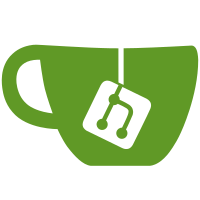
13 changed files with 452 additions and 241 deletions
@ -0,0 +1,88 @@
@@ -0,0 +1,88 @@
|
||||
/* |
||||
* https://github.com/morethanwords/tweb
|
||||
* Copyright (C) 2019-2021 Eduard Kuzmenko |
||||
* https://github.com/morethanwords/tweb/blob/master/LICENSE
|
||||
*/ |
||||
|
||||
import InputField from "../inputField"; |
||||
import PopupElement from "."; |
||||
import { attachClickEvent } from "../../helpers/dom/clickEvent"; |
||||
import EditPeer from "../editPeer"; |
||||
import { _i18n } from "../../lib/langPack"; |
||||
import TelInputField from "../telInputField"; |
||||
import appUsersManager from "../../lib/appManagers/appUsersManager"; |
||||
import { formatPhoneNumber } from "../../helpers/formatPhoneNumber"; |
||||
import { toastNew } from "../toast"; |
||||
|
||||
export default class PopupCreateContact extends PopupElement { |
||||
constructor() { |
||||
super('popup-create-contact popup-send-photo popup-new-media', null, {closable: true, withConfirm: 'Add'}); |
||||
|
||||
_i18n(this.title, 'AddContactTitle'); |
||||
|
||||
attachClickEvent(this.btnConfirm, () => { |
||||
const promise = appUsersManager.importContact(nameInputField.value, lastNameInputField.value, telInputField.value); |
||||
|
||||
promise.then(() => { |
||||
this.hide(); |
||||
}, (err) => { |
||||
if(err.type === 'NO_USER') { |
||||
toastNew({langPackKey: 'Contacts.PhoneNumber.NotRegistred'}); |
||||
editPeer.disabled = false; |
||||
} |
||||
}); |
||||
|
||||
editPeer.lockWithPromise(promise); |
||||
}, {listenerSetter: this.listenerSetter}); |
||||
|
||||
const inputFields: InputField[] = []; |
||||
const div = document.createElement('div'); |
||||
div.classList.add('name-fields'); |
||||
const nameInputField = new InputField({ |
||||
label: 'FirstName', |
||||
name: 'create-contact-name', |
||||
maxLength: 70, |
||||
required: true |
||||
}); |
||||
const lastNameInputField = new InputField({ |
||||
label: 'LastName', |
||||
name: 'create-contact-lastname', |
||||
maxLength: 70 |
||||
}); |
||||
const telInputField = new TelInputField({required: true}); |
||||
inputFields.push(nameInputField, lastNameInputField, telInputField); |
||||
|
||||
const onInput = () => { |
||||
const name = nameInputField.value + ' ' + lastNameInputField.value; |
||||
// const abbr = RichTextProcessor.getAbbreviation(name);
|
||||
editPeer.avatarElem.setAttribute('peer-title', name); |
||||
editPeer.avatarElem.update(); |
||||
}; |
||||
|
||||
this.listenerSetter.add(nameInputField.input)('input', onInput); |
||||
this.listenerSetter.add(lastNameInputField.input)('input', onInput); |
||||
|
||||
const user = appUsersManager.getSelf(); |
||||
const formatted = formatPhoneNumber(user.phone); |
||||
if(formatted) { |
||||
telInputField.validate = () => { |
||||
return !!telInputField.value.match(/\d/); |
||||
}; |
||||
|
||||
telInputField.value = '+' + formatted.code.country_code; |
||||
} |
||||
|
||||
const editPeer = new EditPeer({ |
||||
inputFields, |
||||
listenerSetter: this.listenerSetter, |
||||
doNotEditAvatar: true, |
||||
nextBtn: this.btnConfirm, |
||||
avatarSize: 100 |
||||
}); |
||||
|
||||
div.append(nameInputField.container, lastNameInputField.container, editPeer.avatarElem); |
||||
this.container.append(div, telInputField.container); |
||||
|
||||
this.show(); |
||||
} |
||||
} |
@ -1,14 +0,0 @@
@@ -1,14 +0,0 @@
|
||||
/* |
||||
* https://github.com/morethanwords/tweb
|
||||
* Copyright (C) 2019-2021 Eduard Kuzmenko |
||||
* https://github.com/morethanwords/tweb/blob/master/LICENSE
|
||||
*/ |
||||
|
||||
import { SliderSuperTab } from "../../slider"; |
||||
|
||||
export default class AppAddContactTab extends SliderSuperTab { |
||||
protected init() { |
||||
this.container.classList.add('add-contact-container'); |
||||
this.setTitle('AddContactTitle'); |
||||
} |
||||
} |
@ -0,0 +1,110 @@
@@ -0,0 +1,110 @@
|
||||
/* |
||||
* https://github.com/morethanwords/tweb
|
||||
* Copyright (C) 2019-2021 Eduard Kuzmenko |
||||
* https://github.com/morethanwords/tweb/blob/master/LICENSE
|
||||
*/ |
||||
|
||||
import placeCaretAtEnd from "../helpers/dom/placeCaretAtEnd"; |
||||
import { formatPhoneNumber } from "../helpers/formatPhoneNumber"; |
||||
import { isApple, isAndroid, isAppleMobile } from "../helpers/userAgent"; |
||||
import { HelpCountry, HelpCountryCode } from "../layer"; |
||||
import InputField, { InputFieldOptions } from "./inputField"; |
||||
|
||||
export default class TelInputField extends InputField { |
||||
private pasted = false; |
||||
public lastValue = ''; |
||||
|
||||
constructor(options: InputFieldOptions & { |
||||
onInput?: (formatted: ReturnType<typeof formatPhoneNumber>) => void |
||||
} = {}) { |
||||
super({ |
||||
label: 'Contacts.PhoneNumber.Placeholder', |
||||
//plainText: true,
|
||||
name: 'phone', |
||||
...options |
||||
}); |
||||
|
||||
this.container.classList.add('input-field-phone'); |
||||
|
||||
let telEl = this.input; |
||||
if(telEl instanceof HTMLInputElement) { |
||||
telEl.type = 'tel'; |
||||
telEl.autocomplete = 'rr55RandomRR55'; |
||||
} else { |
||||
telEl.inputMode = 'decimal'; |
||||
|
||||
const pixelRatio = window.devicePixelRatio; |
||||
if(pixelRatio > 1) { |
||||
let letterSpacing: number; |
||||
if(isApple) { |
||||
letterSpacing = pixelRatio * -.16; |
||||
} else if(isAndroid) { |
||||
letterSpacing = 0; |
||||
} |
||||
|
||||
telEl.style.setProperty('--letter-spacing', letterSpacing + 'px'); |
||||
} |
||||
|
||||
const originalFunc = this.setValueSilently.bind(this); |
||||
this.setValueSilently = (value) => { |
||||
originalFunc(value); |
||||
placeCaretAtEnd(this.input, true); |
||||
}; |
||||
} |
||||
|
||||
telEl.addEventListener('input', () => { |
||||
//console.log('input', this.value);
|
||||
telEl.classList.remove('error'); |
||||
|
||||
const value = this.value; |
||||
const diff = Math.abs(value.length - this.lastValue.length); |
||||
if(diff > 1 && !this.pasted && isAppleMobile) { |
||||
this.setValueSilently(this.lastValue + value); |
||||
} |
||||
|
||||
this.pasted = false; |
||||
|
||||
this.setLabel(); |
||||
|
||||
let formattedPhoneNumber: ReturnType<typeof formatPhoneNumber>; |
||||
let formatted: string, country: HelpCountry, countryCode: HelpCountryCode, leftPattern = ''; |
||||
if(this.value.replace(/\++/, '+') === '+') { |
||||
this.setValueSilently('+'); |
||||
} else { |
||||
formattedPhoneNumber = formatPhoneNumber(this.value); |
||||
formatted = formattedPhoneNumber.formatted; |
||||
country = formattedPhoneNumber.country; |
||||
leftPattern = formattedPhoneNumber.leftPattern; |
||||
countryCode = formattedPhoneNumber.code; |
||||
this.setValueSilently(this.lastValue = formatted ? '+' + formatted : ''); |
||||
} |
||||
|
||||
telEl.dataset.leftPattern = leftPattern/* .replace(/X/g, '0') */; |
||||
|
||||
//console.log(formatted, country);
|
||||
|
||||
options.onInput && options.onInput(formattedPhoneNumber); |
||||
}); |
||||
|
||||
telEl.addEventListener('paste', () => { |
||||
this.pasted = true; |
||||
//console.log('paste', telEl.value);
|
||||
}); |
||||
|
||||
/* telEl.addEventListener('change', (e) => { |
||||
console.log('change', telEl.value); |
||||
}); */ |
||||
|
||||
telEl.addEventListener('keypress', (e) => { |
||||
//console.log('keypress', this.value);
|
||||
if(/\D/.test(e.key) && !(e.metaKey || e.ctrlKey) && e.key !== 'Backspace' && !(e.key === '+' && e.shiftKey/* && !this.value */)) { |
||||
e.preventDefault(); |
||||
return false; |
||||
} |
||||
}); |
||||
|
||||
/* telEl.addEventListener('focus', function(this: typeof telEl, e) { |
||||
this.removeAttribute('readonly'); // fix autocomplete
|
||||
});*/ |
||||
} |
||||
} |
Loading…
Reference in new issue