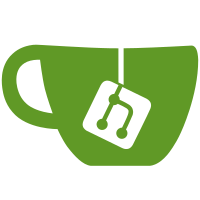
14 changed files with 667 additions and 0 deletions
@ -0,0 +1,131 @@
@@ -0,0 +1,131 @@
|
||||
/* |
||||
* Copyright 2014 A.C.R. Development |
||||
*/ |
||||
package acr.browser.lightning.constant; |
||||
|
||||
import android.app.Application; |
||||
import android.support.annotation.NonNull; |
||||
import android.support.annotation.Nullable; |
||||
|
||||
import com.anthonycr.bonsai.Single; |
||||
import com.anthonycr.bonsai.SingleAction; |
||||
import com.anthonycr.bonsai.SingleOnSubscribe; |
||||
import com.anthonycr.bonsai.SingleSubscriber; |
||||
|
||||
import java.io.File; |
||||
import java.io.FileWriter; |
||||
import java.io.IOException; |
||||
import java.util.List; |
||||
|
||||
import javax.inject.Inject; |
||||
|
||||
import acr.browser.lightning.R; |
||||
import acr.browser.lightning.app.BrowserApp; |
||||
import acr.browser.lightning.database.downloads.DownloadItem; |
||||
import acr.browser.lightning.database.downloads.DownloadsModel; |
||||
import acr.browser.lightning.utils.Preconditions; |
||||
import acr.browser.lightning.utils.Utils; |
||||
|
||||
public final class DownloadsPage { |
||||
|
||||
/** |
||||
* The download page standard suffix |
||||
*/ |
||||
public static final String FILENAME = "downloads.html"; |
||||
|
||||
private static final String HEADING_1 = "<!DOCTYPE html><html xmlns=http://www.w3.org/1999/xhtml>\n" + |
||||
"<head>\n" + |
||||
"<meta content=en-us http-equiv=Content-Language />\n" + |
||||
"<meta content='text/html; charset=utf-8' http-equiv=Content-Type />\n" + |
||||
"<meta name=viewport content='width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no'>\n" + |
||||
"<title>"; |
||||
|
||||
private static final String HEADING_2 = "</title>\n" + |
||||
"</head>\n" + |
||||
"<style>body{background:#f5f5f5;max-width:100%;min-height:100%}#content{width:100%;max-width:800px;margin:0 auto;text-align:center}.box{vertical-align:middle;text-align:center;position:relative;display:inline-block;height:45px;width:80%;margin:10px;background-color:#fff;box-shadow:0 3px 6px rgba(0,0,0,0.25);font-family:Arial;color:#444;font-size:12px;-moz-border-radius:2px;-webkit-border-radius:2px;border-radius:2px}.box-content{height:25px;width:100%;vertical-align:middle;text-align:center}p.ellipses{" + |
||||
"width:100%;font-size: small;font-family: Arial, Helvetica, 'sans-serif';white-space:nowrap;overflow:hidden;text-align:left;vertical-align:middle;margin:auto;text-overflow:ellipsis;-o-text-overflow:ellipsis;-ms-text-overflow:ellipsis}.box a{width:100%;height:100%;position:absolute;left:0;top:0}img{vertical-align:middle;margin-right:10px;width:20px;height:20px;}.margin{margin:10px}</style>\n" + |
||||
"<body><div id=content>"; |
||||
|
||||
private static final String PART1 = "<div class=box><a href='"; |
||||
|
||||
private static final String PART2 = "'></a>\n" + |
||||
"<div class=margin>\n" + |
||||
"<div class=box-content>\n" + |
||||
"<p class=ellipses><b>"; |
||||
|
||||
private static final String PART3 = "</b></p>\n<p class=ellipses>"; |
||||
|
||||
private static final String PART4 = "</p></div></div></div>"; |
||||
|
||||
private static final String END = "</div></body></html>"; |
||||
|
||||
private File mFilesDir; |
||||
|
||||
@Inject Application mApp; |
||||
@Inject DownloadsModel mManager; |
||||
|
||||
@NonNull private final String mTitle; |
||||
|
||||
public DownloadsPage() { |
||||
BrowserApp.getAppComponent().inject(this); |
||||
mTitle = mApp.getString(R.string.action_downloads); |
||||
} |
||||
|
||||
@NonNull |
||||
public Single<String> getDownloadsPage() { |
||||
return Single.create(new SingleAction<String>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<String> subscriber) { |
||||
mFilesDir = mApp.getFilesDir(); |
||||
|
||||
buildDownloadsPage(null); |
||||
|
||||
File downloadsWebPage = new File(mFilesDir, FILENAME); |
||||
|
||||
subscriber.onItem(Constants.FILE + downloadsWebPage); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
private void buildDownloadsPage(@Nullable final String folder) { |
||||
mManager.getAllDownloads() |
||||
.subscribe(new SingleOnSubscribe<List<DownloadItem>>() { |
||||
@Override |
||||
public void onItem(@Nullable List<DownloadItem> list) { |
||||
Preconditions.checkNonNull(list); |
||||
|
||||
final File downloadsWebPage; |
||||
if (folder == null || folder.isEmpty()) { |
||||
downloadsWebPage = new File(mFilesDir, FILENAME); |
||||
} else { |
||||
downloadsWebPage = new File(mFilesDir, folder + '-' + FILENAME); |
||||
} |
||||
final StringBuilder downloadsBuilder = new StringBuilder(HEADING_1 + mTitle + HEADING_2); |
||||
|
||||
for (int n = 0, size = list.size(); n < size; n++) { |
||||
final DownloadItem item = list.get(n); |
||||
downloadsBuilder.append(PART1); |
||||
downloadsBuilder.append(item.getUrl()); |
||||
downloadsBuilder.append(PART2); |
||||
downloadsBuilder.append(item.getTitle()); |
||||
downloadsBuilder.append(PART3); |
||||
downloadsBuilder.append(item.getContentSize()); |
||||
downloadsBuilder.append(PART4); |
||||
} |
||||
downloadsBuilder.append(END); |
||||
FileWriter bookWriter = null; |
||||
try { |
||||
//noinspection IOResourceOpenedButNotSafelyClosed
|
||||
bookWriter = new FileWriter(downloadsWebPage, false); |
||||
bookWriter.write(downloadsBuilder.toString()); |
||||
} catch (IOException e) { |
||||
e.printStackTrace(); |
||||
} finally { |
||||
Utils.close(bookWriter); |
||||
} |
||||
} |
||||
}); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,96 @@
@@ -0,0 +1,96 @@
|
||||
/* |
||||
* Copyright 2014 A.C.R. Development |
||||
*/ |
||||
package acr.browser.lightning.database.downloads; |
||||
|
||||
import android.support.annotation.NonNull; |
||||
import android.support.annotation.Nullable; |
||||
|
||||
import acr.browser.lightning.utils.Preconditions; |
||||
|
||||
public class DownloadItem implements Comparable<DownloadItem> { |
||||
|
||||
// private variables
|
||||
@NonNull |
||||
private String mUrl = ""; |
||||
|
||||
@NonNull |
||||
private String mTitle = ""; |
||||
|
||||
@NonNull |
||||
private String mContentSize = ""; |
||||
|
||||
public DownloadItem() {} |
||||
|
||||
public DownloadItem(@NonNull String url, @NonNull String title, @NonNull String size) { |
||||
Preconditions.checkNonNull(url); |
||||
Preconditions.checkNonNull(title); |
||||
this.mUrl = url; |
||||
this.mTitle = title; |
||||
this.mContentSize = size; |
||||
} |
||||
|
||||
@NonNull |
||||
public String getUrl() { |
||||
return this.mUrl; |
||||
} |
||||
|
||||
public void setUrl(@Nullable String url) { |
||||
this.mUrl = (url == null) ? "" : url; |
||||
} |
||||
|
||||
@NonNull |
||||
public String getTitle() { |
||||
return this.mTitle; |
||||
} |
||||
|
||||
public void setTitle(@Nullable String title) { |
||||
this.mTitle = (title == null) ? "" : title; |
||||
} |
||||
|
||||
@NonNull |
||||
public String getContentSize() { |
||||
return this.mContentSize; |
||||
} |
||||
|
||||
public void setContentSize(@Nullable String size) { |
||||
this.mContentSize = (size == null) ? "" : size; |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public String toString() { |
||||
return mTitle; |
||||
} |
||||
|
||||
@Override |
||||
public int compareTo(@NonNull DownloadItem another) { |
||||
int compare = this.mTitle.compareTo(another.mTitle); |
||||
if (compare == 0) { |
||||
return this.mUrl.compareTo(another.mUrl); |
||||
} |
||||
return compare; |
||||
} |
||||
|
||||
@Override |
||||
public boolean equals(@Nullable Object object) { |
||||
|
||||
if (this == object) return true; |
||||
if (object == null) return false; |
||||
if (!(object instanceof DownloadItem)) return false; |
||||
|
||||
DownloadItem that = (DownloadItem) object; |
||||
|
||||
return this.mTitle.equals(that.mTitle) && this.mUrl.equals(that.mUrl) |
||||
&& this.mContentSize.equals(that.mContentSize); |
||||
} |
||||
|
||||
@Override |
||||
public int hashCode() { |
||||
|
||||
int result = mUrl.hashCode(); |
||||
result = 31 * result + mTitle.hashCode(); |
||||
|
||||
return result; |
||||
} |
||||
} |
@ -0,0 +1,261 @@
@@ -0,0 +1,261 @@
|
||||
package acr.browser.lightning.database.downloads; |
||||
|
||||
import android.app.Application; |
||||
import android.content.ContentValues; |
||||
import android.database.Cursor; |
||||
import android.database.DatabaseUtils; |
||||
import android.database.sqlite.SQLiteDatabase; |
||||
import android.database.sqlite.SQLiteOpenHelper; |
||||
import android.support.annotation.NonNull; |
||||
import android.support.annotation.Nullable; |
||||
import android.support.annotation.WorkerThread; |
||||
|
||||
import com.anthonycr.bonsai.Completable; |
||||
import com.anthonycr.bonsai.CompletableAction; |
||||
import com.anthonycr.bonsai.CompletableSubscriber; |
||||
import com.anthonycr.bonsai.Single; |
||||
import com.anthonycr.bonsai.SingleAction; |
||||
import com.anthonycr.bonsai.SingleSubscriber; |
||||
|
||||
import java.util.ArrayList; |
||||
import java.util.List; |
||||
|
||||
import javax.inject.Inject; |
||||
import javax.inject.Singleton; |
||||
|
||||
import acr.browser.lightning.R; |
||||
|
||||
/** |
||||
* The disk backed download database. |
||||
* See {@link DownloadsModel} for method |
||||
* documentation. |
||||
*/ |
||||
@Singleton |
||||
public class DownloadsDatabase extends SQLiteOpenHelper implements DownloadsModel { |
||||
|
||||
private static final String TAG = "DownloadsDatabase"; |
||||
|
||||
// Database Version
|
||||
private static final int DATABASE_VERSION = 1; |
||||
|
||||
// Database Name
|
||||
private static final String DATABASE_NAME = "downloadManager"; |
||||
|
||||
// HistoryItems table name
|
||||
private static final String TABLE_DOWNLOADS = "download"; |
||||
|
||||
// HistoryItems Table Columns names
|
||||
private static final String KEY_ID = "id"; |
||||
private static final String KEY_URL = "url"; |
||||
private static final String KEY_TITLE = "title"; |
||||
private static final String KEY_SIZE = "size"; |
||||
|
||||
@NonNull private final String DEFAULT_DOWNLOADS_TITLE; |
||||
|
||||
@Nullable private SQLiteDatabase mDatabase; |
||||
|
||||
@Inject |
||||
public DownloadsDatabase(@NonNull Application application) { |
||||
super(application, DATABASE_NAME, null, DATABASE_VERSION); |
||||
DEFAULT_DOWNLOADS_TITLE = application.getString(R.string.untitled); |
||||
} |
||||
|
||||
/** |
||||
* Lazily initializes the database |
||||
* field when called. |
||||
* |
||||
* @return a non null writable database. |
||||
*/ |
||||
@WorkerThread |
||||
@NonNull |
||||
private SQLiteDatabase lazyDatabase() { |
||||
if (mDatabase == null || !mDatabase.isOpen()) { |
||||
mDatabase = getWritableDatabase(); |
||||
} |
||||
|
||||
return mDatabase; |
||||
} |
||||
|
||||
// Creating Tables
|
||||
@Override |
||||
public void onCreate(@NonNull SQLiteDatabase db) { |
||||
String CREATE_BOOKMARK_TABLE = "CREATE TABLE " + |
||||
DatabaseUtils.sqlEscapeString(TABLE_DOWNLOADS) + '(' + |
||||
DatabaseUtils.sqlEscapeString(KEY_ID) + " INTEGER PRIMARY KEY," + |
||||
DatabaseUtils.sqlEscapeString(KEY_URL) + " TEXT," + |
||||
DatabaseUtils.sqlEscapeString(KEY_TITLE) + " TEXT," + |
||||
DatabaseUtils.sqlEscapeString(KEY_SIZE) + " TEXT" + ')'; |
||||
db.execSQL(CREATE_BOOKMARK_TABLE); |
||||
} |
||||
|
||||
// Upgrading database
|
||||
@Override |
||||
public void onUpgrade(@NonNull SQLiteDatabase db, int oldVersion, int newVersion) { |
||||
// Drop older table if it exists
|
||||
db.execSQL("DROP TABLE IF EXISTS " + DatabaseUtils.sqlEscapeString(TABLE_DOWNLOADS)); |
||||
// Create tables again
|
||||
onCreate(db); |
||||
} |
||||
|
||||
@NonNull |
||||
private static ContentValues bindBookmarkToContentValues(@NonNull DownloadItem downloadItem) { |
||||
ContentValues contentValues = new ContentValues(3); |
||||
contentValues.put(KEY_TITLE, downloadItem.getTitle()); |
||||
contentValues.put(KEY_URL, downloadItem.getUrl()); |
||||
contentValues.put(KEY_SIZE, downloadItem.getContentSize()); |
||||
|
||||
return contentValues; |
||||
} |
||||
|
||||
@NonNull |
||||
private static DownloadItem bindCursorToDownloadItem(@NonNull Cursor cursor) { |
||||
DownloadItem download = new DownloadItem(); |
||||
|
||||
download.setUrl(cursor.getString(cursor.getColumnIndex(KEY_URL))); |
||||
download.setTitle(cursor.getString(cursor.getColumnIndex(KEY_TITLE))); |
||||
download.setContentSize(cursor.getString(cursor.getColumnIndex(KEY_SIZE))); |
||||
|
||||
return download; |
||||
} |
||||
|
||||
@NonNull |
||||
private static List<DownloadItem> bindCursorToDownloadItemList(@NonNull Cursor cursor) { |
||||
List<DownloadItem> downloads = new ArrayList<>(); |
||||
|
||||
while (cursor.moveToNext()) { |
||||
downloads.add(bindCursorToDownloadItem(cursor)); |
||||
} |
||||
|
||||
cursor.close(); |
||||
|
||||
return downloads; |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<DownloadItem> findDownloadForUrl(@NonNull final String url) { |
||||
return Single.create(new SingleAction<DownloadItem>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<DownloadItem> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_DOWNLOADS, null, KEY_URL + "=?", new String[]{url}, null, null, "1"); |
||||
|
||||
if (cursor.moveToFirst()) { |
||||
subscriber.onItem(bindCursorToDownloadItem(cursor)); |
||||
} else { |
||||
subscriber.onItem(null); |
||||
} |
||||
|
||||
cursor.close(); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<Boolean> isDownload(@NonNull final String url) { |
||||
return Single.create(new SingleAction<Boolean>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<Boolean> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_DOWNLOADS, null, KEY_URL + "=?", new String[]{url}, null, null, null, "1"); |
||||
|
||||
subscriber.onItem(cursor.moveToFirst()); |
||||
|
||||
cursor.close(); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<Boolean> addDownloadIfNotExists(@NonNull final DownloadItem item) { |
||||
return Single.create(new SingleAction<Boolean>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<Boolean> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_DOWNLOADS, null, KEY_URL + "=?", new String[]{item.getUrl()}, null, null, "1"); |
||||
|
||||
if (cursor.moveToFirst()) { |
||||
cursor.close(); |
||||
subscriber.onItem(false); |
||||
subscriber.onComplete(); |
||||
return; |
||||
} |
||||
|
||||
cursor.close(); |
||||
|
||||
long id = lazyDatabase().insert(TABLE_DOWNLOADS, null, bindBookmarkToContentValues(item)); |
||||
|
||||
subscriber.onItem(id != -1); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Completable addDownloadsList(@NonNull final List<DownloadItem> bookmarkItems) { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull CompletableSubscriber subscriber) { |
||||
lazyDatabase().beginTransaction(); |
||||
|
||||
for (DownloadItem item : bookmarkItems) { |
||||
addDownloadIfNotExists(item).subscribe(); |
||||
} |
||||
|
||||
lazyDatabase().setTransactionSuccessful(); |
||||
lazyDatabase().endTransaction(); |
||||
|
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<Boolean> deleteDownload(@NonNull final DownloadItem bookmark) { |
||||
return Single.create(new SingleAction<Boolean>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<Boolean> subscriber) { |
||||
int rows = lazyDatabase().delete(TABLE_DOWNLOADS, KEY_URL + "=?", new String[]{bookmark.getUrl()}); |
||||
|
||||
subscriber.onItem(rows > 0); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Completable deleteAllDownloads() { |
||||
return Completable.create(new CompletableAction() { |
||||
@Override |
||||
public void onSubscribe(@NonNull CompletableSubscriber subscriber) { |
||||
lazyDatabase().delete(TABLE_DOWNLOADS, null, null); |
||||
|
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@NonNull |
||||
@Override |
||||
public Single<List<DownloadItem>> getAllDownloads() { |
||||
return Single.create(new SingleAction<List<DownloadItem>>() { |
||||
@Override |
||||
public void onSubscribe(@NonNull SingleSubscriber<List<DownloadItem>> subscriber) { |
||||
Cursor cursor = lazyDatabase().query(TABLE_DOWNLOADS, null, null, null, null, null, null); |
||||
|
||||
subscriber.onItem(bindCursorToDownloadItemList(cursor)); |
||||
subscriber.onComplete(); |
||||
} |
||||
}); |
||||
} |
||||
|
||||
@Override |
||||
public long count() { |
||||
return DatabaseUtils.queryNumEntries(lazyDatabase(), TABLE_DOWNLOADS); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,97 @@
@@ -0,0 +1,97 @@
|
||||
package acr.browser.lightning.database.downloads; |
||||
|
||||
import android.support.annotation.NonNull; |
||||
import android.support.annotation.WorkerThread; |
||||
|
||||
import com.anthonycr.bonsai.Completable; |
||||
import com.anthonycr.bonsai.Single; |
||||
|
||||
import java.util.List; |
||||
|
||||
/** |
||||
* The interface that should be used to |
||||
* communicate with the download database. |
||||
* <p> |
||||
* Created by anthonycr on 5/6/17. |
||||
*/ |
||||
public interface DownloadsModel { |
||||
|
||||
/** |
||||
* Determines if a URL is associated with a download. |
||||
* |
||||
* @param url the URL to check. |
||||
* @return an observable that will emit true if |
||||
* the URL is a download, false otherwise. |
||||
*/ |
||||
@NonNull |
||||
Single<Boolean> isDownload(@NonNull String url); |
||||
|
||||
/** |
||||
* Gets the download associated with the URL. |
||||
* |
||||
* @param url the URL to look for. |
||||
* @return an observable that will emit either |
||||
* the download associated with the URL or null. |
||||
*/ |
||||
@NonNull |
||||
Single<DownloadItem> findDownloadForUrl(@NonNull String url); |
||||
|
||||
/** |
||||
* Adds a download if one does not already exist with |
||||
* the same URL. |
||||
* |
||||
* @param item the download to add. |
||||
* @return an observable that emits true if the download |
||||
* was added, false otherwise. |
||||
*/ |
||||
@NonNull |
||||
Single<Boolean> addDownloadIfNotExists(@NonNull DownloadItem item); |
||||
|
||||
/** |
||||
* Adds a list of downloads to the database. |
||||
* |
||||
* @param downloadItems the downloads to add. |
||||
* @return an observable that emits a complete event |
||||
* when all the downloads have been added. |
||||
*/ |
||||
@NonNull |
||||
Completable addDownloadsList(@NonNull List<DownloadItem> downloadItems); |
||||
|
||||
/** |
||||
* Deletes a download from the database. |
||||
* |
||||
* @param download the download to delete. |
||||
* @return an observable that emits true when |
||||
* the download is deleted, false otherwise. |
||||
*/ |
||||
@NonNull |
||||
Single<Boolean> deleteDownload(@NonNull DownloadItem download); |
||||
|
||||
/** |
||||
* Deletes all downloads in the database. |
||||
* |
||||
* @return an observable that emits a completion |
||||
* event when all downloads have been deleted. |
||||
*/ |
||||
@NonNull |
||||
Completable deleteAllDownloads(); |
||||
|
||||
/** |
||||
* Emits a list of all downloads |
||||
* |
||||
* @return an observable that emits a list |
||||
* of all downloads. |
||||
*/ |
||||
@NonNull |
||||
Single<List<DownloadItem>> getAllDownloads(); |
||||
|
||||
/** |
||||
* A synchronous call to the model |
||||
* that returns the number of downloads. |
||||
* Should be called from a background thread. |
||||
* |
||||
* @return the number of downloads in the database. |
||||
*/ |
||||
@WorkerThread |
||||
long count(); |
||||
} |
Loading…
Reference in new issue