mirror of
https://github.com/twisterarmy/twister-core.git
synced 2025-01-10 06:48:07 +00:00
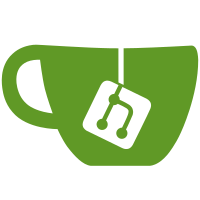
- this pull adds an InitMessage() function to noui.cpp, which outputs init messages to debug.log (this allows to remove some printf() calls from init.cpp) - change InitMessage() in bitcoin.cpp to also write init messages to debug.log to ensure nothting is missing in the log because of the removal of printf() calls in init.cpp
52 lines
1.5 KiB
C++
52 lines
1.5 KiB
C++
// Copyright (c) 2010 Satoshi Nakamoto
|
|
// Copyright (c) 2009-2012 The Bitcoin developers
|
|
// Distributed under the MIT/X11 software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#include "ui_interface.h"
|
|
#include "init.h"
|
|
#include "bitcoinrpc.h"
|
|
|
|
#include <string>
|
|
|
|
static int noui_ThreadSafeMessageBox(const std::string& message, const std::string& caption, unsigned int style)
|
|
{
|
|
std::string strCaption;
|
|
// Check for usage of predefined caption
|
|
switch (style) {
|
|
case CClientUIInterface::MSG_ERROR:
|
|
strCaption += _("Error");
|
|
break;
|
|
case CClientUIInterface::MSG_WARNING:
|
|
strCaption += _("Warning");
|
|
break;
|
|
case CClientUIInterface::MSG_INFORMATION:
|
|
strCaption += _("Information");
|
|
break;
|
|
default:
|
|
strCaption += caption; // Use supplied caption (can be empty)
|
|
}
|
|
|
|
printf("%s: %s\n", strCaption.c_str(), message.c_str());
|
|
fprintf(stderr, "%s: %s\n", strCaption.c_str(), message.c_str());
|
|
return 4;
|
|
}
|
|
|
|
static bool noui_ThreadSafeAskFee(int64 /*nFeeRequired*/)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
static void noui_InitMessage(const std::string &message)
|
|
{
|
|
printf("init message: %s\n", message.c_str());
|
|
}
|
|
|
|
void noui_connect()
|
|
{
|
|
// Connect bitcoind signal handlers
|
|
uiInterface.ThreadSafeMessageBox.connect(noui_ThreadSafeMessageBox);
|
|
uiInterface.ThreadSafeAskFee.connect(noui_ThreadSafeAskFee);
|
|
uiInterface.InitMessage.connect(noui_InitMessage);
|
|
}
|