||||||
} |
|
||||||
); |
|
||||||
|
|
||||||
// Init pending function |
|
||||||
$server->setPending( |
|
||||||
function ( |
|
||||||
string $request, |
|
||||||
string $connect |
|
||||||
): ?string |
|
||||||
{ |
|
||||||
global $config, |
|
||||||
$session; |
|
||||||
|
|
||||||
// Filter request |
|
||||||
$request = trim( |
|
||||||
$request |
|
||||||
); |
|
||||||
|
|
||||||
// Debug request on enabled |
|
||||||
if ($config->nps->action->pending->debug->enabled) |
|
||||||
{ |
|
||||||
// Print debug from template |
|
||||||
print( |
|
||||||
str_ireplace( |
|
||||||
[ |
|
||||||
'{time}', |
|
||||||
'{host}', |
|
||||||
'{port}', |
|
||||||
'{sent}', |
|
||||||
'{code}' |
|
||||||
], |
|
||||||
[ |
|
||||||
(string) date('c'), |
|
||||||
(string) $session[$connect]['host'], |
|
||||||
(string) $session[$connect]['port'], |
|
||||||
(string) $request, |
|
||||||
(string) $session[$connect]['code'] |
|
||||||
], |
|
||||||
$config->nps->action->pending->debug->template |
|
||||||
) . PHP_EOL |
|
||||||
); |
|
||||||
} |
|
||||||
|
|
||||||
// Session valid |
|
||||||
if ($session[$connect]['code'] == $request) |
|
||||||
{ |
|
||||||
// Return success message |
|
||||||
return implode( |
|
||||||
PHP_EOL, |
|
||||||
$config->nps->action->pending->message->success |
|
||||||
) . PHP_EOL; |
|
||||||
} |
|
||||||
|
|
||||||
// @TODO disconnect, stop handler |
|
||||||
|
|
||||||
// Cleanup session |
|
||||||
unset($session[$connect]); |
|
||||||
|
|
||||||
// Return failure message |
|
||||||
return implode( |
|
||||||
PHP_EOL, |
|
||||||
$config->nps->action->pending->message->failure |
|
||||||
) . PHP_EOL; |
|
||||||
} |
|
||||||
); |
|
||||||
|
|
||||||
// Init handler function |
|
||||||
$server->setHandler( |
|
||||||
function ( |
|
||||||
bool $success, |
|
||||||
string $content, |
|
||||||
string $request, |
|
||||||
string $connect |
|
||||||
): ?string |
|
||||||
{ |
|
||||||
global $config, |
|
||||||
$session; |
|
||||||
|
|
||||||
// Filter request |
|
||||||
$request = trim( |
|
||||||
$request |
|
||||||
); |
|
||||||
|
|
||||||
// Filter content |
|
||||||
$content = trim( |
|
||||||
$content |
|
||||||
); |
|
||||||
|
|
||||||
// Build response |
|
||||||
if ($session[$connect]['code'] == $request) |
|
||||||
{ |
|
||||||
// @TODO save content in blockchain with kevacoin-php |
|
||||||
|
|
||||||
$response = implode( |
|
||||||
PHP_EOL, |
|
||||||
$config->nps->action->handler->message->success |
|
||||||
) . PHP_EOL; |
|
||||||
} |
|
||||||
|
|
||||||
else |
|
||||||
{ |
|
||||||
$response = implode( |
|
||||||
PHP_EOL, |
|
||||||
$config->nps->action->handler->message->failure |
|
||||||
) . PHP_EOL; |
|
||||||
} |
|
||||||
|
|
||||||
// Debug request on enabled |
|
||||||
if ($config->nps->action->handler->debug->enabled) |
|
||||||
{ |
|
||||||
// Print debug from template |
|
||||||
print( |
|
||||||
str_ireplace( |
|
||||||
[ |
|
||||||
'{time}', |
|
||||||
'{host}', |
|
||||||
'{port}', |
|
||||||
'{code}', |
|
||||||
'{sent}', |
|
||||||
'{size}', |
|
||||||
'{data}' |
|
||||||
], |
|
||||||
[ |
|
||||||
(string) date('c'), |
|
||||||
(string) $session[$connect]['host'], |
|
||||||
(string) $session[$connect]['port'], |
|
||||||
(string) $session[$connect]['code'], |
|
||||||
(string) str_replace('%', '%%', $request), |
|
||||||
(string) mb_strlen($content), |
|
||||||
(string) PHP_EOL . $content, |
|
||||||
], |
|
||||||
$config->nps->action->handler->debug->template |
|
||||||
) . PHP_EOL |
|
||||||
); |
|
||||||
} |
|
||||||
|
|
||||||
// Cleanup session |
|
||||||
unset($session[$connect]); |
|
||||||
|
|
||||||
// Result |
|
||||||
return $response; |
|
||||||
} |
|
||||||
); |
|
||||||
|
|
||||||
// Start server |
|
||||||
if ($config->nps->action->start->debug->enabled) |
|
||||||
{ |
|
||||||
print( |
|
||||||
str_ireplace( |
|
||||||
[ |
|
||||||
'{time}', |
|
||||||
'{host}', |
|
||||||
'{port}', |
|
||||||
'{keva}' |
|
||||||
], |
|
||||||
[ |
|
||||||
date('c'), |
|
||||||
$config->nps->server->host, |
|
||||||
$config->nps->server->port, |
|
||||||
$kevacoin->getBalance( |
|
||||||
$config->kevacoin->wallet->account |
|
||||||
) |
|
||||||
], |
|
||||||
$config->nps->action->start->debug->template |
|
||||||
) . PHP_EOL |
|
||||||
); |
|
||||||
} |
|
||||||
|
|
||||||
$server->start(); |
|
Loading…
Reference in new issue