mirror of https://github.com/GOSTSec/poolserver
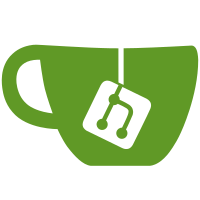
7 changed files with 200 additions and 13 deletions
@ -0,0 +1,72 @@
@@ -0,0 +1,72 @@
|
||||
#include "VarInt.h" |
||||
#include "Script.h" |
||||
#include "Transaction.h" |
||||
|
||||
using namespace Bitcoin; |
||||
|
||||
ByteBuffer& Bitcoin::operator<<(ByteBuffer& a, VarInt& b) |
||||
{ |
||||
if (b.value < 0xfd) { |
||||
a.Append(b.value, 1); |
||||
} else if (b.value <= 0xffff) { |
||||
a.Append(0xfd, 1); |
||||
a.Append(b.value, 2); |
||||
} else if (b.value <= 0xffffffff) { |
||||
a.Append(0xfe, 1); |
||||
a.Append(b.value, 4); |
||||
} else { |
||||
a.Append(0xff, 1); |
||||
a.Append(b.value, 8); |
||||
} |
||||
return a; |
||||
} |
||||
|
||||
ByteBuffer& Bitcoin::operator<<(ByteBuffer& a, Script& b) |
||||
{ |
||||
VarInt size(b.script.size()); |
||||
a << size; |
||||
a << b.script; |
||||
return a; |
||||
} |
||||
|
||||
ByteBuffer& Bitcoin::operator<<(ByteBuffer& a, OutPoint& b) |
||||
{ |
||||
a << b.hash; |
||||
a << b.n; |
||||
return a; |
||||
} |
||||
|
||||
ByteBuffer& Bitcoin::operator<<(ByteBuffer& a, TxIn& b) |
||||
{ |
||||
a << b.prevout; |
||||
a << b.script; |
||||
a << b.n; |
||||
return a; |
||||
} |
||||
|
||||
ByteBuffer& Bitcoin::operator<<(ByteBuffer& a, TxOut& b) |
||||
{ |
||||
a << b.value; |
||||
a << b.scriptPubKey; |
||||
return a; |
||||
} |
||||
|
||||
ByteBuffer& Bitcoin::operator<<(ByteBuffer& a, Transaction& b) |
||||
{ |
||||
a << b.version; |
||||
|
||||
// Inputs
|
||||
VarInt insize(b.in.size()); |
||||
a << insize; |
||||
for (uint32 i = 0; i < b.in.size(); ++i) |
||||
a << b.in[i]; |
||||
|
||||
// Outputs
|
||||
VarInt outsize(b.out.size()); |
||||
a << outsize; |
||||
for (uint32 i = 0; i < b.out.size(); ++i) |
||||
a << b.out[i]; |
||||
|
||||
a << b.lockTime; |
||||
return a; |
||||
} |
@ -0,0 +1,21 @@
@@ -0,0 +1,21 @@
|
||||
#ifndef BITCOIN_VARINT_H_ |
||||
#define BITCOIN_VARINT_H_ |
||||
|
||||
#include "Common.h" |
||||
#include "ByteBuffer.h" |
||||
|
||||
namespace Bitcoin |
||||
{ |
||||
class VarInt |
||||
{ |
||||
public: |
||||
VarInt(uint64 data) : value(data) {} |
||||
uint64 value; |
||||
|
||||
friend ByteBuffer& operator<<(ByteBuffer& a, VarInt& b); |
||||
}; |
||||
|
||||
ByteBuffer& operator<<(ByteBuffer& a, VarInt& b); |
||||
} |
||||
|
||||
#endif |
@ -0,0 +1,75 @@
@@ -0,0 +1,75 @@
|
||||
#ifndef BYTEBUFFER_H_ |
||||
#define BYTEBUFFER_H_ |
||||
|
||||
#include "Common.h" |
||||
#include <vector> |
||||
|
||||
class ByteBuffer |
||||
{ |
||||
public: |
||||
ByteBuffer& operator<<(ByteBuffer& b) |
||||
{ |
||||
Append(b.vec); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(std::vector<byte>& b) |
||||
{ |
||||
Append(b); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(uint8& b) |
||||
{ |
||||
Append(b, 1); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(uint16& b) |
||||
{ |
||||
Append(b, 2); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(uint32& b) |
||||
{ |
||||
Append(b, 4); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(uint64& b) |
||||
{ |
||||
Append(b, 8); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(int8& b) |
||||
{ |
||||
Append(b, 1); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(int16& b) |
||||
{ |
||||
Append(b, 2); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(int32& b) |
||||
{ |
||||
Append(b, 4); |
||||
return *this; |
||||
} |
||||
ByteBuffer& operator<<(int64& b) |
||||
{ |
||||
Append(b, 8); |
||||
return *this; |
||||
} |
||||
|
||||
void Append(uint64 data, size_t size) |
||||
{ |
||||
for (int i = 0; i < size; i++) |
||||
vec.push_back(data >> (i * 8)); |
||||
} |
||||
|
||||
void Append(std::vector<byte> data) |
||||
{ |
||||
vec.insert(vec.end(), data.begin(), data.end()); |
||||
} |
||||
|
||||
std::vector<byte> vec; |
||||
}; |
||||
|
||||
#endif |
Loading…
Reference in new issue