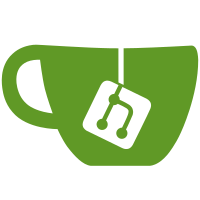
14 changed files with 171 additions and 23 deletions
@ -0,0 +1,5 @@
@@ -0,0 +1,5 @@
|
||||
API_ID=1025907 |
||||
API_HASH=452b0359b988148995f22ff0f4229750 |
||||
VERSION=0.8.6 |
||||
VERSION_FULL=0.8.6 (1) |
||||
BUILD=1 |
@ -0,0 +1,39 @@
@@ -0,0 +1,39 @@
|
||||
// @ts-check
|
||||
|
||||
const { spawn } = require('child_process'); |
||||
|
||||
const version = process.argv[2] || 'same'; |
||||
const changelog = ''; |
||||
const child = spawn(`npm`, ['run', 'change-version', version, changelog].filter(Boolean)); |
||||
child.stdout.on('data', (chunk) => { |
||||
console.log(chunk.toString()); |
||||
}); |
||||
|
||||
child.on('close', (code) => { |
||||
if(code != 0) { |
||||
console.log(`child process exited with code ${code}`); |
||||
} |
||||
|
||||
const child = spawn(`npm`, ['run', 'build']); |
||||
child.stdout.on('data', (chunk) => { |
||||
console.log(chunk.toString()); |
||||
}); |
||||
|
||||
child.on('close', (code) => { |
||||
if(code != 0) { |
||||
console.error(`child process exited with code ${code}`); |
||||
} else { |
||||
console.log('Compiled successfully.'); |
||||
} |
||||
}); |
||||
}); |
||||
|
||||
/* exec(`npm run change-version ${version}${changelog ? ' ' + changelog : ''}; npm run build`, (err, stdout, stderr) => { |
||||
if(err) { |
||||
return; |
||||
} |
||||
|
||||
// the *entire* stdout and stderr (buffered)
|
||||
console.log(`stdout: ${stdout}`); |
||||
console.log(`stderr: ${stderr}`); |
||||
}); */ |
@ -0,0 +1,50 @@
@@ -0,0 +1,50 @@
|
||||
/* |
||||
* https://github.com/morethanwords/tweb
|
||||
* Copyright (C) 2019-2021 Eduard Kuzmenko |
||||
* https://github.com/morethanwords/tweb/blob/master/LICENSE
|
||||
*/ |
||||
|
||||
// @ts-check
|
||||
|
||||
const fs = require('fs'); |
||||
|
||||
const version = process.argv[2]; |
||||
const changelog = process.argv[3]; |
||||
|
||||
const envStr = fs.readFileSync('./.env').toString(); |
||||
const env = {}; |
||||
envStr.split('\n').forEach(line => { |
||||
if(!line) return; |
||||
const [key, value] = line.split('=', 2); |
||||
env[key] = value; |
||||
}); |
||||
|
||||
if(version !== 'same') { |
||||
env.VERSION = version; |
||||
} |
||||
|
||||
env.BUILD = +env.BUILD + 1; |
||||
env.VERSION_FULL = `${env.VERSION} (${env.BUILD})`; |
||||
|
||||
const lines = []; |
||||
for(const key in env) { |
||||
lines.push(`${key}=${env[key]}`); |
||||
} |
||||
fs.writeFileSync('./.env', lines.join('\n') + '\n', 'utf-8'); |
||||
|
||||
if(changelog) { |
||||
const data = fs.readFileSync('./CHANGELOG.md'); |
||||
const fd = fs.openSync('./CHANGELOG.md', 'w+'); |
||||
const lines = [ |
||||
`### ${env.VERSION_FULL}` |
||||
]; |
||||
changelog.trim().split('\n').forEach(line => { |
||||
lines.push(`* ${line}`); |
||||
}); |
||||
const insert = Buffer.from(lines.join('\n') + '\n\n'); |
||||
fs.writeSync(fd, insert, 0, insert.length, 0); |
||||
fs.writeSync(fd, data, 0, data.length, insert.length); |
||||
fs.close(fd, () => { |
||||
process.exit(0); |
||||
}); |
||||
} |
Loading…
Reference in new issue