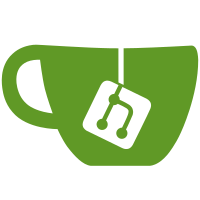
26 changed files with 406 additions and 170 deletions
@ -0,0 +1,125 @@
@@ -0,0 +1,125 @@
|
||||
// * Jolly Cobra's schedulers
|
||||
import { AnyToVoidFunction } from "../types"; |
||||
|
||||
//type Scheduler = typeof requestAnimationFrame | typeof onTickEnd | typeof runNow;
|
||||
|
||||
export function debounce<F extends AnyToVoidFunction>( |
||||
fn: F, |
||||
ms: number, |
||||
shouldRunFirst = true, |
||||
shouldRunLast = true, |
||||
) { |
||||
let waitingTimeout: number | null = null; |
||||
|
||||
return (...args: Parameters<F>) => { |
||||
if(waitingTimeout) { |
||||
clearTimeout(waitingTimeout); |
||||
waitingTimeout = null; |
||||
} else if(shouldRunFirst) { |
||||
// @ts-ignore
|
||||
fn(...args); |
||||
} |
||||
|
||||
waitingTimeout = window.setTimeout(() => { |
||||
if(shouldRunLast) { |
||||
// @ts-ignore
|
||||
fn(...args); |
||||
} |
||||
|
||||
waitingTimeout = null; |
||||
}, ms); |
||||
}; |
||||
} |
||||
|
||||
/* export function throttle<F extends AnyToVoidFunction>( |
||||
fn: F, |
||||
ms: number, |
||||
shouldRunFirst = true, |
||||
) { |
||||
let interval: number | null = null; |
||||
let isPending: boolean; |
||||
let args: Parameters<F>; |
||||
|
||||
return (..._args: Parameters<F>) => { |
||||
isPending = true; |
||||
args = _args; |
||||
|
||||
if (!interval) { |
||||
if (shouldRunFirst) { |
||||
isPending = false; |
||||
// @ts-ignore
|
||||
fn(...args); |
||||
} |
||||
|
||||
interval = window.setInterval(() => { |
||||
if (!isPending) { |
||||
window.clearInterval(interval!); |
||||
interval = null; |
||||
return; |
||||
} |
||||
|
||||
isPending = false; |
||||
// @ts-ignore
|
||||
fn(...args); |
||||
}, ms); |
||||
} |
||||
}; |
||||
} */ |
||||
|
||||
/* export function throttleWithRaf<F extends AnyToVoidFunction>(fn: F) { |
||||
return throttleWith(fastRaf, fn); |
||||
} |
||||
|
||||
export function throttleWithTickEnd<F extends AnyToVoidFunction>(fn: F) { |
||||
return throttleWith(onTickEnd, fn); |
||||
} |
||||
|
||||
export function throttleWithNow<F extends AnyToVoidFunction>(fn: F) { |
||||
return throttleWith(runNow, fn); |
||||
} |
||||
|
||||
export function throttleWith<F extends AnyToVoidFunction>(schedulerFn: Scheduler, fn: F) { |
||||
let waiting = false; |
||||
let args: Parameters<F>; |
||||
|
||||
return (..._args: Parameters<F>) => { |
||||
args = _args; |
||||
|
||||
if (!waiting) { |
||||
waiting = true; |
||||
|
||||
schedulerFn(() => { |
||||
waiting = false; |
||||
// @ts-ignore
|
||||
fn(...args); |
||||
}); |
||||
} |
||||
}; |
||||
} |
||||
|
||||
export function onTickEnd(cb: NoneToVoidFunction) { |
||||
Promise.resolve().then(cb); |
||||
} |
||||
|
||||
function runNow(fn: NoneToVoidFunction) { |
||||
fn(); |
||||
} */ |
||||
|
||||
export const pause = (ms: number) => new Promise((resolve) => { |
||||
setTimeout(resolve, ms); |
||||
}); |
||||
|
||||
/* let fastRafCallbacks: NoneToVoidFunction[] | undefined; |
||||
export function fastRaf(callback: NoneToVoidFunction) { |
||||
if (!fastRafCallbacks) { |
||||
fastRafCallbacks = [callback]; |
||||
|
||||
requestAnimationFrame(() => { |
||||
const currentCallbacks = fastRafCallbacks!; |
||||
fastRafCallbacks = undefined; |
||||
currentCallbacks.forEach((cb) => cb()); |
||||
}); |
||||
} else { |
||||
fastRafCallbacks.push(callback); |
||||
} |
||||
} */ |
Loading…
Reference in new issue