mirror of https://github.com/GOSTSec/gostexplr
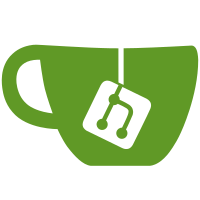
21 changed files with 225 additions and 165 deletions
@ -0,0 +1,43 @@ |
|||||||
|
#!/usr/bin/env node
|
||||||
|
var exec = require('child_process').exec; |
||||||
|
var models = require('../models'); |
||||||
|
var env = require('../config/config.json')['env'] || 'development'; |
||||||
|
var config = require(__dirname + '/../config/config.json')['database'][env]; |
||||||
|
|
||||||
|
if (process.argv.length < 4) { |
||||||
|
console.log('Provide root user name and password for mysql'); |
||||||
|
process.exit(0); |
||||||
|
} |
||||||
|
|
||||||
|
const dropUserDB = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "drop database ${config.database};drop user ${config.username}"` |
||||||
|
const createdb = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "create database ${config.database}"`; |
||||||
|
const createUser = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "create user ${config.username} identified by '${config.password}'"`; |
||||||
|
const grantAccess = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "grant all on ${config.database}.* to ${config.username}"`; |
||||||
|
|
||||||
|
exec(dropUserDB, function(err,stdout,stderr) { |
||||||
|
console.log(stdout); |
||||||
|
exec(createdb, function(err,stdout,stderr) { |
||||||
|
if (err) { |
||||||
|
console.log(err); |
||||||
|
process.exit(0); |
||||||
|
} else { |
||||||
|
console.log(stdout); |
||||||
|
exec(createUser, function(err, stdout, stderr) { |
||||||
|
if (err) { |
||||||
|
console.log(err); |
||||||
|
process.exit(0); |
||||||
|
} else { |
||||||
|
console.log(stdout); |
||||||
|
exec(grantAccess, function(err, stdout, stderr) { |
||||||
|
if (err) { |
||||||
|
console.log(err); |
||||||
|
} else { |
||||||
|
console.log(`\nUSER (${config.username}) AND DATABASE (${config.database}) CREATED SUCCESSFULLY`); |
||||||
|
console.log(stdout); |
||||||
|
} |
||||||
|
}); |
||||||
|
} |
||||||
|
}); |
||||||
|
} |
||||||
|
}); |
||||||
|
}); |
@ -1,51 +1,12 @@ |
|||||||
#!/usr/bin/env node
|
#!/usr/bin/env node
|
||||||
var exec = require('child_process').exec; |
|
||||||
var models = require('../models'); |
var models = require('../models'); |
||||||
var env = require('../config/config.json')['env'] || 'development'; |
|
||||||
var config = require(__dirname + '/../config/config.json')['database'][env]; |
|
||||||
|
|
||||||
if (process.argv.length < 4) { |
models.sequelize.sync({force: true}) |
||||||
console.log('Provide root user name and password for mysql'); |
.then(() => { |
||||||
|
console.log('Tables created'); |
||||||
|
process.exit(0); |
||||||
|
}) |
||||||
|
.catch((err) => { |
||||||
|
console.log(err); |
||||||
process.exit(0); |
process.exit(0); |
||||||
} |
|
||||||
|
|
||||||
const dropUserDB = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "drop database ${config.database};drop user ${config.username}"` |
|
||||||
const createdb = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "create database ${config.database}"`; |
|
||||||
const createUser = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "create user ${config.username} identified by '${config.password}'"`; |
|
||||||
const grantAccess = `mysql -u${process.argv[2]} -p${process.argv[3]} -e "grant all on ${config.database}.* to ${config.username}"`; |
|
||||||
|
|
||||||
exec(dropUserDB, function(err,stdout,stderr) { |
|
||||||
console.log(stdout); |
|
||||||
exec(createdb, function(err,stdout,stderr) { |
|
||||||
if (err) { |
|
||||||
console.log(err); |
|
||||||
process.exit(0); |
|
||||||
} else { |
|
||||||
console.log(stdout); |
|
||||||
exec(createUser, function(err, stdout, stderr) { |
|
||||||
if (err) { |
|
||||||
console.log(err); |
|
||||||
process.exit(0); |
|
||||||
} else { |
|
||||||
console.log(stdout); |
|
||||||
exec(grantAccess, function(err, stdout, stderr) { |
|
||||||
if (err) { |
|
||||||
console.log(err); |
|
||||||
} else { |
|
||||||
console.log(stdout); |
|
||||||
models.sequelize.sync({force: true}) |
|
||||||
.then(() => { |
|
||||||
console.log(`\nUSER (${config.username}) AND DATABASE (${config.database}) CREATED SUCCESSFULLY`); |
|
||||||
process.exit(0); |
|
||||||
}) |
|
||||||
.catch((err) => { |
|
||||||
console.log(err); |
|
||||||
process.exit(0); |
|
||||||
}); |
|
||||||
} |
|
||||||
}); |
|
||||||
} |
|
||||||
}); |
|
||||||
} |
|
||||||
}); |
|
||||||
}); |
}); |
||||||
|
@ -1,15 +0,0 @@ |
|||||||
extends layout |
|
||||||
|
|
||||||
block content |
|
||||||
h3 Address |
|
||||||
div= address |
|
||||||
|
|
||||||
h3 Transactions |
|
||||||
each val in txes |
|
||||||
div |
|
||||||
a(href='/transaction/#{val.txid}/') #{val.txid} |
|
||||||
div.pagination |
|
||||||
if prevpage |
|
||||||
a(href='/address/#{address}/#{prevpage}/', style='float:left') Back |
|
||||||
if nextpage |
|
||||||
a(href='/address/#{address}/#{nextpage}/', style='float:right') Next |
|
@ -0,0 +1,21 @@ |
|||||||
|
extends layout |
||||||
|
|
||||||
|
block content |
||||||
|
h3 Address |
||||||
|
div= address.address |
||||||
|
|
||||||
|
h3 Transactions |
||||||
|
table |
||||||
|
each vout in address.Vouts |
||||||
|
tr |
||||||
|
if vout.Transactions[0].TransactionVouts.direction == 1 |
||||||
|
td OUT |
||||||
|
else |
||||||
|
td IN |
||||||
|
td |
||||||
|
a(href=`/transaction/${vout.Transactions[0].txid}/`) #{vout.Transactions[0].txid} |
||||||
|
div.pagination |
||||||
|
if prevpage |
||||||
|
a(href=`/address/#{address}/${prevpage}/`, style='float:left') Back |
||||||
|
if nextpage |
||||||
|
a(href=`/address/${address}/${nextpage}/`, style='float:right') Next |
@ -1,37 +0,0 @@ |
|||||||
extends layout |
|
||||||
|
|
||||||
block content |
|
||||||
h3 Transaction |
|
||||||
|
|
||||||
table |
|
||||||
tr |
|
||||||
td Hash |
|
||||||
td #{transaction.txid} |
|
||||||
tr |
|
||||||
td Block |
|
||||||
td |
|
||||||
a(href='/block/#{transaction.Block.hash}/') #{transaction.Block.hash} |
|
||||||
tr |
|
||||||
td Block time |
|
||||||
td #{transaction.blockTime} |
|
||||||
tr |
|
||||||
td Confirmations |
|
||||||
td #{confirmations} |
|
||||||
|
|
||||||
h3 In |
|
||||||
if transaction.txtx.length |
|
||||||
table |
|
||||||
each val in transaction.txtx |
|
||||||
tr |
|
||||||
td |
|
||||||
a(href='/transaction/#{val.txid}/') #{val.txid} |
|
||||||
else |
|
||||||
div Mined |
|
||||||
|
|
||||||
h3 Out |
|
||||||
table |
|
||||||
each val in vouts |
|
||||||
tr |
|
||||||
td |
|
||||||
a(href='/address/#{val.address}/') #{val.address} |
|
||||||
td #{val.value} |
|
@ -0,0 +1,54 @@ |
|||||||
|
extends layout |
||||||
|
|
||||||
|
block content |
||||||
|
h3 Transaction |
||||||
|
|
||||||
|
table |
||||||
|
tr |
||||||
|
td Hash |
||||||
|
td #{transaction.txid} |
||||||
|
tr |
||||||
|
td Block |
||||||
|
td |
||||||
|
a(href=`/block/${transaction.Block.hash}/`) #{transaction.Block.hash} |
||||||
|
tr |
||||||
|
td Block time |
||||||
|
td #{transaction.blockTime} |
||||||
|
tr |
||||||
|
td Confirmations |
||||||
|
td #{confirmations} |
||||||
|
|
||||||
|
h3 In |
||||||
|
if transaction.vins.length |
||||||
|
table |
||||||
|
tr |
||||||
|
th Address |
||||||
|
th Value |
||||||
|
th Transaction |
||||||
|
each vin in transaction.vins |
||||||
|
tr |
||||||
|
td |
||||||
|
a(href=`/address/${vin.Addresses[0].address}/`) #{vin.Addresses[0].address} |
||||||
|
td #{vin.value} |
||||||
|
td |
||||||
|
a(href=`/transaction/${vin.Transactions[0].txid}/`) #{vin.Transactions[0].txid} |
||||||
|
else |
||||||
|
div Mined |
||||||
|
|
||||||
|
h3 Out |
||||||
|
table |
||||||
|
tr |
||||||
|
th Address |
||||||
|
th Value |
||||||
|
th Transaction |
||||||
|
each vout in transaction.vouts |
||||||
|
each address in vout.Addresses |
||||||
|
tr |
||||||
|
td |
||||||
|
a(href=`/address/${address.address}/`) #{address.address} |
||||||
|
td #{vout.value} |
||||||
|
td |
||||||
|
if vout.Transactions[0].TransactionVouts.direction == 0 |
||||||
|
a(href=`/transaction/${vout.Transactions[0].txid}/`) #{vout.Transactions[0].txid} |
||||||
|
else |
||||||
|
span No yet |
Loading…
Reference in new issue