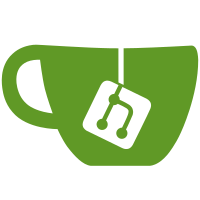
@ -0,0 +1,36 @@
@@ -0,0 +1,36 @@
|
||||
twister-html authors |
||||
==================== |
||||
|
||||
Miguel Freitas |
||||
Original javascript code and basic UI functionality |
||||
|
||||
Lucas Leal |
||||
Original theme, CSS + artwork |
||||
|
||||
Msjoinder |
||||
Polyglot support (internationalization), multiple fixes |
||||
|
||||
erkan yüksel |
||||
Image preview, automatic unicode conversion, split long posts, hiding posts with mentions and more. |
||||
|
||||
Hedgehog |
||||
Calm theme, CSS + artwork and more. |
||||
|
||||
Denis Ryabov |
||||
Tox and bitmessage profile fields, multiple fixes |
||||
|
||||
Giacomo Lacava |
||||
i18n branch maintainer, fixes |
||||
|
||||
BlockTester |
||||
Secret key button, exit button, fixes |
||||
|
||||
Dionysis Zindros |
||||
Several visual fixes |
||||
|
||||
digital-dreamer |
||||
Guest mode browsing |
||||
|
||||
Mylène Bressan |
||||
Nin theme |
||||
|
@ -0,0 +1,21 @@
@@ -0,0 +1,21 @@
|
||||
The MIT License (MIT) |
||||
|
||||
Copyright (c) 2013-2014 Miguel Freitas, Lucas Leal and twister project developers. |
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy |
||||
of this software and associated documentation files (the "Software"), to deal |
||||
in the Software without restriction, including without limitation the rights |
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
||||
copies of the Software, and to permit persons to whom the Software is |
||||
furnished to do so, subject to the following conditions: |
||||
|
||||
The above copyright notice and this permission notice shall be included in |
||||
all copies or substantial portions of the Software. |
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN |
||||
THE SOFTWARE. |
After Width: | Height: | Size: 4.8 KiB |
After Width: | Height: | Size: 3.0 KiB |
@ -1,463 +0,0 @@
@@ -1,463 +0,0 @@
|
||||
// interface_common.js
|
||||
// 2013 Lucas Leal, Miguel Freitas
|
||||
//
|
||||
// Common interface functions to all pages, modal manipulation, button manipulation etc
|
||||
// Profile, mentions and hashtag modal
|
||||
// Post actions: submit, count characters
|
||||
|
||||
//dispara o modal genérico
|
||||
//o modalClass me permite fazer tratamentos específicos de CSS para cada modal
|
||||
function openModal( modalClass ) |
||||
{ |
||||
var $oldModal = $("body").children(".modal-blackout"); |
||||
var $template = $( "#templates" ); |
||||
var $templateModal = $template.find( ".modal-blackout" ).clone(true); |
||||
|
||||
$templateModal.addClass( modalClass ); |
||||
if( $oldModal.length ) { |
||||
$templateModal.show(); |
||||
$oldModal.replaceWith($templateModal); |
||||
} else { |
||||
$templateModal.prependTo( "body" ).fadeIn( "fast" ); |
||||
} |
||||
|
||||
//escondo o overflow da tela
|
||||
var $body = $( "body" ); |
||||
$body.css({ |
||||
"overflow": "hidden", |
||||
}) |
||||
} |
||||
|
||||
//fecha o modal removendo o conteúdo por detach
|
||||
function closeModal($this) |
||||
{ |
||||
var $body = $( "body" ); |
||||
var $modalWindows = $( "body" ).children( ".modal-blackout" ); |
||||
|
||||
$modalWindows.fadeOut( "fast", function() |
||||
{ |
||||
$modalWindows.detach(); |
||||
}); |
||||
$body.css({ |
||||
"overflow": "auto", |
||||
"margin-right": "0" |
||||
}); |
||||
} |
||||
|
||||
function checkNetworkStatusAndAskRedirect(cbFunc, cbArg) { |
||||
networkUpdate(function(args) { |
||||
if( !twisterdConnectedAndUptodate ) { |
||||
var redirect = |
||||
window.confirm(polyglot.t("switch_to_network")); |
||||
if( redirect ) |
||||
$.MAL.goNetwork(); |
||||
} else { |
||||
if( args.cbFunc ) |
||||
args.cbFunc(args.cbArg); |
||||
} |
||||
}, {cbFunc:cbFunc,cbArg:cbArg}); |
||||
} |
||||
|
||||
function timeGmtToText(t) { |
||||
var d = new Date(0); |
||||
d.setUTCSeconds(t); |
||||
return d.toString().replace(/GMT.*/g,""); |
||||
} |
||||
|
||||
function timeSincePost(t) { |
||||
var d = new Date(0); |
||||
d.setUTCSeconds(t); |
||||
var now = new Date(); |
||||
var t_delta = Math.ceil((now - d) / 1000); |
||||
var expression = ""; |
||||
if(t_delta < 60) { |
||||
expression = polyglot.t("seconds", t_delta); |
||||
} |
||||
else if(t_delta < 60 * 60) { |
||||
expression = polyglot.t("minutes", Math.floor(t_delta/60)); |
||||
} |
||||
else if(t_delta < 24 * 60 * 60) { |
||||
expression = polyglot.t("hours", Math.floor(t_delta/60/60)); |
||||
} |
||||
else { |
||||
expression = polyglot.t("days", Math.floor(t_delta/24/60/60)); |
||||
} |
||||
return polyglot.t("time_ago", { time: expression }); |
||||
} |
||||
|
||||
//
|
||||
// Profile, mentions, hashtag, and following modal
|
||||
// -----------------------------------
|
||||
|
||||
function newProfileModal(username) { |
||||
var profileModalContent = $( "#profile-modal-template" ).children().clone(true); |
||||
|
||||
updateProfileData(profileModalContent, username); |
||||
|
||||
return profileModalContent; |
||||
} |
||||
|
||||
function openProfileModal(e) |
||||
{ |
||||
e.stopPropagation(); |
||||
e.preventDefault(); |
||||
|
||||
var $this = $( this ); |
||||
var username = $.MAL.urlToUser( $this.attr("href") ); |
||||
|
||||
var profileModalClass = "profile-modal"; |
||||
openModal( profileModalClass ); |
||||
|
||||
var profileModalContent = newProfileModal( username ); |
||||
profileModalContent.appendTo("." +profileModalClass + " .modal-content"); |
||||
|
||||
//título do modal
|
||||
$( "."+profileModalClass + " h3" ).text( polyglot.t("users_profile", { username: username }) ); |
||||
} |
||||
|
||||
function newHashtagModal(hashtag) { |
||||
var hashtagModalContent = $( "#hashtag-modal-template" ).children().clone(true); |
||||
hashtagModalContent.find( ".postboard-news").click(function (){ |
||||
$(this).hide(); |
||||
displayHashtagPending($(".hashtag-modal .postboard-posts")); |
||||
}); |
||||
|
||||
clearHashtagProcessed(); |
||||
updateHashtagModal( hashtagModalContent.find(".postboard-posts"), hashtag ); |
||||
|
||||
return hashtagModalContent; |
||||
} |
||||
|
||||
function openHashtagModal(e) |
||||
{ |
||||
e.stopPropagation(); |
||||
e.preventDefault(); |
||||
|
||||
var $this = $( this ); |
||||
var hashtag = $this.text().substring(1); |
||||
|
||||
var hashtagModalClass = "hashtag-modal"; |
||||
openModal( hashtagModalClass ); |
||||
$( "."+hashtagModalClass ).attr("data-resource","hashtag"); |
||||
|
||||
var hashtagModalContent = newHashtagModal( hashtag ); |
||||
hashtagModalContent.appendTo("." +hashtagModalClass + " .modal-content"); |
||||
|
||||
//título do modal
|
||||
$( "."+hashtagModalClass + " h3" ).text( "#" + hashtag ); |
||||
} |
||||
|
||||
function updateHashtagModal(postboard,hashtag) { |
||||
var $hashtagModalClass = $(".hashtag-modal"); |
||||
if( !$hashtagModalClass.length || $hashtagModalClass.css("display") == 'none' ) |
||||
return; |
||||
|
||||
var resource = $hashtagModalClass.attr("data-resource"); |
||||
|
||||
requestHashtag(postboard,hashtag,resource); |
||||
setTimeout( function() {updateHashtagModal(postboard,hashtag);}, 5000); |
||||
} |
||||
|
||||
function openMentionsModal(e) |
||||
{ |
||||
e.stopPropagation(); |
||||
e.preventDefault(); |
||||
|
||||
// reuse the same hashtag modal to show mentions
|
||||
var hashtagModalClass = "hashtag-modal"; |
||||
openModal( hashtagModalClass ); |
||||
$( "."+hashtagModalClass ).attr("data-resource","mention"); |
||||
|
||||
var username; |
||||
var $userInfo = $(this).closest("[data-screen-name]"); |
||||
if( $userInfo.length ) |
||||
username = $userInfo.attr("data-screen-name"); |
||||
else |
||||
username = defaultScreenName; |
||||
|
||||
var hashtagModalContent = newHashtagModal( username ); |
||||
hashtagModalContent.appendTo("." +hashtagModalClass + " .modal-content"); |
||||
|
||||
//título do modal
|
||||
$( "."+hashtagModalClass + " h3" ).text( polyglot.t("users_mentions", { username: username }) ); |
||||
|
||||
// obtain already cached mention posts from twister_newmsgs.js
|
||||
processHashtag(hashtagModalContent.find(".postboard-posts"), defaultScreenName, getMentionsData() ); |
||||
resetMentionsCount(); |
||||
} |
||||
|
||||
function newFollowingModal(username) { |
||||
var followingModalContent = $( "#following-modal-template" ).children().clone(true); |
||||
|
||||
updateFollowingData(followingModalContent, username); |
||||
|
||||
return followingModalContent; |
||||
} |
||||
|
||||
function openFollowingModal(e) |
||||
{ |
||||
e.stopPropagation(); |
||||
e.preventDefault(); |
||||
|
||||
var $this = $( this ); |
||||
var username = $.MAL.followingUrlToUser( $this.attr("href") ); |
||||
|
||||
var followingModalClass = "following-modal"; |
||||
openModal( followingModalClass ); |
||||
|
||||
var followingModalContent = newFollowingModal( username ); |
||||
followingModalContent.appendTo("." +followingModalClass + " .modal-content"); |
||||
|
||||
//título do modal
|
||||
$( "."+followingModalClass + " h3" ).text( polyglot.t("followed_by", { username: username }) ); |
||||
} |
||||
|
||||
//
|
||||
// Post actions, submit, count characters
|
||||
// --------------------------------------
|
||||
//dispara o modal de retweet
|
||||
var reTwistPopup = function( e ) |
||||
{ |
||||
var reTwistClass = "reTwist"; |
||||
openModal( reTwistClass ); |
||||
|
||||
//título do modal
|
||||
$( ".reTwist h3" ).text( polyglot.t("retransmit_this") ); |
||||
|
||||
var postdata = $(this).parents(".post-data").attr("data-userpost"); |
||||
var postElem = postToElem($.evalJSON(postdata),""); |
||||
postElem.appendTo( ".reTwist .modal-content" ); |
||||
|
||||
e.stopPropagation(); |
||||
} |
||||
|
||||
//Expande Área do Novo post
|
||||
var replyInitPopup = function(e, post) |
||||
{ |
||||
var replyClass = "reply"; |
||||
openModal( replyClass ); |
||||
|
||||
//título do modal
|
||||
var fullname = post.find(".post-info-name").text(); |
||||
$( ".reply h3" ).text( polyglot.t("reply_to", { fullname: fullname }) ); |
||||
|
||||
//para poder exibir a thread selecionada...
|
||||
var replyModalContent = $(".reply .modal-content").hide(); |
||||
var retweetContent = $( "#reply-modal-template" ).children().clone(true); |
||||
retweetContent.appendTo(replyModalContent); |
||||
|
||||
var postdata = post.find(".post-data").attr("data-userpost"); |
||||
var postElem = postToElem($.evalJSON(postdata),""); |
||||
postElem.appendTo(replyModalContent); |
||||
|
||||
var replyArea = $(".reply .post-area .post-area-new"); |
||||
replyArea.addClass("open"); |
||||
var replyText = replyArea.find("textarea"); |
||||
var postInlineReplyText = $(".reply .post .post-area-new textarea"); |
||||
|
||||
var attrToCopy = ["placeholder", "data-reply-to"]; |
||||
$.each(attrToCopy, function( i, attribute ) { |
||||
replyText.attr( attribute, postInlineReplyText.attr(attribute) ); |
||||
}); |
||||
composeNewPost(e, replyArea); |
||||
|
||||
replyModalContent.fadeIn( "fast" ); |
||||
} |
||||
|
||||
//abre o menu dropdown de configurações
|
||||
var dropDownMenu = function( e ) |
||||
{ |
||||
var $configMenu = $( ".config-menu" ); |
||||
$configMenu.slideToggle( "fast" ); |
||||
e.stopPropagation(); |
||||
} |
||||
|
||||
//fecha o config menu ao clicar em qualquer lugar da tela
|
||||
var closeThis = function() |
||||
{ |
||||
$( this ).slideUp( "fast" ); |
||||
}; |
||||
|
||||
var postExpandFunction = function( e, postLi ) |
||||
{ |
||||
if( !postLi.hasClass( "original" ) ) { |
||||
return; |
||||
} |
||||
|
||||
var originalPost = postLi.find(".post-data.original"); |
||||
var $postInteractionText = originalPost.find( ".post-expand" ); |
||||
var $postExpandedContent = originalPost.find( ".expanded-content" ); |
||||
var $postsRelated = postLi.find(".related"); |
||||
|
||||
var openClass = "open"; |
||||
if( !postLi.hasClass( openClass ) ) { |
||||
originalPost.detach(); |
||||
postLi.empty(); |
||||
postLi.addClass( openClass ); |
||||
$postInteractionText.text( polyglot.t("Collapse") ); |
||||
|
||||
var itemOl = $("<ol/>", {class:"expanded-post"}).appendTo(postLi); |
||||
var originalLi = $("<li/>", {class: "module post original"}).appendTo(itemOl); |
||||
originalLi.append(originalPost); |
||||
|
||||
$postExpandedContent.slideDown( "fast" ); |
||||
|
||||
// insert "reply_to" before
|
||||
requestRepliedBefore(originalLi); |
||||
// insert replies to this post after
|
||||
requestRepliesAfter(originalLi); |
||||
// RTs faces and counter
|
||||
requestRTs(originalLi); |
||||
} |
||||
else |
||||
{ |
||||
postLi.removeClass( openClass ); |
||||
$postInteractionText.text( polyglot.t("Expand") ); |
||||
|
||||
if( $postsRelated ) $postsRelated.slideUp( "fast" ); |
||||
$postExpandedContent.slideUp( "fast", function() |
||||
{ |
||||
originalPost.detach(); |
||||
postLi.empty(); |
||||
postLi.append(originalPost); |
||||
}); |
||||
} |
||||
|
||||
e.stopPropagation(); |
||||
} |
||||
|
||||
var postReplyClick = function( e ) |
||||
{ |
||||
var post = $(this).closest(".post"); |
||||
if( !post.hasClass( "original" ) ) { |
||||
replyInitPopup(e, post); |
||||
} else { |
||||
var postLiOpen = post.parents(".post.open"); |
||||
if( !postLiOpen.length ) { |
||||
postExpandFunction(e, post); |
||||
} |
||||
var postAreaNew = post.find(".post-area-new") |
||||
composeNewPost(e, postAreaNew); |
||||
} |
||||
e.stopPropagation(); |
||||
} |
||||
|
||||
//Expande Área do Novo post
|
||||
var composeNewPost = function( e, postAreaNew ) |
||||
{ |
||||
e.stopPropagation(); |
||||
if( !postAreaNew.hasClass("open") ) { |
||||
postAreaNew.addClass( "open" ); |
||||
//se o usuário clicar fora é pra fechar
|
||||
postAreaNew.clickoutside( unfocusThis ) |
||||
} |
||||
|
||||
var textArea = postAreaNew.find("textarea"); |
||||
textArea.focus(); |
||||
if( textArea.attr("data-reply-to") && !textArea.val().length ) { |
||||
textArea.val(textArea.attr("data-reply-to")); |
||||
} |
||||
} |
||||
|
||||
//Reduz Área do Novo post
|
||||
var unfocusThis = function() |
||||
{ |
||||
var $this = $( this ); |
||||
$this.removeClass( "open" ); |
||||
} |
||||
|
||||
function replyTextKeypress(e) { |
||||
e = e || event; |
||||
var $this = $( this ); |
||||
var tweetForm = $this.parents("form"); |
||||
if( tweetForm != undefined ) { |
||||
var c = 140 - $this.val().length; |
||||
var remainingCount = tweetForm.find(".post-area-remaining"); |
||||
remainingCount.text(c); |
||||
if( c < 0 ) remainingCount.addClass("warn"); |
||||
else remainingCount.removeClass("warn"); |
||||
|
||||
var tweetAction = tweetForm.find(".post-submit"); |
||||
if( !tweetAction.length ) tweetAction = tweetForm.find(".dm-submit"); |
||||
if( c >= 0 && c < 140 && |
||||
$this.val() != $this.attr("data-reply-to") ) { |
||||
$.MAL.enableButton(tweetAction); |
||||
} else { |
||||
$.MAL.disableButton(tweetAction); |
||||
} |
||||
|
||||
if (e.keyCode === 13) { |
||||
if (!e.ctrlKey) { |
||||
$this.val($this.val().trim()); |
||||
if( !tweetAction.hasClass("disabled") ) { |
||||
tweetAction.click(); |
||||
} |
||||
} else { |
||||
$this.val($this.val() + "\r"); |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
||||
var postSubmit = function(e) |
||||
{ |
||||
e.stopPropagation(); |
||||
e.preventDefault(); |
||||
var $this = $( this ); |
||||
var $replyText = $this.closest(".post-area-new").find("textarea"); |
||||
|
||||
var $postOrig = $this.closest(".post-data"); |
||||
|
||||
if (!$postOrig.length) { |
||||
$postOrig = $this.closest(".modal-content").find(".post-data"); |
||||
} |
||||
|
||||
newPostMsg($replyText.val(), $postOrig); |
||||
|
||||
$replyText.val(""); |
||||
$replyText.attr("placeholder", polyglot.t("Your message was sent!")); |
||||
var tweetForm = $this.parents("form"); |
||||
var remainingCount = tweetForm.find(".post-area-remaining"); |
||||
remainingCount.text(140); |
||||
$replyText.attr("placeholder", "Your message was sent!"); |
||||
closeModal($this); |
||||
} |
||||
|
||||
|
||||
var retweetSubmit = function(e) |
||||
{ |
||||
e.stopPropagation(); |
||||
e.preventDefault(); |
||||
var $this = $( this ); |
||||
|
||||
var $postOrig = $this.closest(".modal-wrapper").find(".post-data"); |
||||
|
||||
newRtMsg($postOrig); |
||||
|
||||
closeModal($this); |
||||
} |
||||
|
||||
|
||||
|
||||
function initInterfaceCommon() { |
||||
$( "body" ).on( "click", ".cancel" , function() { closeModal($(this)); } ); |
||||
$( ".post-reply" ).bind( "click", postReplyClick ); |
||||
$( ".post-propagate" ).bind( "click", reTwistPopup ); |
||||
$( ".userMenu-config-dropdown" ).bind( "click", dropDownMenu ); |
||||
$( ".config-menu" ).clickoutside( closeThis ); |
||||
$( ".module.post" ).bind( "click", function(e) { |
||||
postExpandFunction(e,$(this)); }); |
||||
$( ".post-area-new" ).bind( "click", function(e) { |
||||
composeNewPost(e,$(this));} ); |
||||
$( ".post-area-new" ).clickoutside( unfocusThis ); |
||||
$( ".post-submit").click( postSubmit ); |
||||
$( ".modal-propagate").click( retweetSubmit ); |
||||
|
||||
var $replyText = $( ".post-area-new textarea" ); |
||||
$replyText.keyup( replyTextKeypress ); |
||||
|
||||
$( ".open-profile-modal").bind( "click", openProfileModal ); |
||||
$( ".open-hashtag-modal").bind( "click", openHashtagModal ); |
||||
$( ".open-following-modal").bind( "click", openFollowingModal ); |
||||
$( ".userMenu-connections a").bind( "click", openMentionsModal ); |
||||
} |
@ -1,85 +0,0 @@
@@ -1,85 +0,0 @@
|
||||
// interface_home.js
|
||||
// 2013 Lucas Leal, Miguel Freitas
|
||||
//
|
||||
// Specific interface functions for home.html
|
||||
|
||||
//***********************************************
|
||||
//******************* DECLARATIONS **************
|
||||
//***********************************************
|
||||
var InterfaceFunctions = function() |
||||
{ |
||||
//faço os binds no init
|
||||
this.init = function() |
||||
{ |
||||
$( ".wrapper .postboard-news").click(function() { |
||||
requestTimelineUpdate("latest",postsPerRefresh,followingUsers);}); |
||||
|
||||
initInterfaceCommon(); |
||||
initUserSearch(); |
||||
initInterfaceDirectMsg(); |
||||
|
||||
initUser(initHome); |
||||
} |
||||
|
||||
function initHome(cbFunc, cbArg) { |
||||
if( !defaultScreenName ) { |
||||
alert(polyglot.t("username_undefined")); |
||||
$.MAL.goLogin(); |
||||
return; |
||||
} |
||||
checkNetworkStatusAndAskRedirect(); |
||||
|
||||
//$("span.screen-name").text('@' + user);
|
||||
var $miniProfile = $(".mini-profile"); |
||||
$miniProfile.find("a.mini-profile-name").attr("href",$.MAL.userUrl(defaultScreenName)); |
||||
$miniProfile.find("a.open-profile-modal").attr("href",$.MAL.userUrl(defaultScreenName)); |
||||
$miniProfile.find(".mini-profile-name").text(defaultScreenName); |
||||
getFullname( defaultScreenName, $miniProfile.find(".mini-profile-name") ); |
||||
getAvatar( defaultScreenName, $miniProfile.find(".mini-profile-photo").find("img") ); |
||||
getPostsCount( defaultScreenName, $miniProfile.find(".posts-count") ); |
||||
getFollowers( defaultScreenName, $miniProfile.find(".followers-count") ); |
||||
|
||||
loadFollowing( function(args) { |
||||
$(".mini-profile .following-count").text(followingUsers.length-1); |
||||
requestLastHave(); |
||||
setInterval("requestLastHave()", 1000); |
||||
initMentionsCount(); |
||||
initDMsCount(); |
||||
requestTimelineUpdate("latestFirstTime",postsPerRefresh,followingUsers); |
||||
|
||||
// install scrollbottom handler to load more posts as needed
|
||||
$(window).scroll(function(){ |
||||
if ($(window).scrollTop() >= $(document).height() - $(window).height() - 20){ |
||||
if( timelineLoaded ) { |
||||
requestTimelineUpdate("older", postsPerRefresh, followingUsers); |
||||
} |
||||
} |
||||
}); |
||||
|
||||
setTimeout("getRandomFollowSuggestion(processSuggestion)", 1000); |
||||
setTimeout("getRandomFollowSuggestion(processSuggestion)", 1000); |
||||
setTimeout("getRandomFollowSuggestion(processSuggestion)", 1000); |
||||
if( args.cbFunc ) |
||||
args.cbFunc(args.cbArg); |
||||
}, {cbFunc:cbFunc, cbArg:cbArg}); |
||||
} |
||||
} |
||||
|
||||
//***********************************************
|
||||
//******************* INIT **************
|
||||
//***********************************************
|
||||
var interfaceFunctions = new InterfaceFunctions; |
||||
$( document ).ready( interfaceFunctions.init ); |
||||
|
||||
|
||||
//função no window que fixa o header das postagens
|
||||
function fixDiv() |
||||
{ |
||||
var $cache = $('.postboard h2'); |
||||
if ($(window).scrollTop() > 26) |
||||
$cache.addClass( "fixed" ); |
||||
else |
||||
$cache.removeClass( "fixed" ); |
||||
} |
||||
|
||||
$(window).scroll(fixDiv); |
@ -0,0 +1,35 @@
@@ -0,0 +1,35 @@
|
||||
$(function(){ |
||||
$('#showqr').on('click', function(){ |
||||
if($('#qrcode img')[0]) return; |
||||
var skey = document.getElementById('skey').innerText; |
||||
new QRCode(document.getElementById("qrcode"), skey); |
||||
}); |
||||
$('.tox-ctc').on('click', function(){ |
||||
window.prompt('Press Ctrl/Cmd+C to copy then Enter to close', $(this).attr('data')) |
||||
}) |
||||
$('.bitmessage-ctc').on('click', function(){ |
||||
window.prompt('Press Ctrl/Cmd+C to copy then Enter to close', $(this).attr('data')) |
||||
}) |
||||
}) |
||||
|
||||
|
||||
function dhtIndicatorBg(){ |
||||
var bgcolor = ''; |
||||
if(twisterDhtNodes <= 20){bgcolor = '#770900' |
||||
}else if(twisterDhtNodes <= 60){bgcolor = '#773400' |
||||
}else if(twisterDhtNodes <= 90){bgcolor = '#774c00' |
||||
}else if(twisterDhtNodes <= 120){bgcolor = '#776400' |
||||
}else if(twisterDhtNodes <= 150){bgcolor = '#707500' |
||||
}else if(twisterDhtNodes <= 180){bgcolor = '#3f6900' |
||||
}else if(twisterDhtNodes <= 210){bgcolor = '#005f15' |
||||
}else if(twisterDhtNodes >= 250){bgcolor = '#009922' |
||||
} |
||||
$('.userMenu-dhtindicator').animate({'background-color': bgcolor }); |
||||
}; |
||||
setTimeout(dhtIndicatorBg, 300); |
||||
setTimeout(function() {setInterval(dhtIndicatorBg, 2000)}, 400); |
||||
|
||||
|
||||
function homeIntInit () { |
||||
setTimeout(mensAutocomplete, 800); |
||||
} |
@ -0,0 +1,177 @@
@@ -0,0 +1,177 @@
|
||||
// interface_home.js
|
||||
// 2013 Lucas Leal, Miguel Freitas
|
||||
//
|
||||
// Specific interface functions for home.html
|
||||
|
||||
var promotedPostsOnly = false; |
||||
|
||||
//***********************************************
|
||||
//******************* DECLARATIONS **************
|
||||
//***********************************************
|
||||
var InterfaceFunctions = function() |
||||
{ |
||||
//faço os binds no init
|
||||
this.init = function() |
||||
{ |
||||
$( ".wrapper .postboard-news").click(function() { |
||||
requestTimelineUpdate("latest",postsPerRefresh,followingUsers,promotedPostsOnly);}); |
||||
|
||||
|
||||
/*$( ".promoted-posts-only").click(function() { |
||||
promotedPostsOnly = !promotedPostsOnly; |
||||
$(this).text( promotedPostsOnly ? "Switch to Normal posts" : "Switch to Promoted posts" ); |
||||
timelineChangedUser(); |
||||
$.MAL.getStreamPostsParent().empty(); |
||||
requestTimelineUpdate("latestFirstTime",postsPerRefresh,followingUsers,promotedPostsOnly); |
||||
});*/ |
||||
|
||||
|
||||
// Add refresh posts for home link in menu
|
||||
$( ".userMenu-home.current a").click(function() { |
||||
requestTimelineUpdate("latest",postsPerRefresh,followingUsers,promotedPostsOnly);}); |
||||
|
||||
// modified the way promoted posts are shown
|
||||
$( ".promoted-posts-only").click(function() { |
||||
promotedPostsOnly = !promotedPostsOnly; |
||||
//active promoted posts tab
|
||||
$(this).children('.promoted-posts').addClass(promotedPostsOnly ? "active" : "disabled"); |
||||
$(this).children('.normal-posts').addClass(promotedPostsOnly ? "disabled" : "active"); |
||||
$('#postboard-top').removeClass(promotedPostsOnly ? "show" : "hide"); |
||||
//active normal posts
|
||||
$(this).children('.promoted-posts').removeClass(promotedPostsOnly ? "disabled" : "active"); |
||||
$(this).children('.normal-posts').removeClass(promotedPostsOnly ? "active" : "disabled"); |
||||
$('#postboard-top').addClass(promotedPostsOnly ? "hide" : "show"); |
||||
|
||||
timelineChangedUser(); |
||||
$.MAL.getStreamPostsParent().empty(); |
||||
requestTimelineUpdate("latestFirstTime",postsPerRefresh,followingUsers,promotedPostsOnly); |
||||
}); |
||||
|
||||
initInterfaceCommon(); |
||||
initUserSearch(); |
||||
initInterfaceDirectMsg(); |
||||
|
||||
initUser(initHome); |
||||
}; |
||||
|
||||
function initHome(cbFunc, cbArg) { |
||||
checkNetworkStatusAndAskRedirect(); |
||||
|
||||
//$("span.screen-name").text('@' + user);
|
||||
var $miniProfile = $(".mini-profile"); |
||||
if(!defaultScreenName) |
||||
{ |
||||
$(".userMenu-profile > a").text(polyglot.t("Login")); |
||||
$(".userMenu-profile > a").attr("href","login.html"); |
||||
$(".post-area-new > textarea").attr("placeholder",polyglot.t("You have to log in to post messages.")); |
||||
$(".post-area-new > textarea").attr("disabled","true"); |
||||
$miniProfile.find(".mini-profile-name").text("guest"); |
||||
$miniProfile.find(".posts-count").text("0"); |
||||
$miniProfile.find(".following-count").text("0"); |
||||
$miniProfile.find(".followers-count").text("0"); |
||||
$miniProfile.find("a.open-following-page").attr("href","#"); |
||||
$miniProfile.find("a.open-following-page").bind("click", function() |
||||
{ alert(polyglot.t("You are not following anyone because you are not logged in."))} ); |
||||
$miniProfile.find("a.open-followers").bind("click", function() |
||||
{ alert(polyglot.t("You don't have any followers because you are not logged in."))} ); |
||||
$(".dropdown-menu-following").attr("href","#"); |
||||
$(".dropdown-menu-following").bind("click", function() |
||||
{ alert(polyglot.t("You are not following anyone because you are not logged in."))} ); |
||||
twisterRpc("gettrendinghashtags", [10], |
||||
function(args, ret) { |
||||
for( var i = 0; i < ret.length; i++ ) { |
||||
|
||||
var $li = $("<li>"); |
||||
var hashtagLinkTemplate = $("#hashtag-link-template").clone(true); |
||||
hashtagLinkTemplate.removeAttr("id"); |
||||
hashtagLinkTemplate.attr("href",$.MAL.hashtagUrl(ret[i])); |
||||
hashtagLinkTemplate.text("#"+ret[i]); |
||||
$li.append(hashtagLinkTemplate); |
||||
$(".toptrends-list").append($li); |
||||
} |
||||
}, {}, |
||||
function(args, ret) { |
||||
console.log("Error with gettrendinghashtags. Older twister daemon?"); |
||||
}, {}); |
||||
} |
||||
else |
||||
{ |
||||
$miniProfile.find("a.mini-profile-name").attr("href",$.MAL.userUrl(defaultScreenName)); |
||||
$miniProfile.find("a.open-profile-modal").attr("href",$.MAL.userUrl(defaultScreenName)); |
||||
$miniProfile.find(".mini-profile-name").text(defaultScreenName); |
||||
getFullname( defaultScreenName, $miniProfile.find(".mini-profile-name") ); |
||||
getAvatar( defaultScreenName, $miniProfile.find(".mini-profile-photo").find("img") ); |
||||
// add avatar in postboard-top
|
||||
getAvatar( defaultScreenName, $("#postboard-top").find("img") ); |
||||
getPostsCount( defaultScreenName, $miniProfile.find(".posts-count") ); |
||||
getFollowers( defaultScreenName, $miniProfile.find(".followers-count") ); |
||||
|
||||
loadFollowing( function(args) { |
||||
$(".mini-profile .following-count").text(followingUsers.length-1); |
||||
requestLastHave(); |
||||
setInterval("requestLastHave()", 1000); |
||||
initMentionsCount(); |
||||
initDMsCount(); |
||||
requestTimelineUpdate("latestFirstTime",postsPerRefresh,followingUsers,promotedPostsOnly); |
||||
|
||||
// install scrollbottom handler to load more posts as needed
|
||||
$(window).scroll(function(){ |
||||
if ($(window).scrollTop() >= $(document).height() - $(window).height() - 20){ |
||||
if( timelineLoaded ) { |
||||
requestTimelineUpdate("older", postsPerRefresh, followingUsers, promotedPostsOnly); |
||||
} |
||||
} |
||||
}); |
||||
|
||||
twisterFollowingO = TwisterFollowing(defaultScreenName); |
||||
|
||||
setTimeout("getRandomFollowSuggestion(processSuggestion)", 1000); |
||||
setTimeout("getRandomFollowSuggestion(processSuggestion)", 1000); |
||||
setTimeout("getRandomFollowSuggestion(processSuggestion)", 1000); |
||||
|
||||
twisterRpc("gettrendinghashtags", [10], |
||||
function(args, ret) { |
||||
for( var i = 0; i < ret.length; i++ ) { |
||||
|
||||
var $li = $("<li>"); |
||||
var hashtagLinkTemplate = $("#hashtag-link-template").clone(true); |
||||
hashtagLinkTemplate.removeAttr("id"); |
||||
hashtagLinkTemplate.attr("href",$.MAL.hashtagUrl(ret[i])); |
||||
hashtagLinkTemplate.text("#"+ret[i]); |
||||
$li.append(hashtagLinkTemplate); |
||||
$(".toptrends-list").append($li); |
||||
} |
||||
}, {}, |
||||
function(args, ret) { |
||||
console.log("Error with gettrendinghashtags. Older twister daemon?"); |
||||
}, {}); |
||||
|
||||
if( args.cbFunc ) |
||||
args.cbFunc(args.cbArg); |
||||
}, {cbFunc:cbFunc, cbArg:cbArg}); |
||||
|
||||
|
||||
|
||||
|
||||
} |
||||
} |
||||
}; |
||||
|
||||
//***********************************************
|
||||
//******************* INIT **************
|
||||
//***********************************************
|
||||
var interfaceFunctions = new InterfaceFunctions; |
||||
$( document ).ready( interfaceFunctions.init ); |
||||
$( window ).resize(replaceDashboards); |
||||
|
||||
//função no window que fixa o header das postagens
|
||||
function fixDiv() |
||||
{ |
||||
var $cache = $('.postboard h2'); |
||||
if ($(window).scrollTop() > 26) |
||||
$cache.addClass( "fixed" ); |
||||
else |
||||
$cache.removeClass( "fixed" ); |
||||
} |
||||
$(window).scroll(fixDiv); |
||||
|
@ -0,0 +1,12 @@
@@ -0,0 +1,12 @@
|
||||
/* |
||||
Color animation 1.6.0 |
||||
http://www.bitstorm.org/jquery/color-animation/
|
||||
Copyright 2011, 2013 Edwin Martin <edwin@bitstorm.org> |
||||
Released under the MIT and GPL licenses. |
||||
*/ |
||||
'use strict';(function(d){function h(a,b,e){var c="rgb"+(d.support.rgba?"a":"")+"("+parseInt(a[0]+e*(b[0]-a[0]),10)+","+parseInt(a[1]+e*(b[1]-a[1]),10)+","+parseInt(a[2]+e*(b[2]-a[2]),10);d.support.rgba&&(c+=","+(a&&b?parseFloat(a[3]+e*(b[3]-a[3])):1));return c+")"}function f(a){var b;return(b=/#([0-9a-fA-F]{2})([0-9a-fA-F]{2})([0-9a-fA-F]{2})/.exec(a))?[parseInt(b[1],16),parseInt(b[2],16),parseInt(b[3],16),1]:(b=/#([0-9a-fA-F])([0-9a-fA-F])([0-9a-fA-F])/.exec(a))?[17*parseInt(b[1],16),17*parseInt(b[2], |
||||
16),17*parseInt(b[3],16),1]:(b=/rgb\(\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*\)/.exec(a))?[parseInt(b[1]),parseInt(b[2]),parseInt(b[3]),1]:(b=/rgba\(\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9\.]*)\s*\)/.exec(a))?[parseInt(b[1],10),parseInt(b[2],10),parseInt(b[3],10),parseFloat(b[4])]:l[a]}d.extend(!0,d,{support:{rgba:function(){var a=d("script:first"),b=a.css("color"),e=!1;if(/^rgba/.test(b))e=!0;else try{e=b!=a.css("color","rgba(0, 0, 0, 0.5)").css("color"), |
||||
a.css("color",b)}catch(c){}return e}()}});var k="color backgroundColor borderBottomColor borderLeftColor borderRightColor borderTopColor outlineColor".split(" ");d.each(k,function(a,b){d.Tween.propHooks[b]={get:function(a){return d(a.elem).css(b)},set:function(a){var c=a.elem.style,g=f(d(a.elem).css(b)),m=f(a.end);a.run=function(a){c[b]=h(g,m,a)}}}});d.Tween.propHooks.borderColor={set:function(a){var b=a.elem.style,e=[],c=k.slice(2,6);d.each(c,function(b,c){e[c]=f(d(a.elem).css(c))});var g=f(a.end); |
||||
a.run=function(a){d.each(c,function(d,c){b[c]=h(e[c],g,a)})}}};var l={aqua:[0,255,255,1],azure:[240,255,255,1],beige:[245,245,220,1],black:[0,0,0,1],blue:[0,0,255,1],brown:[165,42,42,1],cyan:[0,255,255,1],darkblue:[0,0,139,1],darkcyan:[0,139,139,1],darkgrey:[169,169,169,1],darkgreen:[0,100,0,1],darkkhaki:[189,183,107,1],darkmagenta:[139,0,139,1],darkolivegreen:[85,107,47,1],darkorange:[255,140,0,1],darkorchid:[153,50,204,1],darkred:[139,0,0,1],darksalmon:[233,150,122,1],darkviolet:[148,0,211,1],fuchsia:[255, |
||||
0,255,1],gold:[255,215,0,1],green:[0,128,0,1],indigo:[75,0,130,1],khaki:[240,230,140,1],lightblue:[173,216,230,1],lightcyan:[224,255,255,1],lightgreen:[144,238,144,1],lightgrey:[211,211,211,1],lightpink:[255,182,193,1],lightyellow:[255,255,224,1],lime:[0,255,0,1],magenta:[255,0,255,1],maroon:[128,0,0,1],navy:[0,0,128,1],olive:[128,128,0,1],orange:[255,165,0,1],pink:[255,192,203,1],purple:[128,0,128,1],violet:[128,0,128,1],red:[255,0,0,1],silver:[192,192,192,1],white:[255,255,255,1],yellow:[255,255, |
||||
0,1],transparent:[255,255,255,0]}})(jQuery); |
@ -0,0 +1,554 @@
@@ -0,0 +1,554 @@
|
||||
/*! |
||||
* jQuery.textcomplete.js |
||||
* |
||||
* Repositiory: https://github.com/yuku-t/jquery-textcomplete
|
||||
* License: MIT |
||||
* Author: Yuku Takahashi |
||||
*/ |
||||
|
||||
;(function ($) { |
||||
|
||||
'use strict'; |
||||
|
||||
/** |
||||
* Exclusive execution control utility. |
||||
*/ |
||||
var lock = function (func) { |
||||
var free, locked; |
||||
free = function () { locked = false; }; |
||||
return function () { |
||||
var args; |
||||
if (locked) return; |
||||
locked = true; |
||||
args = toArray(arguments); |
||||
args.unshift(free); |
||||
func.apply(this, args); |
||||
}; |
||||
}; |
||||
|
||||
/** |
||||
* Convert arguments into a real array. |
||||
*/ |
||||
var toArray = function (args) { |
||||
var result; |
||||
result = Array.prototype.slice.call(args); |
||||
return result; |
||||
}; |
||||
|
||||
/** |
||||
* Get the styles of any element from property names. |
||||
*/ |
||||
var getStyles = (function () { |
||||
var color; |
||||
color = $('<div></div>').css(['color']).color; |
||||
if (typeof color !== 'undefined') { |
||||
return function ($el, properties) { |
||||
return $el.css(properties); |
||||
}; |
||||
} else { // for jQuery 1.8 or below
|
||||
return function ($el, properties) { |
||||
var styles; |
||||
styles = {}; |
||||
$.each(properties, function (i, property) { |
||||
styles[property] = $el.css(property); |
||||
}); |
||||
return styles; |
||||
}; |
||||
} |
||||
})(); |
||||
|
||||
/** |
||||
* Default template function. |
||||
*/ |
||||
var identity = function (obj) { return obj; }; |
||||
|
||||
/** |
||||
* Memoize a search function. |
||||
*/ |
||||
var memoize = function (func) { |
||||
var memo = {}; |
||||
return function (term, callback) { |
||||
if (memo[term]) { |
||||
callback(memo[term]); |
||||
} else { |
||||
func.call(this, term, function (data) { |
||||
memo[term] = (memo[term] || []).concat(data); |
||||
callback.apply(null, arguments); |
||||
}); |
||||
} |
||||
}; |
||||
}; |
||||
|
||||
/** |
||||
* Determine if the array contains a given value. |
||||
*/ |
||||
var include = function (array, value) { |
||||
var i, l; |
||||
if (array.indexOf) return array.indexOf(value) != -1; |
||||
for (i = 0, l = array.length; i < l; i++) { |
||||
if (array[i] === value) return true; |
||||
} |
||||
return false; |
||||
}; |
||||
|
||||
/** |
||||
* Textarea manager class. |
||||
*/ |
||||
var Completer = (function () { |
||||
var html, css, $baseWrapper, $baseList, _id; |
||||
|
||||
html = { |
||||
wrapper: '<div class="textcomplete-wrapper"></div>', |
||||
list: '<ul class="dropdown-menu"></ul>' |
||||
}; |
||||
css = { |
||||
wrapper: { |
||||
position: 'relative' |
||||
}, |
||||
list: { |
||||
position: 'absolute', |
||||
top: 0, |
||||
left: 0, |
||||
zIndex: '100', |
||||
display: 'none' |
||||
} |
||||
}; |
||||
$baseWrapper = $(html.wrapper).css(css.wrapper); |
||||
$baseList = $(html.list).css(css.list); |
||||
_id = 0; |
||||
|
||||
function Completer($el) { |
||||
var focus; |
||||
this.el = $el.get(0); // textarea element
|
||||
focus = this.el === document.activeElement; |
||||
// Cannot wrap $el at initialize method lazily due to Firefox's behavior.
|
||||
this.$el = wrapElement($el); // Focus is lost
|
||||
this.id = 'textComplete' + _id++; |
||||
this.strategies = []; |
||||
if (focus) { |
||||
this.initialize(); |
||||
this.$el.focus(); |
||||
} else { |
||||
this.$el.one('focus.textComplete', $.proxy(this.initialize, this)); |
||||
} |
||||
} |
||||
|
||||
/** |
||||
* Completer's public methods |
||||
*/ |
||||
$.extend(Completer.prototype, { |
||||
|
||||
/** |
||||
* Prepare ListView and bind events. |
||||
*/ |
||||
initialize: function () { |
||||
var $list, globalEvents; |
||||
$list = $baseList.clone(); |
||||
this.listView = new ListView($list, this); |
||||
this.$el |
||||
.before($list) |
||||
.on({ |
||||
'keyup.textComplete': $.proxy(this.onKeyup, this), |
||||
'keydown.textComplete': $.proxy(this.listView.onKeydown, |
||||
this.listView) |
||||
}); |
||||
globalEvents = {}; |
||||
globalEvents['click.' + this.id] = $.proxy(this.onClickDocument, this); |
||||
globalEvents['keyup.' + this.id] = $.proxy(this.onKeyupDocument, this); |
||||
$(document).on(globalEvents); |
||||
}, |
||||
|
||||
/** |
||||
* Register strategies to the completer. |
||||
*/ |
||||
register: function (strategies) { |
||||
this.strategies = this.strategies.concat(strategies); |
||||
}, |
||||
|
||||
/** |
||||
* Show autocomplete list next to the caret. |
||||
*/ |
||||
renderList: function (data) { |
||||
if (this.clearAtNext) { |
||||
this.listView.clear(); |
||||
this.clearAtNext = false; |
||||
} |
||||
if (data.length) { |
||||
if (!this.listView.shown) { |
||||
this.listView |
||||
.setPosition(this.getCaretPosition()) |
||||
.clear() |
||||
.activate(); |
||||
this.listView.strategy = this.strategy; |
||||
} |
||||
data = data.slice(0, this.strategy.maxCount); |
||||
this.listView.render(data); |
||||
} |
||||
|
||||
if (!this.listView.data.length && this.listView.shown) { |
||||
this.listView.deactivate(); |
||||
} |
||||
}, |
||||
|
||||
searchCallbackFactory: function (free) { |
||||
var self = this; |
||||
return function (data, keep) { |
||||
self.renderList(data); |
||||
if (!keep) { |
||||
// This is the last callback for this search.
|
||||
free(); |
||||
self.clearAtNext = true; |
||||
} |
||||
}; |
||||
}, |
||||
|
||||
/** |
||||
* Keyup event handler. |
||||
*/ |
||||
onKeyup: function (e) { |
||||
var searchQuery, term; |
||||
if (this.skipSearch(e)) { return; } |
||||
|
||||
searchQuery = this.extractSearchQuery(this.getTextFromHeadToCaret()); |
||||
if (searchQuery.length) { |
||||
term = searchQuery[1]; |
||||
if (this.term === term) return; // Ignore shift-key or something.
|
||||
this.term = term; |
||||
this.search(searchQuery); |
||||
} else { |
||||
this.term = null; |
||||
this.listView.deactivate(); |
||||
} |
||||
}, |
||||
|
||||
/** |
||||
* Suppress searching if it returns true. |
||||
*/ |
||||
skipSearch: function (e) { |
||||
if (this.skipNextKeyup) { |
||||
this.skipNextKeyup = false; |
||||
return true; |
||||
} |
||||
switch (e.keyCode) { |
||||
case 40: |
||||
case 38: |
||||
return true; |
||||
} |
||||
}, |
||||
|
||||
onSelect: function (value) { |
||||
var pre, post, newSubStr; |
||||
pre = this.getTextFromHeadToCaret(); |
||||
post = this.el.value.substring(this.el.selectionEnd); |
||||
|
||||
newSubStr = this.strategy.replace(value); |
||||
if ($.isArray(newSubStr)) { |
||||
post = newSubStr[1] + post; |
||||
newSubStr = newSubStr[0]; |
||||
} |
||||
pre = pre.replace(this.strategy.match, newSubStr); |
||||
this.$el.val(pre + post) |
||||
.trigger('change') |
||||
.trigger('textComplete:select', value); |
||||
this.el.focus(); |
||||
this.el.selectionStart = this.el.selectionEnd = pre.length; |
||||
this.skipNextKeyup = true; |
||||
}, |
||||
|
||||
/** |
||||
* Global click event handler. |
||||
*/ |
||||
onClickDocument: function (e) { |
||||
if (e.originalEvent && !e.originalEvent.keepTextCompleteDropdown) { |
||||
this.listView.deactivate(); |
||||
} |
||||
}, |
||||
|
||||
/** |
||||
* Global keyup event handler. |
||||
*/ |
||||
onKeyupDocument: function (e) { |
||||
if (this.listView.shown && e.keyCode === 27) { // ESC
|
||||
this.listView.deactivate(); |
||||
this.$el.focus(); |
||||
} |
||||
}, |
||||
|
||||
/** |
||||
* Remove all event handlers and the wrapper element. |
||||
*/ |
||||
destroy: function () { |
||||
var $wrapper; |
||||
this.$el.off('.textComplete'); |
||||
$(document).off('.' + this.id); |
||||
if (this.listView) { this.listView.destroy(); } |
||||
$wrapper = this.$el.parent(); |
||||
$wrapper.after(this.$el).remove(); |
||||
this.$el.data('textComplete', void 0); |
||||
this.$el = null; |
||||
}, |
||||
|
||||
// Helper methods
|
||||
// ==============
|
||||
|
||||
/** |
||||
* Returns caret's relative coordinates from textarea's left top corner. |
||||
*/ |
||||
getCaretPosition: function () { |
||||
// Browser native API does not provide the way to know the position of
|
||||
// caret in pixels, so that here we use a kind of hack to accomplish
|
||||
// the aim. First of all it puts a div element and completely copies
|
||||
// the textarea's style to the element, then it inserts the text and a
|
||||
// span element into the textarea.
|
||||
// Consequently, the span element's position is the thing what we want.
|
||||
|
||||
if (this.el.selectionEnd === 0) return; |
||||
var properties, css, $div, $span, position, dir; |
||||
|
||||
dir = this.$el.attr('dir') || this.$el.css('direction'); |
||||
properties = ['border-width', 'font-family', 'font-size', 'font-style', |
||||
'font-variant', 'font-weight', 'height', 'letter-spacing', |
||||
'word-spacing', 'line-height', 'text-decoration', 'text-align', |
||||
'width', 'padding-top', 'padding-right', 'padding-bottom', |
||||
'padding-left', 'margin-top', 'margin-right', 'margin-bottom', |
||||
'margin-left' |
||||
]; |
||||
css = $.extend({ |
||||
position: 'absolute', |
||||
overflow: 'auto', |
||||
'white-space': 'pre-wrap', |
||||
top: 0, |
||||
left: -9999, |
||||
direction: dir |
||||
}, getStyles(this.$el, properties)); |
||||
|
||||
$div = $('<div></div>').css(css).text(this.getTextFromHeadToCaret()); |
||||
$span = $('<span></span>').text('.').appendTo($div); |
||||
this.$el.before($div); |
||||
position = $span.position(); |
||||
position.top += $span.height() - this.$el.scrollTop(); |
||||
if (dir === 'rtl') { position.left -= this.listView.$el.width(); } |
||||
$div.remove(); |
||||
return position; |
||||
}, |
||||
|
||||
getTextFromHeadToCaret: function () { |
||||
var text, selectionEnd, range; |
||||
selectionEnd = this.el.selectionEnd; |
||||
if (typeof selectionEnd === 'number') { |
||||
text = this.el.value.substring(0, selectionEnd); |
||||
} else if (document.selection) { |
||||
range = this.el.createTextRange(); |
||||
range.moveStart('character', 0); |
||||
range.moveEnd('textedit'); |
||||
text = range.text; |
||||
} |
||||
return text; |
||||
}, |
||||
|
||||
/** |
||||
* Parse the value of textarea and extract search query. |
||||
*/ |
||||
extractSearchQuery: function (text) { |
||||
// If a search query found, it returns used strategy and the query
|
||||
// term. If the caret is currently in a code block or search query does
|
||||
// not found, it returns an empty array.
|
||||
|
||||
var i, l, strategy, match; |
||||
for (i = 0, l = this.strategies.length; i < l; i++) { |
||||
strategy = this.strategies[i]; |
||||
match = text.match(strategy.match); |
||||
if (match) { return [strategy, match[strategy.index]]; } |
||||
} |
||||
return []; |
||||
}, |
||||
|
||||
search: lock(function (free, searchQuery) { |
||||
var term; |
||||
this.strategy = searchQuery[0]; |
||||
term = searchQuery[1]; |
||||
this.strategy.search(term, this.searchCallbackFactory(free)); |
||||
}) |
||||
}); |
||||
|
||||
/** |
||||
* Completer's private functions |
||||
*/ |
||||
var wrapElement = function ($el) { |
||||
return $el.wrap($baseWrapper.clone().css('display', $el.css('display'))); |
||||
}; |
||||
|
||||
return Completer; |
||||
})(); |
||||
|
||||
/** |
||||
* Dropdown menu manager class. |
||||
*/ |
||||
var ListView = (function () { |
||||
|
||||
function ListView($el, completer) { |
||||
this.data = []; |
||||
this.$el = $el; |
||||
this.index = 0; |
||||
this.completer = completer; |
||||
|
||||
this.$el.on('click.textComplete', 'li.textcomplete-item', |
||||
$.proxy(this.onClick, this)); |
||||
} |
||||
|
||||
$.extend(ListView.prototype, { |
||||
shown: false, |
||||
|
||||
render: function (data) { |
||||
var html, i, l, index, val; |
||||
|
||||
html = ''; |
||||
for (i = 0, l = data.length; i < l; i++) { |
||||
val = data[i]; |
||||
if (include(this.data, val)) continue; |
||||
index = this.data.length; |
||||
this.data.push(val); |
||||
html += '<li class="textcomplete-item" data-index="' + index + '"><a>'; |
||||
html += this.strategy.template(val); |
||||
html += '</a></li>'; |
||||
if (this.data.length === this.strategy.maxCount) break; |
||||
} |
||||
this.$el.append(html); |
||||
if (!this.data.length) { |
||||
this.deactivate(); |
||||
} else { |
||||
this.activateIndexedItem(); |
||||
} |
||||
}, |
||||
|
||||
clear: function () { |
||||
this.data = []; |
||||
this.$el.html(''); |
||||
this.index = 0; |
||||
return this; |
||||
}, |
||||
|
||||
activateIndexedItem: function () { |
||||
this.$el.find('.active').removeClass('active'); |
||||
this.getActiveItem().addClass('active'); |
||||
}, |
||||
|
||||
getActiveItem: function () { |
||||
return $(this.$el.children().get(this.index)); |
||||
}, |
||||
|
||||
activate: function () { |
||||
if (!this.shown) { |
||||
this.$el.show(); |
||||
this.completer.$el.trigger('textComplete:show'); |
||||
this.shown = true; |
||||
} |
||||
return this; |
||||
}, |
||||
|
||||
deactivate: function () { |
||||
if (this.shown) { |
||||
this.$el.hide(); |
||||
this.completer.$el.trigger('textComplete:hide'); |
||||
this.shown = false; |
||||
this.data = this.index = null; |
||||
} |
||||
return this; |
||||
}, |
||||
|
||||
setPosition: function (position) { |
||||
this.$el.css(position); |
||||
return this; |
||||
}, |
||||
|
||||
select: function (index) { |
||||
var self = this; |
||||
this.completer.onSelect(this.data[index]); |
||||
// Deactive at next tick to allow other event handlers to know whether
|
||||
// the dropdown has been shown or not.
|
||||
setTimeout(function () { self.deactivate(); }, 0); |
||||
}, |
||||
|
||||
onKeydown: function (e) { |
||||
if (!this.shown) return; |
||||
if (e.keyCode === 38) { // UP
|
||||
e.preventDefault(); |
||||
if (this.index === 0) { |
||||
this.index = this.data.length-1; |
||||
} else { |
||||
this.index -= 1; |
||||
} |
||||
this.activateIndexedItem(); |
||||
} else if (e.keyCode === 40) { // DOWN
|
||||
e.preventDefault(); |
||||
if (this.index === this.data.length - 1) { |
||||
this.index = 0; |
||||
} else { |
||||
this.index += 1; |
||||
} |
||||
this.activateIndexedItem(); |
||||
} else if (e.keyCode === 13 || e.keyCode === 9) { // ENTER or TAB
|
||||
e.preventDefault(); |
||||
|
||||
this.select(parseInt(this.getActiveItem().data('index'), 10)); |
||||
} |
||||
}, |
||||
|
||||
onClick: function (e) { |
||||
var $e = $(e.target); |
||||
e.originalEvent.keepTextCompleteDropdown = true; |
||||
if (!$e.hasClass('textcomplete-item')) { |
||||
$e = $e.parents('li.textcomplete-item'); |
||||
} |
||||
this.select(parseInt($e.data('index'), 10)); |
||||
}, |
||||
|
||||
destroy: function () { |
||||
this.deactivate(); |
||||
this.$el.off('click.textComplete').remove(); |
||||
this.$el = null; |
||||
} |
||||
}); |
||||
|
||||
return ListView; |
||||
})(); |
||||
|
||||
$.fn.textcomplete = function (strategies) { |
||||
var i, l, strategy, dataKey; |
||||
|
||||
dataKey = 'textComplete'; |
||||
|
||||
if (strategies === 'destroy') { |
||||
return this.each(function () { |
||||
var completer = $(this).data(dataKey); |
||||
if (completer) { completer.destroy(); } |
||||
}); |
||||
} |
||||
|
||||
for (i = 0, l = strategies.length; i < l; i++) { |
||||
strategy = strategies[i]; |
||||
if (!strategy.template) { |
||||
strategy.template = identity; |
||||
} |
||||
if (strategy.index == null) { |
||||
strategy.index = 2; |
||||
} |
||||
if (strategy.cache) { |
||||
strategy.search = memoize(strategy.search); |
||||
} |
||||
strategy.maxCount || (strategy.maxCount = 10); |
||||
} |
||||
|
||||
return this.each(function () { |
||||
var $this, completer; |
||||
$this = $(this); |
||||
completer = $this.data(dataKey); |
||||
if (!completer) { |
||||
completer = new Completer($this); |
||||
$this.data(dataKey, completer); |
||||
} |
||||
completer.register(strategies); |
||||
}); |
||||
}; |
||||
|
||||
})(window.jQuery || window.Zepto); |
@ -0,0 +1,361 @@
@@ -0,0 +1,361 @@
|
||||
$(function() { |
||||
|
||||
}); |
||||
|
||||
var TwisterOptions = function() |
||||
{ |
||||
this.getOption = function(optionName, defaultValue) { |
||||
var keyName = "options:" + optionName; |
||||
if( $.localStorage.isSet(keyName) ) |
||||
return $.localStorage.get(keyName); |
||||
else |
||||
return defaultValue; |
||||
} |
||||
|
||||
this.setOption = function(optionName, value) { |
||||
var keyName = "options:" + optionName; |
||||
$.localStorage.set(keyName, value); |
||||
} |
||||
|
||||
this.soundNotifOptions = function() { |
||||
$('#notifyForm select').each(function(){ |
||||
this.value = $.Options.getOption(this.id, "false"); |
||||
}); |
||||
|
||||
var player = $('#player'); |
||||
player[0].pause(); |
||||
$('#player').empty(); |
||||
|
||||
$('form#notifyForm').on('change','select',function(){ |
||||
$.Options.setOption(this.id, this.value); |
||||
|
||||
if(this.value == false) {player[0].pause(); return;} |
||||
if (player[0].canPlayType('audio/mpeg;')) { |
||||
player.attr('type', 'audio/mpeg'); |
||||
player.attr('src', 'sound/'+this.value+'.mp3'); |
||||
} else { |
||||
player.attr('type', 'audio/ogg'); |
||||
player.attr('src', 'sound/'+this.value+'.ogg'); |
||||
} |
||||
|
||||
player[0].play(); |
||||
}); |
||||
} |
||||
|
||||
this.volumeControl = function() { |
||||
var playerVol = $('#playerVol'); |
||||
playerVol[0].value = $.Options.getOption(playerVol[0].id, 100); |
||||
$('.volValue').text((playerVol[0].value * 100).toFixed()); |
||||
|
||||
playerVol.on('change',function(){ |
||||
$.Options.setOption(this.id, this.value); |
||||
$('#player')[0].volume = (this.value); |
||||
$('.volValue').text((this.value * 100).toFixed()); |
||||
}); |
||||
} |
||||
|
||||
this.DMsNotif = function() { |
||||
var sndDM = $.Options.getOption('sndDM', "false"); |
||||
if( sndDM == "false") return; |
||||
var player = $('#player'); |
||||
$('#player').empty(); |
||||
|
||||
if (player[0].canPlayType('audio/mpeg;')) { |
||||
player.attr('type', 'audio/mpeg'); |
||||
player.attr('src', 'sound/'+sndDM+'.mp3'); |
||||
} else { |
||||
player.attr('type', 'audio/ogg'); |
||||
player.attr('src', 'sound/'+sndDM+'.ogg'); |
||||
} |
||||
player[0].volume = $.Options.getOption('playerVol',100); |
||||
player[0].play(); |
||||
} |
||||
|
||||
this.mensNotif = function() { |
||||
var sndMention = $.Options.getOption('sndMention', "false"); |
||||
if(sndMention == "false") return; |
||||
var player = $('#player'); |
||||
$('#playerSec').empty(); |
||||
|
||||
if (player[0].canPlayType('audio/mpeg;')) { |
||||
player.attr('type', 'audio/mpeg'); |
||||
player.attr('src', 'sound/'+sndMention+'.mp3'); |
||||
} else { |
||||
player.attr('type', 'audio/ogg'); |
||||
player.attr('src', 'sound/'+sndMention+'.ogg'); |
||||
} |
||||
player[0].volume = $.Options.getOption('playerVol',100); |
||||
player[0].play(); |
||||
} |
||||
|
||||
this.keysSendDefault = "ctrlenter"; |
||||
this.keysSend = function() { |
||||
$('#keysOpt select')[0].value = $.Options.getOption('keysSend',this.keysSendDefault); |
||||
|
||||
$('#keysOpt select').on('change', function(){ |
||||
$.Options.setOption(this.id, this.value); |
||||
}) |
||||
} |
||||
|
||||
this.keyEnterToSend = function() { |
||||
return $.Options.getOption('keysSend',this.keysSendDefault) == "enter"; |
||||
} |
||||
|
||||
this.setLang = function() { |
||||
$('#language').val($.Options.getOption('locLang','auto')) |
||||
$('#language').on('change', function(){ |
||||
$.Options.setOption('locLang', $(this).val()); |
||||
location.reload(); |
||||
}) |
||||
} |
||||
|
||||
this.getTheme = function() { |
||||
return $.Options.getOption('theme','original'); |
||||
} |
||||
|
||||
this.setTheme = function() { |
||||
$('#theme').val(this.getTheme()) |
||||
$('#theme').on('change', function(){ |
||||
$.Options.setOption('theme', $(this).val()); |
||||
location.reload(); |
||||
}); |
||||
} |
||||
|
||||
this.getLineFeedsOpt = function() { |
||||
return $.Options.getOption('displayLineFeeds',"disable"); |
||||
} |
||||
|
||||
this.setLineFeedsOpt = function() { |
||||
$('#lineFeedsOpt select')[0].value = this.getLineFeedsOpt(); |
||||
|
||||
$('#lineFeedsOpt select').on('change', function(){ |
||||
$.Options.setOption(this.id, this.value); |
||||
}) |
||||
} |
||||
|
||||
this.getShowPreviewOpt = function() { |
||||
return $.Options.getOption('displayPreview',"disable"); |
||||
} |
||||
|
||||
this.setShowPreviewOpt = function () { |
||||
$('#showPreviewOpt select')[0].value = this.getShowPreviewOpt(); |
||||
|
||||
$('#showPreviewOpt select').on('change', function(){ |
||||
$.Options.setOption(this.id, this.value); |
||||
}); |
||||
} |
||||
|
||||
this.getUnicodeConversionOpt = function () { |
||||
return $.Options.getOption('unicodeConversion', "disable"); |
||||
} |
||||
|
||||
this.setUnicodeConversionOpt = function () { |
||||
$("#unicodeConversion")[0].value = this.getUnicodeConversionOpt(); |
||||
|
||||
if (this.getUnicodeConversionOpt() === "custom") |
||||
$("#unicodeConversionOpt .suboptions")[0].style.height = "230px"; |
||||
|
||||
$("#unicodeConversion").on('change', function () { |
||||
$.Options.setOption(this.id, this.value); |
||||
if (this.value === "custom") |
||||
$("#unicodeConversionOpt .suboptions")[0].style.height = "230px"; |
||||
else |
||||
$("#unicodeConversionOpt .suboptions")[0].style.height = "0px"; |
||||
}); |
||||
} |
||||
|
||||
this.getConvertPunctuationsOpt = function() { |
||||
return $.Options.getOption('convertPunctuationsOpt', false); |
||||
} |
||||
|
||||
this.setConvertPunctuationsOpt = function () { |
||||
$('#convertPunctuationsOpt')[0].checked = this.getConvertPunctuationsOpt(); |
||||
|
||||
$('#convertPunctuationsOpt').on('change', function(){ |
||||
$.Options.setOption(this.id, this.checked); |
||||
}); |
||||
} |
||||
|
||||
this.getConvertEmotionsOpt = function() { |
||||
return $.Options.getOption('convertEmotionsOpt', false); |
||||
} |
||||
|
||||
this.setConvertEmotionsOpt = function () { |
||||
$('#convertEmotionsOpt')[0].checked = this.getConvertEmotionsOpt(); |
||||
|
||||
$('#convertEmotionsOpt').on('change', function(){ |
||||
$.Options.setOption(this.id, this.checked); |
||||
}); |
||||
} |
||||
|
||||
this.getConvertSignsOpt = function() { |
||||
return $.Options.getOption('convertSignsOpt', false); |
||||
} |
||||
|
||||
this.setConvertSignsOpt = function () { |
||||
$('#convertSignsOpt')[0].checked = this.getConvertSignsOpt(); |
||||
|
||||
$('#convertSignsOpt').on('change', function(){ |
||||
$.Options.setOption(this.id, this.checked); |
||||
}); |
||||
} |
||||
|
||||
this.getConvertFractionsOpt = function() { |
||||
return $.Options.getOption('convertFractionsOpt', false); |
||||
} |
||||
|
||||
this.setConvertFractionsOpt = function () { |
||||
$('#convertFractionsOpt')[0].checked = this.getConvertFractionsOpt(); |
||||
|
||||
$('#convertFractionsOpt').on('change', function(){ |
||||
$.Options.setOption(this.id, this.checked); |
||||
}); |
||||
} |
||||
|
||||
this.getUseProxyOpt = function () { |
||||
return $.Options.getOption('useProxy', 'disable'); |
||||
} |
||||
|
||||
this.setUseProxyOpt = function () { |
||||
$('#useProxy')[0].value = this.getUseProxyOpt(); |
||||
|
||||
if (this.getUseProxyOpt() === 'disable') |
||||
$('#useProxyForImgOnly').attr('disabled','disabled'); |
||||
|
||||
$('#useProxy').on('change', function () { |
||||
$.Options.setOption(this.id, this.value); |
||||
|
||||
if (this.value === 'disable') |
||||
$('#useProxyForImgOnly').attr('disabled','disabled'); |
||||
else |
||||
$('#useProxyForImgOnly').removeAttr('disabled'); |
||||
}); |
||||
} |
||||
|
||||
this.getUseProxyForImgOnlyOpt = function () { |
||||
return $.Options.getOption('useProxyForImgOnly', false); |
||||
} |
||||
|
||||
this.setUseProxyForImgOnlyOpt = function () { |
||||
$('#useProxyForImgOnly')[0].checked = this.getUseProxyForImgOnlyOpt(); |
||||
|
||||
$('#useProxyForImgOnly').on('change', function () { |
||||
$.Options.setOption(this.id, this.checked); |
||||
}); |
||||
} |
||||
|
||||
this.getSplitPostsOpt = function (){ |
||||
return $.Options.getOption('splitPosts', 'disable'); |
||||
} |
||||
|
||||
this.setSplitPostsOpt = function () { |
||||
$('#splitPosts')[0].value = this.getSplitPostsOpt(); |
||||
|
||||
$('#splitPosts').on('change', function () { |
||||
$.Options.setOption(this.id, this.value); |
||||
}); |
||||
} |
||||
|
||||
this.getHideRepliesOpt = function () { |
||||
return $.Options.getOption('hideReplies', 'following'); |
||||
} |
||||
|
||||
this.setHideRepliesOpt = function () { |
||||
$('#hideReplies')[0].value = this.getHideRepliesOpt(); |
||||
|
||||
$('#hideReplies').on('change', function () { |
||||
$.Options.setOption(this.id, this.value); |
||||
}); |
||||
} |
||||
|
||||
this.getHideCloseRTsOpt = function () { |
||||
return $.Options.getOption('hideCloseRTs', 'disable'); |
||||
}; |
||||
|
||||
this.setHideCloseRTsOpt = function () { |
||||
$('#hideCloseRTs')[0].value = this.getHideCloseRTsOpt(); |
||||
|
||||
if (this.getHideCloseRTsOpt() === 'disable') { |
||||
$('#hideCloseRTsDesc')[0].style.display = 'none'; |
||||
} else { |
||||
$('#hideCloseRTsDesc')[0].style.display = 'inline'; |
||||
} |
||||
|
||||
$('#hideCloseRTs').on('change', function () { |
||||
$.Options.setOption(this.id, this.value); |
||||
|
||||
if (this.value === 'disable') { |
||||
$('#hideCloseRTsDesc')[0].style.display = 'none'; |
||||
} else { |
||||
$('#hideCloseRTsDesc')[0].style.display = 'inline'; |
||||
} |
||||
}); |
||||
}; |
||||
|
||||
this.getHideCloseRTsHourOpt = function () { |
||||
return parseInt($.Options.getOption('hideCloseRtsHour', '1')); |
||||
}; |
||||
|
||||
this.setHideCloseRTsHourOpt = function () { |
||||
$('#hideCloseRtsHour')[0].value = this.getHideCloseRTsHourOpt().toString(); |
||||
|
||||
$('#hideCloseRtsHour').on('keyup', function () { |
||||
if (/^\d+$/.test(this.value)) { |
||||
this.style.backgroundColor = ''; |
||||
$.Options.setOption(this.id, this.value); |
||||
$(this).next('span').text(polyglot.t('hour(s)')); |
||||
} else { |
||||
this.style.backgroundColor = '#f00'; |
||||
$(this).next('span').text(polyglot.t('only numbers are allowed!')); |
||||
} |
||||
}); |
||||
}; |
||||
|
||||
this.getIsFollowingMeOpt = function () { |
||||
return $.Options.getOption('isFollowingMe'); |
||||
}; |
||||
|
||||
this.setIsFollowingMeOpt = function () { |
||||
$('#isFollowingMe')[0].value = this.getIsFollowingMeOpt(); |
||||
|
||||
$('#isFollowingMe').on('change', function () { |
||||
$.Options.setOption(this.id, this.value); |
||||
}); |
||||
}; |
||||
|
||||
this.InitOptions = function() { |
||||
this.soundNotifOptions(); |
||||
this.volumeControl(); |
||||
this.keysSend(); |
||||
this.setLang(); |
||||
this.setTheme(); |
||||
this.setLineFeedsOpt(); |
||||
this.setShowPreviewOpt(); |
||||
this.setUnicodeConversionOpt(); |
||||
this.setConvertPunctuationsOpt(); |
||||
this.setConvertEmotionsOpt(); |
||||
this.setConvertSignsOpt(); |
||||
this.setConvertFractionsOpt(); |
||||
this.setUseProxyOpt(); |
||||
this.setUseProxyForImgOnlyOpt(); |
||||
this.setSplitPostsOpt(); |
||||
this.setHideRepliesOpt(); |
||||
this.setHideCloseRTsHourOpt(); |
||||
this.setHideCloseRTsOpt(); |
||||
this.setIsFollowingMeOpt(); |
||||
} |
||||
} |
||||
|
||||
jQuery.Options = new TwisterOptions; |
||||
|
||||
function localizeLabels() |
||||
{ |
||||
$("label[for=tab_language]").text(polyglot.t("Language")); |
||||
$("label[for=t-2]").text(polyglot.t("Theme")); |
||||
$("label[for=t-3]").text(polyglot.t("Sound")); |
||||
$("label[for=t-4]").text(polyglot.t("Keys")); |
||||
$("label[for=t-5]").text(polyglot.t("Postboard")); |
||||
$("label[for=t-6]").text(polyglot.t("Users")); |
||||
} |
||||
|
||||
$(document).ready(localizeLabels); |
@ -0,0 +1,286 @@
@@ -0,0 +1,286 @@
|
||||
<!DOCTYPE html> |
||||
<html> |
||||
<head> |
||||
<meta charset="utf-8"/> |
||||
<title>Options</title> |
||||
<link id="stylecss" rel="stylesheet" href="css/style.css" type="text/css"/> |
||||
<script src="js/jquery.min.js"></script> |
||||
<script src="js/jQueryPlugins.js"></script> |
||||
<script src="js/jquery.json-2.4.js"></script> |
||||
<script src="js/jquery.jsonrpcclient.js"></script> |
||||
<script src="js/jquery.storageapi.js"></script> |
||||
<script src="js/options.js"></script> |
||||
<script src="js/mobile_abstract.js"></script> |
||||
<script src="js/twister_io.js"></script> |
||||
<script src="js/twister_network.js"></script> |
||||
<script src="js/twister_user.js"></script> |
||||
<script src="js/interface_common.js"></script> |
||||
<script src="js/interface_login.js"></script> |
||||
<script src="js/polyglot.min.js"></script> |
||||
<script src="js/interface_localization.js"></script> |
||||
<script>$(function(){ |
||||
initInterfaceCommon(); |
||||
$.Options.InitOptions();}) |
||||
changeStyle(); |
||||
</script> |
||||
</head> |
||||
|
||||
<body> |
||||
|
||||
<!-- MENU SUPERIOR INIT --> |
||||
<nav class="userMenu"> |
||||
<ul> |
||||
<li class="userMenu-home"><a href="home.html"><span class="selectable_theme theme_original label">Home</span></a></li> |
||||
<li class="userMenu-options current"><a class="label" href="options.html">Options</a></li> |
||||
<li class="userMenu-config"> |
||||
<a class="userMenu-config-dropdown" href="#"> |
||||
<div class="config-menu dialog-modal"> |
||||
<a class="dropdown-menu-item" href="options.html">Options</a> |
||||
<a class="dropdown-menu-item" href="network.html">Network config</a> |
||||
<a class="dropdown-menu-item" href="profile-edit.html">Setup account</a> |
||||
<a class="dropdown-menu-item" href="following.html">Following users</a> |
||||
<a class="dropdown-menu-item" href="login.html">Change user</a> |
||||
</div> |
||||
</a> |
||||
</li> |
||||
</ul> |
||||
</nav> |
||||
|
||||
<div class="wrapper"> |
||||
<div class="options"> |
||||
|
||||
<input id="tab_language" name="option_tab" type="radio" checked="checked" class="selectable_theme theme_nin"/> |
||||
<label for="tab_language" class="tabs selectable_theme theme_nin">Language</label> |
||||
<input id="t-2" name="option_tab" type="radio" class="selectable_theme theme_nin"/> |
||||
<label for="t-2" class="tabs selectable_theme theme_nin">Theme</label> |
||||
<input id="t-3" name="option_tab" type="radio" class="selectable_theme theme_nin"/> |
||||
<label for="t-3" class="tabs selectable_theme theme_nin">Sound</label> |
||||
<input id="t-4" name="option_tab" type="radio" class="selectable_theme theme_nin"/> |
||||
<label for="t-4" class="tabs selectable_theme theme_nin">Keys</label> |
||||
<input id="t-5" name="option_tab" type="radio" class="selectable_theme theme_nin"/> |
||||
<label for="t-5" class="tabs selectable_theme theme_nin">Postboard</label> |
||||
<input id="t-6" name="option_tab" type="radio" class="selectable_theme theme_nin" /> |
||||
<label for="t-6" class="tabs selectable_theme theme_nin">Users</label> |
||||
|
||||
<div class="tab-content"> |
||||
|
||||
<div class="language"> |
||||
|
||||
<div class="module"> |
||||
<p class="label"> Use language </p> |
||||
<div> |
||||
<form action="" id="selectLanguage"> |
||||
<select name="" id="language"> |
||||
<option value="auto">Auto</option> |
||||
<option value="pt-BR">Brazilian Portuguese</option> |
||||
<option value="zh">Chinese</option> |
||||
<option value="cs">Czech</option> |
||||
<option value="nl">Dutch</option> |
||||
<option value="en">English</option> |
||||
<option value="fr">French</option> |
||||
<option value="de">German</option> |
||||
<option value="it">Italian</option> |
||||
<option value="ja">Japanese</option> |
||||
<option value="ru">Russian</option> |
||||
<option value="es">Spanish</option> |
||||
<option value="tr">Turkish</option> |
||||
</select> |
||||
</form> |
||||
</div> |
||||
</div> |
||||
</div> |
||||
|
||||
<div class="theme"> |
||||
<div class="module"> |
||||
<p class="label"> Theme </p> |
||||
<div> |
||||
<form action="" id="selectTheme"> |
||||
<select name="" id="theme"> |
||||
<option value="original">Original</option> |
||||
<option value="calm">Calm</option> |
||||
<option value="nin">Nin</option> |
||||
</select> |
||||
</form> |
||||
<span class="selectable_theme theme_calm"> |
||||
For new features check <a href="https://github.com/iHedgehog/twister-calm">twister-calm repository</a>! |
||||
</span> |
||||
</div> |
||||
</div> |
||||
</div> |
||||
|
||||
<div class="sounds"> |
||||
|
||||
<div class="module"> |
||||
<p class="label"> Sound notifications </p> |
||||
<div> |
||||
<form action="" id="notifyForm"> |
||||
<div> |
||||
<p class="label">Mentions</p> |
||||
<select name="" id="sndMention" class="sndOpt"> |
||||
<option value="false">none</option> |
||||
<option value="1">beat</option> |
||||
<option value="2">pip</option> |
||||
<option value="3">vibro</option> |
||||
<option value="4">flip</option> |
||||
<option value="5">click</option> |
||||
</select> |
||||
</div> |
||||
<div> |
||||
<p class="label">Direct Messages</p> |
||||
<select name="" id="sndDM" class="sndOpt"> |
||||
<option value="false">none</option> |
||||
<option value="1">beat</option> |
||||
<option value="2">pip</option> |
||||
<option value="3">vibro</option> |
||||
<option value="4">flip</option> |
||||
<option value="5">click</option> |
||||
</select> |
||||
</div> |
||||
<input type="range" name="playerVol" id="playerVol" min="0" max="1" step="0.01"><span class="volValue">0</span> |
||||
</form> |
||||
<audio id="player"></audio> |
||||
</div> |
||||
</div> |
||||
</div> |
||||
|
||||
<div class="keys"> |
||||
<div class="module"> |
||||
<p class="label"> Keys </p> |
||||
<div> |
||||
<form action="" id="keysOpt"> |
||||
<p class="label">Send key</p> |
||||
<select name="" id="keysSend"> |
||||
<option value="enter">Enter</option> |
||||
<option value="ctrlenter">Ctrl/Cmd+Enter</option> |
||||
</select> |
||||
</form> |
||||
</div> |
||||
</div> |
||||
</div> |
||||
|
||||
<div class="postboard-display"> |
||||
<div class="module"> |
||||
<p class="label"> Postboard displays </p> |
||||
<div> |
||||
<form action="" id="hideRepliesOpt"> |
||||
<p class="label">Posts that begin with mention</p> |
||||
<select name="" id="hideReplies"> |
||||
<option value="disable">Show all</option> |
||||
<option value="only-me">Show only if I am in</option> |
||||
<option value="following">Show if it's between users I follow</option> |
||||
</select> |
||||
</form> |
||||
</div> |
||||
<div> |
||||
<form action="" id="hideCloseRTsOpt"> |
||||
<p class="label">RTs those are close to original twist</p> |
||||
<select name="" id="hideCloseRTs"> |
||||
<option value="disable">Show all</option> |
||||
<option value="show-if">Show if the original is older than</option> |
||||
</select> |
||||
<div id="hideCloseRTsDesc"> |
||||
<input name="" id="hideCloseRtsHour" maxlength="2" size="3"/> <span class="label">hour(s)</span> |
||||
</div> |
||||
</form> |
||||
</div> |
||||
</div> |
||||
<div class="module"> |
||||
<p class="label"> Posts display </p> |
||||
<div> |
||||
<form action="" id="lineFeedsOpt"> |
||||
<p class="label">Line feeds</p> |
||||
<select name="" id="displayLineFeeds"> |
||||
<option value="disable">Ignore</option> |
||||
<option value="enable">Display</option> |
||||
</select> |
||||
</form> |
||||
</div> |
||||
<div> |
||||
<form action="" id="showPreviewOpt"> |
||||
<p class="label">Inline image preview</p> |
||||
<select name="" id="displayPreview"> |
||||
<option value="disable">Ignore</option> |
||||
<option value="enable">Display</option> |
||||
</select> |
||||
</form> |
||||
</div> |
||||
<div> |
||||
<form action="" id="useProxyOpt"> |
||||
<p class="label">Use external links behind a proxy</p> |
||||
<select name="" id="useProxy"> |
||||
<option value="disable">none</option> |
||||
<option value="ssl-proxy-my-addr">ssl-proxy.my-addr.org</option> |
||||
<option value="anonymouse">anonymouse.org</option> |
||||
</select> |
||||
<input name="" id="useProxyForImgOnly" type="checkbox" /> <span class="label">Use proxy for image preview only</span> |
||||
</form> |
||||
</div> |
||||
</div> |
||||
<div class="post-editor"> |
||||
<div class="module"> |
||||
<p class="label"> Post editor</p> |
||||
<div> |
||||
<form action="" id="unicodeConversionOpt"> |
||||
<p class="label">Automatic unicode conversion options</p> |
||||
<select name="" id="unicodeConversion"> |
||||
<option value="disable">Ignore</option> |
||||
<option value="enable">Convert all</option> |
||||
<option value="custom">Custom</option> |
||||
</select> |
||||
<div class="suboptions unicode-suboptions"> |
||||
<input name="" id="convertPunctuationsOpt" type="checkbox" /> <span class="label">Convert punctuations to unicode</span><br/> |
||||
<div> |
||||
<label class="label">Supported punctuations: </label> |
||||
<span>‥ … ⁇ ⁈ ⁉ ‼ — ⁓</span> |
||||
</div> |
||||
<input name="" id="convertEmotionsOpt" type="checkbox" /> <span class="label">Convert emotions codes to unicode symbols</span> |
||||
<div> |
||||
<label class="label">Supported emotions: </label> |
||||
<span>😃 😇 🍺 😈 ❤ 😕 😢 😞 😎 😊 😊 😗 😆 😛 😉 😉 😮 😱 😐</span> |
||||
</div> |
||||
<input name="" id="convertSignsOpt" type="checkbox" /> <span class="label">Convert common signs to unicode</span><br/> |
||||
<div> |
||||
<label class="label">Supported signs:</label> |
||||
<span>℡ ℻</span> |
||||
</div> |
||||
<input name="" id="convertFractionsOpt" type="checkbox" /> <span class="label">Convert fractions to unicode</span><br/> |
||||
<div> |
||||
<label class="label">Supported fractions:</label> |
||||
<span>½ ⅓ ⅔ ¼ ¾ ⅕ ⅖ ⅗ ⅘ ⅙ ⅚ ⅐ ⅛ ⅜ ⅝ ⅞ ⅑ ⅒</span> |
||||
</div> |
||||
</div> |
||||
</form> |
||||
</div> |
||||
|
||||
<div> |
||||
<form action="" id="splitPostsOpt"> |
||||
<p class="label">Split long posts</p> |
||||
<select name="" id="splitPosts"> |
||||
<option value="disable">Don't split</option> |
||||
<option value="enable">Split all</option> |
||||
<option value="only-new">Split only new post</option> |
||||
</select> |
||||
</form> |
||||
</div> |
||||
|
||||
</div> |
||||
</div> |
||||
</div> |
||||
|
||||
<div class="users"> |
||||
<div class="module"> |
||||
<form action="" id="isFollowingMeOpt"> |
||||
<p class="label">Show if a user follows me</p> |
||||
<select name="" id="isFollowingMe"> |
||||
<option value="in-profile">Show at profile modal only</option> |
||||
<option value="everywhere">Show with every user name</option> |
||||
</select> |
||||
</form> |
||||
</div> |
||||
</div> |
||||
|
||||
</div><!-- /tab-content --> |
||||
</div><!-- /options --> |
||||
</div><!-- /options --> |
||||
</body> |
||||
</html> |
@ -0,0 +1 @@
@@ -0,0 +1 @@
|
||||
../../css/OpenSans-Bold.ttf |
@ -0,0 +1 @@
@@ -0,0 +1 @@
|
||||
../../css/OpenSans-Regular.ttf |
@ -0,0 +1,443 @@
@@ -0,0 +1,443 @@
|
||||
/************************************** |
||||
********************* PROFILE PHOTO *** |
||||
***************************************/ |
||||
.profile-card |
||||
{ |
||||
padding: 9px; |
||||
background: rgba( 255, 255, 255, .5 ); |
||||
border: solid 1px rgba( 69, 71, 77, .05 ); |
||||
position: relative; |
||||
background: #43464d; |
||||
} |
||||
.profile-card-main |
||||
{ |
||||
width: 520px; |
||||
padding: 10px; |
||||
text-align: center; |
||||
position: relative; |
||||
transition: all .2s linear; |
||||
background: rgba(255,255,255, .1); |
||||
} |
||||
/*.profile-card-main:before |
||||
{ |
||||
content: ""; |
||||
border: solid 0px #fff; |
||||
transition: all .2s linear; |
||||
position: absolute; |
||||
left: 1px; |
||||
top: 1px; |
||||
right: 1px; |
||||
bottom: 1px; |
||||
z-index: 0; |
||||
}*/ |
||||
.profile-card-main * |
||||
{ |
||||
position: relative; |
||||
z-index: 1; |
||||
} |
||||
.profile-card-photo |
||||
{ |
||||
width: 74px; |
||||
height: 74px; |
||||
border: solid 3px #45474d; |
||||
border-radius: 10%; |
||||
box-sizing: content-box; |
||||
float:left; |
||||
top: 50%; |
||||
background-color: #3b3c41; |
||||
} |
||||
.profile-card-main h1 |
||||
{ |
||||
font-size: 24px; |
||||
font-weight: bold; |
||||
color: #fff; |
||||
} |
||||
.profile-card-main h2 { |
||||
color: #7691ce; |
||||
font-size: 14px; |
||||
} |
||||
.forEdition .profile-card-main h2 { |
||||
left: 20%; |
||||
top: 19%; |
||||
color: #f8f8f8; |
||||
} |
||||
.profile-card .direct-messages, |
||||
.profile-card .direct-messages-with-user, |
||||
.profile-card .follow, |
||||
.profile-card .profileUnfollow |
||||
{ |
||||
display: block; |
||||
position: absolute; |
||||
bottom: 20px; |
||||
right: 3px; |
||||
padding: 10px 3px; |
||||
font-size: 12px; |
||||
width: 120px; |
||||
text-align: center; |
||||
color: rgba( 0, 0, 0, .7 ); |
||||
background: rgba( 0, 0, 0, .1 ); |
||||
border: none; |
||||
transition: all .2s linear; |
||||
} |
||||
.profile-card .follow, |
||||
.profile-card .profileUnfollow |
||||
{ |
||||
right: 128px; |
||||
} |
||||
.profile-card .direct-messages:hover, |
||||
.profile-card .direct-messages-with-user:hover, |
||||
.profile-card .follow:hover, |
||||
.profile-card .profileUnfollow:hover |
||||
{ |
||||
background: rgba( 0, 0, 0, .3 ); |
||||
} |
||||
.profile-card.forEdition |
||||
{ |
||||
margin: 0 auto; |
||||
width: 540px; |
||||
} |
||||
.forEdition .profile-card-photo |
||||
{ |
||||
border: solid 2px #fff; |
||||
position: relative; |
||||
cursor: pointer; |
||||
transition: all .2s linear; |
||||
z-index: 10; |
||||
} |
||||
.forEdition .profile-card-main:hover:after, |
||||
.forEdition .profile-card-photo:after |
||||
{ |
||||
content: ""; |
||||
width: 36px; |
||||
height: 36px; |
||||
position: absolute; |
||||
top: -5px; |
||||
right: 0px; |
||||
background: url(../img/edit.png) no-repeat right top; |
||||
} |
||||
.forEdition .profile-card-main:hover:before |
||||
{ |
||||
border: solid 5px #fff; |
||||
} |
||||
.forEdition .profile-card-main h2 |
||||
{ |
||||
margin-bottom: 8px; |
||||
} |
||||
.forEdition .profile-card-main input |
||||
{ |
||||
display: block; |
||||
margin: 0 auto; |
||||
background: rgba( 255, 255, 255, .8 ); |
||||
border: none; |
||||
padding: 6px 4px; |
||||
margin-bottom: 4px; |
||||
text-align: center; |
||||
transition: all .2s linear; |
||||
} |
||||
.forEdition .profile-card-main input:hover |
||||
{ |
||||
background: rgba( 255, 255, 255, .7 ); |
||||
} |
||||
.forEdition .profile-card-photo:hover{ |
||||
background: #9096a5; |
||||
} |
||||
.forEdition .profile-card-main input:focus{ |
||||
background: #fff; |
||||
color: #4d4d4d; |
||||
} |
||||
|
||||
/* inputs placeholders color */ |
||||
.forEdition .profile-card-main input::-webkit-input-placeholder { |
||||
color: #4d4d4d; |
||||
} |
||||
.forEdition .profile-card-main input:-moz-placeholder { |
||||
color: #4d4d4d; |
||||
} |
||||
.forEdition .profile-card-main input::-moz-placeholder { |
||||
color: #4d4d4d; |
||||
} |
||||
.forEdition .profile-card-main input:-ms-input-placeholder { |
||||
color: #4d4d4d; |
||||
} |
||||
.forEdition .profile-card-main input:focus::-webkit-input-placeholder { |
||||
color: #fff; |
||||
} |
||||
.forEdition .profile-card-main input:focus:-moz-placeholder { |
||||
color: #fff; |
||||
} |
||||
.forEdition .profile-card-main input:focus::-moz-placeholder { |
||||
color: #fff; |
||||
} |
||||
.forEdition .profile-card-main input:focus::-ms-input-placeholder { |
||||
color: #fff; |
||||
} |
||||
|
||||
.profile-card-main input.input-name{ |
||||
position: absolute; |
||||
top: 30px; |
||||
right: 20px; |
||||
} |
||||
.profile-card-main input.input-description |
||||
{ |
||||
width: 90%; |
||||
margin-top: 100px; |
||||
} |
||||
.input-name |
||||
{ |
||||
font-size: 20px; |
||||
} |
||||
.forEdition .profile-card-main .input-website, |
||||
.forEdition .profile-card-main .input-city |
||||
{ |
||||
display: inline-block; |
||||
margin-top: 10px; |
||||
} |
||||
.forEdition .profile-card-main .input-tox, |
||||
.forEdition .profile-card-main .input-bitmessage |
||||
{ |
||||
width: 90%; |
||||
margin-top: 10px; |
||||
} |
||||
.profile-edition-buttons |
||||
{ |
||||
padding: 10px; |
||||
text-align: right; |
||||
} |
||||
.profile-edition-buttons button { |
||||
background: #f1f1f1; |
||||
color: #333; |
||||
} |
||||
.profile-edition-buttons button:hover { |
||||
background: #fff; |
||||
transition: background-color 300ms ease-in; |
||||
} |
||||
.secret-key-container .label { |
||||
color: #9096a5; |
||||
} |
||||
.secret-key-container .secret-key { |
||||
color: #d2dbf1; |
||||
} |
||||
/************************************* |
||||
****************** PROFILE MODAL |
||||
**************************************/ |
||||
.profile-modal .modal-wrapper |
||||
{ |
||||
width: 580px; |
||||
border-radius: 5px; |
||||
overflow: hidden; |
||||
position: absolute; |
||||
top:5%; |
||||
height: 90%; |
||||
margin-left: -300px; |
||||
|
||||
} |
||||
.profile-modal .modal-header, .profile-modal .profile-card { |
||||
z-index: 1; |
||||
} |
||||
.profile-modal .modal-content |
||||
{ |
||||
padding: 3px; |
||||
height: 100%; |
||||
} |
||||
.profile-modal h1.profile-name { |
||||
color: #43464d; |
||||
} |
||||
.profile-modal .profile-location { |
||||
color: #48577d; |
||||
} |
||||
.profile-modal .profile-url { |
||||
font-size: 14px; |
||||
font-style: italic; |
||||
} |
||||
.profile-modal .profile-bio { |
||||
text-align: center; |
||||
} |
||||
#msngrswr { |
||||
display: none; |
||||
margin-top: 20px; |
||||
height: 30px; |
||||
} |
||||
.profile-extra-contact { |
||||
float: left; |
||||
display: none; |
||||
margin-right: 35px; |
||||
} |
||||
.bitmessage-ctc, .tox-ctc { |
||||
position: absolute; |
||||
height: 30px; |
||||
width: 30px; |
||||
display: inline-block; |
||||
margin-right: 5px; |
||||
border: 1px solid #d6d8dc; |
||||
background: #e3e5ea url(img/clipboard.png) center no-repeat; |
||||
opacity: .8; |
||||
-webkit-border-radius: 0 5px 5px 0; |
||||
-moz-border-radius: 0 5px 5px 0; |
||||
border-radius: 0 5px 5px 0; |
||||
-webkit-transition: all 20ms; |
||||
-moz-transition: all 20ms; |
||||
-ms-transition: all 20ms; |
||||
-o-transition: all 20ms; |
||||
transition: all 20ms; |
||||
font-size: 18px; |
||||
} |
||||
.bitmessage-ctc:hover, .tox-ctc:hover { |
||||
background-color: #f0f2f8; |
||||
opacity: 1; |
||||
cursor: pointer; |
||||
text-decoration: none; |
||||
} |
||||
.bitmessage-ctc:active, .tox-ctc:active { |
||||
background-color: #edfced; |
||||
} |
||||
.bitmessage-ctc:after, |
||||
.tox-ctc:after { |
||||
content: '📋'; |
||||
} |
||||
.profile-modal .profile-tox, .profile-modal .profile-bitmessage { |
||||
display: inline-block; |
||||
width: 70px; |
||||
height: 30px; |
||||
border: 1px solid #c0c2c6; |
||||
opacity: .8; |
||||
-webkit-border-radius: 5px 0 0 5px; |
||||
-moz-border-radius: 5px 0 0 5px; |
||||
border-radius: 5px 0 0 5px; |
||||
-webkit-transition: all 200ms; |
||||
-moz-transition: all 200ms; |
||||
-ms-transition: all 200ms; |
||||
-o-transition: all 200ms; |
||||
transition: all 200ms; |
||||
} |
||||
.profile-modal .profile-tox:hover, .profile-modal .profile-bitmessage:hover { |
||||
opacity: 1; |
||||
background-color: #f0f2f8; |
||||
} |
||||
.profile-modal .profile-tox:active, .profile-modal .profile-bitmessage:active { |
||||
background-color: #edfced; |
||||
} |
||||
.profile-modal .profile-tox { |
||||
background: #e3e5ea url(../img/tox.png) center no-repeat; |
||||
} |
||||
.profile-modal .profile-bitmessage { |
||||
background: #e3e5ea url(../img/bm.png) center no-repeat; |
||||
} |
||||
.profile-modal .profile-data |
||||
{ |
||||
display: inline-block; |
||||
margin-left: -4px; |
||||
border-bottom: 0; |
||||
} |
||||
button.follow:hover { |
||||
background: #b2d67b; |
||||
color: #fff; |
||||
} |
||||
.profile-modal button.follow:hover { |
||||
background: #b2d67b; |
||||
color: #fff; |
||||
} |
||||
.profile-card .profileUnfollow:hover { |
||||
background: #e18882; |
||||
color: #fff; |
||||
} |
||||
.profile-modal button.direct-messages-with-user:hover { |
||||
background: #b2d67b; |
||||
color: #fff; |
||||
} |
||||
.profile-modal .separator { |
||||
height: 10px; |
||||
width: 100%; |
||||
background-color: #000; |
||||
} |
||||
.profile-modal .postboard |
||||
{ |
||||
margin-left: 0; |
||||
padding: 5px 0 5px 0; |
||||
height: 60%; |
||||
} |
||||
.profile-modal .postboard h2 { |
||||
width: auto; |
||||
} |
||||
.profile-modal .postboard-posts |
||||
{ |
||||
display: block; |
||||
height: 90%; |
||||
overflow: auto; |
||||
} |
||||
.profile-modal .postboard h2 span { |
||||
font: 18px/40px 'Open Sans Condensed', sans-serif; |
||||
padding-left: 10px; |
||||
} |
||||
.profile-modal .postboard-posts-wrapper |
||||
{ |
||||
position: absolute; |
||||
top: 0; |
||||
height: 100%; |
||||
width: 99%; |
||||
box-sizing: border-box; |
||||
border-top: 292px solid transparent; |
||||
overflow: auto; |
||||
z-index: 0; |
||||
} |
||||
.profile-modal .profile-card-main |
||||
{ |
||||
background: #f3f5fb; |
||||
color: #8d8d8d; |
||||
width:100%; |
||||
} |
||||
.profile-modal .profile-card-main a { |
||||
color: #8bb9e0; |
||||
} |
||||
.profile-modal .postboard-posts .post |
||||
{ |
||||
padding: 0; |
||||
} |
||||
.profile-modal .post-interactions |
||||
{ |
||||
margin: 2px 10px 3px 60px; |
||||
} |
||||
.profile-modal .profile-card |
||||
{ |
||||
margin: 0; |
||||
padding: 0; |
||||
background: #f3f5fb; |
||||
} |
||||
/* |
||||
.profile-card-main |
||||
{ |
||||
height: 200px; |
||||
} |
||||
*/ |
||||
.profile-modal .direct-messages, |
||||
.profile-modal .direct-messages-with-user, |
||||
.profile-modal .follow, |
||||
.profile-card .profileUnfollow |
||||
{ |
||||
bottom: 10px; |
||||
} |
||||
|
||||
h1.profile-name { |
||||
display: inline; |
||||
} |
||||
h2.profile-screen-name { |
||||
display: inline; |
||||
bottom: 0; |
||||
color: #8f95a4; |
||||
} |
||||
|
||||
.profile-modal .modal-buttons { |
||||
display: none; |
||||
} |
||||
|
||||
.profile-modal h1.profile-name, |
||||
.profile-modal h2.profile-screen-name, |
||||
.profile-modal span.profile-location, |
||||
.profile-modal a.profile-url { |
||||
display: block; |
||||
} |
||||
|
||||
.profile-modal span.profile-location:empty, |
||||
.profile-modal a.profile-url:empty, |
||||
.profile-modal a.profile-bio:empty { |
||||
display: none; |
||||
} |
After Width: | Height: | Size: 6.1 KiB |
After Width: | Height: | Size: 4.8 KiB |
After Width: | Height: | Size: 456 B |
After Width: | Height: | Size: 405 B |
After Width: | Height: | Size: 394 B |
After Width: | Height: | Size: 340 B |
After Width: | Height: | Size: 199 B |
After Width: | Height: | Size: 365 B |
After Width: | Height: | Size: 134 B |
After Width: | Height: | Size: 2.4 KiB |
After Width: | Height: | Size: 2.4 KiB |
After Width: | Height: | Size: 616 B |
After Width: | Height: | Size: 1.8 KiB |
After Width: | Height: | Size: 1.8 KiB |
After Width: | Height: | Size: 3.8 KiB |
After Width: | Height: | Size: 3.8 KiB |
After Width: | Height: | Size: 2.2 KiB |
After Width: | Height: | Size: 254 B |
After Width: | Height: | Size: 691 B |
After Width: | Height: | Size: 440 B |
After Width: | Height: | Size: 287 B |
After Width: | Height: | Size: 299 B |
After Width: | Height: | Size: 264 B |
After Width: | Height: | Size: 107 KiB |
After Width: | Height: | Size: 4.1 KiB |
After Width: | Height: | Size: 452 B |
After Width: | Height: | Size: 452 B |
After Width: | Height: | Size: 312 B |
After Width: | Height: | Size: 4.3 KiB |
After Width: | Height: | Size: 3.0 KiB |
After Width: | Height: | Size: 564 B |
After Width: | Height: | Size: 78 B |
@ -0,0 +1 @@
@@ -0,0 +1 @@
|
||||
../../../css/OpenSans-Bold.ttf |
@ -0,0 +1 @@
@@ -0,0 +1 @@
|
||||
../../../css/OpenSans-Regular.ttf |